CSC/ECE 517 Spring 2024 - E2417. Reimplement submitted content controller.rb (Phase 2)
E2417. Reimplement Submitted Content Controller
This page provides a description of the Expertiza based OSS Project E2417. Reimplement the SubmittedContentController for Phase 2
About Expertiza
Expertiza is an open-source web application facilitating peer feedback and assessment in educational courses. It enables students to submit work, review peers' submissions, and receive feedback. The platform supports anonymous peer reviews, grading rubrics, and discussion forums. Its goal is to enhance collaborative learning and improve the quality of student work through constructive criticism.
Objective
A submitted content controller should have the functionalities to manage the submitted content, for eg, the CRUD operations for submissions, different types of submissions, etc. The goal here is to implement this controller via API's and use principles of Object-Oriented Programming such as SOLID.
Problem Description
The reimplementation of SubmittedContentController in Expertiza needs to be done to enhance its functionality and maintainability. It should handle various tasks like file submissions and uploads, hyperlink submissions, and downloads, but the controller suffers from code redundancy and mixed responsibilities within methods. The reimplementation aims to ensure participants receive appropriate messages and HTTP status codes for actions like uploading files and deleting them, while also using SOLID principles, refactoring DRY code and minimizing excessive use of instance variables. The goal is to optimize the controller's design, adhering to best practices in readability and modularity.
Phase 1 implementation
Some operations of the SubmittedContentController in Expertiza were reimplemented in the phase 1 of the program, using SOLID and DRY principles. The following implementations were done in phase 1:
- Create, Show and Index methods for the controller
- SignedUpTeam, SignedUpTopic, SubmissionRecord, Participant, Assignment, Assignment Participant, SignUpSheet, Team, Team User, DutyLate Policy Models
- Submitted Content Helper
Error handling for file manipulation remains robust, ensuring reliable operation. Overall, the refactoring simplifies the codebase, improving its readability and facilitating future modifications. - The PR link for phase 1 implementation : https://github.com/expertiza/reimplementation-back-end/pull/78/files
Phase 2 implementation
The controller can further be more enhanced by reimplementing some more functionalities and refactoring the code by using SOLID and DRY principles. The following is the list of things that we are aiming to achieve in this phase.
Dependency Diagram
The blue colored boxes are controller methods and the green boxes are the models. Their dependency to each other, i.e. what controller models are called by what models, and then model to model dependency.
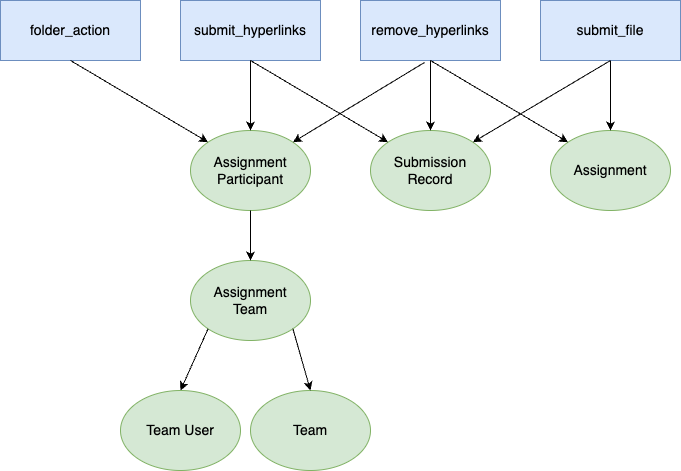
UML Diagram
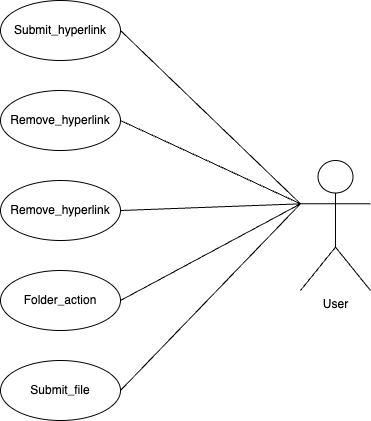
Functionalities
submit_hyperlink
About: It handles the submission of a hyperlink for an assignment by a participant. Before submitting the hyperlink it checks if the current user is a participant and if the team has already submitted a hyperlink. At last we add the hyperlink to teams and create a submission record to track changes. We have not implemented the mailing feature as it is currently not a major requirement.
Problem: The process of handling hyperlink submissions for assignments by participants posed several challenges within the system. The code violated the DRY (Don't Repeat Yourself) principle by redundantly instantiating the participant variable across multiple controller methods, leading to potential inconsistencies and unnecessary duplication. Furthermore, the logic for creating a submission record and the submission hyperlink were intertwined, making the code harder to understand and modify.
Solution: To address these challenges, we implemented several refinements to enhance the functionality and maintainability of the system. Firstly, we centralized the instantiation of the participant variable, ensuring consistency and eliminating redundancy across controller methods. This was achieved by extracting the participant instantiation process into a private method within the controller. Secondly, we streamlined the codebase by separating the creation of a submission record and the submission hyperlink into distinct functions. This not only improved code readability but also adhered to best practices in software design by promoting a modular and focused approach to function implementation.
Parameters:
- id : participant id
- submission: hyperlink to be submitted
Responses: 200 for submission of hyperlink, 422 for any issues with the request.
Code Link: submit_hyperlink
remove_hyperlink
About: For the remove hyperlink we remove the hyperlink previous submitted from the team table and also created a submission record for the delete action.
