CSC/ECE 517 Spring 2023 - E2309. Refactor Node model and its subclasses
Overview of Expertiza
Expertiza is a learning management system that is developed with Ruby on Rails and is accessible as open source software. It can create assignments, tests, assignment teams, and courses, among a wide range of other features and functions. It also has a thorough system for giving other teams and groups of teammates peer reviews and feedback. The files that are largely addressed in this project, such as assignment_node.rb
, course_node.rb
, team_node.rb
, folder_node.rb
, and questionnaire_node.rb
, are essential in executing this functionality.
Description of Project
The following is an Expertiza based OSS project which deals primarily with the Node model.
The Node Ruby class, which derives from ApplicationRecord and offers functionality for creating and modifying tree structures, is defined in the node.rb file. The class provides a number of methods, including get partial name, get name, get directory, and get child type, for collecting details about the node and its descendants. It also contains the has paper trail method for versioning relationships with parent and child nodes through belongs to and has many associations, respectively. The Node class provides a flexible framework for creating and modifying trees in Ruby applications and is designed to be subclassed for usage in tree display code.
The node model defines a tree structure containing Assignments, Courses_table, Questionnaire Types, Questionnaires, and Teams. The node model class is a superclass for the seven files namely assignment_node.rb
, course_node.rb
, folder_node.rb
, questionnaire_node.rb
, questionnaire_type_node.rb
, team_node.rb
and team_user_node.rb
. Most of the methods written in node.rb
are implemented by polymorphism in their respective subclass files.
The following changes were made:
1. Method names were made more informative of the method wherever necessary.
2. Some common methods in the subclasses were implemented in different ways. So the methods in the subclasses were refactored so that they are uniformly implemented.
3. Appropriate comments were added to the existing code so that the functionality is easier to understand.
4. A method was created to return true/false based on courses.private.
5. Finally, tests were updated according to the changes made in the methods.
Files Modified
Changes to app/models/assignment_node.rb
# | Change | Why? |
---|---|---|
1 | Redefined self.get method and added two new methods self.get_assignment_query_conditions and self.get_assignments_managed_by_user .
|
The self.get method calls these two methods and creates the database query for getting the assignments. Creating two extra methods and calling them from self.get makes the code readable and uniform among all the files.
|
2 | self.get_assignment_query_conditions has all the conditions needed for the database query.
|
It makes it easy to list all the query conditions at one place rather than in one method. |
3 | self.get_assignments_managed_by_user manages all the user permissions with various conditions.
|
It is better to maintain all the rights and accesses at one place rather than in the same method as self.get .
|
4 | Renamed the methods get_max_team_size , get_is_intelligent , get_require_quiz , get_allow_suggestions to get_max_assignment_team_size , get_assignment_is_intelligent , get_assignment_require_quiz get_assignment_allow_suggestions .
|
Adding the word assignment to the methods name makes the methods more informative and adds meaning. |
5 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to app/controllers/tree_display_controller.rb
# | Change | Why? |
---|---|---|
1 | Renamed the methods that were renamed in assignment_node.rb above
|
The methods tha renamed in assignment_node.rb have to be the same in controller so that when they are called, it does not give an error.
|
Changes to app/models/course_node.rb
# | Change | Why? |
---|---|---|
1 | Renamed the method get_survey_distribution_id to get_course_survey_distribution_id .
|
Adding the word course to the method name makes the method more informative and adds meaning to survey distribution id. |
2 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
3 | Added a method named - course_is_private? | Currently the courses are categorized as private or not private. In future there can be a use case where true or false needs to be returned depending on whether the course is private or not. This has been handled by the course_is_private? method which is commented now and can be uncommented when required. |
Changes to app/models/folder_node.rb
# | Change | Why? |
---|---|---|
1 | Renamed the method get_child_type to get_folder_child_type .
|
Adding the word folder to the method name makes the method more informative and adds meaning to the child type of the folder. |
2 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to app/models/node.rb
# | Change | Why? |
---|---|---|
1 | Changed the method name from get_child_type to get_folder_child_type
|
The method name was changed in the folder_node.rb . Since node.rb is its super class, the method name in it also has to be the same
|
Changes to app/models/questionnaire_node.rb
# | Change | Why? |
---|---|---|
1 | Redefined self.get method and added two new methods self.get_questionnaire_query_conditions and self.get_questionnaires_managed_by_user .
