Object-Relational Mapping
Object-relational Mapping (ORM, O/RM, and O/R mapping) in computer science is a programming technique for converting data between incompatible type systems in object-oriented programming languages. This creates, in effect, a "virtual object database" that can be used from within the programming language. There are both free and commercial packages available that perform object-relational mapping, although some programmers opt to create their own ORM tools.Object code is written in object-oriented programming (OOP) languages such as Java, Ruby, C++ or C#. ORM converts data between type systems that are unable to coexist within relational databases and OOP languages.<ref name=techopedia>Techopedia,Object-Relational Mapping</ref>
Overview
In Object-oriented programming, data management tasks act on Object-oriented (OO) objects that are almost always non-scalar values. For example, consider an address book entry that represents a single person along with zero or more phone numbers and zero or more addresses. This could be modeled in an object-oriented implementation by a "Person object" with attributes/fields to hold each data item that the entry comprises: the person's name, a list of phone numbers, and a list of addresses. The list of phone numbers would itself contain "PhoneNumber objects" and so on. The address book entry is treated as a single object by the programming language ( It can be referenced by a single variable containing a pointer to the object, for instance). Various methods can be associated with the object, such as a method to return the preferred phone number, the home address and so on.
However, many popular database products such as Structured Query Language(SQL) Database Management Systems (DBMS) can only store and manipulate scalar values such as integers and strings organized within tables. The programmer must either convert the object values into groups of simpler values for storage in the database (and convert them back upon retrieval), or only use simple scalar values within the program. Object-relational mapping is used to implement the first approach.
The core problem is translating the logical representation of the objects into an atomized form that is capable of being stored in the database, while preserving the properties of the objects and their relationships so that they can be reloaded as objects when needed. If this storage and retrieval functionality is implemented, the objects are said to be persistent.
ORM Architecture and Framework
Why to use ORM framework?
ORM framework were designed to reduce the amount of work needed to develop an object-oriented system that stores data in a relational database. The structure of in-memory objects does not match up well with the structure of data in relational databases, and it can be a slow and repetitive process to develop all the code that is required to move the information between the object space and the relational space. <ref name=Frameworks>introduction to ColdFusion frameworks</ref>
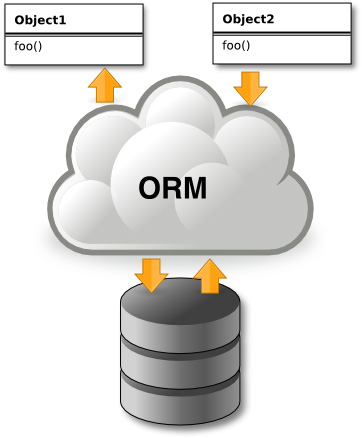
What goal to use ORM framework?
The goal of a ORM framework is to automatically map objects to and from a database seamlessly so that information can be quickly and easily retrieved in object format, and then subsequently stored in its relational structure. This aids in system development and maintenance greatly. The mundane details of writing your own create, read, update, and delete functions are taken care of for you, so you can concentrate on designing and building your application.
Consider a project in which the application configuration is working smoothly. Your Model, View, and Controller logic is separated wonderfully, and your dependency injection framework is handling your CFC relationships. The annoying thing is, you’re spending three quarters of your time writing boring SQL statements to put data in the database, take it out, and edit it. Writing that SQL and matching it with CFCs that represent the data makes developing your application really slow, and maintaining it requires you to make numerous changes just for something simple, such as adding a column to a table.
If you use a ORM framework, things are far easier. You don’t have to write the SQL to do create, read, update, and delete operations anymore — it is generated for you. Pulling information from the database is as simple as requesting a CFC that represents it from the framework. To update that data, you simply pass that CFC back to the framework, which manages it for you. Suddenly you find that you are spending far more time working on how your application is meant to function and how to design it, rather than the mundane details of pushing and pulling data from your database.
The below figure illustrates what occurs in the mapping layer. The yellow lines show the mapping. Note, however, that the mapping lines do not connect directly to the color swatch objects. This represents that the join involving the "Size Color" table is creating the reference from the size objects to the color swatch objects.<ref>Service Architecture-Mapping Layer</ref>
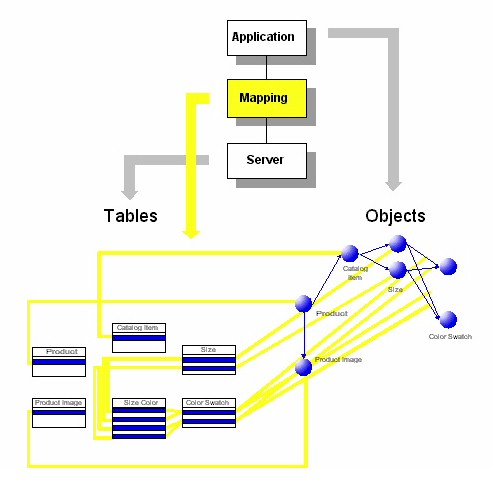
What does an ORM framework do?
