CSC/ECE 517 Spring 2022 - E2232: Revision planning tool
Project Goal
The primary objective for this project is to create a tool that can be used for the revision of projects at a time after their original submission upon the delivery of constructive feedback from their peers or instructors. The revision planning tool is an important device that will be used to give students the ability to learn from the mistakes of their submissions, and improve the quality of their work prior to the due date. This will be done by completing the existing implementation for revision planning using the following project plan.
Project Plan
To merge code for revision planning into current beta.
E2152's work was to develop a revision planning tool and merge it into the beta branch of Expertiza. They completed their task but developed based on a previous beta branch version and thus could not be merged into the current beta. Our goal is to reimplement their change so that it merges into the current beta branch. We will first merge the modification in the following files to the current beta and solve the conflicts.
Files to be merged
- app/controllers/revision_plan_questionnaires_controller.rb
- app/models/team.rb
- app/controllers/grades_controller.rb
- app/helpers/grades_helper.rb
- app/models/assignment_participant.rb
- app/models/response_map.rb
- app/views/grades/_participant_charts.html.erb
- app/views/grades/view_team.html.erb
- app/views/student_task/view.html.erb
- app/controllers/response_controller.rb
- app/views/response/response.html.erb
- config/routes.rb
- db/schema.rb
- spec/models/response_spec.rb
- spec/models/review_response_map_spec.rb
- spec/features/assignment_creation_general_tab_spec.rb
- app/models/revision_plan_team_map.rb
- spec/controllers/advice_controller_spec.rb
- app/models/response.rb
Current Project Implementation
The implementation of this now fits within the framework created by E2161 (Fall 2021).
What it does: In the first round of Expertiza reviews, we ask reviewers to give authors some guidance on how to improve their work. Then in the second round, reviewers rate how well authors have followed their suggestions. Authors are now able to leave a plan of work attached to their reviews in order to indicate their plan to move forward with the criticism given by the peer reviews. This is done by utilizing controller classes for advice and response that are tasked with creating the objects, as implemented in the advice and response model classes respectively, saving them to the database and displaying them in the html files. We also ensure that response can only be performed by the appropriate members by indicating that actions are only permitted by those with instructor privilege (teaching assistants, admins, and instructors) as well as the student who has been assigned the review can alter them (seen here).
def action_allowed? questionnaire = Questionnaire.find(params[:id]) if(user_logged_in? && questionnaire.owner?(session[:user].id)) return true end current_user_has_ta_privileges? end
Helper methods such as summary_helper.rb are used in order to receive values from existing objects, for example receiving the sentences as broken up into seperate array entries as is needed for the comments of the answers in the reviews.
def get_sentences(answer) if answer.nil? return nil end sentences = answer.comments.split(/[.,?,!]/) sentences.each{ |sentence| sentence.strip! } sentences end
Rationale
The general workflow will be maintained from the previous iterations working on this project. The workflow after merging the past pull request is as follows.
Previous implementation
This project was last done in Fall 2021 (E2152). However, related merged code from E2161 (link above) means the implementation this semester may need to be changed from how E2152 did it.
- Primary Pull Request for E2152
- Second Pull Request for Revision Planning
- Previous write up for E2152
Current Flow
Current flow is dictated by previous iterations. The following content and images are created using those previous write ups.
Prior to the round 2 submission, you could view your work, but not the revision plan.