|
The self.get method calls these two methods and creates the database query for getting the assignments. Creating two extra methods and calling them from self.get makes the code readable and uniform among all the files.
|
2 | self.get_questionnaire_query_conditions has all the conditions needed for the database query.
|
It makes it easy to list all the query conditions at one place rather than in one method. |
3 | self.get_questionnaires_managed_by_user manages all the user permissions with various conditions.
|
It is better to maintain all the rights and accesses at one place rather than in the same method as self.get .
|
4 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to app/models/questionnaire_type_node.rb
# | Change | Why? |
---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to app/models/team_node.rb
# | Change | Why? |
---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to app/models/team_user_node.rb
# | Change | Why? |
---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Changes to spec/models/course_node_spec.rb
# | Change | Why? |
---|---|---|
1 | Changed the method name from get_survey_distribution_id to get_course_survey_distribution_id in the test case where it is used
|
Any changes made to the name of methods in the files should also be reflected in the test cases for them to pass successfully. If a method name is changed in a file and the same is not updated in the test case, the test case will fail |
Testing
Testing was not in the scope for this project as it did not involve creating new methods or functions. However, care was taken that the existing test cases pass after refactoring the code as mentioned in the previous sections. Screenshots for all passing test cases have been attached below
Test Plan - Manual/System Test Cases
We ran a few system tests manually to make sure the functionality works as expected.
1) Test instructor login & redirect to home page
Login page was displayed properly from our branch. After logging in user is properly redirected to the home page.
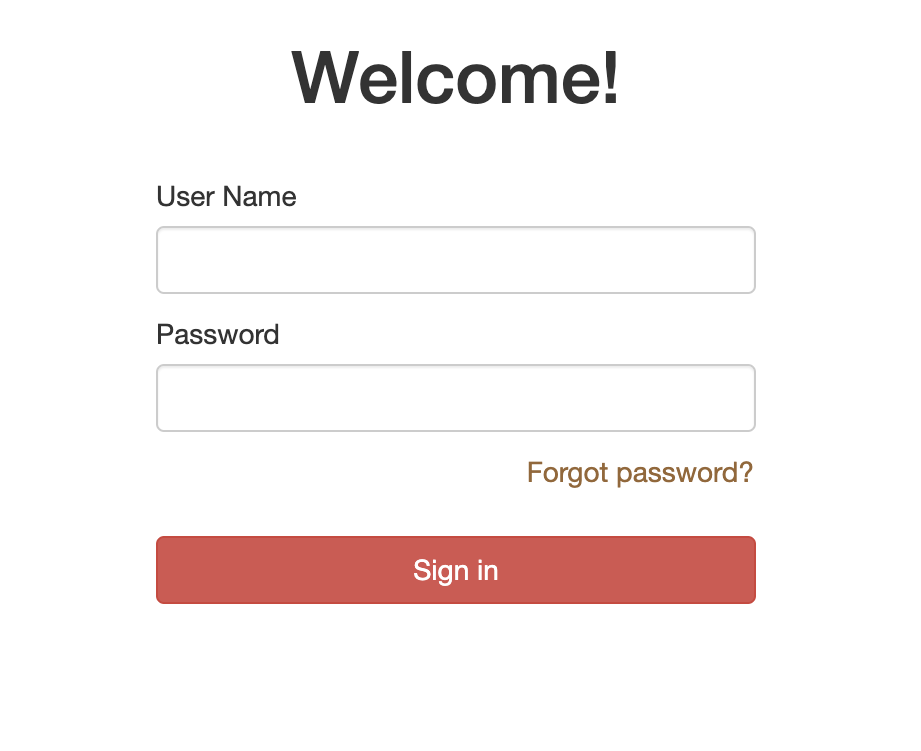
2) Test Course Section
Then, when the user clicks the course section, the course section is loaded with all the public and private courses displayed.
