CSC/ECE 517 Spring 2017/oss E1712
Introduction
This project is part of an educational web application called Expertiza. This wiki gives the details on the refactoring tasks undertaken as part of continuous improvement of Expertiza.
Background
Expertiza is an open source project managed by the faculty and students of NCSU. It is a web application which offers a platform for assignments and allows learning through peer reviews.
Motivation
In the existing code the InvitationController and the JoinTeamRequestsController do the expected task but the there is a lot of redundancy and duplicity in the code, and the comments in the code are few and sparse. The aim of our project was to reduce the complexity of the controller by removing redundant code adding comments so that the code is easy to understand and all the variables used in the code are clearly explained.
Current Implementation
Invitations Controller
Invitations controller handles the functions required to send invitations out to potential teammates for a project. Once a student decides to invite someone into his assignment team the controller performs some checks before sending out the invitations. These checks are performed in the create function. The current implementation has a lot of redundant code and many nested if statements. The ABC size of the method is also very high. The controller also has functions which take care of cases where an invitee accepts/declines an invitation. There is also an option to retract a sent invitation which is handled by the cancel function in the controller.
Tasks Identified
- Rename to invitations_controller.rb, in accordance with current naming convention.
- The ABC size for create method is too high and needs to be refactored along by removing other nested if's in create and update_join_team_request methods.
- Use find_by instead of find_by_name and find_by_user_id_and_assignment_id.
- Add comments explaining what each method does, and comments on how important variables are used.
- Pending invitations needs to be displayed in the same format as other tables.
- Feature tests need to be written for the controller
Join Team Requests Controller
JoinTeamRequests controller deals with the actions to be performed after a student comes across an advertisement to join a project team. Whenever a project team creates an advertisement for others to join their team, students can see a link to the advertisement in the signup sheet. Once the student clicks on the link to the advertisement the student can then request to join the team. The create function in the JoinTeamRequestsController handles the checks before sending out the request. Again, the ABC size for this method is very high as there a lot of nested if conditions. Also, there is duplicate code in some of the functions in this controller which can be moved to a separate function.
Tasks Identified
- Add comments on why some important variables are used.
- The ABC size for create method is too high.
- Nested if's need to be refactored.
- Duplicate code needs to be removed.
- Pending join team requests needs to be displayed in the same format as other tables
- Feature tests need to be written for the controller
- Decline request is not working: Post route is not defined
- JoinTeamRequest.find(params[:id]. This retrieval is common to five methods which are :show, :edit, :update, :destroy, :decline. Can be put into private before action
Change Specifics
The tasks identified above were implemented and the details are given below.
Invitations Controller
- A new private function check_users was added which performs some basic checks before the create function is called. In the original implementation, there were a lot of nested if conditions which checked if a user is valid, is a participant in the project, and if the team has slots available. All these checks were the cause of the nested if statements because they needed to be checked before an invitation is sent out to a student. The nested if's were removed by moving all these checks into the private function thereby reducing the ABC size of the create method.
- Nested if statements in the update_join_team_request were removed by breaking the nested statements into separate conditions and using return when the condition fails. For example, when checking if the user is valid, instead of using a nested condition to check validity, if the user is not valid then we throw an error message and return from the function.
- Call to the method find_by_user_id_and_assignment_id has been replaced by find_by and passing the user_id and assignment_id as arguments to find_by. The code snippet showing the change made is shown below.
Before refactoring
AssignmentParticipant.find_by_user_id_and_assignment_id(inviter_user_id, assignment_id)
After refactoring
AssignmentParticipant.find_by(user_id: inviter_user_id, parent_id: assignment_id)
- Styling was added to the pending invitations table in accordance with the existing tables so that the view is uniform across the entire page.
- The name of the controller was changed in accordance with the current naming convention and comments were added explaining why some important variables were used and also giving more clarity to whoever wants to modify the code in the future. There were very few comments earlier and they did not explain much about what was going on in the functions.
Join Team Requests Controller
- A new private function check_team was added which takes most of the initial checks that are performed before a new join team request is successfully created. This significantly reduces the ABC size of the create method as almost all the conditional statements are moved to the private function which is called before the create function is called. The nested conditionals are also removed by making use of return statements just as in the invitations controller methods.
- The methods index, show and new all have code which is repeated. Another private function respond_after was written which makes the code DRYer.
- The view here too contained tables which did not follow the styling that is present throughout the other sections of the page. Styling was added to the pending join team requests table to make it same as the other tables.
