CSC/ECE 517 Spring 2024 - E2435 Implement Frontend for the My Profile
Expertiza
Expertiza stands as a versatile open-source web application constructed on the robust Ruby on Rails framework. Its primary function revolves around empowering instructors to craft tailored assignments, complete with curated topic lists, providing students with ample options for selection. Moreover, the platform extends its capabilities to facilitate seamless team formation, enabling students to collaborate efficiently on assignments and larger-scale projects.
One of Expertiza's standout features lies in its support for peer review processes, a crucial aspect of modern education. Through this functionality, students can engage in constructive feedback exchanges, offering evaluations and critiques on their peers' submissions. What sets Expertiza apart is its flexibility in accommodating various document formats, including URLs and wiki pages, ensuring comprehensive assessment avenues.
In essence, Expertiza represents more than just a mere educational tool; it embodies a comprehensive solution for managing assignments, fostering teamwork, and facilitating peer evaluations. Its open-source nature further enhances accessibility, making it freely available to educational institutions and individuals alike, thereby democratizing access to quality education tools.
Overview
The My Profile page in Expertiza currently implemented in Ruby needs to be reimplemented using React and TypeScript to enhance user experience and maintainability. The reimplementation aims to maintain feature parity while improving intuitiveness and usability.
Exisiting My Profile Page
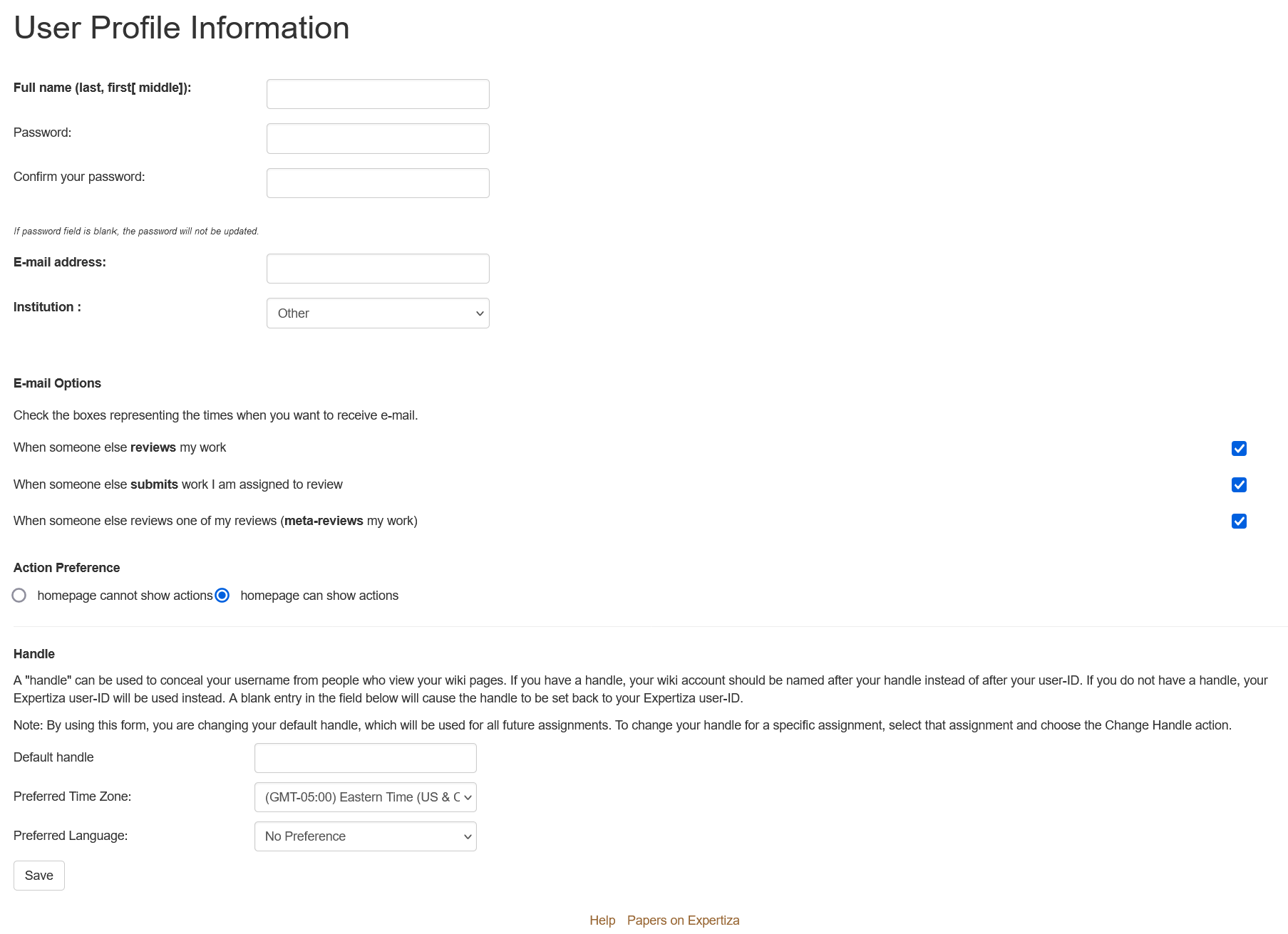
Existing My Profile page within expertiza
Flow Diagram
The actor can interact with the 'My Profile Page' by viewing the current information about the user and can also edit/update the information present on it. The Flow diagram below represents the interaction between the user (Actor) and the model, controllers and the database.