ORM framework generates objects that virtually map the tables in a database. As a programmer, you would use these objects to interact with the database. So the main idea, is to try and shield the programmer from having to write optimized SQL code – the ORM generated objects take care of that for you. So let’s take a look at a simple example:
Say for instance you had a database with two tables:
- Clients
- Products
With a little bit of configuration on your part, the ORM framework would create corresponding objects (say, clients_object and products_object) that would handle all the database interaction. So let’s say you need to add a new client to the database, you would just have to use the ORM’s clients_object to add the new client. For example, it could be as simple as calling the object’s ‘save()’ method:
client = new clients_object("Stefan","Mischook"); client.save();
The above of code, is just pseudo code, mainly because the syntax will vary from ORM framework and from language to language. But hopefully you get the general idea of how much easier an ORM framework can make things.<ref name=framework>What are ORM Frameworks?, http://www.killerphp.com/articles/what-are-orm-frameworks/#sthash.xY8Xrpj9.dpuf</ref>
In other words, instead of something like this:
String sql = "SELECT ... FROM persons WHERE id = 10" DbCommand cmd = new DbCommand(connection, sql); Result res = cmd.Execute(); String name = res[0]["FIRST_NAME"];
When to use an ORM framework?
From my personal experience, an ORM framework becomes more useful as the size and complexity of the project increases. If you just have a simple database with say 5 tables and 5-6 queries … setting up an ORM framework may be overkill. I would start considering the use of ORM when:<ref name=framework/>
- You have 3 or more programmers on a web application.
- Your database consist of 10+ tables.
- You have say 10+ queries to make.
How does Object-Relational Mapping work?
<ref name=serviceArch>Service Architecture,Transparent Persistence in Object-Relational Mapping</ref> In object-relational mapping products, the ability to directly manipulate data stored in a relational database using an object programming language is called transparent persistence. This is in contrast to a database sub-language used by embedded SQL or a call interface used by ODBC or JDBC. See transparent persistence vs. JDBC call-level interface.
Using an object-relational mapping product means you will have less code to write and, depending on how you use your data, you might have higher performance over an embedded SQL or a call interface. See caching for object-relational mapping.
With transparent persistence, the manipulation and traversal of persistent objects is performed directly by the object programming language in the same manner as in-memory, non-persistent objects. This is achieved through the use of intelligent caching as this animation shows. For coding examples, see how to access data in a relational database.
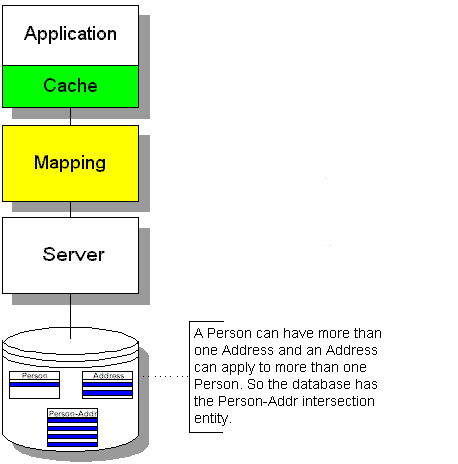
Comparison with Traditional Methods
Compared to traditional techniques of exchange between an object-oriented language and a relational database, ORM often reduces the amount of code that needs to be written.<ref>Douglas Barry, Torsten Stanienda, "Solving the Java Object Storage Problem," Computer, vol. 31, no. 11, pp. 33-40, Nov. 1998, http://www2.computer.org/portal/web/csdl/doi/10.1109/2.730734, Excerpt at http://www.service-architecture.com/object-relational-mapping/articles/transparent_persistence_vs_jdbc_call-level_interface.html. Lines of code using O/R are only a fraction of those needed for a call-level interface (1:4). For this exercise, 496 lines of code were needed using the ODMG Java Binding compared to 1,923 lines of code using JDBC.</ref>
Disadvantages of O/R mapping tools generally stem from the high level of abstraction obscuring what is actually happening in the implementation code. Also, heavy reliance on ORM software has been cited as a major factor in producing poorly designed databases.<ref>Josh Berkus, "Wrecking Your Database", Computer, Aug. 2009, http://it.toolbox.com/blogs/database-soup/wrecking-your-database-33298, Webcast at http://www.youtube.com/watch?v=uFLRc6y_O3s</ref>
Simple Example with ORM
<ref name=sample>StackOverFlow,What is an Object-Relational Mapping Framework?</ref>
String sql = "SELECT ... FROM persons WHERE id = 10" DbCommand cmd = new DbCommand(connection, sql); Result res = cmd.Execute(); String name = res[0]["FIRST_NAME"];
you do something like this:
Person p = repository.GetPerson(10); String name = p.FirstName;
or similar code (lots of variations here.) Some frameworks also put a lot of the code in as static methods on the classes themselves, which means you could do something like this instead:
Person p = Person.Get(10);
Some also implement complex query systems, so you could do this:
