CSC/ECE 517 Spring 2024 - E2438 Improve assessment360 controller
Introduction
The Ruby on Rails framework serves as the foundation for the open-source project Expertiza. Expertiza offers features that let instructors see a comprehensive, 360-degree overview of the course they teach, including all of the assignments, all of the students enrolled in the course, all of their individual assignment scores, averages, and final scores. The assessment360 controller facilitates this feature.
Here is the class diagram of this controller -
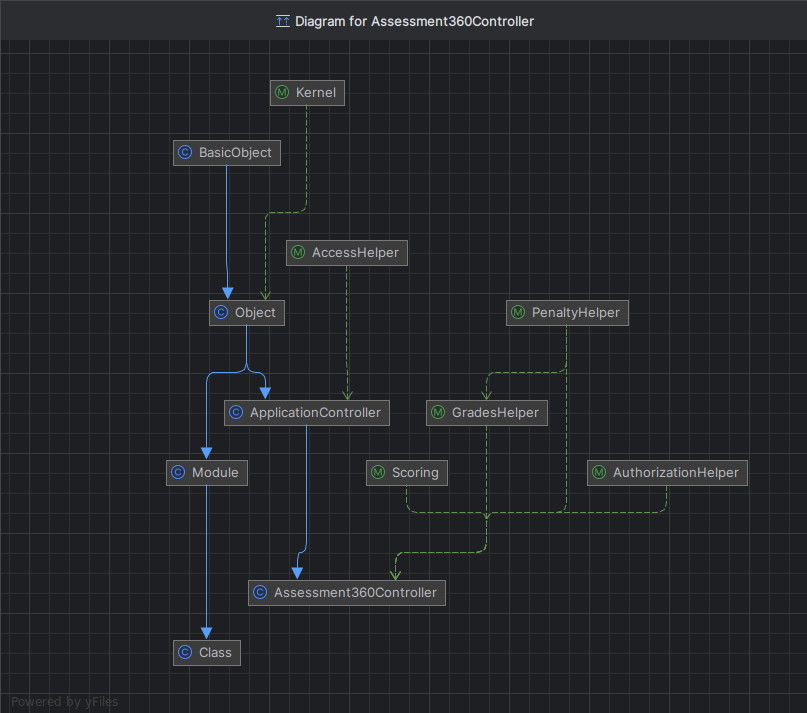
The following two views are mostly under the control of the assessment360 controller:
1) all_students_all_reviews: This view shows all of the student teammate and meta reviews for every program they worked on that semester. It also offers a summary of these review scores collectively.
2) course_student_grade_summary: This view shows the topic, instructor grade, peer score, and summary of each assignment that students have completed. This also applies to the sum and mean of these scores.
Problem Statement
The current implementation of the assessment360_controller.rb suffers from several issues such as repetitive elements, cryptic variable names, inaccurate grade calculations, and lack of flexibility in displaying different types of review scores. The objective of this project is to enhance the user interface and functionality of the assessment360_controller.rb in the application.
Following are the issues to be addressed in this project:
Issues
The main goals of this project are as follows:
- Issue #1: Refactor the all_students_all_reviews method to accept parameters specifying the type(s) of review scores to be displayed, and suppress columns for scores not selected.
- Issue #2: Rename the @teamed_count variable to a more descriptive name or remove it if not used.
- Issue #3: Ensure accuracy in grade calculations by including only the assignments that were attempted by the student in average grade calculation.
- Issue #4: Enhance the course_student_grade_summary method to include average peer-review scores and instructor-assigned scores.
- Issue #5: Columns for various types of review scores should be displayed based on user selection.
- Issue #6: Utilize the Bullet gem to minimize database accesses and optimize performance.
- Issue #7: Identify and address edge cases from the UI perspective to ensure consistent and clean behavior across different scenarios.
- Issue #8: Implement separate columns for student name and student ID to improve readability in the UI.
- Issue #9: Replace hyphen '-' with an en-dash '–'.