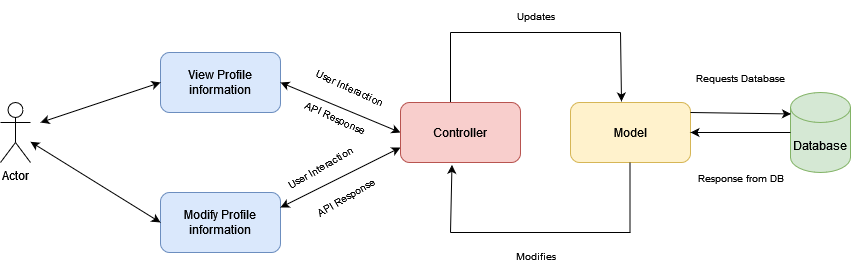
Flow Diagram
Reimplementation Details
Technologies
- React: Front-end library for building user interfaces.
- TypeScript: Adds static typing to JavaScript, enhancing code quality and maintainability.
Reimplementation in React and TypeScript
- Rewrite the Profile Controller logic in React components.
- Convert form submissions and updates to asynchronous requests using React hooks or class-based components.
Anonymized View
- Ensure the option for anonymized view is preserved in the new implementation.
- Create a toggle or checkbox to switch between anonymized and standard views.
Timezones
- Description: Users will have the ability to set their preferred timezone, ensuring that timestamps and deadlines are displayed accurately according to their local time.
- Details
- Users can select their timezone from a dropdown menu or similar interface, choosing from a list of supported timezones.
- The selected timezone will be used to convert timestamps and deadlines to the user's local time, providing a more accurate representation of time-sensitive information.
- This feature improves usability and reduces confusion by presenting time-related information in a format that is familiar and relevant to the user.
Email Options
- Description: Users can customize their email notification preferences for various events such as work reviews, submissions, and meta-reviews.
- Details
- Checkboxes or similar controls will allow users to opt-in or opt-out of email notifications for specific events.
- Users can select the types of notifications they wish to receive, tailoring their email preferences to suit their needs and preferences.
- This feature provides users with greater control over their communication preferences, reducing unnecessary email notifications and improving overall user satisfaction.
User Interface Enhancement
- Description: The user interface (UI) will undergo a redesign to make it more intuitive and visually appealing. The goal is to create a modern look and feel that aligns with current design trends while ensuring consistency with the existing Expertiza interface.The UI design will be homogeneous to the current reimplementation of Expertiza to maintain consistency across the platform. It will feature a clean and intuitive layout with clear navigation and easily identifiable controls.
- Details:
- The redesign will focus on improving the layout, typography, color scheme, and overall aesthetic appeal of the user profile page.
- Elements such as buttons, forms, and navigation will be redesigned to provide a more seamless and enjoyable user experience.
- Homogeneity with the current reimplementation of Expertiza will be maintained to provide continuity and familiarity for existing users.
Form Handling
- Refactor the form handling logic to work seamlessly with React's state management system.
- Implement form validation and error handling to provide a smoother user experience.
- Adjust routing and navigation to accommodate the new React components.
- Ensure proper redirection upon form submissions and updates.
Modularization and Componentization
- Break down complex functionalities into smaller, reusable components in React.
- Each component should have a single responsibility, making it easier to understand and maintain.
- Use clear and descriptive names for components, variables, and functions to convey their purpose effectively.
Consistent Coding Style
- Enforce a consistent coding style across the project using linters and code formatting tools.