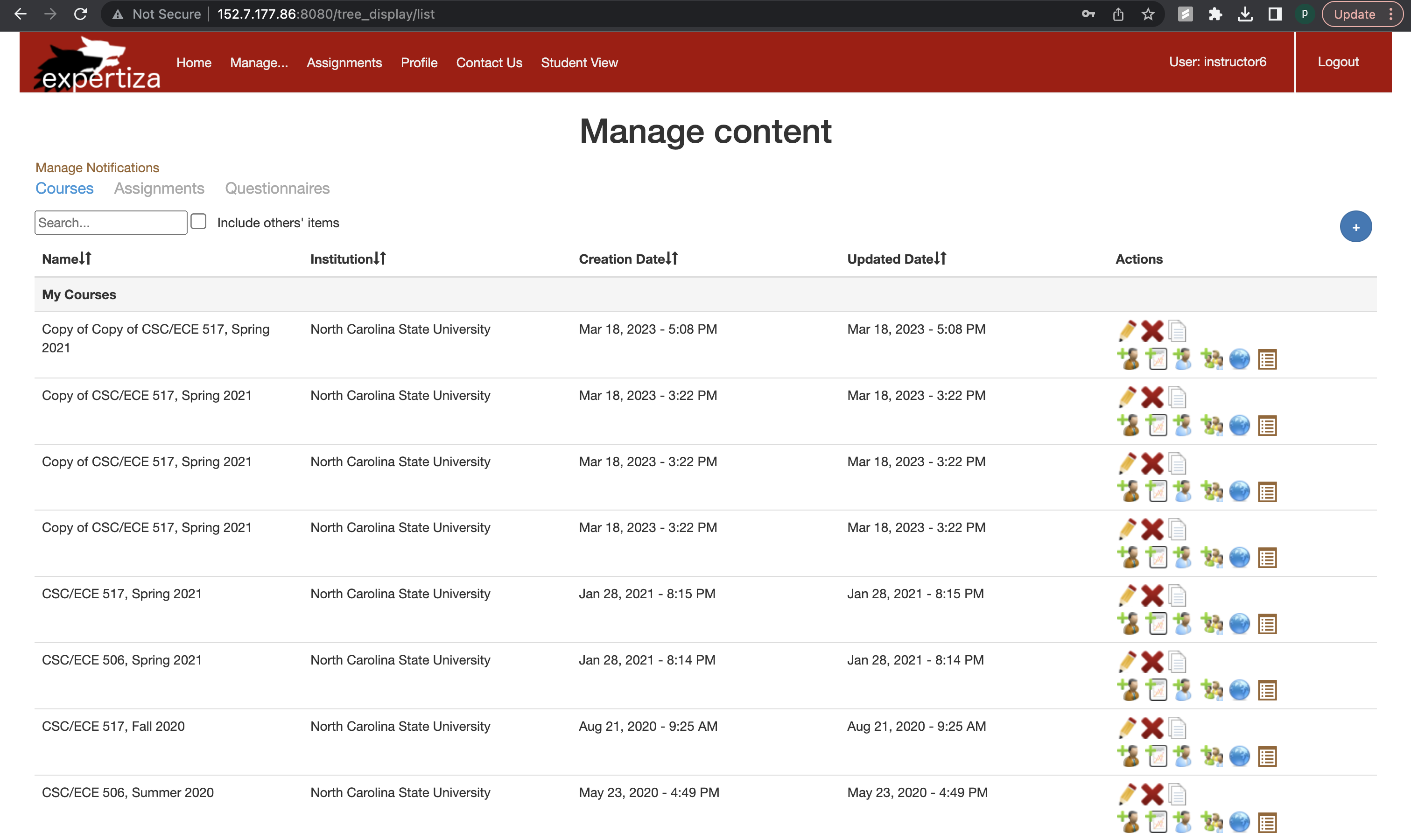
3) Test Assignment section
Then, when the user clicks the assignment section, the assignment section is loaded with all the assignments displayed.