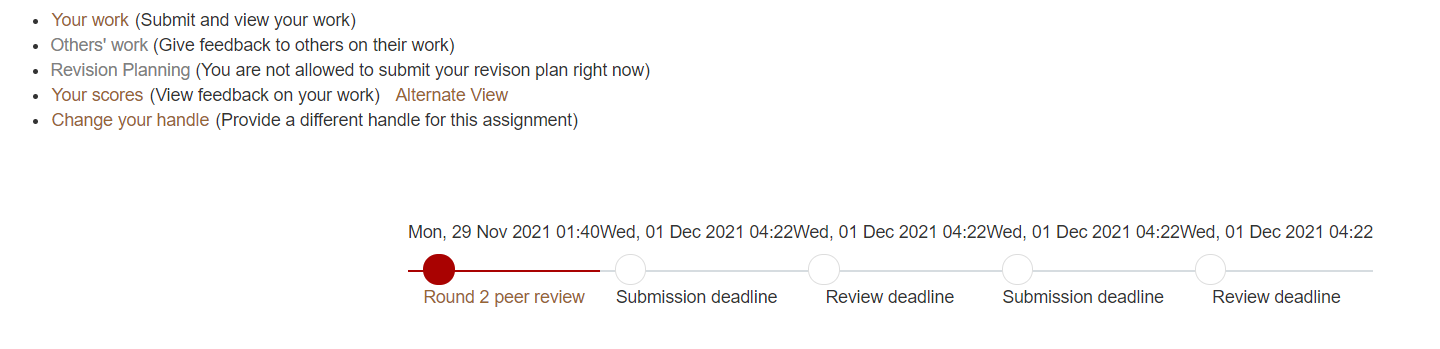
If there is a round 2 submission, and we did not do the "Revision Planning", then "Your work" becomes gray.
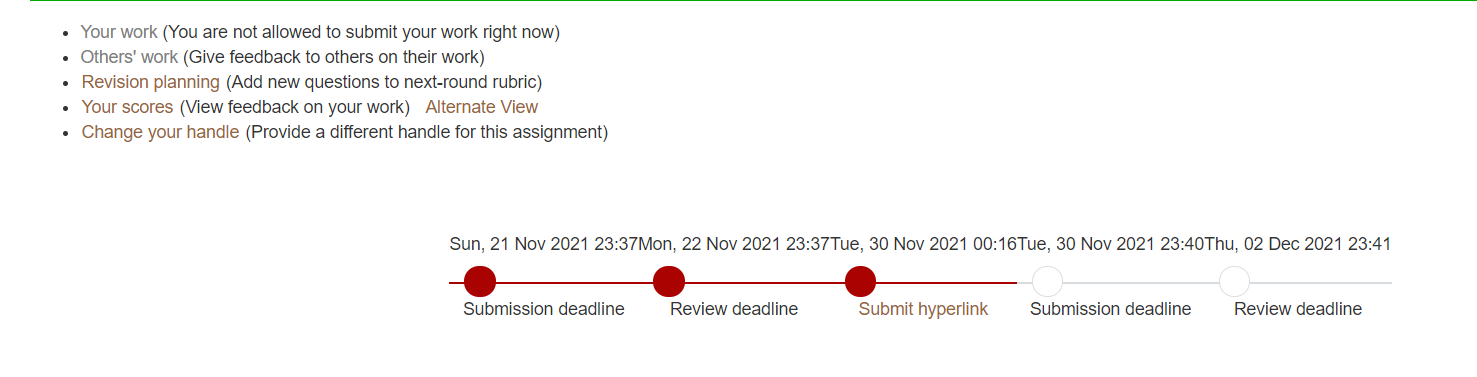
After editing the "Revision Planning", we can submit our work.
