E1726 - Remove Cache Field in Roles Table
Introduction
Background
Expertiza is an open source web-based peer review system developed using Ruby on Rails. It is a dashboard containing all assignments for a particular course which is managed by the Professors and Teaching Assistants.
It eases the process of selecting assignment topics and choosing team-mates for the projects and greatly reduces the burden on the Professors and Teaching Assistants of assigning and managing teams for each assignment.
The main motive of this project is to make learning more effective through peer review. It allows students to review each other’s work and improve their work upon this feedback. It not only helps one improve their work but also provides a new way to learn.
Students upload or provide URLs of their work on expertiza which would be reviewed by the instructors and peers. Students later get to improve their work based on the reviews provided their peers.
Problem statement : Remove cache field in roles table
The project requires us to remove the cache column from the roles table in database. Expertiza's 'roles' table has a 'cache' column, used by the superfish-rails gem to display different menus for different roles (super-administrator, administrator, instructor, teaching assistant, student, and unregistered user).
There is a Role model which makes use of the long text string data stored in the roles table under the cache column to load the menus (e.g., Users \n Questionnaires \n Courses …) for a particular role at the top of the page after login. Storing such long strings in databases is a bad idea because if we want to change that large text we are required to write a migration file which would be inconvenient and also there is a restriction on the number of characters allowed for long string datatype in MySQL.
Design Plan
The Design plan followed here is as follows:
- Move the cache column value to YAML files for each role.
- Load the YAML value on environment/server startup.
- Use the corresponding YAML values for the corresponding role for the user logging in.
The reason for using this design is to have a pre-defined menu list for each roles. This gives more DRY principle for the application code, instead of going with the new object declaration every time the role model is needed.
Tasks Identified
- Create YAML files containing the cache column data for each role from roles table in database.
- Create YAML files under 'config' folder named 'role_admin.yml' and put the 'cache' column value in it split across rails environment
- Create ruby script under 'config/initializers' folder named 'load_roles.rb' - to load the corresponding YAML values once the environment is set up
- Change the role.rb model to facilitate the use of above defined YAML files
- Remove the cache column from the roles table by migration
- Write rspec unit test for 'role.rb'
Implementation
Created YAML files containing the cache column data for each role from roles table in database
Created YAML files under 'config' folder named 'role_admin.yml' and put the 'cache' column value in it split across rails environment
- 6 new files (role_admin.yml, role_instructor.yml, role_student.yml, role_super_admin.yml, role_ta.yml, role_unreg_user.yml) each corresponding to specific role are created
- Content of cache column from roles table are added for development, production and test environments
Created ruby script under 'config/initializers' folder named 'load_roles.rb' - to load the corresponding YAML values once the environment is set up
This code loads content of "/config/role_admin.yml" file and assigns to CACHED_ADMIN_MENU. Similarly, fields corresponding to different roles are populated from corresponding "/config/role_[ROLE]_.yml" file

Changed the role.rb model to facilitate the use of above defined YAML files
Rebuid_credentials and rebuild_menu method are modified to load content from yml file. Here ApplicationHelper exposes get_cache_roles method to retrieve cache content corresponding to role id.
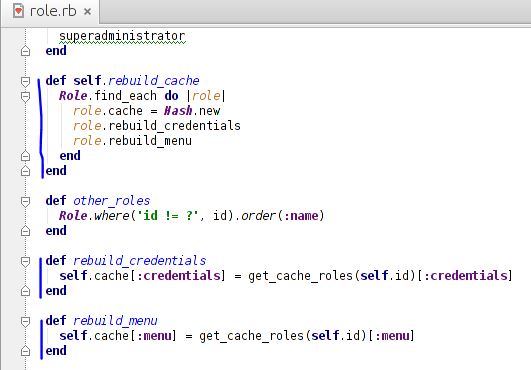
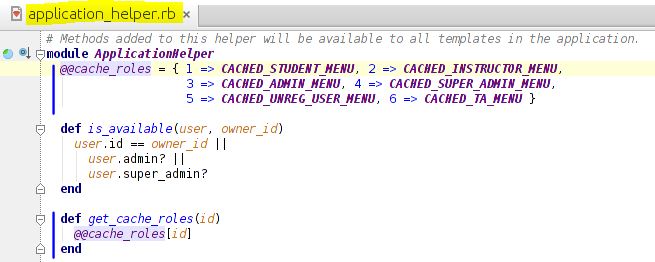
In rebuild_cache method, role.save line is removed as cache field is not saved in database now. Since cache field is removed from roles table, this field is added to role model using attr_accessible. It’s getter and setter are also created
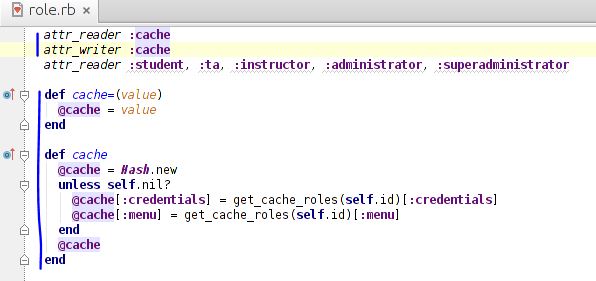
Removed the cache column from the roles table by migration
Before removing cache column in roles table