Problem: Similar to the issues encountered in the submit_hyperlink method, the remove_hyperlink method also suffered from violations of the DRY (Don't Repeat Yourself) principle and a lack of separation of concerns. The method combined multiple tasks within a single function, leading to increased complexity and reduced code readability.
Solution: To mitigate these issues and improve the overall quality of the codebase, we undertook a systematic approach to optimize the remove_hyperlink method. By adhering to the DRY principle, we refactored the implementation to separate distinct tasks into individual functions. This modularization not only enhances code organization but also facilitates better readability and comprehension.
Parameters:
- id : participant id
- check_links: index for the hyperlinks
Responses: 204 for successfully deleting a file and 422 for unprocessable entity.
Code Link: remove_hyperlink
submit_file
About: This function is responsible for handling the submission of a file by a participant in an assignment. It performs various checks on the file size and type before saving the file to the appropriate directory. It also creates a submission record.
Problem: The previous implementation suffered from long and convoluted functions that performed multiple tasks, resulting in decreased code readability and maintainability. These overly complex functions combined disparate responsibilities, such as creating a submission record, performing file checks, and storing the file, into a single monolithic block of code.
Solution: To address these issues we underwent a refactoring process. The primary focus was to separate the various tasks performed by the functions into individual methods, each with a single, well-defined responsibility. By isolating the creation of a submission record, file checks, and file storage into their respective methods, the code became more organized and easier to comprehend.
Parameters:
- id: participant id
- uploaded_file: file to be uploaded
Responses: 200 for a successful file submission 422 for unprocessable request.
Code Link: submit_file
folder_action
About: This handles various folder-related actions for an assignment participant, such as deleting files, renaming files, moving files, copying files, and creating new folders based on request parameters.
Enhancement: The folder action method was already optimized, and the DRY principle was followed; however, the functions were implemented as private methods within the controller. To enhance code organization and reduce clutter in the controller methods, we refactored these functions into helper methods. By moving them to helper methods, we maintain code cleanliness and improve readability, ensuring that the controller methods focus solely on coordinating actions and handling requests without unnecessary implementation details.
Issues Faced: The copy file folder actions were not implemented due to incomplete information on how it is used in the original expertiza. Also, the previous implementation in expertiza its copying the file from the same directory param as the one we will be pasting it to. So we skipped copying for now.
Parameters:
- id : participant id
- directories: [array of root directory paths]
- file_names: [array of files names]
- chk_files: index for the directory and file name array
- faction: { rename: new file name
- move: complete path including file name to new destination
- delete: file to be deleted
- create: path to create a new directory }
Responses: 200 for successful actions, 204 for the file has been deleted, 400 for a file with that name already exists - 'Please delete the existing file before copying' message, 404 for the referenced file does not exist, 409 for a file that already exists in this directory with the name, 422 if problem in file operation.
Code Link: folder_action
download
About: This method is responsible for handling the download of files from a specified folder. It takes the folder name and file name as parameters and checks if they are valid. If the file exists, it sends the file to the user for download.
Enhancement: We enhanced the function to adhere more closely to REST API principles by refining its design and ensuring it aligns with standard conventions. Additionally, we refactored the changes introduced by other methods, streamlining the codebase and promoting consistency throughout the application.
Parameters:
- current_folder : relative root directory path
- download : file name
Responses: 200 for successful file downloaded, 400 for bad request: Cannot send a whole folder, 404 for file not found.
Code Link: download
Models
The following models have been updated adding associations, principles, etc.
- assignment.rb
- assignment_participant.rb
- assignment_team.rb
- course.rb
- participant.rb
- submission_record.rb
- team.rb
- teams_user.rb
- user.rb
Test Plan
For comprehensive testing of submitted_content_controller, the test plan given below will be taken into consideration:
- Automated Tests: Test scenarios along with edge cases wherever applicable to ensure proper working of functions as intended.
- Manual UI Tests: Testing functionalities like adding or removing hyperlinks/files to make sure any modifications don't damage the system's general functionality.
Automated Tests (using RSpec): The following outlines the scenarios to be tested for the Submitted Content functionality within the system:
Submit Hyperlink
- Scenario: Ensure that submission record is created when user submits a valid hyperlink and hyperlink is added to the team.
- Edge Case: User is not a participant of the assignment.
- Edge Case: Team has submitted the same hyperlink.
- Edge Case: Hyperlink is invalid.
Remove Hyperlink
- Scenario: Ensure that hyperlink is removed from the team and a submission record is created when user removes a submitted hyperlink.
- Edge Case: User is not a participant of the assignment.
Submit File
- Scenario: Ensure that necessary directory is created and file is saved.
- Edge Case: File size exceeds the limit.
- Edge Case: File extension is not permitted.
- Edge Case: User is not a participant of the assignment.
Download
- Scenario: Ensure that file is downloaded.
- Edge Case: File name is nill.
- Edge Case: Folder name is nill.
- Edge Case: File not found.
- Edge Case: User is not a participant of the assignment.
Folder Action
- Scenario: Ensure that selected file is deleted when delete action is selected.
- Scenario: Ensure that selected file is renamed when rename action is selected.
- Scenario: Ensure that selected file is moved when move action is selected.
- Scenario: Ensure that selected file is copied when copy action is selected.
- Scenario: Ensure that a new folder is created when create action is selected.
- Edge Case: User is not a participant of the assignment.
Login Credentials
- username: student1@test.com
- password: student
Reasoning: Only allow students who are participating in a specific assignment with a specific team
Demo Video Link
https://youtu.be/Xbs22yeHIko?si=fnSY9C5UyzP8MTY_
Github Repo
https://github.com/Neel317/reimplementation-back-end