- Follow established coding conventions and best practices to ensure readability and maintainability.
- Document code with comments and annotations to explain complex logic or implementation details.
Testing and Quality Assurance
- Conduct thorough testing of the reimplementation to ensure functionality and compatibility across browsers and devices.
- Address any bugs or issues discovered during testing before deploying the new version.
Design Principles followed
Single Responsibility Principle (SRP)
- Each React component will have a single responsibility, such as displaying user information, managing language preferences, or handling email options.
- Separate concerns such as data fetching, state management, and rendering to improve modularity and maintainability.
Separation of Concerns (SoC)
- Dividing the user profile page into distinct components for different sections, such as profile information, language preferences, and email options.
Don't Repeat Yourself (DRY)
- Refactoring redundant code or logic into reusable React components, hooks, or utility functions.
- Avoiding duplicate data-fetching or state management logic across multiple components by centralizing it in shared modules.
Open/Closed Principle (OCP)
- Designing components to be open for extension by allowing for customization or configuration through props or context.
- Ensuring that components can be extended or modified without altering their core functionality by adhering to React's composition model.
Files Added / Changed
Edit.tsx
The Edit component is a React functional component that manages the user profile information form. It integrates the Formik library to handle form state, validation, and submission efficiently. The form includes various input fields such as full name, password, confirm password, email, institution, action preference, handle, preferred time zone, and preferred language. Each field is initialized with default values using the initialValues object.
To enforce validation rules, a Yup validation schema is defined. It ensures that fields like full name, password, confirm password, email, and handle are required and follow specific formats. Validation errors are displayed below each input field using the ErrorMessage component from Formik.