- This controller had very comments and most of the variables were not explained, especially the codes used for reply_status which is used when a new request is sent and updated is not explained at all. The status codes 'A' - awaiting a reply etc. are clearly commented. Comments were also added explaining important pieces of code.
- POST route added to decline action in the routes file
- Common statement in show, edit, update, destroy, decline
Before refactoring
@join_team_request = JoinTeamRequest.find(params[:id])
After refactoring added private method find_request
def find_request @join_team_request = JoinTeamRequest.find(params[:id]) end
Added before_action to the methods
before_action :find_request, only: [:show, :edit, :update, :destroy, :decline]
Testing
The below test cases written can be found at spec/features/invitation_check_spec.rb.
A student is initialised and an assignment is assigned to the student. This serves as a base for testing.
@user1 = User.find_by(name: "student2064") stub_current_user(@user1, @user1.role.name, @user1.role) visit '/student_task/list' # Assignment name expect(page).to have_content('final2') click_link 'final2' expect(page).to have_content('Submit or Review work for final2') click_link 'Signup sheet' expect(page).to have_content('Signup sheet for final2 assignment') # click Signup check button @assignment_id = Assignment.first.id visit "/sign_up_sheet/sign_up?id=#{@assignment_id}&topic_id=1" expect(page).to have_content('Your topic(s): Hello world! ') visit '/student_task/list' expect(page).to have_content('final2') click_link 'final2' expect(page).to have_content('Submit or Review work for final2') click_link 'Your team' expect(page).to have_content('final2_Team1') fill_in 'user_name', with: 'student2065' click_button 'Invite' expect(page).to have_content('Waiting for reply')
Test Cases
To invite a student with no topic
describe 'one student who signup for a topic should send an invitation to the other student who has no topic' do before(:each) do user = User.find_by(name: "student2065") stub_current_user(user, user.role.name, user.role) visit '/student_task/list' expect(page).to have_content('final2') click_link 'final2' click_link 'Your team' end it 'is able to accept the invitation and form team' do visit '/invitations/accept?inv_id=1&student_id=1&team_id=0' visit '/student_teams/view?student_id=1' expect(page).to have_content('Team Name: final2_Team1') end it 'is not able to form team on rejecting' do visit '/invitations/decline?inv_id=1&student_id=1' visit '/student_teams/view?student_id=1' expect(page).to have_content('You no longer have a team!') end end
To invite a student with a topic
describe 'one student who has a topic sends an invitation to other student who also has a topic' do before(:each) do user = User.find_by(name: "student2065") stub_current_user(user, user.role.name, user.role) visit '/student_task/list' expect(page).to have_content('final2') click_link 'final2' expect(page).to have_content('Submit or Review work for final2') click_link 'Signup sheet' expect(page).to have_content('Signup sheet for final2 assignment') visit '/sign_up_sheet/sign_up?assignment_id=1&id=2' visit '/student_task/list' click_link 'final2' click_link 'Your team' end it 'Student should accept the invitation sent by the other student and both have topics' do visit '/invitations/accept?inv_id=1&student_id=1&team_id=2' visit '/student_teams/view?student_id=1' expect(page).to have_content('Team Name: final2_Team1') end it 'student should reject the invitation sent by the other student and both have topics' do visit '/invitations/decline?inv_id=1&student_id=1' visit '/student_teams/view?student_id=1' expect(page).to have_content('Team Name: final2_Team2') end end
To retract an invitation
describe 'one student sends an invitation and retracts it' do before(:each) do visit '/student_task/list' click_link 'final2' click_link 'Your team' end it 'should remove entry from database' do before_count = Invitation.count click_link 'Retract' expect(Invitation.count).to eq(before_count - 1) end end
Feature Test Scenarios
Some of the possible test cases which can be used for the controllers are given below.
- Test for create method in invitations controller: Before sending out an invitation to another student there a lot of checks that need to be performed such as checking if the invitee is a valid user, if the student is a participant in the project etc. All these scenarios are tested and finally, we make sure that a new invitation has been created in the database. The image below gives a visual representation of all the checks performed before sending out an invitation.
