CSC/ECE 517 Fall 2021 - E2159. Expertiza internationalization
Important Links
- Git PR - https://github.com/expertiza/expertiza/pull/2151
- Git beta branch - https://github.com/arnavjulka/expertiza/tree/beta
- Overall Video - https://drive.google.com/file/d/1dc9St9J77q-Mb_vRuYBg4vs9Xv0SHm4d/view?usp=sharing
- Testing Video - https://drive.google.com/file/d/1TOatjhe0wzxTpmWDuC_ryVFmtQ-X7FJq/view?usp=sharing
Introduction - Purpose & Problem
Currently, all Expertiza screens can only be viewed in English. Many Expertiza users are from other countries. This is a problem since this limits the accessibility of Expertiza. An important step in solving this problem is by making Expertiza available in more languages, which is a form of internationalization. W3C defines internationalization as “the design and development of a product, application or document content that enables easy localization for target audiences that vary in culture, region, or language.”
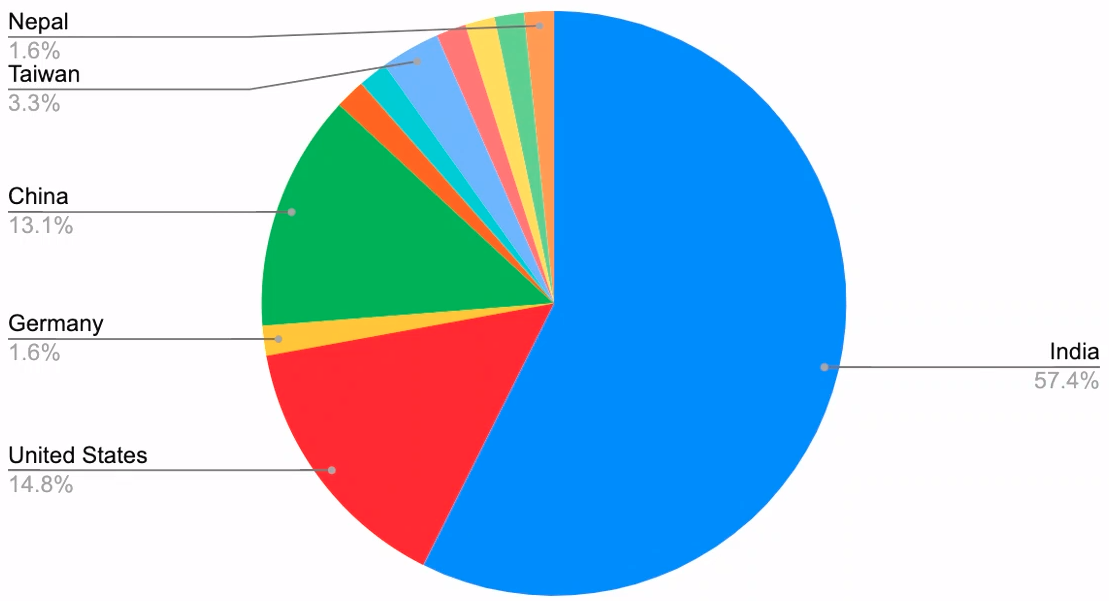
Survey of home country of Expertiza users for a given course
Scope: The scope of this project is limited however only to static strings. i.e. user-generated content will not be automatically translated to other languages.
Breaking down internationalization into 3 sequential subproblems:
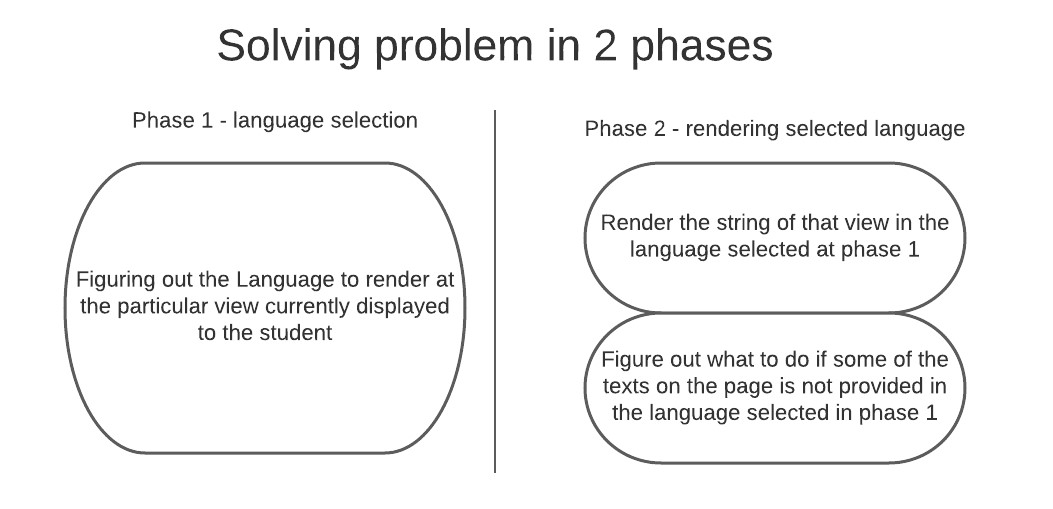
1. Language selection
Every user has an option to set a language preference in the User Profile Information Edit page.
There is also an option where the instructor can choose a default language for a course.
Thus, the language not only varies from user to user but also across screens for the same user. Thus we need to recompute the language in which the page is to be rendered. If the student has given a language preference, then the choice is given weightage over the course default language chosen by the instructor.
2. Rendering the language
How do we go about rendering the screen in that language?
In the previous step, we’ve identified the language in which a view is to be rendered. It is important to note that this language may not only differ for a different user, but also for a different page for the same user. The question now becomes, how do we render the screen in this language?
To tackle this question, we explore 2 fundamental concepts:
2.1 Views are composed of independent language elements
While this is something we typically take for granted, it becomes especially important in the context of translation. That is, a view is not a single contiguous block of text, nor is it an atomic visualization of information (unlike a screenshot), but rather a carefully organized collection of individual elements such as texts, buttons, etc that each contain language that needs to be translated separately. Crucially, this means that we cannot swap out a page in one language in its entirety for a page in another language in its entirety. Instead, each individual element needs to be swapped out individually so as to only impact the language and not the structural composition of the page itself.
2.2 Views require translation at a large number of call sites
Given that a view is composed of individual elements, this also means that the computation of how to translate such an element such as a button’s text would need to be done separately for each element. This would result in not only a single centralized
3. Translation gaps
What if the translation for the target language is not available? Even translation for a language like Hindi may be available in general, the translation for a given text may not be available Hindi. This may be due to a variety of reasons:
- The specific text may have been accidentally missed out (remember the translation is not being done at runtime through an API, but rather curated by the developer)
- The given text is non-trivial to translate to the target language
Our design would thus need to accommodate this by seamlessly falling back on another language whose translation is available.
Scope
The scope of this project is limited however only to static strings. I.e. user-generated content will not be automatically translated to other languages.
Design
Proposed Solution
We explore and solve each of the three problems above in isolation:
1. Language selection
The language in which the page is rendered depends on a set of conditions.
The below functions are added and called before page rendering to bring in this logic.
before_action :set_locale def set_locale # Checks whether the user is logged in, else the default locale is used if logged_in? # If the current user has set his preferred language, the locale is set according to their preference if @current_user.locale != "no_pref" I18n.locale = @current_user.locale # If the user doesn't have any preference, the locale is taken from the course locale, if the current page is a course specific page or else default locale is used elsif current_user_role? && current_user_role.student? && ["student_task", "sign_up_sheet", "student_teams", "student_review", "grades", "submitted_content", "participants"].include?(params[:controller]) set_new_locale_for_student else I18n.locale = I18n.default_locale end else I18n.locale = I18n.default_locale end end def set_new_locale_for_student # Gets participant using student from params if !params[:id].nil? || !params[:student_id].nil? participant_id = params[:id] || params[:student_id] participant = AssignmentParticipant.find_by(id: participant_id) # If id or student_id not correct, revert to locale based on courses. if participant.nil? find_locale_from_courses return end # Find assignment from participant and find locale from the assigment assignment = participant.assignment if !assignment.course.nil? new_locale = assignment.course.locale if !new_locale.nil? I18n.locale = new_locale else I18n.locale = I18n.default_locale end else I18n.locale = I18n.default_locale end else find_locale_from_courses end end def find_locale_from_courses # If the page is a course or assignment page with no specific student id, every course of that student is checked and if # the language for all these course are same, the page is displayed in that language courseParticipants = CourseParticipant.where(user_id: current_user.id) courseParticipantsLocales = courseParticipants.map { |cp| cp.course.locale } # If no tasks, then possible to have no courses assigned. if courseParticipantsLocales.uniq.length == 1 #&& !@tasks.empty? course = courseParticipants.first.course if course.locale? I18n.locale = course.locale else I18n.locale = I18n.default_locale end else I18n.locale = I18n.default_locale end end
2. Rendering the language
Once we have determined the language in which to render the page, we now need to update the rails view code to actually generate the appropriate HTML in the target language. This is the responsibility of the view. However, currently, the actual content of the HTML is hardcoded in (red) English. For example:
<h2>Summary Report for assignment: <%= @assignment.name %></h2> <h4>Team: <%= @team.name %></h4>
As discussed earlier, “Views are composed of independent language elements” and so we cannot simply swap out the entire page content for a separate page in another language (Why? - See “swap out the entire view” in the alternative approaches section):
<h2>Rapport de synthèse pour l'affectation: <%= @assignment.name %></h2> <h4>Équipe: <%= @team.name %></h4>
Instead, the better approach is to have a single unified view that dynamically changes based on the selected language:
<h2><%=language==’en’ ? ‘Summary Report for assignment’ : ‘Rapport de synthèse pour l'affectation’>: <%= @assignment.name %></h2> <h4><%=language==’en’ ? ‘Team’ : ‘Équipe’>: <%= @team.name %></h4>
However this is clearly infeasible considering the number of call sites where we have to apply this logic. Thus, in order to maximize how concise the call site is, we can pull out this logic into a common helper function:
<h2><%=localized(“report_summary.page_header”)>: <%= @assignment.name %></h2> <h4><%=localized(“report_summary.team_label”)>: <%= @team.name %></h4>
Here the assumption is that the localized(“key”) is aware of the currently selected language and that “report_summary.page_header” is the key for the header which is available in English and Hindi. The helper intelligently selects and returns the right text based on the currently selected language. Through this approach, we are able to render the view in multiple possible languages without any significant increase in the verbosity of the view.
What’s more, is that the above problem is a common problem for user-facing applications and a standard library for doing the above and much more already exists for rails applications called i18n
I18n - Internationalisation, How are we using I18n in Ruby on Rail
I18n is a ruby library/gem which helps us render the static string on the view dynamically. Dynamicity means that based on a local decision of choosing the current language of the view, the library returns the strings of that particular language at runtime.
These strings, instead of hardcoding in the view.rb, are provided in external .yml files. Now at the View level, we can pass an id to the 't' function and the I18n library returns the string for that id of the current set language(called as locale).
For this purpose, we have to provide .yml files for each language and in those language-specific .yml, we have to provide a map of key-value pairs where the key is the id of the string and value is the string in that particular language. By default the language or locale is set to be English(:en).
THE GREATEST BENEFITS of using this library are -
- The strings aren't hardcoded in the code/build. The strings are being fetched from external files which can be easily modified. For example, if one needs to change the text from 'Please Help' to 'Help', then one just needs to make a change in the .yml file and the change would reflect on the browser. For this change, we didn't need to redeploy the server. We just Decoupled the view when and where from what content is to be shown.
- Adding more languages is now super easy. Adding support for another language is just one line change in the config and adding a .yml file (having all the key-value pairs for the strings) for the new language. By doing this we can extend the language support as much as we want.
3. Translation gaps
Also, if the language is set to be Hindi or any other language but the key-value pair is not set for that particular string then, automatically the library returns the default which is the English text.
config.i18n.fallbacks = [:en]
Alternate approaches
1. Why not simply swap out the entire view for another language instead of each text?
A view is an organized collection of multiple separate elements, views often involve dynamic generation and some level of sophistication. If we were to swap out one page in its entirety for another page this would often violate the DRY principle since we would have to have two separate implementations of the view in each language. Thus in the worst case, you have a complex view that has to be replicated dozens of times in various languages.
The majority of complexity of the page is not in the content itself but rather the code that executes to generate that content, thus it makes sense to instead maintain a single view and embed the language switching logic at each location where translation needs to occur to avoid replication of everything around it.
2. Why did we pick i18n over implementing it ourselves?
Doing it ourselves felt like a great option. But i18n not just selects a language string at runtime for us it does a lot more under the hood which makes our lives easier. For example, it manages locale over the session for us, even if we change the urls and shift tabs and other stuff it manages our locale.
Also there is a great principle that says - “ If it isn’t broken, Don’t fix it”. The library manages the registering of different language yml files, points to the right right text at runtime, manages session locale and a lot more. So we want to explore this library instead of reimplementing the same thing again.
3, Why did we store the default language choices in database instead of in session?
We had to decide on whether to keep the student’s language preference in the database or in the session. We have thought about both approaches and below listed are the advantages and disadvantages.
In the work done by the previous team, they added a dropdown in the navbar where a student can choose the preferred language. One of the changes that we did was to move this field to the user profile page. The earlier team used session storage to store the preferred language. This session resets on every logout, but since the preferred language can easily be set from any page this wasn't much of an issue.
If we are moving the language preference field to the user profile page, it will create some user experience issues if the preference is stored in session. The student will have to visit the user profile page every time they log in. To avoid this scenario, it’s better to keep the language preference in the database, so that the preference stays saved across sessions. The disadvantage of moving the language preference to the database is that it brings forth a schema change and this always requires a very careful going through to ensure that nothing else is affected.
The main advantage of storing the language preference in session storage is that no schema change is involved. This decreases the chance of other features being affected. The main disadvantage is that the student will have to go to the user profile page every time they log in to set the preference, causing a user experience issue. Keep in mind that we’re moving the field away from the navbar to a user profile page. After considering these points, we finally decided to go store the user preference in the database itself as the project requires us to add a language preference field in user profile page.
Use Case Diagram
Major design patterns and principles used
1. DRY: The i18n ‘t’ function
We observe an extreme case of DRY with the t function of i18n.
For some background, the t function of the library takes in a key representing a text and returns the content referred to by that key in the preferred language of the user. It also performs many other functions such as falling back to a backup language if the translation for the preferred language has not been configured for that specific key. Thus since this logic has to be applied at every element of the view, it would behove us to extract this logic into a reusable function which is what the designers of the i18n library have employed
The I18n library gives us an option to have many fall back options. When the required text for a given language is not found, we then move on to find the text for the next language in the chain and so on until we find it. And in the end we will put english as default language. So if the key value pair for the text is not found in none of the yml files then the text will be fetched from the English language yml file.
3. Open closed principle & Strategy Pattern
As discussed in the “Language selection" design proposal above, if the user has not selected a preferred language, we do not simply default the view to render in the application language (English). Instead, the project requirements state that for course specific screens such as the assignment view, we would need to render the view in the course’s language.
While we could implement this code into the application controller that checks the controller being access and accordingly applies overrides on the default language, this violates the open closed principle since we would need to extend this logic any time we introduce a new course specific screen. Instead a better approach is to delegate the decision of selecting the language to the view itself since the view would be most aware of whether the view is course specific or not. We can also preserve the DRYness through mix-ins.
Database Design
We add the locale field to the users and courses tables which has the default values 0 and 1, which is no preference and en_US respectively.
The migrations will look as follows:
class AddLangLocaleToUsers < ActiveRecord::Migration def change add_column :users, :locale, :integer, default: 0 end end
class AddLocaleToCourses < ActiveRecord::Migration def change add_column :courses, :locale, :integer, default: 1 end end
Database Modification
We add a new integer field to the users table to store the user preferred language. The integer will be hashed to the supported languages. The default value in the table will be 0, which will be no_pref.
enum locale: { no_pref: 0, en_US: 1, hi_IN: 2 }
We also add a new integer field to the courses table to store the course preferred language. The integer will be hashed to the supported languages. The default value in the table will be 1, which will be en_US.
enum locale: { en_US: 1, hi_IN: 2 }
Hence the updated courses table will look like following:
create_table "courses", force: :cascade do |t| t.string "name", limit: 255 t.integer "instructor_id", limit: 4 t.string "directory_path", limit: 255 t.text "info", limit: 65535 t.datetime "created_at" t.datetime "updated_at" t.boolean "private", default: false, null: false t.integer "institutions_id", limit: 4 t.integer "locale", limit: 4, default: 1 end
While the updated users table will look like the following:
create_table "users", force: :cascade do |t| t.string "name", limit: 255, default: "", null: false t.string "crypted_password", limit: 40, default: "", null: false t.integer "role_id", limit: 4, default: 0, null: false t.string "password_salt", limit: 255 t.string "fullname", limit: 255 t.string "email", limit: 255 t.integer "parent_id", limit: 4 t.boolean "private_by_default", default: false t.string "mru_directory_path", limit: 128 t.boolean "email_on_review" t.boolean "email_on_submission" t.boolean "email_on_review_of_review" t.boolean "is_new_user", default: true, null: false t.integer "master_permission_granted", limit: 1, default: 0 t.string "handle", limit: 255 t.text "digital_certificate", limit: 16777215 t.string "persistence_token", limit: 255 t.string "timezonepref", limit: 255 t.text "public_key", limit: 16777215 t.boolean "copy_of_emails", default: false t.integer "institution_id", limit: 4 t.boolean "preference_home_flag", default: true t.integer "locale", limit: 4, default: 0 end
The locale field is the last column on both tables:
courses:> t.integer "locale", limit: 4, default: 1
users:> t.integer "locale", limit: 4, default: 0
Test Plan
Manual Testing
Through manual testing, we aim to identify if all the features of the application are working as intended when the language conversion occurs.
UPDATE: All of the below tests have been automated.
Scenario 1
1. Log in to Expertiza as a student.
2. Go to the “User Profile Information” page and change “Preferred Language” to Hindi.
3. Check to see if the English strings on the profile page are translated to Hindi.
4. Check if a page for which Hindi translation keys are added has its English static strings translated to Hindi.
Scenario 2
1. Log in to Expertiza as a student.
2. Go to the “User Profile Information” page and change “Preferred Language” to No Preferences.
3. Check to see if the English strings on the page are unchanged, as the “User Profile Information” translates only if the student has a preference.
4. Go to an assignment page for which Hindi translation keys are added, which is under a course with the default language set to Hindi, and check whether the English strings are translated to Hindi.
Scenario 3
1. Log in to Expertiza as a student.
2. Go to the “User Profile Information” page and change “Preferred Language” to Nil.
3. Check to see if the English strings on the page are unchanged, as the “User Profile Information” translates only if the student has a preference.
4. Go to a non-course-specific page and verify that the page is displayed in English itself, as non of the default languages set for any of the courses should have any role.
Scenario 4
1. Log in to Expertiza as a student.
2. Go to the “User Profile Information” page and change “Preferred Language” to Hindi.
3. Go to a page where translation keys aren’t added completely and make sure that the unspecified keys display strings in English as a fallback.
Automated Testing
The following screenshot describes the complete feature tests introduced as part of this project.
These feature tests cover all the functionality introduced as explained in the Manual Tests section above.
Testing Video - https://drive.google.com/file/d/1TOatjhe0wzxTpmWDuC_ryVFmtQ-X7FJq/view?usp=sharing
In addition to feature tests, we have also introduced unit tests for code that drives the I18n library:
Future Scope
1. Expand to more languages
As part of this project we have targetted Hindi as a second language, however our work makes it easy to extend to other languages as well.
2. Identify and implement the course language override on more course specific screens
As part of this project, we have introduced a generic framework by which any view can provide a preferred language in which it should be rendered, this is required by this project for the course language feature set by the instructor.
We have currently most course specific pages translated according to course language preference when the user has not provided any preference, but we need to identify if we have left out any other course specific page and add those to the list of pages which should take the course language setting into consideration.
Team
Team Members
Reuben M. V. John [rmjohn2@ncsu.edu]
Renji Joseph Sabu [rsabu@ncsu.edu]
Ashwin Das [adas9@ncsu.edu]
Arnav Julka [ajulka@ncsu.edu]
Mentor
Jialin Cui [jcui9@ncsu.edu]