Additionally, the form layout and styling are improved to enhance the user experience. Labels for input fields are styled to have a bold font-weight, enhancing readability. The submit button is styled using React Bootstrap's Button component with a variant of "outline-success" for a visually appealing appearance.
A helpful note is added below the password fields, informing users that leaving the password field blank will result in no updates to the password. This ensures clarity and guides users effectively through the form.
Overall, these changes enhance the user profile page by providing a structured and visually appealing form with robust validation and error handling capabilities, resulting in an improved user experience.
Link to Edit.tsx changes: Edit.tsx
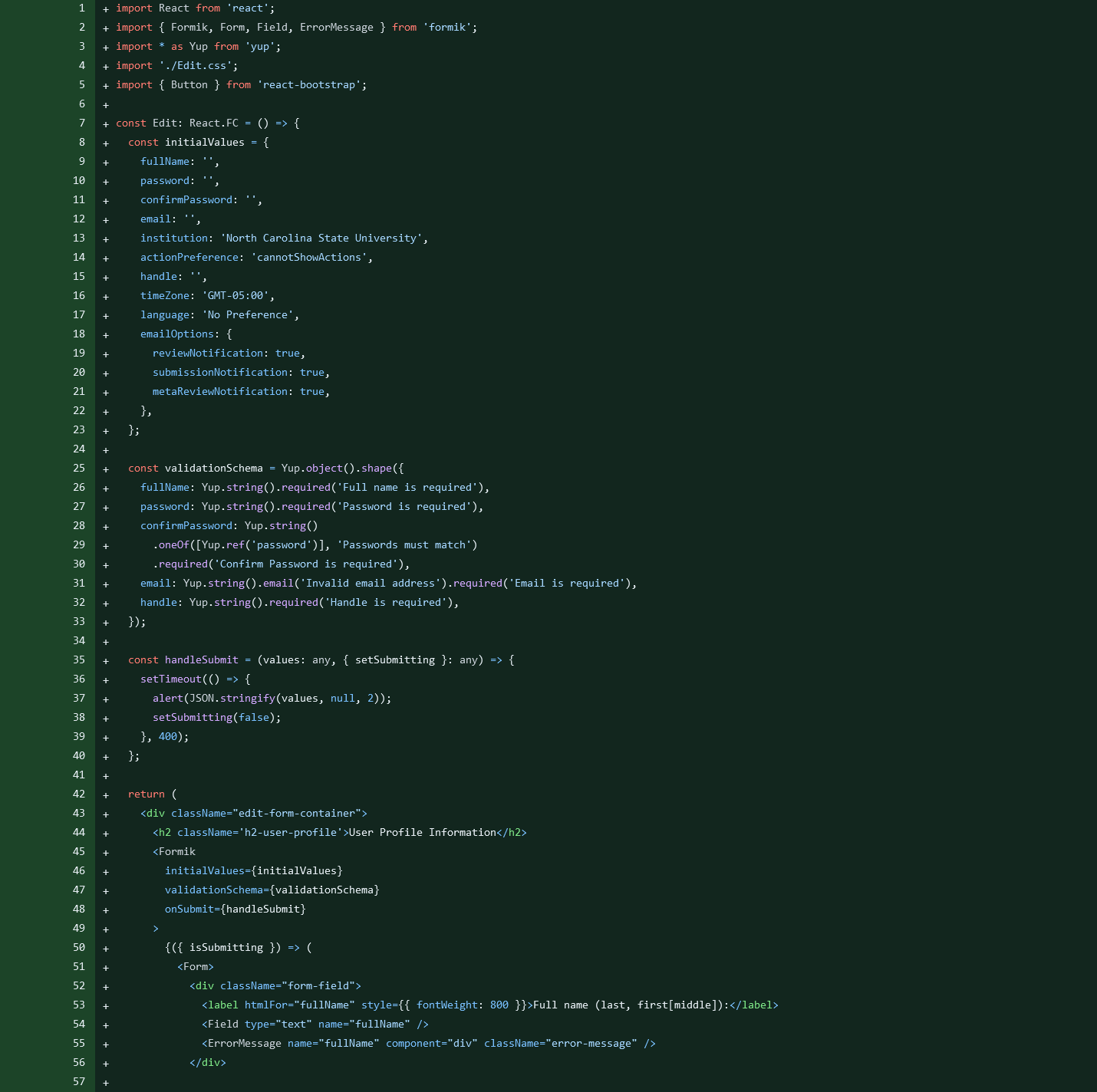
Changes in Edit.tsx
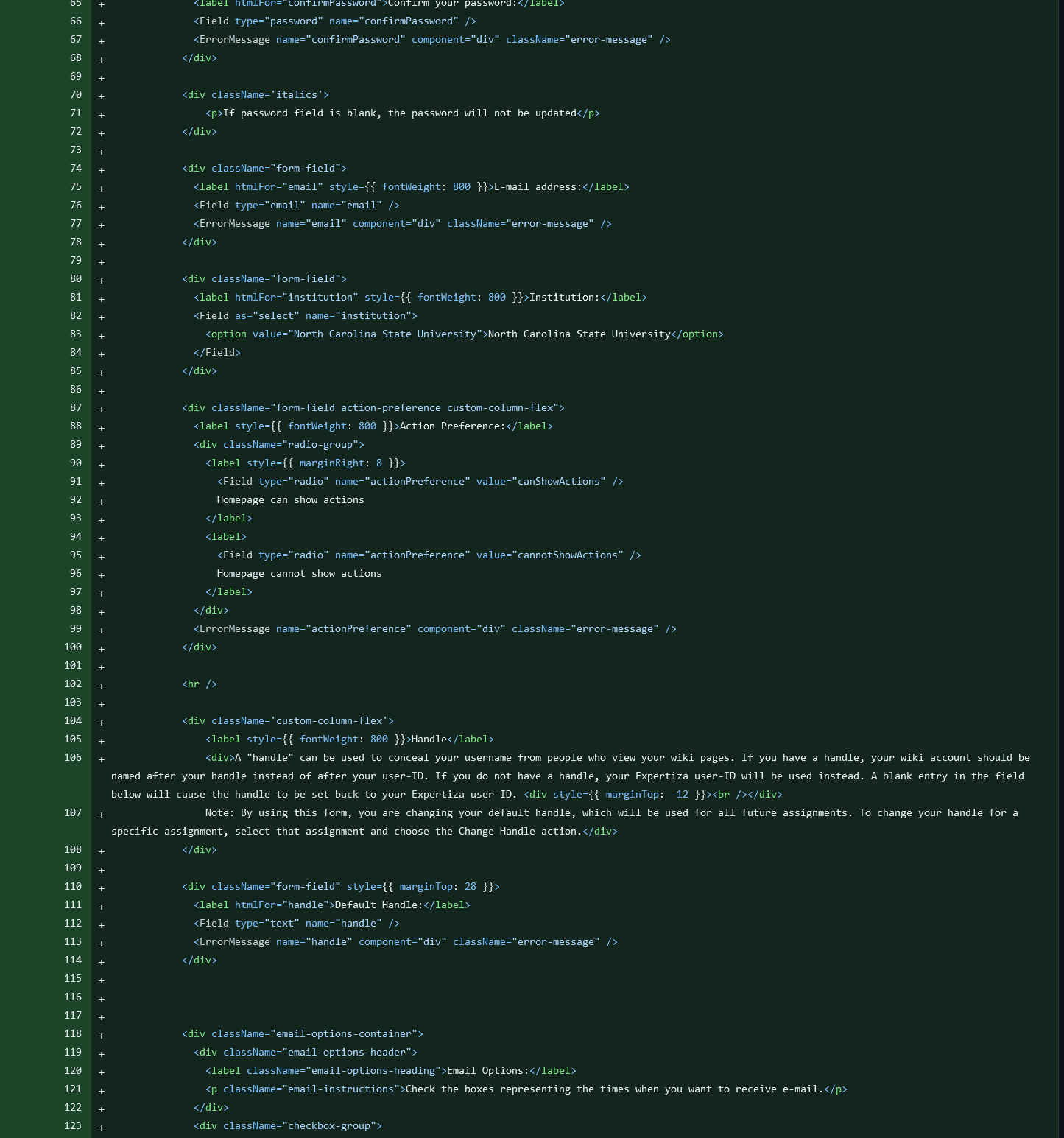
Changes in Edit.tsx
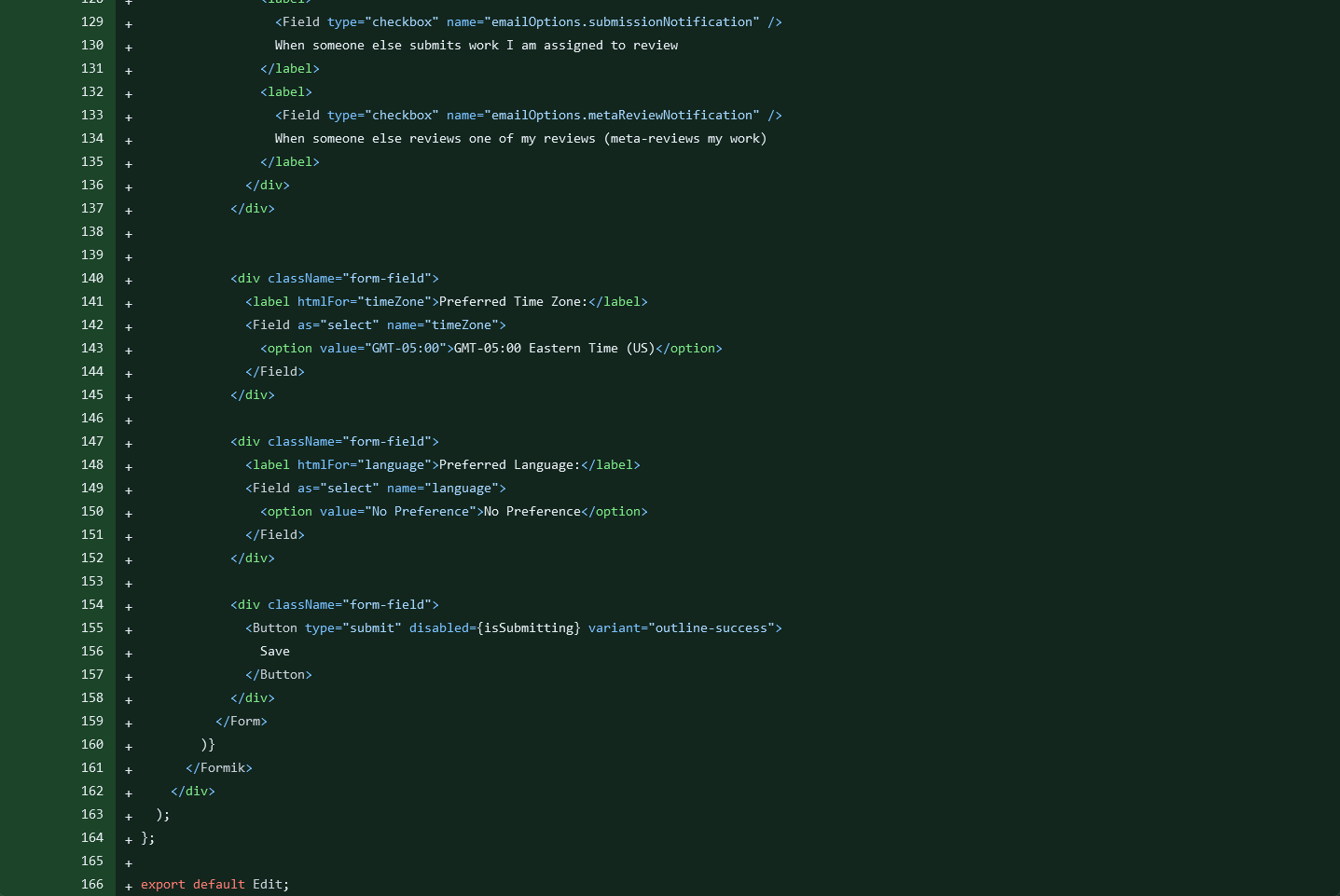
Changes in Edit.tsx
Edit.css
In the edit.css file, additional styles were added to further refine the appearance and layout of the user profile editing page. Specifically, adjustments were made to the .edit-form-container class to refine padding and limit the maximum width of the form container. These modifications aim to optimize the layout and improve readability.