Implementation
The changes made to the assessment360_controller.rb and related files were necessary to improve the code's readability, maintainability, and adherence to best practices. Let's discuss each change and its significance:
Files modified
1. Changes to app/views/assessment360/all_student_all_reviews.html.erb
# | Change | Rationale | Commit Link |
---|---|---|---|
1 | Checked and renamed@teamed_count variable
|
Checked the usage of variable through IDE whether the variable is used. The variable was used to display the total number of teams each student has collaborated with. Thus, it was renamed from @teamed_count to @student_team_counts in both app/controller/assessment360_controller.rb and app/views/assessment360/all_student_all_reviews.html.erb files for better readability and understandability of the code.
|
Commit |
2 | Added check boxes to select columns to display | Added 2 check boxes (meta reviews and teammate reviews) to select/deselect columns to be displayed. | Commit |
3 | Hide empty columns | Added checks to ensure column is displayed only when at least one of the rows has a corresponding value to that column in the database. | Commit |
2. Changes to Gemfile
and config/environments/development.rb
# | Change | Rationale | Commit Link |
---|---|---|---|
1 | Added and initialized the bullet gem | To identify and reduce unnecessary database queries, the bullet gem was added and activated to increase the performance of the application. | Commit |
3. Changes to app/controllers/assessment360_controller.rb
# | Change | Rationale | Commit Link |
---|---|---|---|
1 | Replaced hyphen '-' with an en-dash '–' | Since hyphen was used to represent empty values, it was replaced with an en-dash for enhancing the UI. | Commit |
2 | Refactored calc_overall_review_info()
|
Ensured that if a student has not received a grade for an assignment that row is not considered for calculating the average. | Commit |
3 | For each assignment of each participant, fetched and calculated the average peer review scores using the find_peer_review_score method.
|
Tracked the presence of peer review data to manage the display of this data dynamically in the views. | Commit |