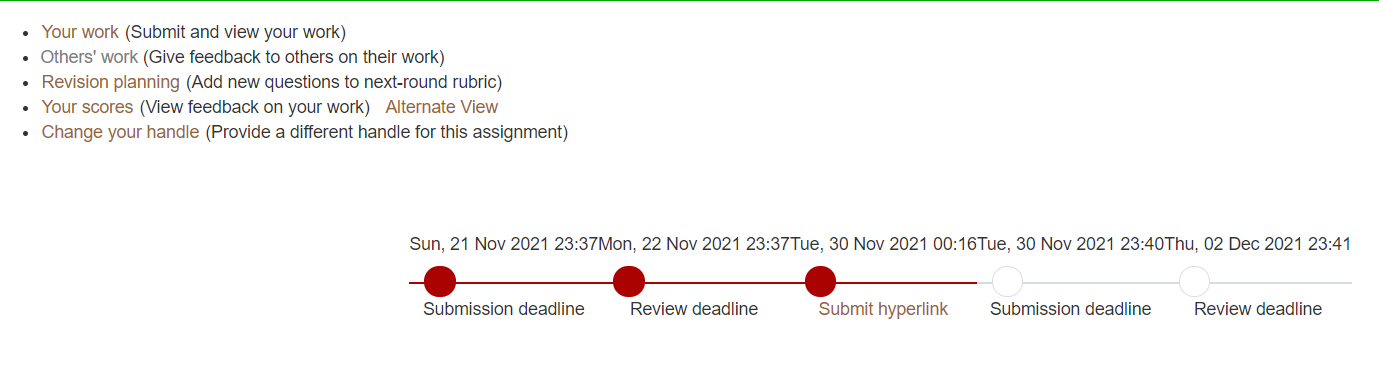
Current User Interface
Current user interface has been put in place by the previous iterations, the following interface image is from those iterations.
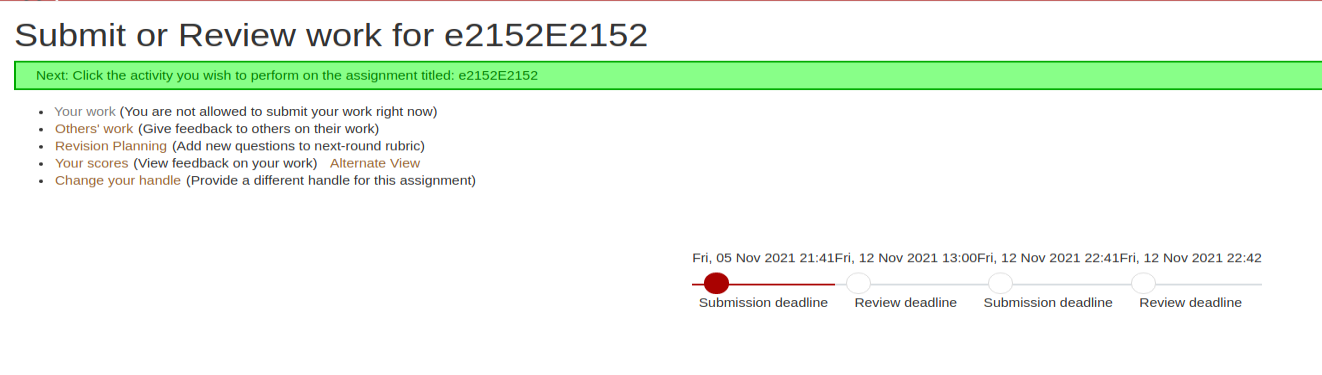
Design Changes
Because the changes to the current implementation is limited to specific implementation, the UML design of the project will remain the same as the previous implementation.