Roles table after removing cache column

Migration file to drop cache column

Test Plan
The test plan followed here is done across 3 roles namely, student, administrator and instructor. The scenarios covered here are as follows:
- Check for the cache field and see if we get the expected value.
- Check if the role is valid role.
- Check for the cache field mismatch, that is, invalid cache field match.
The sections below points out the implementation steps taken to cover the test plan described above.
Manual Testing
- Login into Expertiza as one of the roles - super-admin or admin or student or instructor
- Check the menu tabs on the top and you can find the respective drop-downs according to the role logged in
- On clicking the option from the drop down you will be able to navigate to the respective pages
- This ensures that the code change done by us didn't break the code and it delivered it's expected functionality
Unit Tests
Unit tests have been written to check whether the modules work as it did earlier before. We have written rspec unit test for ‘role.rb’ which can be found here: ‘spec/models/role_spec.rb’ which tests 10 cases
- Evaluate the cache value with YAML values for student
- Evaluate the cache value with YAML values for instructor
- Evaluate the cache value with YAML values for admin
- Validation of name for student
- Validation of name for instructor
- Validation of name for admin
- Check the mismatch for cache values for the student in factories.rb
- Check the mismatch for cache values for the instructor in factories.rb
- Check the mismatch for cache values for the admin in factories.rb
- An invalid check for role object
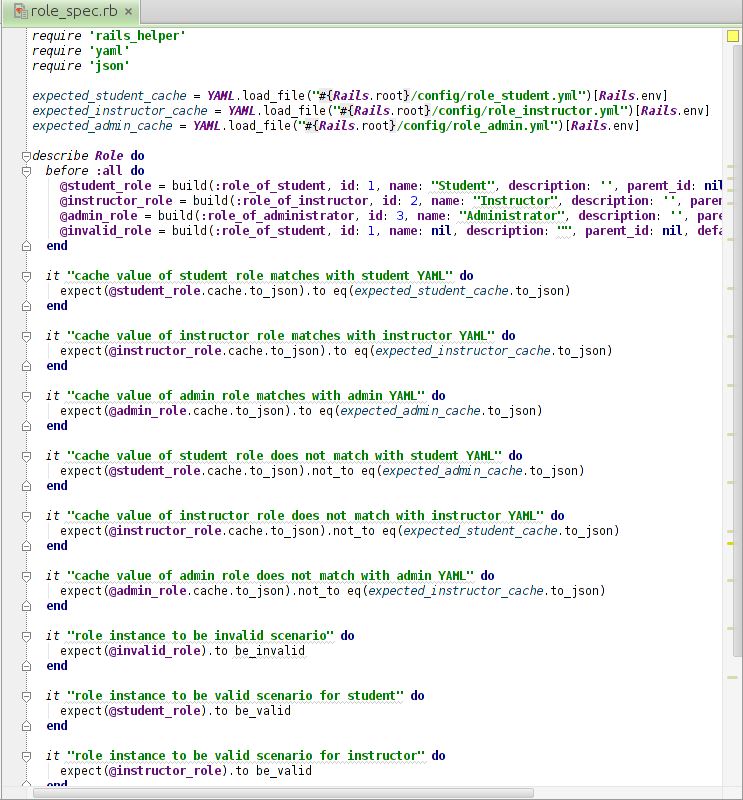
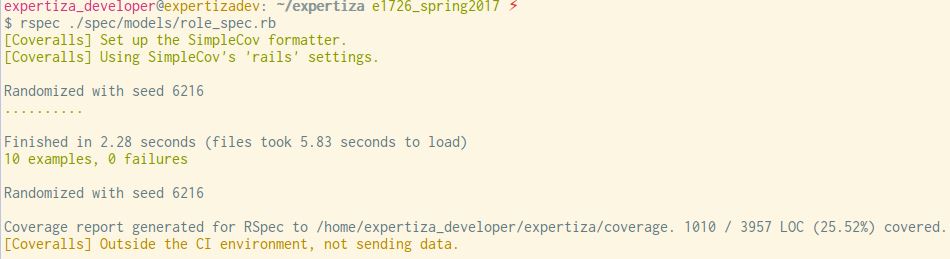
Executing RSpec
The steps required to execute the unit tests as an automated run:
1) cd into the expertiza directory
2) Run the command: rspec spec/models/role_spec.rb
Scope for future improvement
There is a lot of scope for improvement for the current code. For instance, in the present situation, the YAML files have the database data corresponding to menu_items hard coded. We could define YAML file for menu_items table and load the menu_item YAML into the corresponding role YAML during environment setup. This would eradicate the use of menu_item table from the database.