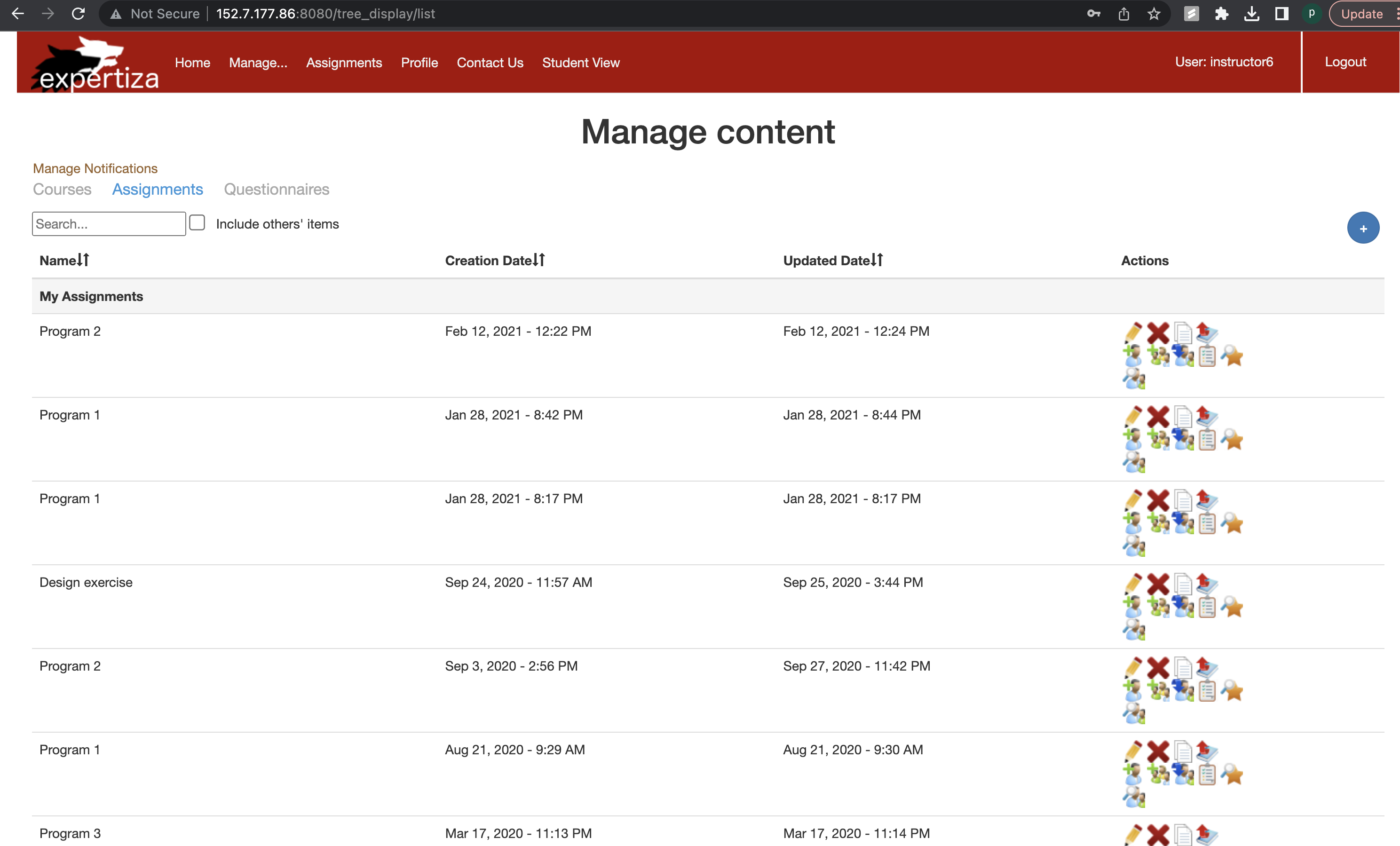
4) Test Questionnaire section
Then, when the user clicks the questionnaire section, the questionnaire section is loaded with all the questionnaires displayed.

Automated Testing of course_node.rb
After refactoring the code for course_node.rb
, all the test cases passed for it.
