CSC 216/s08/eschew disenchantment
Formatting Resources
Formatting Help Guide from MetaWiki
The Hierarchical Merge Sort
The problem
In this exercise, we want to teach students the mechanics of a merge sort algorithm.
Participants and props
Seven students will participate in the exercise. They will need seven numbered note cards, scotch tape, and a series of hats. There should be seven hats as follows: One master hat, two delegate hats, and four peon hats. The instructor should have the means to display our flash animation and then display the pseudocode during the exercise.
The script
Setup
- There should be a pseudocode description of the merge sort algorithm on a large display.
- The numbered notecards must be taped together in a randomly ordered line.
- Students should be shown our animation of the mergeSort algorithm.
Pseudo Code
public int[] mergeSort(int[] m) {
if (m.length == 1) Return m
if (m.length == 2) Sort m, then return m
Split m in half
Call mergeSort on each half
Merge the two halves after they are sorted and returned (iterative merge of two ordered arrays)
Return the array
}
Activity
First, the seven hats need to be assigned to the seven students. They will have different duties according to their different hats. The king represents the original call to mergeSort, the delegates are the first level of recursion, and the peons are the second level of recursion. Every student has the objective of applying mergeSort to the list they are given, but may behave differently due to the length of their list. Ideally the students will be able to infer what to do from the animation and pseudocode, but they may need hints according to the following sequence:
- The king un-tapes the list at the middle and tells a delegate to sort each half.
- Each delegate un-tapes their list in the middle and gives each half to a peon.
- Each peon should notice that their lists are of size two or fewer, and sort where necessary.
- Each delegate should accept the lists back from their two peons and merge them in order.
- The king should accept the lists back from the delegates and merge them in order.
When it is time for a participant to merge two ordered lists, they may do so without adhering to any algorithm.
Further Illustration
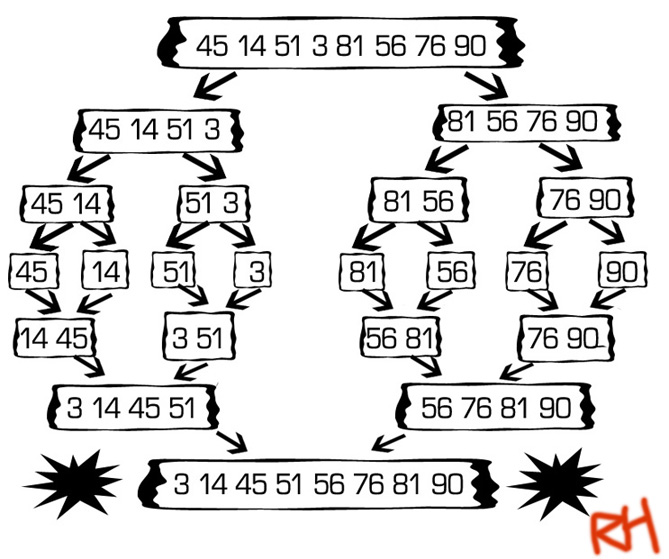