4 | Calculated the instructor-assigned grades adjusted for any penalties using the assignment_grade_summary method. | This involves fetching the team associated with each participant and adjusting the grades based on penalties, ensuring accurate grade calculation and storage.
Stored these scores and updated the running totals to calculate the average across the course. |
Commit |
4. Changes to app/views/assessment360/course_student_grade_summary.html.erb
# | Change | Rationale | Commit Link |
---|---|---|---|
1 | Implemented dynamic column visibility in an HTML table using checkboxes. | Each checkbox controls the display of specific table columns related to student assessments, such as Topics, Peer Scores, and Instructor Grades.
This functionality is achieved by toggling a CSS class that either hides or shows these elements, enhancing user interaction by allowing customizable views based on the checkbox selections. |
Commit |
2 | Separated student name and student ID into different columns | In order to achieve better understanding of the data being displayed, student name and student ID were separated into different columns. | Commit |
UI Changes
The assessment360 controller is responsible for the following two views -
1) all_students_all_reviews.html.erb - This view displays a table of students, their teammate count, and their performance on various assignments. For each assignment, it shows the metareview and teammate review scores. At the end of each row, it displays the aggregate score for metareviews and teammate reviews for each student. The last row of the table provides the class average for each assignment and the overall class average for metareviews and teammate reviews.
Before changes:
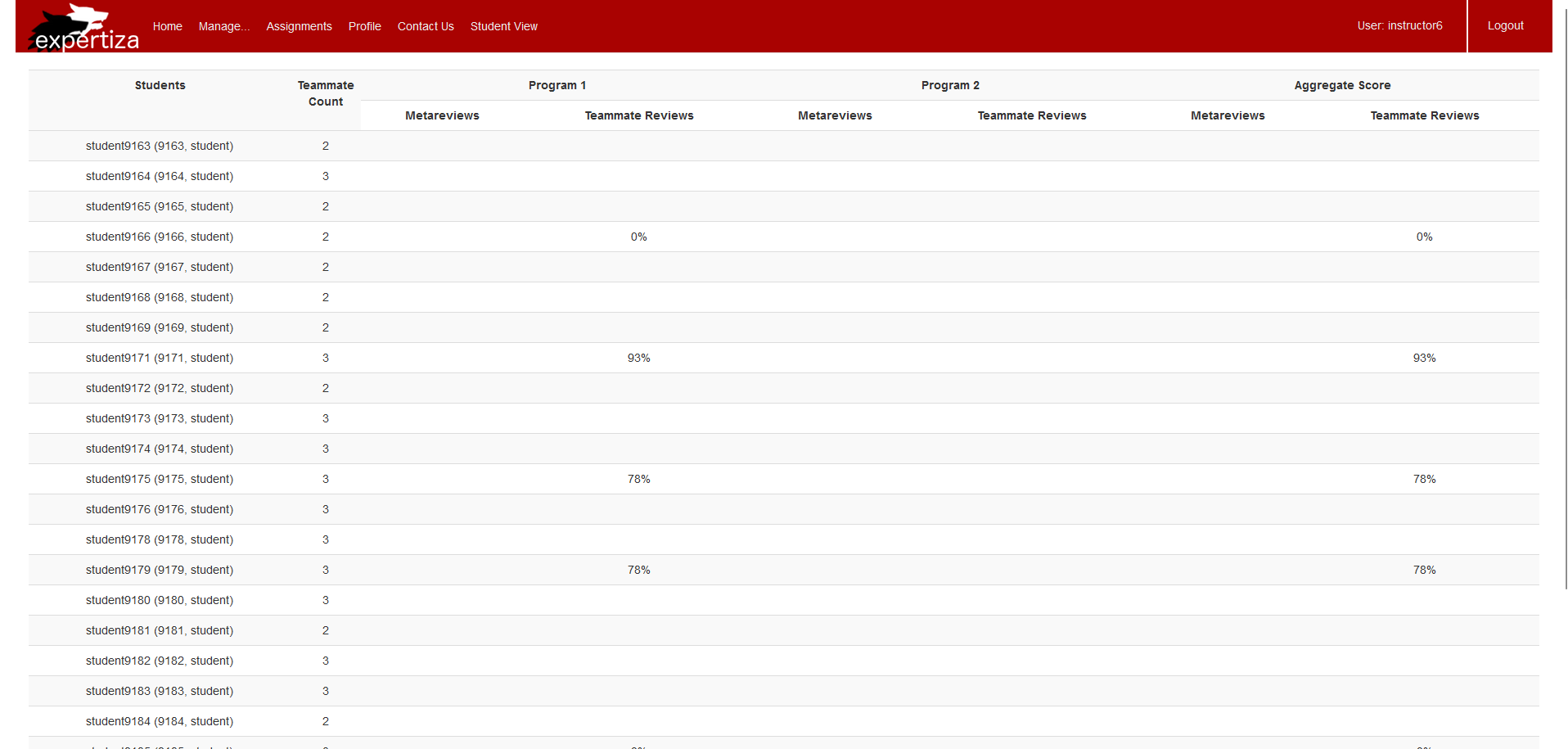
After changes:
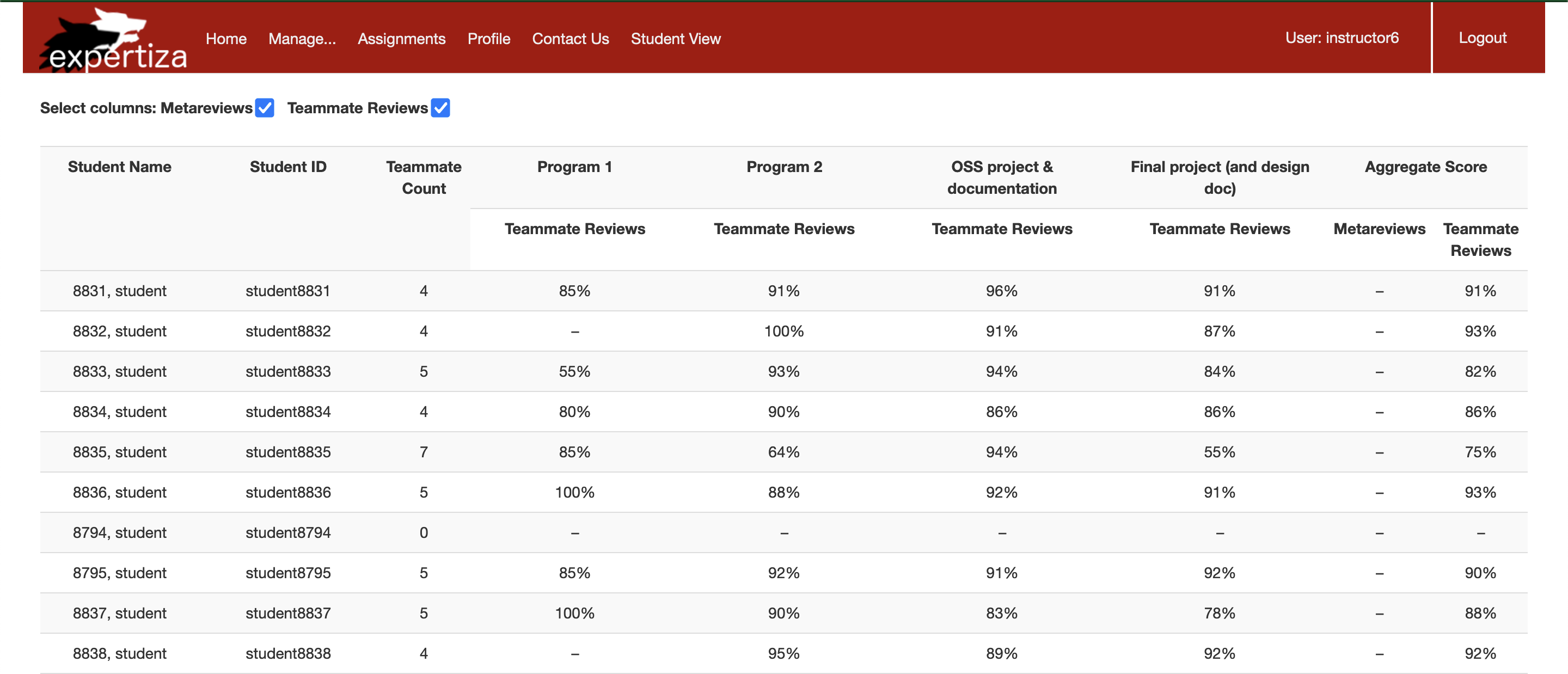
2) course_student_grade_summary.html.erb - This view displays a sortable table of students, their assignments, and their grades. For each assignment, it shows the topic, the peer review score, and the instructor's grade. At the end of each row, it displays the total instructor grade for each student. The table can be sorted by student name or total instructor grade.