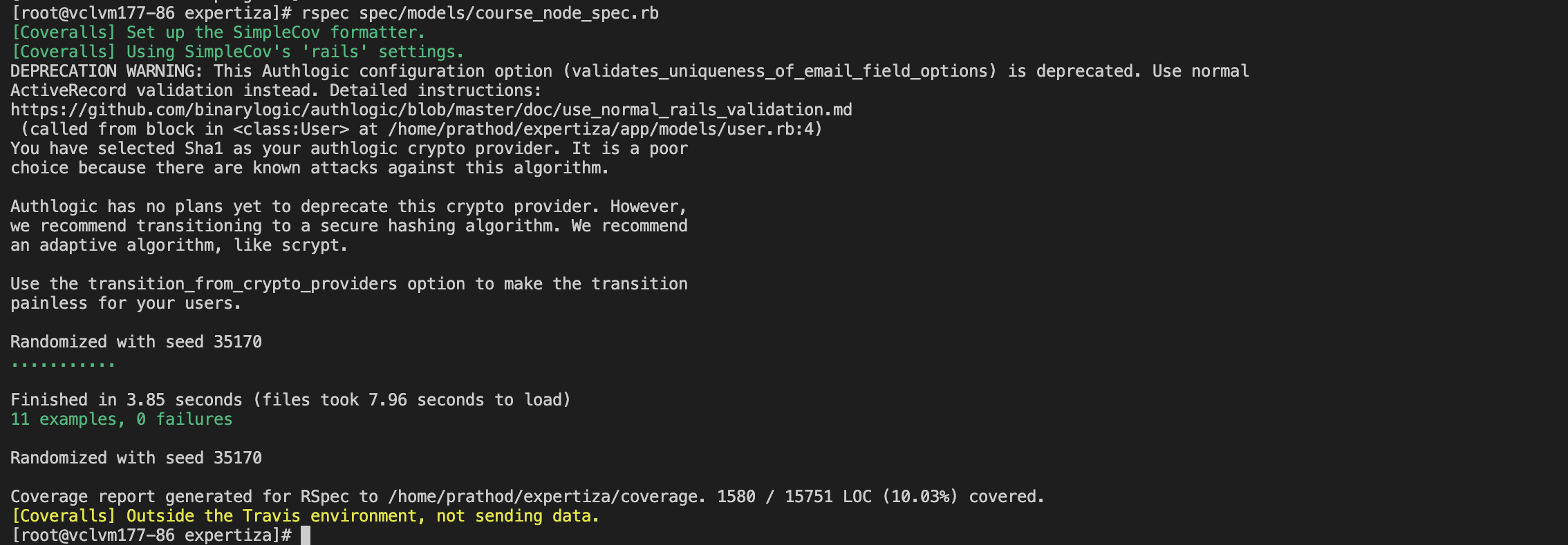
Automated Testing of questionnaire_node.rb
After refactoring the code for questionnaire_node.rb
, all the test cases passed for it.
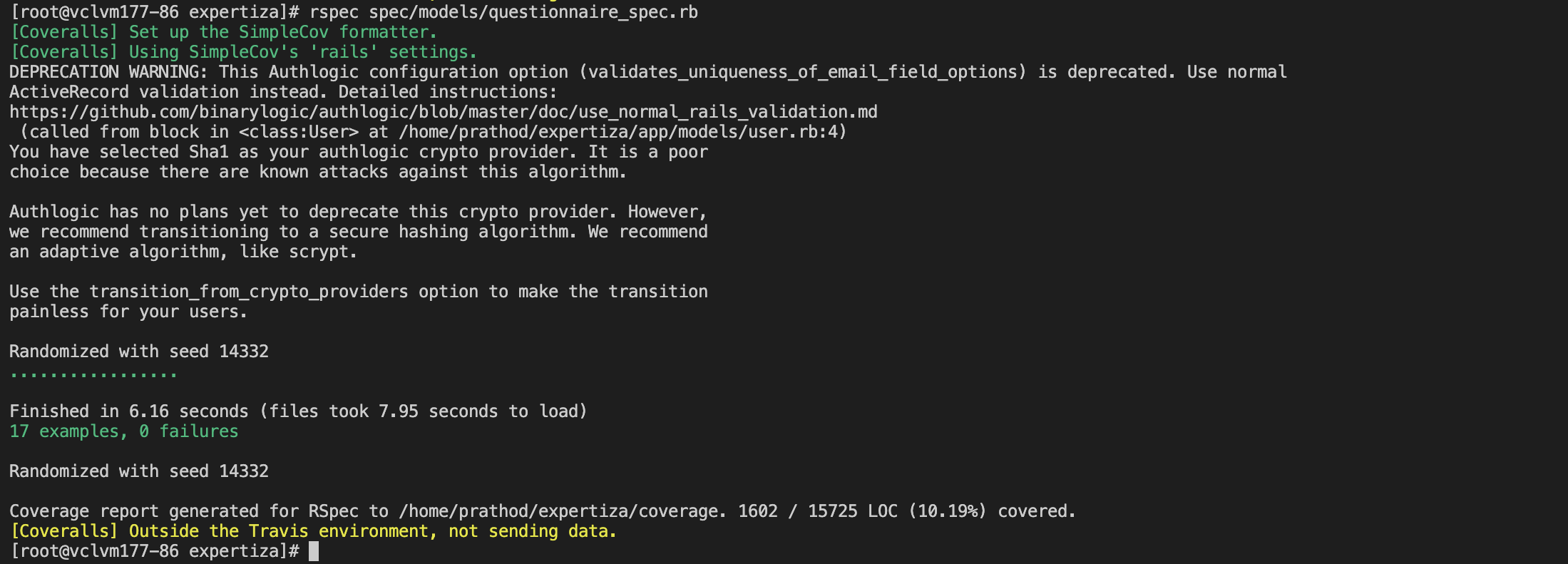
Automated Testing of team_node.rb
After refactoring the code for team_node.rb
, all the test cases passed for it.
