CSC/ECE 517 Spring 2021 - E2115. Mentor management for assignments without topics
Team Members
- Yi Qiu
- Ryan Smith
- Jose Molina Melendez
- Bahati Wanza
Video walk through
5 min video explanation
Problem Statement
Currently, Expertiza has no way to associate mentors with teams. For assignments with topics, like the OSS project, mentors are associated with topics, and then whichever team is assigned to the topic inherits the mentor for that topic However, for assignments without topics (like Program 2), there is no good way to “automatically” assign mentors to projects. The instructor needs to watch teams being formed, and every time a new team is formed, a new mentor needs to be assigned, outside of Expertiza. This leads to a lot of work for the instructor, as well as sometimes long delays before a team is assigned a mentor.
The Plan
For assignments without topics, mentors should be assigned to teams. Assume that things happen in the following order:
- An assignment without topics is created that requires teams of size of up to k.
- Students sign up for teams.
- When a team reaches a target size of greater than 50% of the maximum teammate capacity, then:
- A mentor will be assigned to this team and notified via email.
- Participants in an assignment will be identified as mentors via their participant permissions (see the E1963 documentation). This adds a fourth, “mentor” permission to the existing three permissions (“submit”, “review”, and “take quiz”). Anyone with “mentor” permission for an assignment is eligible to be automatically assigned to mentor a team when a new team is formed.
What needs to be done
Develop a trigger that:
- Is activated when any team has been formed that has k members, where k is greater than 50% of the maximum team capacity
- ex: max members = 4, trigger activated when the team size reaches 3
- Assign a mentor to the team
- Mentors should be evenly assigned to teams, so a good strategy is to assign the mentor who has the fewest teams to mentor so far.
- Notify the mentor via email that they are now assigned to a specific team, and provide the email addresses of the team members.
- Possibly notify the team members that they have been assigned the mentor with contact information (further discussion here).
Preconditions
The mentor assignment feature has a few preconditions and assumptions which have been outlined below.
- An instructor has already added an assignment without topics.
- “mentor” permission exist
- There are users in said assignment with mentor permissions.
- A mentor has yet to be assigned to said assignment.
- Mentor assignment is done only once, does not take into consideration drop offs.
Workflow Diagram
The main workflow of the mentor management for assignments without topics is outline below.
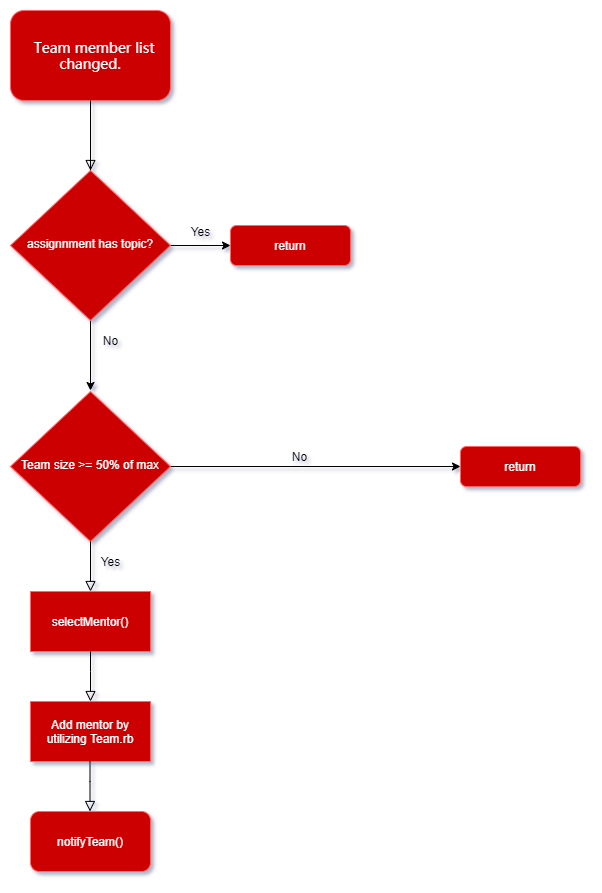
Logic Design
The following main logic components are described below. Each block of logic is to be independent from one another, in order to avoid DRY and de-couple code responsibility.
selectMentor()
In order to assign a mentor to a group, we must first choose a mentor wisely in the system. The requirements document suggests "Mentors should be evenly assigned to teams, so a good strategy is to assign the mentor who has the fewest teams to mentor so far." This can be implemented using a min-heap-based priority queue, where a mentor's priority is the count of the number of teams to which they're assigned.
updateMentorStates()
The responsibility of this function will be to check if the team satisfy the conditions for the auto assign of mentor. This method will utilize the selectMentor() if the conditions are met. It will use the existing methods in the Team.rb that coded by expertiza team to add the mentor.
notifyTeam()
This is going to be a simple function that takes care of sending an email to a) the mentor, and b) the project teammates to:
- Notify the team they have been assigned a mentor and pass along their contact information
- Pass along the contact information for the team members to the mentor
UI Design
A simplistic UI design approach is to be implemented. Below is a mock rendering of the new information that will appear on the student_teams/view?student_id=
expertiza page. The value will be only present if the mentor has been automatically assigned.