A new class named .italics was introduced to style the italicized note below the password field. This class adjusts the font style, size, and margins to ensure the note is visually distinct and easily noticeable.
Lastly, a .custom-column-flex class was created to facilitate a flexbox layout with a column direction. This class helps align form elements vertically, ensuring consistent spacing and alignment across different screen sizes. Overall, these changes contribute to a more polished and user-friendly user profile editing experience.
Link to the Edit.css changes: Edit.css
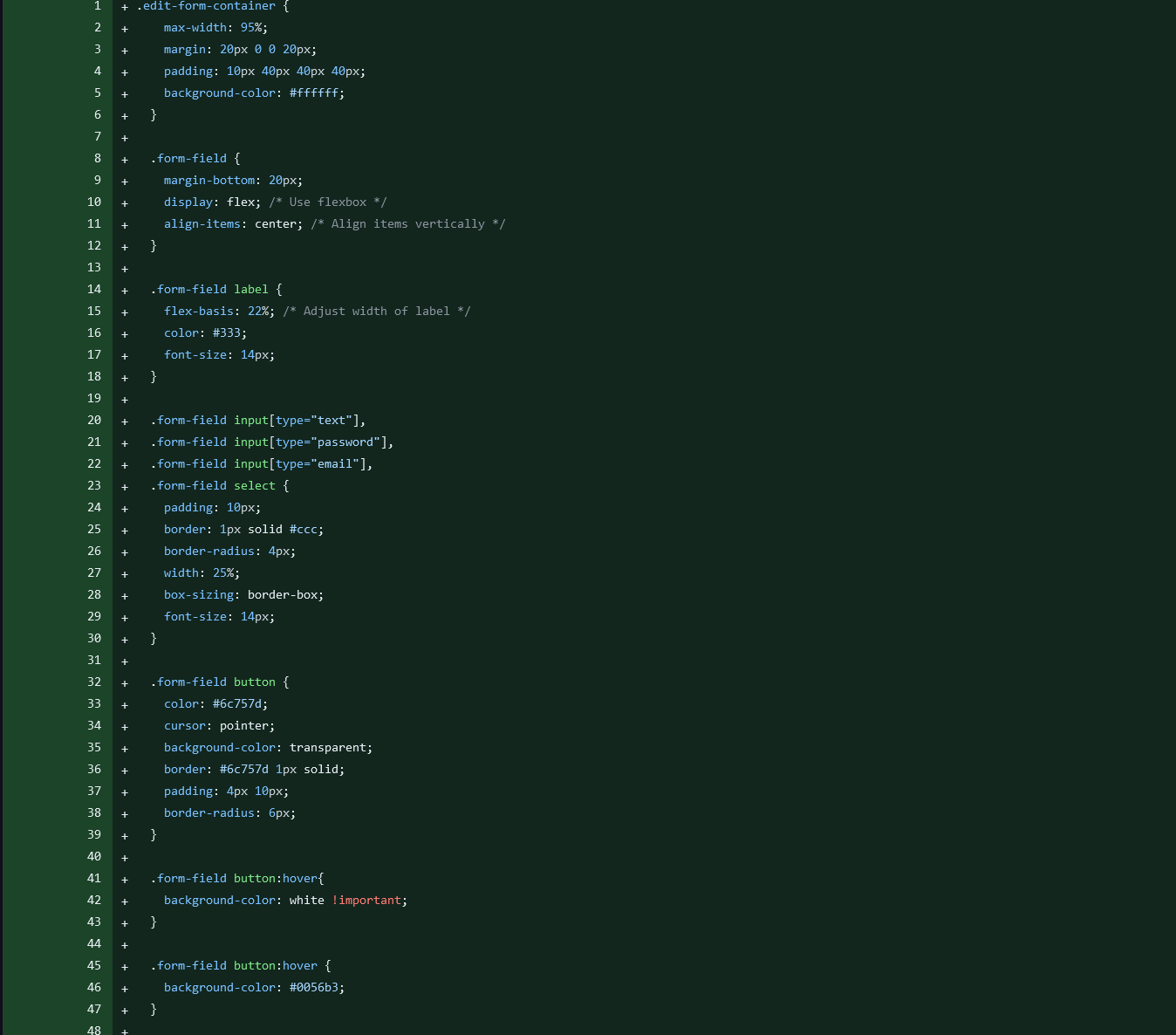
Changes in Edit.css
New My Profile Page
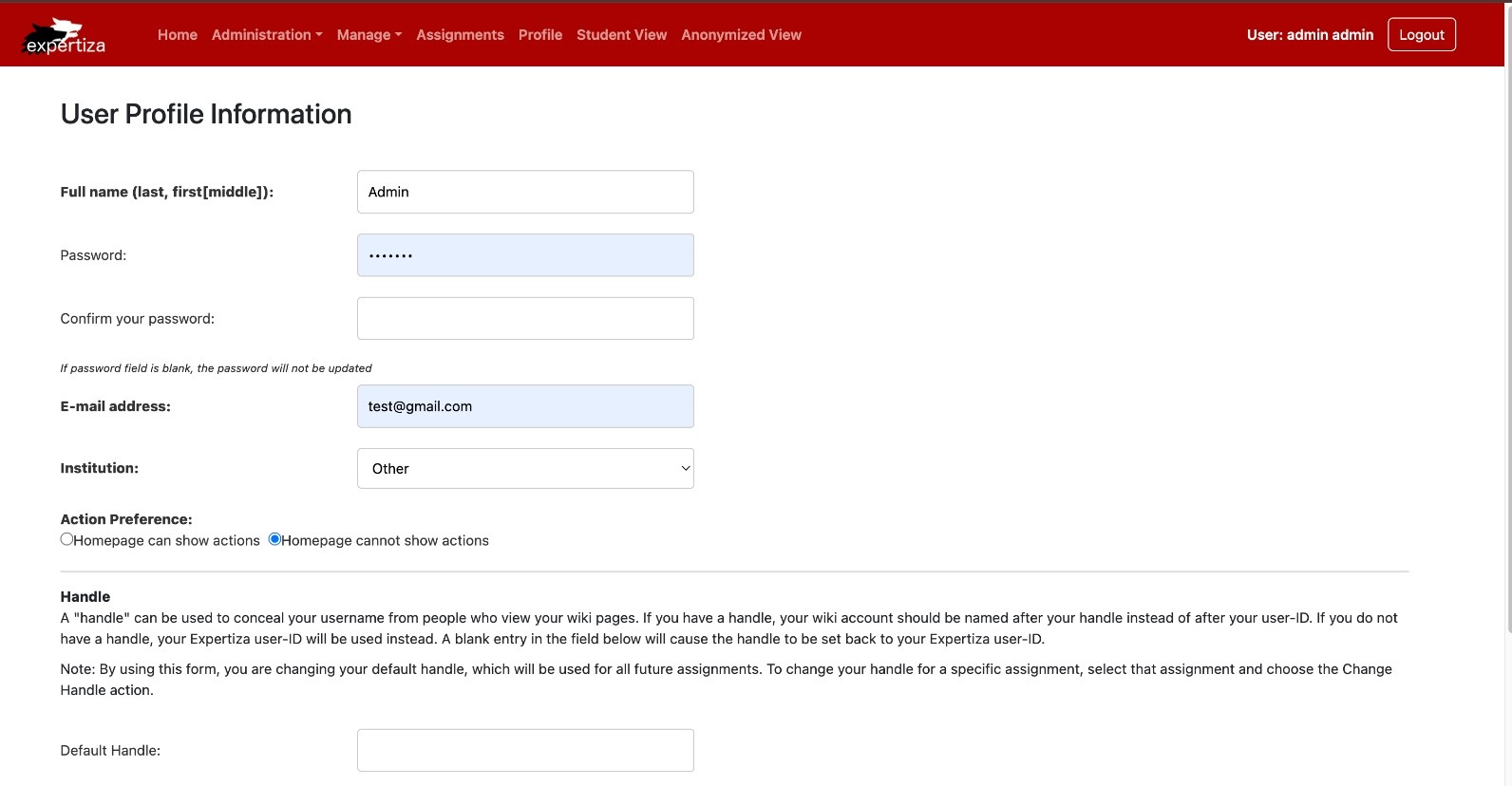
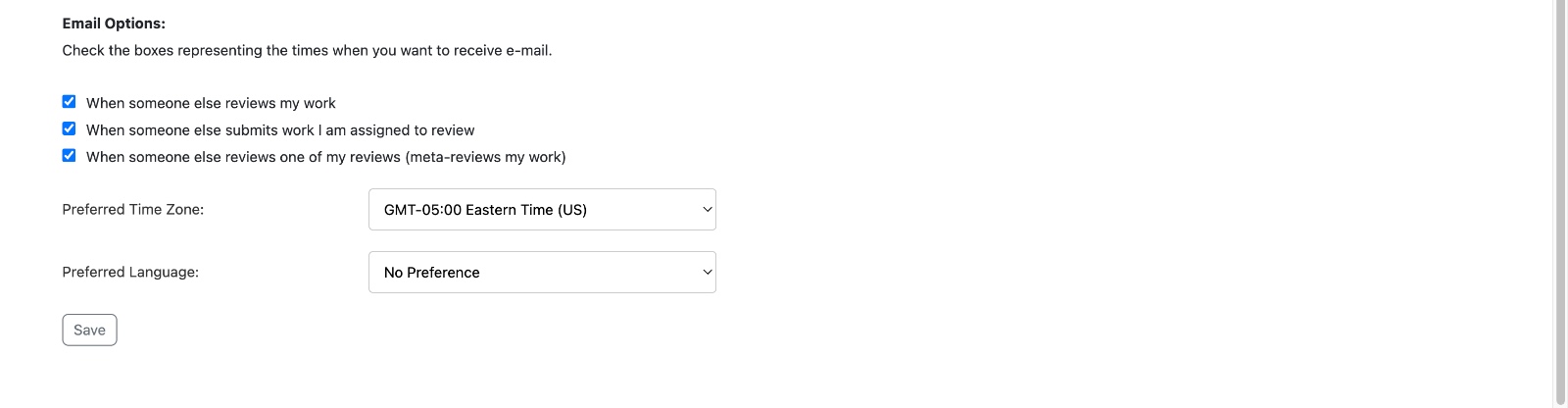
New my profile page
The My Profile page has been reimplemented using React and TypeScript to improve user experience and make it easier to maintain. The goal of the reimplementation is to keep all the existing features while making the page more intuitive and user-friendly.
Testing