Before changes:
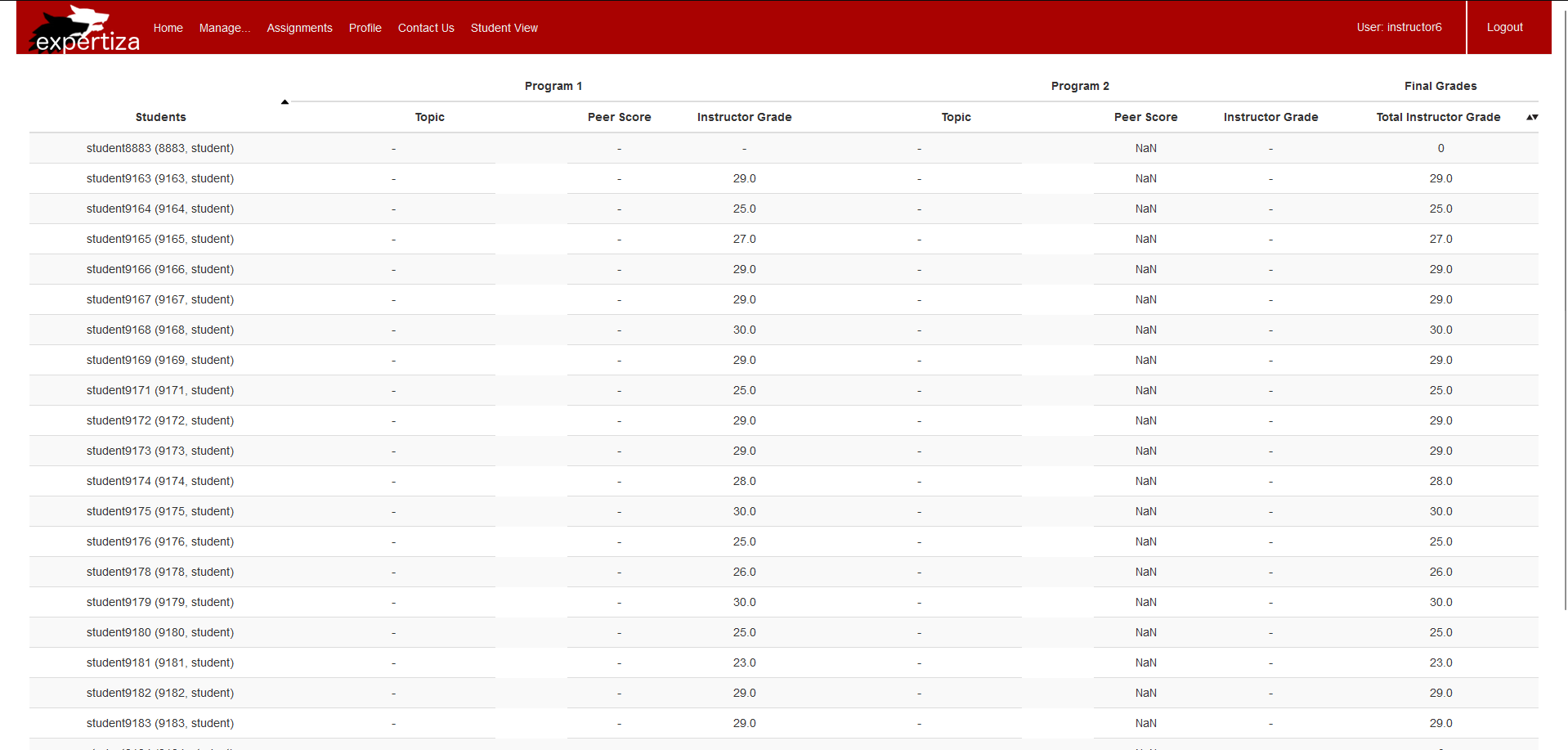
After changes:
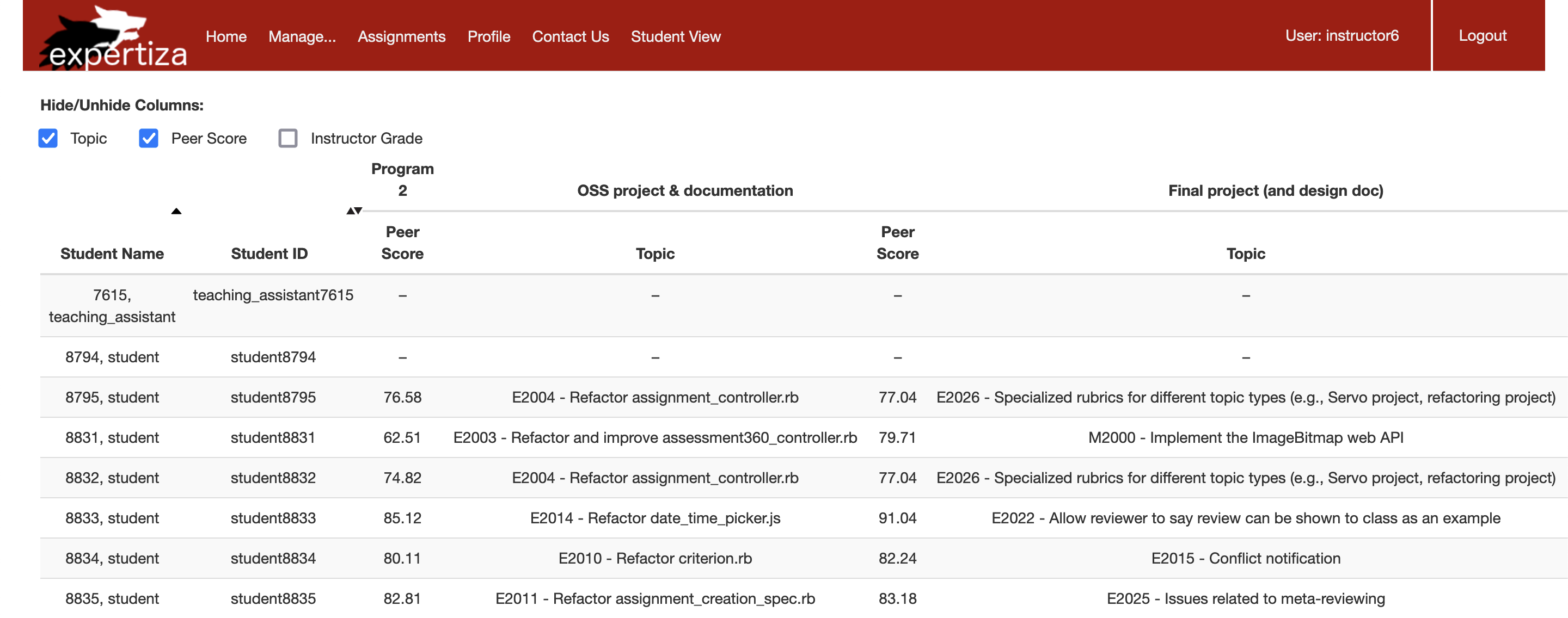
Code Snippets
We created 2 hashes meta_review_exists
and teammate_review_exists
which track if the respective values exist for each assignment. We use these hashes in our view to decide whether to display columns or not.
Here we are assigning values to those hashes based on whether the review exists or not for that particular assignment.
This is the new logic for calculating grade averages ensuring that only those grades are considered which have been reviewed for the average calculation so that the calculation is accurate.
This is the JavaScript code we wrote to ensure that our view changes dynamically based on selection/deselection of the checkboxes.
Changes in assessment360_controller.rb
:
The course_student_grade_summary
method within the Assessment360Controller
has been refactored to adhere to better design principles.
Adding new data structures: New hash data structures such as @number_of_peer_reviews
, @avg_peer_review_score
, and @total_grade
were introduced. This enhances the single responsibility principle, where each hash is now responsible for one piece of data related to student grades and reviews.
Initializing data structures: Previously uninitialized hashes like @assignment_grades_present
, @peer_review_scores_present
, and @assignment_category
are now explicitly initialized to {}. This change improves code clarity, avoids null references, and ensures that the hashes are ready for use.
Ensuring data structure consistency: By ensuring that each hash map is initialized even if no data is present (||= 0), the code now avoids potential nil errors and allows for safer iteration and access patterns.