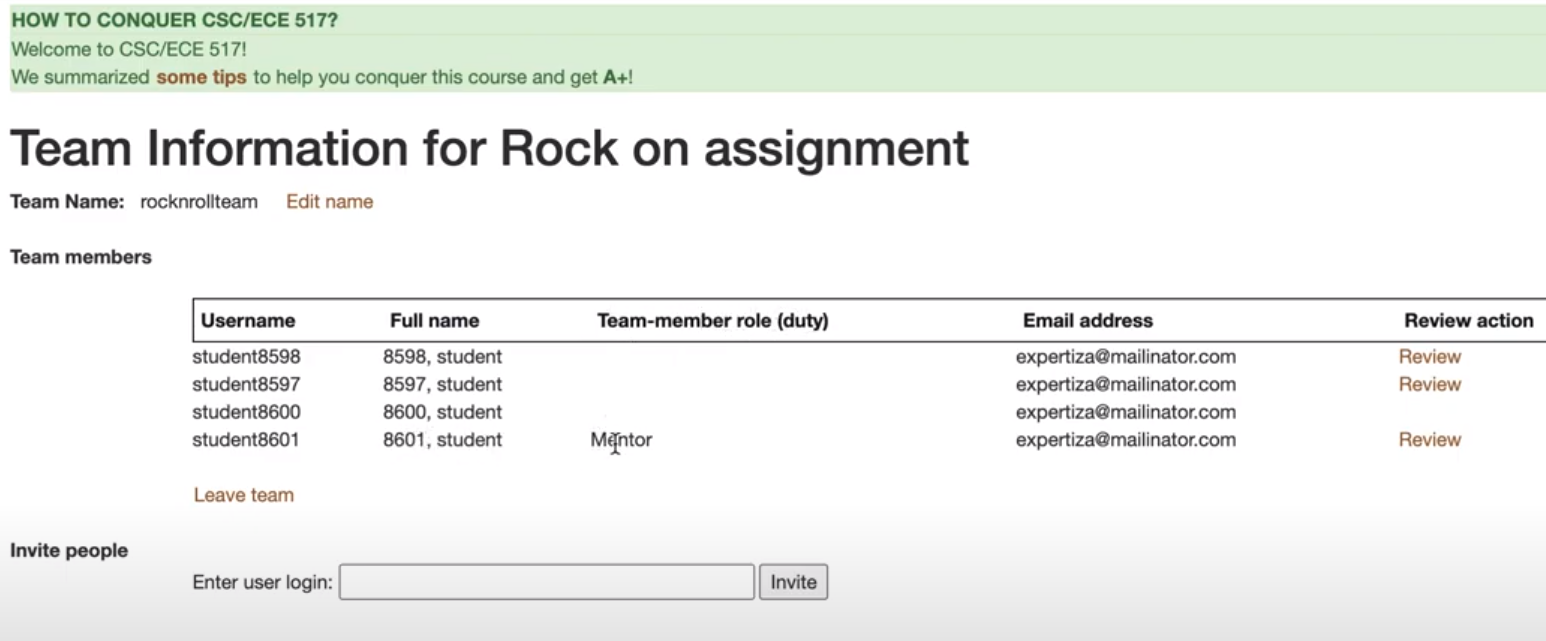
The instructor view will also need to have visibility of what mentors the teams have. Below is a mock rendering of the new information that will appear on the /teams/list?type=Course&id=
expertiza page. The role column will be added.