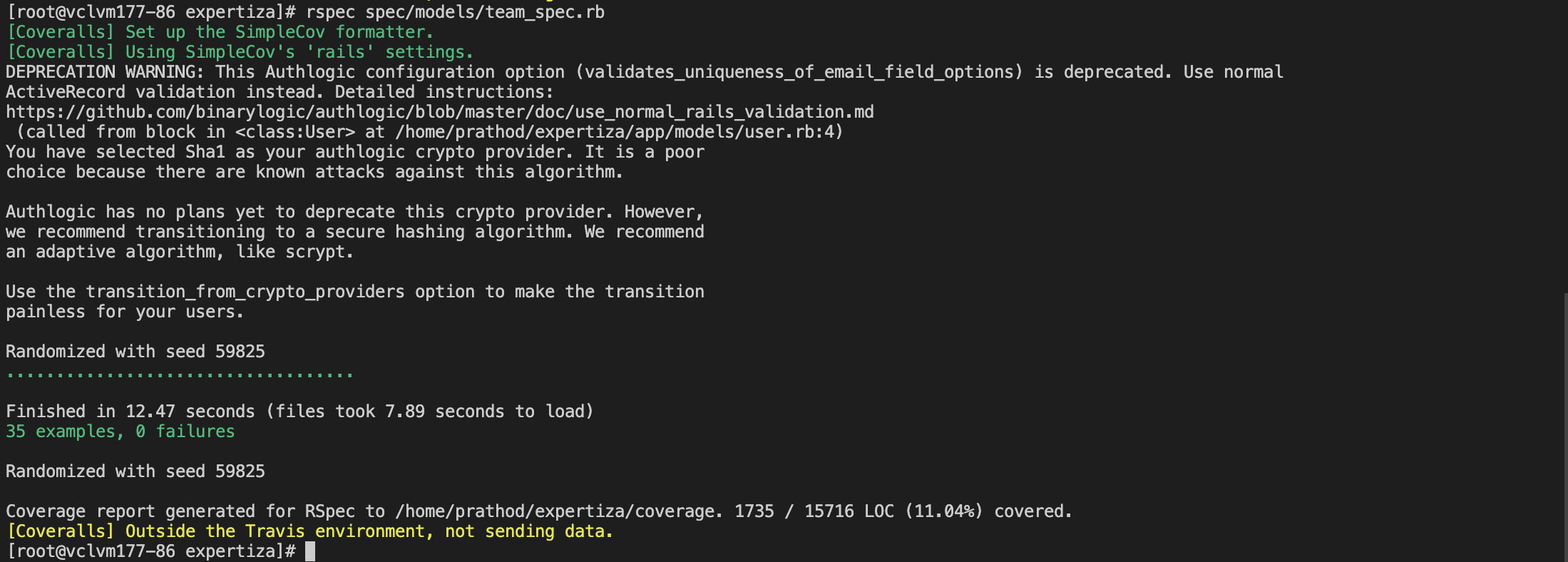
Relevant Links
- Github Repository: https://github.com/palash27/expertiza/
- Pull Request: https://github.com/expertiza/expertiza/pull/2558
- VCL Server: http://152.7.177.86:8080/
Credentials
Username: instructor6
Password: password
Contributors to this project
- Palash Rathod (unityid: prathod, github: palash27)
- Neha Kale (unityid: nkale2, github: nehakale8)
- Vansh Mehta (unityid: vpmehta2, github: vanshmehta-7)