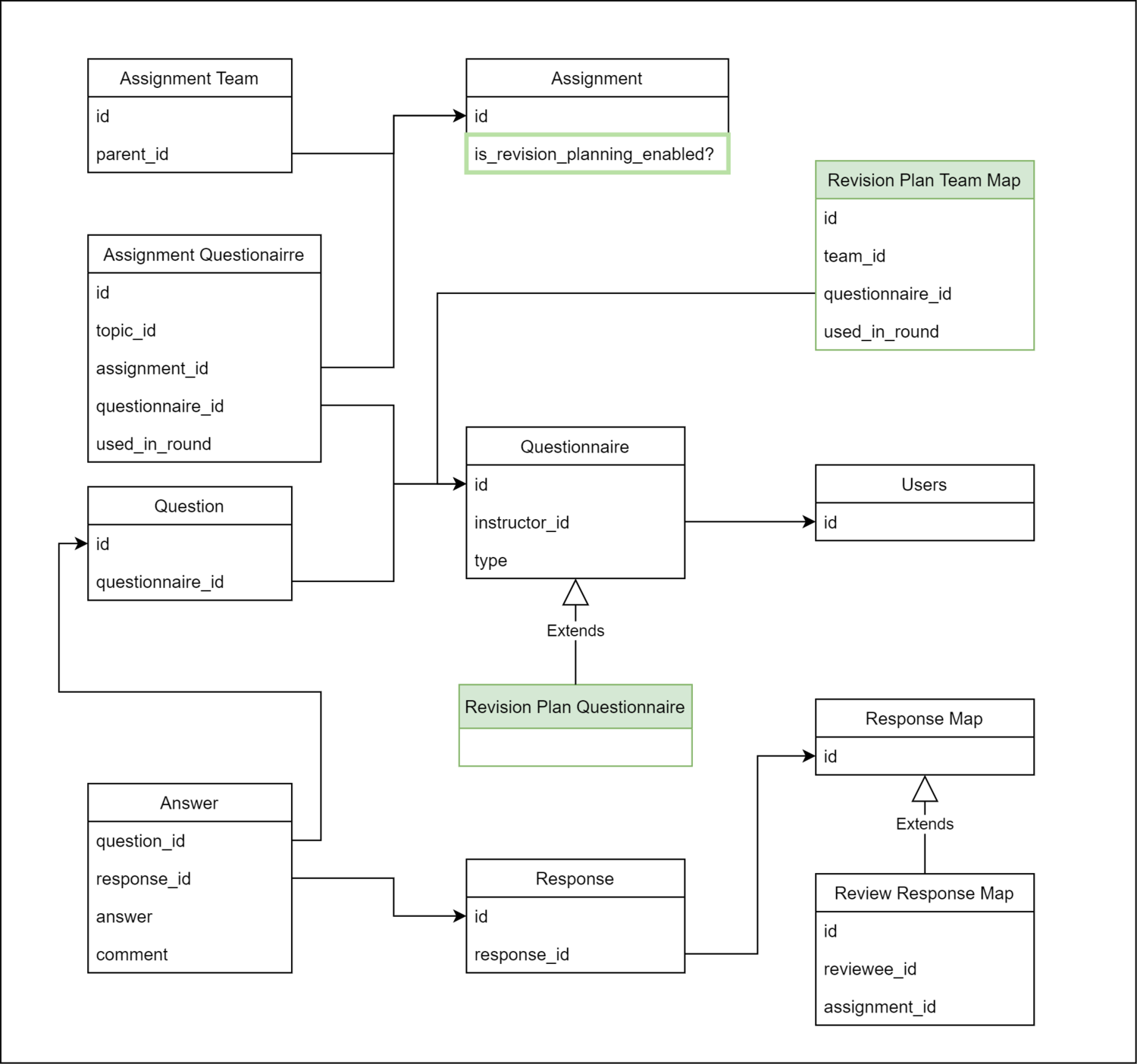
Test Plan
Merge existing RSpec tests for revision planning into current beta
We will first merge the existing RSpec tests of E2152 to the current beta, then run and pass these tests. More comments can be made in rspec tests as well. Observe the coverage of the individual tests. Existing RSpec tests to be merged
- Controllers
- rspec spec/controllers/advice_controller_spec.rb
- rspec spec/controllers/grades_controller_spec.rb
- rspec spec/controllers/questionnaires_controller_spec.rb
- rspec spec/controllers/questions_controller_spec.rb
- rspec spec/controllers/student_teams_controller_spec.rb
- rspec spec/controllers/response_controller_spec.rb
- rspec spec/controllers/revision_plan_questionnaires_controller_spec.rb
- rspec spec/factories/revision_plan_factory.rb
- Models
- spec/models/response_spec.rb.