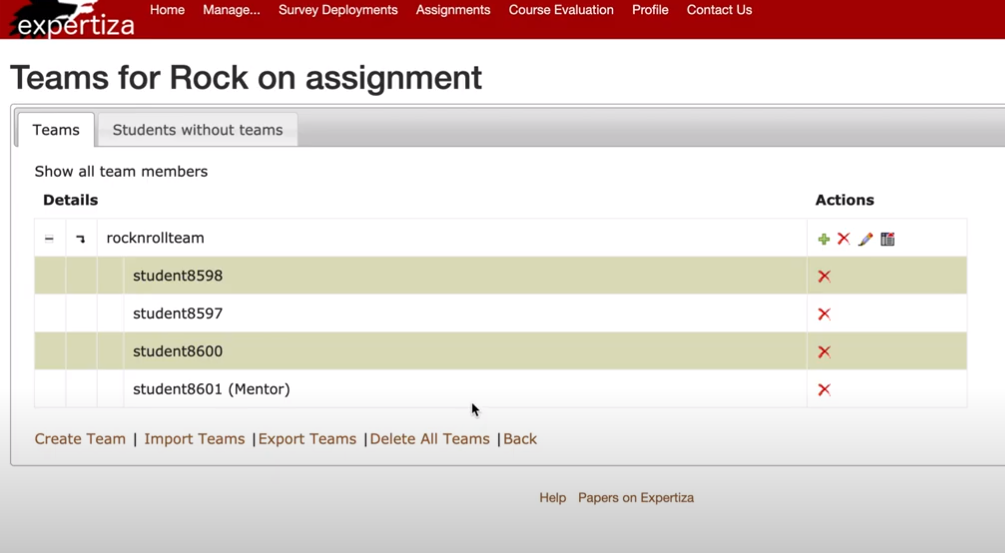
Code Modifications
Controllers
Files
Code Snippets
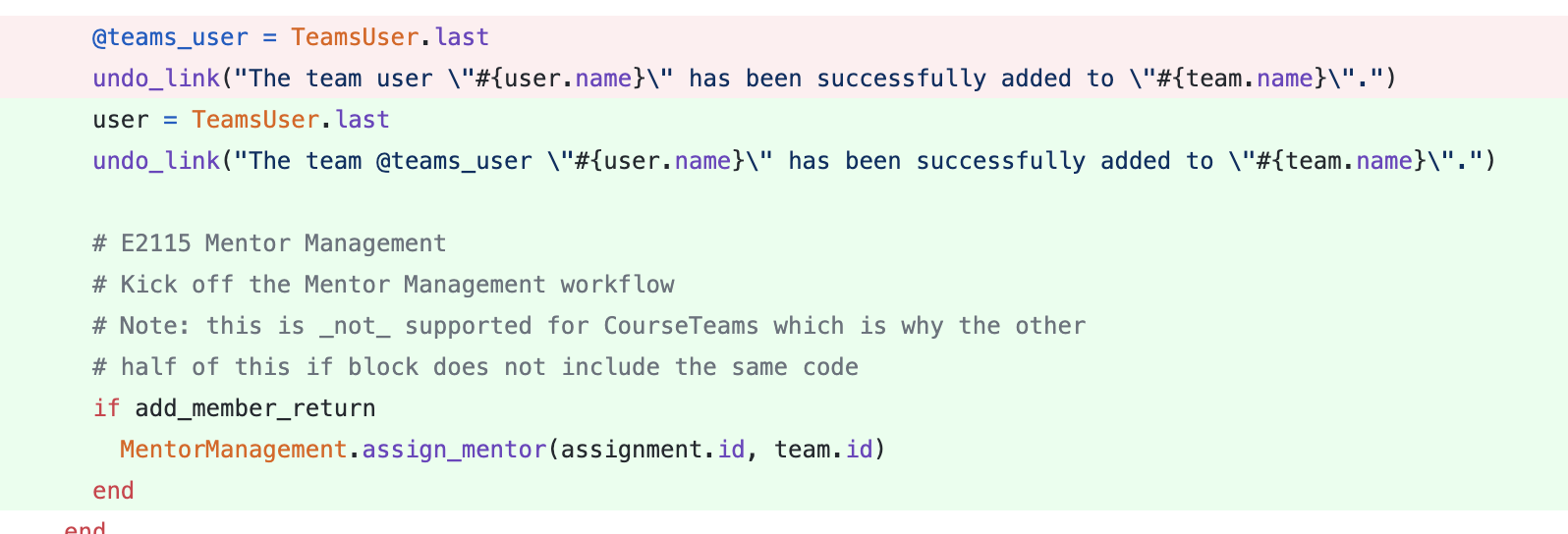
Explanation
This code will Kick off the Mentor Management workflow from the perspective of the instructor when adding members. This is not supported for CourseTeams which is why the other half of this if block does not include the same code. This code can be further enhanced by consolidating where members get added to a single place.
Models
Files and Code Snippets
- app/models/participant.rb
- app/models/mentor_management.rb
- app/models/assignment_form.rb
- app/models/invitation.rb
Explanation
participant.rb
A string constant called DUTY_MENTOR was added to the Participant model, since the duty column of the participants table was used to hold this title. The constant name is prefixed with DUTY_ in the event that other duty titles are added in the future.
mentor_management.rb
Lastly the mentor management class is the main logic for the feature. This class essentially implements the Singleton pattern, but rather than using the Singleton
module it is just a set of class methods.