Person p = Person.Get(Person.Properties.Id == 10);
See Detailed Sample in Java concerning Objected Relational Mapping at A standardized object-relational mapping mechanism for the Java platform
ORM Language and Main Tools
This table just includes several well-known object-relational mapping tools regarding to some popular programming languages. It is not up-to-date or all-inclusive. <ref name=listOfORM>Wikipedia,List of Object-relational mapping Software</ref>
Name | C++ | .NET | Objective-C | Java | Python | Perl | PHP | Ruby |
---|---|---|---|---|---|---|---|---|
Several Object-Relational Mapping Tools | LiteSQL | ADO.NET Entity Framework | core Data | jOOQ | Django | DBIx::Class | CakePHP | ActiveRecord |
ODB | Nhibernate | Enterprise Objects | Apache_Gora | Peewee ORM | Doctrine | Sequel
| ||
Wt::Dbo | OpenAccess ORM | Hibernate ORM | SQLAlchemy | FuelPHP | DataMapper |
Comparison between ORM tools
The following table lists several well-known object-relational mapping tools <ref name=comparisonOfORM> Wikipedia, Comparison of Object-relational mapping Software</ref>
ORM Tools | Platform | Availablity | Version |
---|---|---|---|
LiteSQL | C++ | Open Source | 0.3.15 / August 10, 2012 |
ODB | C++ | Dual-licensed(GNU GPL/Proprietary License) | 2.3.0 / October 30, 2013 |
Wt::Dbo | C++ | Dual-licensed(GNU GPL/Proprietary License) | 3.3.3 / May 27, 2014 |
ADO.NET Entity Framework | .NET3.5(SP1)+ | Part of .NET 4.0 | EF5.0 |
Nhibernate | .NET2.0(SP1)+ | Open Source | NH3.3.1 |
OpenAccess ORM | .NET2.0+ | Free & Commercial | Version 2012.2.816.1 |
core Data | Objective-C | Commercial | 3.2.0 |
Enterprise Objects Framework | Objective-C | Commercial | WebObjects 5, released in 2001 |
jOOQ | Java Virtual Machine | Dual-licensed(ASL 2.0/Commercial) | 3.3.0 / February 14, 2014 |
Apache_Gora | Java Virtual Machine | Open Source | 0.4 / April 14, 2014 |
Hibernate ORM | Java Virtual Machine | Open Source | 4.2.5 / August 28, 2013 |
Django | Python | Open Source | 1.7 / September 2, 2014 |
Peewee ORM | Python | Open Source | 2.2.3 / April 20, 2014 |
SQLAlchemy | Python | Open Source | 0.9.4 / March 28, 2014 |
DBIx::Class | Perl | Open Source | 0.08270 / January 31, 2014 |
CakePHP | PHP | Open Source | 2.5.4 /September 2, 2014 |
Doctrine | PHP | Open Source | 2.4/April 8, 2014 |
FuelPHP | PHP | Open Source | 1.7.2/ July 13, 2014 |
ActiveRecord | Ruby | Open Source | 4.2.0.beta1/ Aug 20, 2014 |
Sequel | Ruby | Open Source | 4.14.0 / September 1, 2014 |
DataMapper | Ruby | Open Source | 1.2.0/October 13, 2011 |
ORM Advantage and Disadvantage
Advantage
ORM is a rapidly growing and popular methodology that provides clear advantages to the developer: Eliminates the fragility of coding CRUD statements to persist data to and from the database Allows logic, business rules and validation to be introduced to the data Provides domain objects that are easy to customise and manage in code Hides the differences between various databases or data sources Saves vast amounts of coding effort
- Simplified development because it automates object-to-table and table-to-object conversion, resulting in lower development and maintenance costs
- Less code compared to embedded SQL and handwritten stored procedures
- Transparent object caching in the application tier, improving system performance
- An optimized solution making an application faster and easier to maintain<ref name=techopedia/>
Disadvantage
- ORM does not perform well and that stored procedures might be a better solution.
- ORM dependence may result in poorly-designed databases in certain circumstances.<ref name=techopedia/>
- ORM is slower than hand-crafted persistence code that directly interacts with a database API such as JDBC or ADO.NET.
- Most ORM systems have unsatisfactory support for revisions to the architecture of a system after a system has been mapped.
- ORM has yet to see widespread adoption in everyday industrial operations.<ref name=abc>Barnes, Jeffrey M. Object-Relational Mapping as a Persistence Mechanism for Object-Oriented Applications. Diss. Macalester College, 2007.WebCast at http://digitalcommons.macalester.edu/mathcs_honors/6/</ref>
See Also
- Wikipedia, Object-relational Mapping
- Wikipedia, Object-oriented Programming
- Hibernate Overview,What is Object/Relational Mapping?
- Object-relational Mapping Overview
- StackOverFlow,Disadvantage for ORM
- Service Architecture, Object-Relational Mapping Articles
- Object-Relational Mapping Concepts
- Choosing an object-relational mapping tool by Fabrice Marguerie
- Mapping Objects to Relational Databases: O/R Mapping In Detail
- RailsGuides Active Record Basics
- Ruby on Rails official website
- Object-Relational Mapping
- Getting started with Object Relational Mapping (ORM)
- Architecture
- Mapping Objects to Relational Databases: O/R Mapping In Detail
- Object Relational Mapping (ORM) Data Access
References
<references></references>