- Component Rendering: During our testing process, we thoroughly validated that all elements within the pages were correctly translated into React.js and Typescript components. We ensured that each component was properly implemented according to the specified requirements and guidelines.
- Styling Consistency: We rigorously checked that the new frontend design maintained uniform styling consistent with the existing application. This involved reviewing CSS files, inspecting page layouts, and verifying color schemes, fonts, and spacing to ensure visual coherence across the entire application.
- Functionality Testing: Our testing efforts focused on assessing the functionality of interactive features such as buttons and input fields to guarantee they operated as intended. We conducted comprehensive tests to validate user interactions, form submissions, and any other dynamic elements on the pages.
- Responsive Design Testing: To ensure a seamless user experience across different screen sizes and devices, we conducted thorough testing for responsiveness. This involved resizing browser windows and testing on various devices to confirm that the pages adapted appropriately to different viewport dimensions.
- Integration Testing: Integration testing was performed to test the integration of different components and modules within the pages. We verified that all components interacted correctly with each other and that data flow and state management were properly handled throughout the application.
- Cross-Browser Testing: To ensure broad compatibility, we conducted cross-browser testing to verify that the pages rendered correctly and functioned as expected across different web browsers. We tested on popular browsers such as Chrome, Firefox, Safari, and Edge to ensure a consistent experience for all users.
Team
Mentor
- Riya Gori (rygori@ncsu.edu)
Members
- Aakarsh Satish (asatish2@ncsu.edu)
- Shubh Nisar (snisar@ncsu.edu)
- Sinchana Shetty (skshett2@ncsu.edu)
Contributors to this project
- Shubh Nisar (unityid: snisar, github: Shubh-Nisar)
- Aakarsh Satish (unityid: asatish2, github: Aakarsh-Satish)
- Sinchana Shetty (unityid: skshett2, github: sinchanashetty11)
- Github repo https://github.com/sinchanashetty11/reimplementation-front-end
- Link to PR https://github.com/expertiza/reimplementation-front-end/pull/53