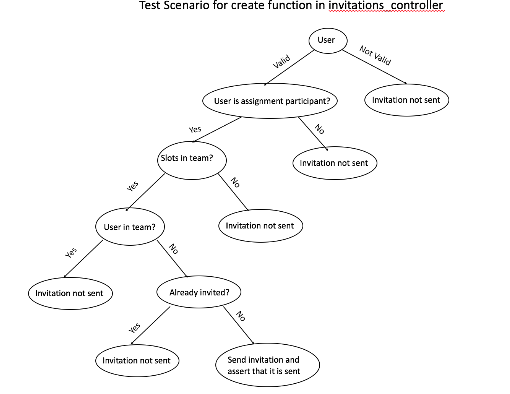
- Test for create method in join teams request controller: Similar to the create method in the invitations controller we need to do a lot of checks before sending out a new join team request. The below flow chart explains all the checks that need to be asserted before sending out a new request.
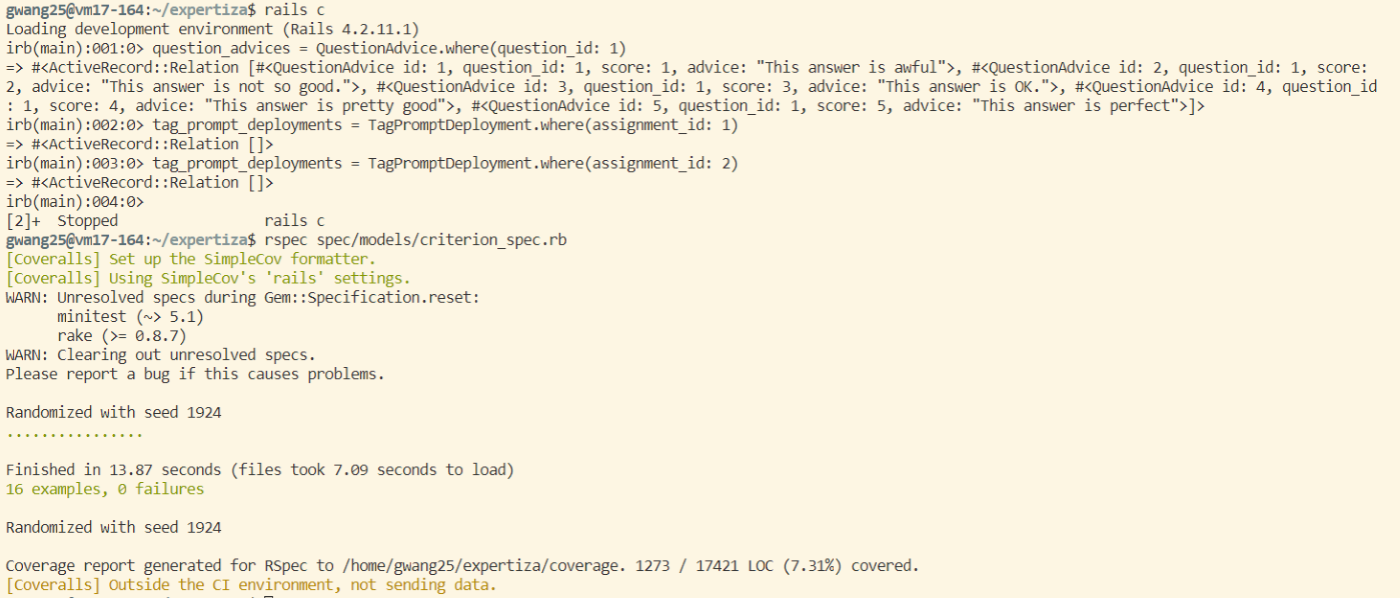
- Test for cancel method: The cancel method is called when the student who sends out an invitation wishes to retract the sent invitation. In this scenario the invitation is destroyed from the database and we assert that change by checking the change in the count of the database. The below diagram is attached for further clarity.
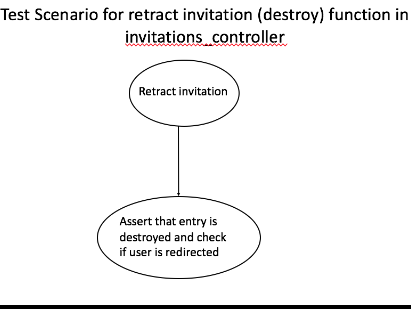
- Test for decline method: In this test, we assert the change of reply_status to 'D' in the database.
Further improvements to this project would be to write feature tests for the scenarios discussed above.
Running project remotely
VCL is used to host the project remotely. The login credentials as an instructor are "instructor6" with password "password". To log in as a student, you can use 'student5425' with password 'password'