#select_mentor
The select mentor method selects a mentor using the following algorithm:
- Find all assignment participants for the assignment with id [assignment_id] whose duty is the same as [Particpant#DUTY_MENTOR].
- Count the number of teams those participants are a part of, acting as a proxy for the number of teams they mentor.
- Return the mentor with the fewest number of teams they're currently mentoring.,This method's runtime is O(n lg n) due to the call to, Hash sort_by. This assertion assumes that the database management system is capable of fetching the required rows at least as quickly.
#assign_mentor
The auto_assign_mentor method is the main entrypoint in to the mentor management workflow.
In Expertiza, team members can be added in two ways:
- A user accepts an invitation to join a team
- A user with high enough privileges (e.g. an instructor) manually adds a user to a team
These two events happen in two different places in the code: invitation.rb and teams_controller.rb respectively. In both cases, assign_mentor is called.
The following preconditions must be met for a mentor to be added to a team:
- The assignment must have the auto_assign_mentor flag enabled (i.e. set to
true
) - Neither the assignment nor the team can have topics assigned to them
- The newly added team member must increase the team size to strictly greater than 50% of the maximum team capacity for the assignment
- The team cannot already have a mentor assigned to the team
If these conditions are met, assign_mentor calls #select_mentor to choose a mentor from the pool of mentors, and then assigns them to the team. If this is successful then a notification email is sent out to all current team members, and the new mentor at the same time so everyone knows how to contact everyone else.
assignment_form.rb
The assignment form needed a new :auto_assign_mentor flag added to its model. This will allow for the auto assign mentor feature to be disabled if the instructor does not want the work flow executed for the assignment.
invitation.rb
One trigger had to be added to invitation.rb so when users accept invitations to join a team the mentor management logic can execute.
Views
Files and Code Snippets
- app/views/assignments/edit/_general.html.erb
- app/views/student_teams/view.html.erb
- app/models/teams_user.rb
Explanation
Two views were updated in the Expertiza application to allow the user to see who the mentor is on their team. The Expertiza application makes use of plenty of view partials to compose the UI. We tried to find the least intrusive place among the pile of view partials where we could add a new column for displaying the duty title for mentors. This also had to be done in two places, since the instructor view of a team is different than the student's view of the same team. For the former, teams_user.rb was updated to append (Mentor)
to a user's name in the view.
Database
Files
Code Snippets

Explanation
Our team tried to avoid any database changes, but in order to add a flag for disabling the mentor management functionality we added a new boolean column to the assignments table called auto_assign_mentor.
Test Files
Files
- Update the assignment factory to include a default value for the new auto_assign_mentor flag for testing.
- Update invitation_spec.rb to resolve failing tests after the addition of code to trigger mentor management in invitation.rb.
- Implement tests for the
MentorManagement
class.
Manual Testing instruction
- Login as instructor6, using 'password' as password and find the Rock on Assignment
- Check the paticipants for this assignment, make sure there are some students attempting this assignment.
- You can add your own students to the assignment if you want.
- Impersonate the student account you found.If there is no team, create one by inviting other student to your team, or create a team using the admin control.
- By toggling the Auto assign mentors when team hits > 50% capacity? in the assignment configuration page you will be able to:
- Enable the functionarities and logics that our team implemented.
- Disable the functionarities and logics that our team implemented to allow an admin to assign mentor manully.
Two Observations (Trigger Enabled)
- Add team members to any team under the Rock on Assignment using any following way:
- Invitate/Accept the team invitation using impersonated student accounts.
- Add student to team by using the admin control.
- Observe that when the member size passing the capacity (3 for this Rock on assignment), a mentor called Student6801 will be added to the assignment team.
- Remove the mentor and the added students of the assignment team using the admin control.
- Observe that when the member size below the capacity (e.g. the second team member added), a mentor will not be added to the assignment team.
- You should also be able to see that mentor is showing on the team list.
Two Observations (Trigger Disabled)
- By doing similar step as we mentioned above, you should not see any of the following:
- A mentor is added to the team after the team size changed.
- A mentor is displaying on the team list unless this mentor account: Student6801 is manually added or invited.
Test Plan
Based on the the workflow diagram we propose, we should be including a minimum of 5 tests: 1 for addMentor
, 1 for removeMentor
, 1 for selectMentor
, and 2 more to exercise the paths through our workflow diagram.
- A team will have a mentor after
addMentor
is called. - A team will have a mentor removed after
removeMentor
is called. - The mentor with lowest number of team assigned will be return after
selectMentor
is called. - The
selectMentor
except not to receive any message if a team already has a mentor. - The
selectMentor
except not to receive any message if a team has size < 50% of the maximum size.