- spec/models/review_response_map_spec.rb
- Helpers
- rspec spec/heplers/grades_helper.rb
Develop New RSpec Tests
The RSpec tests are written to test both controllers and models. RSpec testing will be added in order to increase coverage. To do this we will test the flows associated with different user types. Currently the only passing tests are related to student flows and tests may be added that work with instructors. These may include editing the reviews once they are created, and ensuring that an instructor has the ability to make edits.
Testing is done to ensure actions are allowed for teachers as well as the assigned student:
describe '#action_allowed?' do let(:questionnaire) { build(:questionnaire, id: 1) } context 'when the role of current user is Super-Admin' do # Checking for Super-Admin it 'allows certain action' do controller.params = { id: '1' } stub_current_user(super_admin, super_admin.role.name, super_admin.role) expect(controller.send(:action_allowed?)).to be_truthy end end context 'when the role of current user is Instructor' do # Checking for Instructor it 'allows certain action' do controller.params = { id: '1' } stub_current_user(instructor1, instructor1.role.name, instructor1.role) expect(controller.send(:action_allowed?)).to be_truthy end end context 'when the role of current user is Student' do # Checking for Student it 'refuses certain action' do controller.params = { id: '1' } stub_current_user(student1, student1.role.name, student1.role) expect(controller.send(:action_allowed?)).to be_falsey end end end
Testing is also done in order to ensure this in response controllers, and questionnaire controllers. Testing is done for model objects such as displaying as html (below) and ensuring fields are correctly returned
context 'when prefix is not nil, which means view_score page in instructor end' do it 'returns corresponding html code' do allow(response).to receive(:questionnaire_by_answer).with(answer).and_return(questionnaire) allow(questionnaire).to receive(:max_question_score).and_return(5) allow(questionnaire).to receive(:id).and_return(1) allow(assignment).to receive(:id).and_return(1) allow(question).to receive(:view_completed_question).with(1, answer, 5, nil, nil).and_return('Question HTML code') expect(response.display_as_html('Instructor end', 0)).to eq('<h4><B>Review 0</B></h4><B>Reviewer: </B>no one (no name) '\ "<a href=\"#\" name= \"review_Instructor end_1Link\" onClick=\"toggleElement('review_Instructor end_1','review');return false;\">"\ "hide review</a><BR/><h5>Review Responses</h5><table id=\"review_Instructor end_1\" class=\"table table-bordered\">"\ "<tr class=\"warning\"><td>Question HTML code</td></tr></table><h5>Additional Comment</h5>"\ "<table id=\"review_Instructor end_1\" class=\"table table-bordered\"><tr><td></td></tr></table>") end end
rspec tests are also needed to validate saves for controller classes, and ensuring model functionality in response objects.
Manual Testing
- Instructor
- Can Review rubric varied by topic be enabled?
- Can different roles be chosen for each questionnaire?
- Can an assignment with revision planning enabled be created?
- Can an assignment with 2 rounds of review be set up?
- Assignment participant
- If the revision-planning rubric can be edited or not?
- Are participants allowed to create/edit revision plan when round 1+ (1 or greater than 1) reviews have finished?
- Is revision plan editing disabled when the assignment is in review stage?
- Does participants show a summary of score for revision plan after review deadline has expired?
- Assignment reviewer
- Does the rubric page show the topic-specific rubric?
- Does the rubric page show the revision plan rubric?
Team Information
- Lawrence O'Brien (lpobrien)
- Joshua Lin (jlin36)
- Weiqi Sun (wsun23)
- Wyatt Plaga (wgplaga)
- Mentor: Nicholas Himes (nnhimes)
Links
- Pull request: https://github.com/expertiza/expertiza/pull/2395
- Github repo: https://github.com/wsun23/expertiza/tree/E2232
- VCL: http://152.7.99.215:8080/
- Screencast: