CSC/ECE 517 Spring 2017/E1743
Problem Statement 1
Expertiza has a feature that allows instructor i (or an admin) to impersonate a user whose account was created by i or recursively by a user that i created. This causes Expertiza to show what the impersonated user would see. It is good for tracking down bugs in what students see and also for accessing functionality that cannot be accessed from, or is too slow to access from, the instructor UI. Impersonating is currently performed by going to the pull-down menu at the top of the window and selecting Manage > Impersonate user. However, usually the instructor initiates impersonation after finding the student in some kind of list, like a list of users, or assignment participants, or as a member of a team signed up for a topic, etc. In this case, it is inconvenient to have to go to the pulldown menu and type in the user ID. It would be better if the instructor could just click on the ID in the list, and immediately have the impersonated user’s homepage show up.
Here’s what it looks like:
The idea is to have more convenient options for instructors where a list of students is available, making it easier to both impersonate and view the details of any student with direct links.
Implementation 1
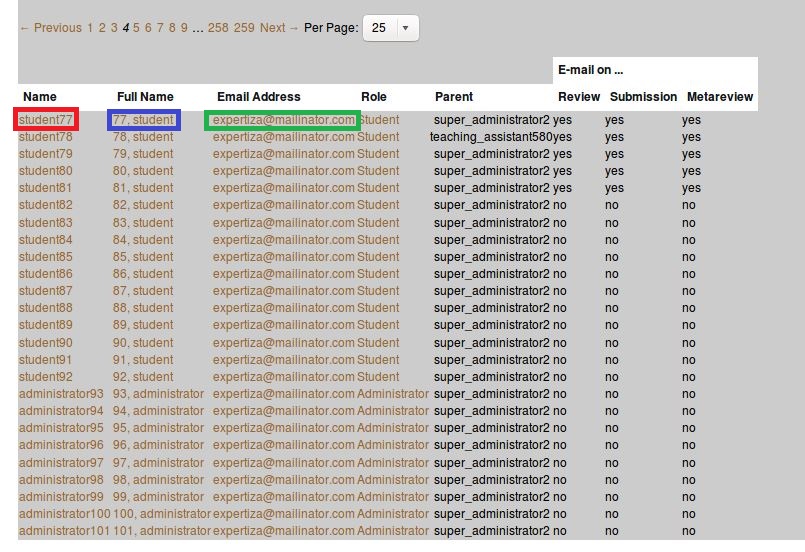
We have made the following changes to the system as shown by the screenshot above.
Red: The student ID previously had a link that directs to the user profile page. We have changed that to a link that would impersonate the user immediately. We did this by calling the impersonate function directly from this page and passing the user's ID as a parameter.
Blue: The user profile page link was shifted to this column instead. This column originally did not have any link on it.
Green: A link on the email address which now invokes a mailto: HTML link. This column also previously did not have any links.
The following screens did not previously show the user-ID, but we have modified them to do so.
- View scores (User IDs could be shown below names in the Contributor column.)
- View review report
- Author feedback report (available from the pull-down menu on Review report).
- Teammate review report ( “ “ “)
All these pages display some part of the user model, meaning they have all the data from the model. So, it was just a matter of displaying the appropriate user-ID.
Changes:
The changes we made on this part are the addition of lines printing the user-ID, and it was presented with a link which calls the impersonate function, using the user's ID as a parameter. The following snippet of code shows the exact lines we have changed. We have also switched the user profile link from the user-ID (what it was originally) to the user's full name. A mailto: HTML link has been added on the email address which generates a pop-up with the email ID.

Problem Statement 2
The second problem is that originally, the default for Manage > Users was to list all 6000+ users on one page, which lead to long delays. The default should be to show the first 25 users and there should be options to list 100, 250, or all users.
Purpose: The reason behind adding this feature is also to make it the list of users easier to read, possibly reduce retrieval and loading time, and improve ease of navigation.
Implementation 2
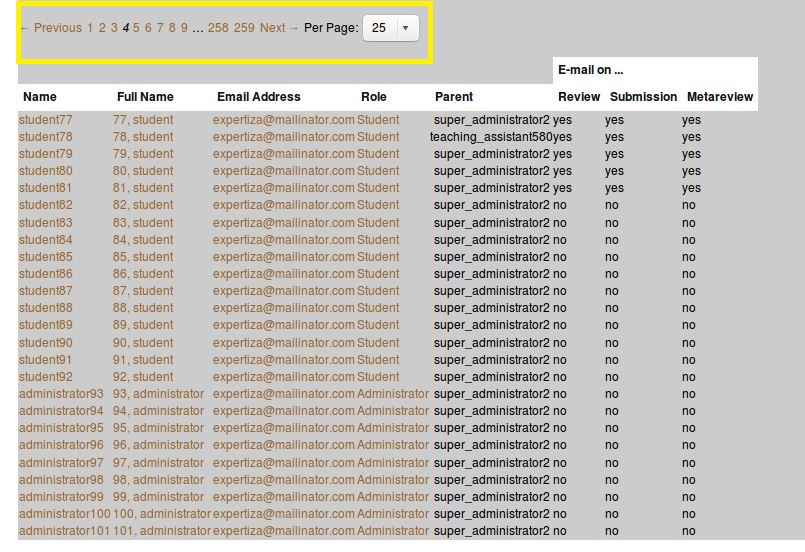
The yellow portion in the above screenshot is where we have added options to display 25, 100, 250, or all users at once, along with links to ‘previous’ and ‘next’ pages.