Refactoring variable initialization: The refactored code uses the ||= operator to provide default values for the hashes if they haven't been initialized yet, improving the robustness and maintainability of the code.
The peer_review_scores
hash is now being correctly populated with the average peer review score, rounded to two decimal places. This change ensures the score is a concise, readable number.
A counter number_of_peer_reviews has been introduced or updated for each course participant (cp.id). This is for tracking the count of peer reviews received.
Instructor-assigned grades are calculated taking into account any penalties. This calculation is stored in assignment_grades[cp.id][assignment_id]
.
The code checks if an instructor grade exists. If so, flags assignment_grades_present
and assignment_category["assignment_grade"]
are set to true and 1 respectively, ensuring that the UI reflects the presence of these grades.
Changes in course_student_grade_summary.html.erb
:
In the course_student_grade_summary.html.erb
file, we've introduced enhancements to the user interface to allow for a more dynamic display of the data table.
New checkbox elements have been added, each linked to a specific column in the data table.
These checkboxes give users the ability to show or hide the columns for topics, peer scores, meta reviews, the number of teammates, and instructor grades.
This feature was implemented to provide users with a more personalized and clutter-free interface, enabling them to focus on the data that is most relevant to their needs at any given time.
Design Principles and Patterns
We have tried to apply the techniques we have learned in the class wherever it was possible. This included DRYing out the code and adding proper comments enhancing its readability and maintainability.
- We created a method called
handleCheckboxChange
which was responsible for listening to various checkbox events, thus helping us to DRY out our code since we did not have to write the same code again for another checkbox.
Test Plan
The following test plan has been identified to ensure that the our changes don't break the existing system and the new code being introduced is thoroughly tested.
Manual Testing
The following tests have been identified to ensure that the changes made work as anticipated:
1. After you login with the provided credentials, select any course
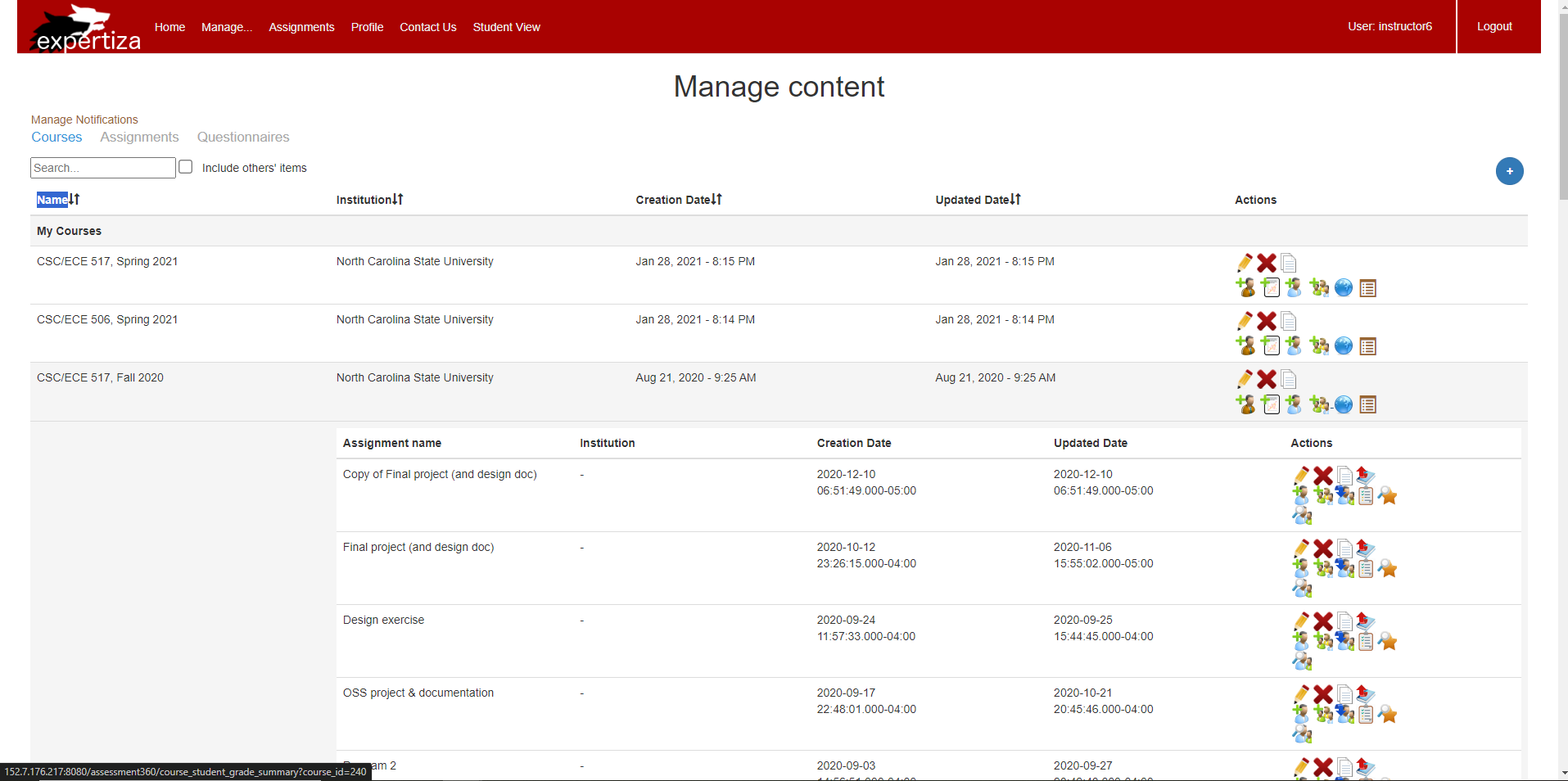
2. Open the all_students_all_reviews page by clicking on the icon next to the globe icon in another tab.
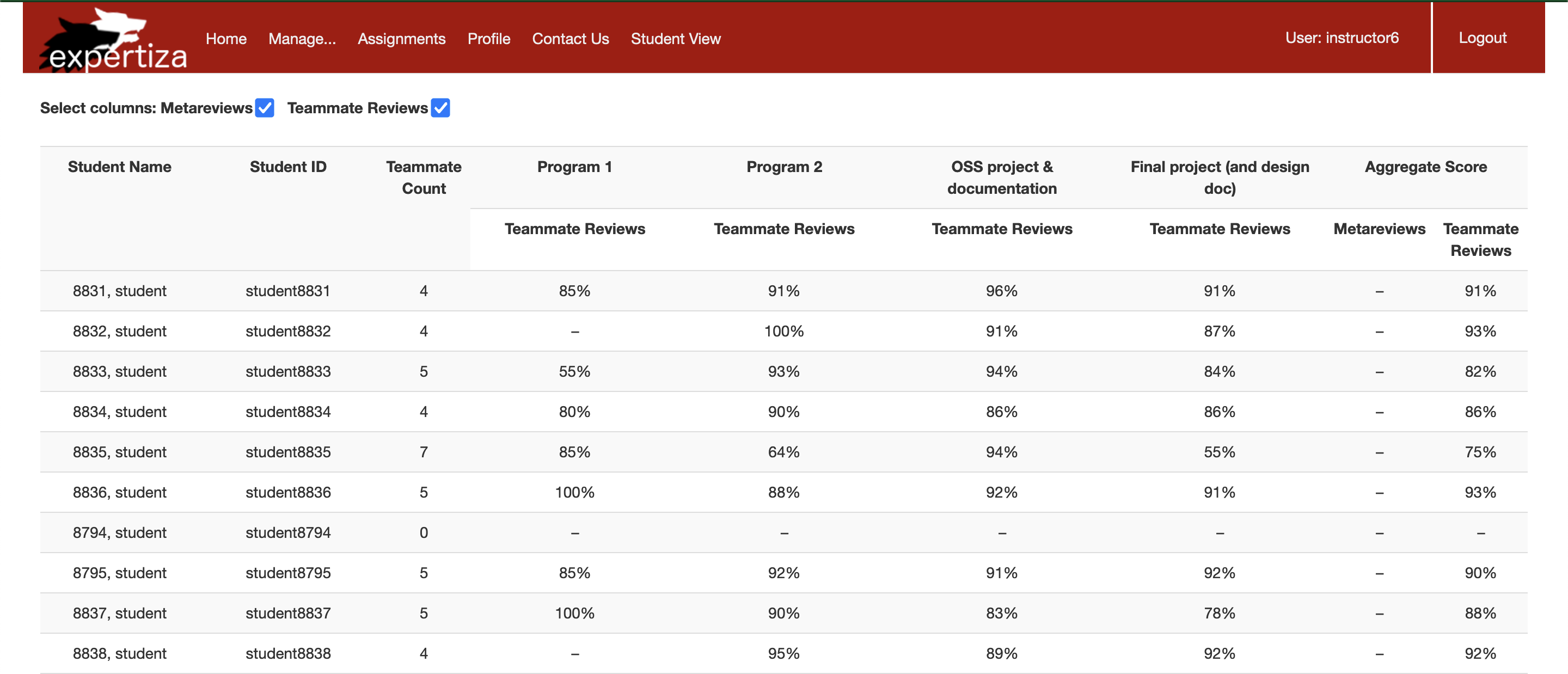
3. Open the course_student_grade_summary page by clicking on the globe icon in new tab.
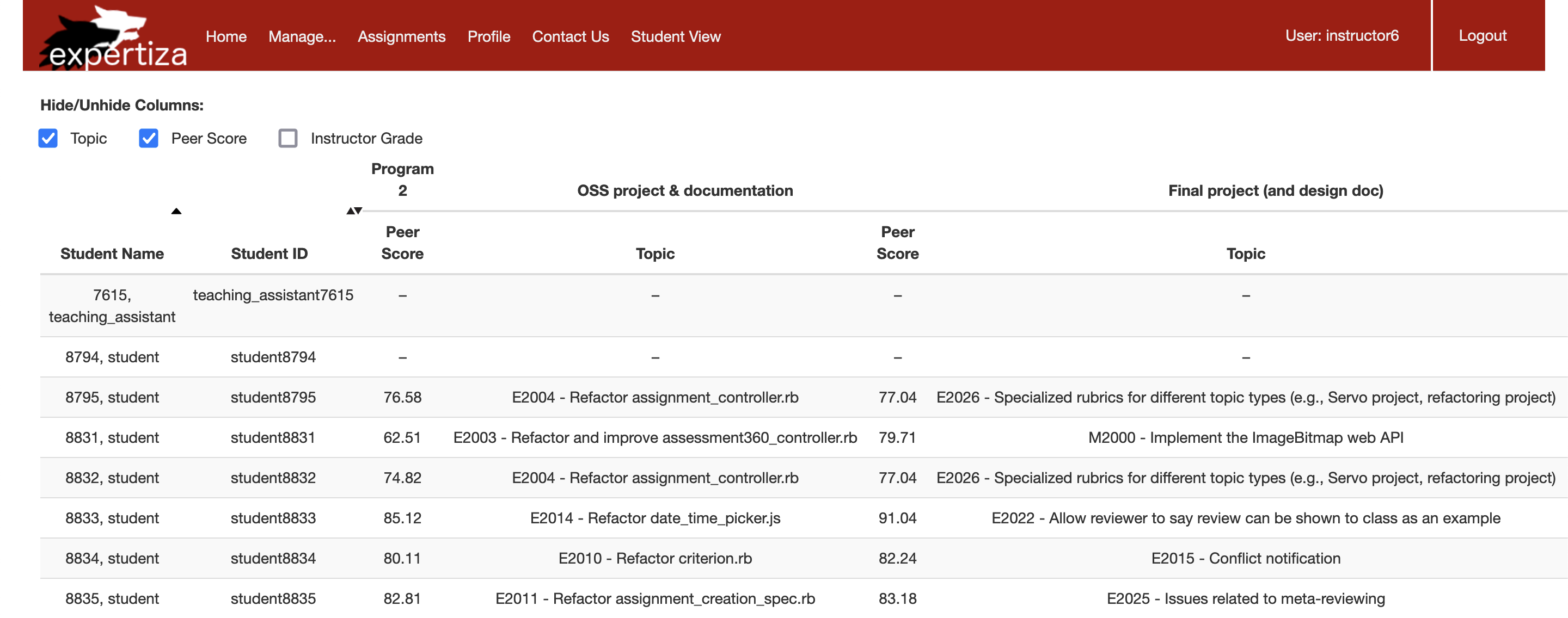
4. Verify that empty data that is shown as '-' (a hyphen) is replaced by an '–' (en-dash).
5. Verify that only those columns are visible with at least one data point.
6. Verify that average peer score column is visible and has correct values.
7. Verify that student name and student ID are in different columns
8. Select/un-select check-boxes provided on the top of the page.
9. Verify only those columns are visible that are checked.
Team
Mentor
Ameya Vaichalkar, (unityID:agvaicha, GitHub:ameyagv)
Team Members
1) Daksh Mehta (unityID: dmehta4, GitHub: dakshmehta23)
2) Soham Patil (unityID: sspatil6, GitHub: ThErOCk07)
3) Unnati Bukhariya (unityID: ubukhar2, GitHub: unnzzz)
Relevant Links
1. Youtube Video: https://www.youtube.com/watch?v=VTvF1abk-n8
2. Github forked repository: https://github.com/ThErOCk07/expertiza
3. Github Pull request: https://github.com/expertiza/expertiza/pull/2792
4. Deployed application: http://152.7.178.104:8080/
Credentials: Username: instructor6 ; Password: password