Changes:
There is a gem called will_paginate that adds a pagination library which integrates with ruby on rails. That gem is already added to the gemfile of expertiza, but was not used in this particular page. Once added, it provides a paginate method which automatically generates a paginated list with a per_page parameter. Then that list is passed to the view, which automatically displays the necessary links such as “previous”, “next”, individual page links, etc
We have reduced the complexity of the logic that was originally implemented. Previously, it populated the user list by querying the course and assignment models.
Here's what the code originally looked like:
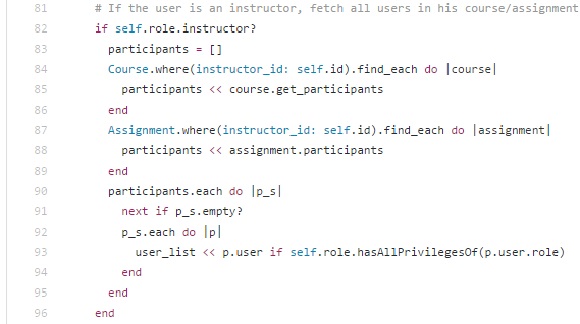
As suggested by Dr. Gehringer, we add a user to the user list array only if the currently logged in user is able to impersonate them.
Here's what we have changed that to:
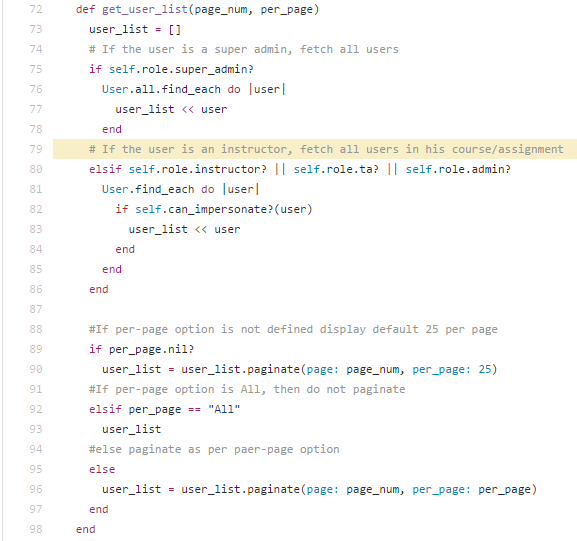
Test Plan
Most of the testing for this project is fairly simple.
Manual:
Problem 1: All of the altered links will need to be tested to ensure they link to the correct pages. Tests that check if the username link correctly initiates impersonation of that user, and that clicking the full name links to the right user profile.
A test user needs to be created and the email link will be used to ensure emails are correctly sent.
Problem 2: For the second part of the project, tests need to be set up to check that the correct number of students are being displayed for each display option, and that the user can display subsequent pages of users.
Automated:
Problem 1 includes changes in view files. Hence, we are not planning to create automated test cases for problem 1.
Problem 2: Unit Tests: We will add unit test cases in user_spec.rb. Case I: Condition: page number = 4 and per page option = 25 Result: Ensure users list contain 25 entries. Case II: Condition: page number = 1 and per page option = 50 Result: Ensure users list contain all entries.
Here's what we have added in the user_spec.rb file as test cases.
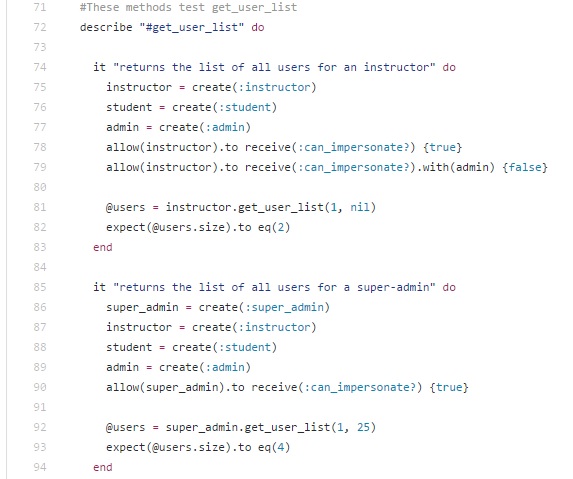
A summary of the above code:
Firstly, the objects for the user, instructor, and admin are created. In the subsequent lines, we create mocks for the impersonation logic to move the test forward. In the third part, the logic is actually tested.
Lines 74 to 83 test as an instructor, while lines 85 to 94 test as a super admin. A new object for a super admin was added in factories.rb
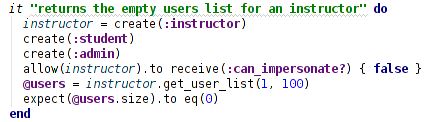
Also, we have added automated test case for negative scenario. In this case instructor is unable to impersonate any user and thus get_user_list method in user.rb returns an empty user list array.