CSC/ECE 517 Fall 2020 - E2064. Refactor reputation web service controller.rb
Introduction
Introduction to Expertiza
Expertiza is a peer review based system which provides incremental learning from the class. This project has been developed together by faculty and students using Ruby on Rails framework. Expertiza allows the instructor to create, edit and delete assignments, create new assignment topics, assign them to a particular class or selected students, have students work on teams and then review each other's assignments at the end. For the students, they can signup for topics, form teams, and submit their projects and assignments. Students then review the work done by other students and give suggestions to improve. Teams after reviews are allotted scores and they can refer to the peer comments to further improve their work. It also supports submission of different file types for assignments, including the URLs and wiki pages.
About Reputation Web Service Controller
Expertiza allows student work to be peer-reviewed, since peers can provide more feedback than the instructor can. However, if we want to assure that all students receive competent feedback, or even use peer-assigned grades, we need a way to judge which peer reviewers are most credible. The solution is the reputation system. Reputation systems have been deployed as web services, peer-review researchers will be able to use them to calculate scores on assignments, both past and present (past data can be used to tune the algorithms). For this project, our team's job is to refactor the file: reputation_web_service_controller.rb. This file is the controller to calculate the reputation scores. A “reputation” measures how close a reviewer’s scores are to other reviewers’ scores. This controller implements the calculation of reputation scores.
Issues to be fixed
reputation_web_service_controller.rb is a fairly complex file. It contains a lot of methods that are long and hard to understand (for e.g. send_post_request). These methods need to be broken down into simpler and more specific methods that are easier to read/understand. Also, the few instances of code duplication that exist should be removed.
- There is a lot of unused/commented code, which should be removed.
- Figure out what the code is doing and write appropriate comments for it.
- Rename bad method names such as (db_query, json_generator). Method names should be verbs, and should say what the method does. Method names should not be so general that they could apply to many different methods..
- In the case of db_query, the name should say what it queries for. Also, this method not only queries, but calculates sums. Since each method should do only one thing, the code for calculating sums should be in another method. And there should be comments in the code!
- json_generator should be generate_json. There needs to be a method comment saying what the parameters are.
- In send_post_request, there are references to specific assignments, such as 724, 735, and 756. They were put in to gather data for a paper published in 2015. They are no longer relevant and should be removed. send_post_request is 91 lines long, far too long.
- There is a password for a private key in the code (and the code is open-sourced!) It should be in the db instead.
- Fix spelling of “dimention”
- client is a bad method name; why is stuff being copied from class variables to instance variables?
Steps taken to resolve the issues
Refactor db_query
Renaming of bad method names such as (db_query
). Method names should be verbs, and should say what the method does.
Method names should not be so general that they could apply to many different methods. The other problem with the method was that it did not follow the good practice of having each method perform only one task. This method got the review responses with a db query. Also, it iterated over the review responses to perform a calculation of peer review grades.
We have split the method into two to have the review responses do only the get responses part.
The code for calculating sums should be in another method.
The result of this method is stored and we have created a new methodcalculate_peer_review_grades
and moved the calculation part to it.
We also added a comment to indicate the calculate weighted sum part.
And then, we have changed the call to the get review responses
and the new method calculate peer review grades accordingly
Similarly, we have changed db_query_with_quiz_scores
to calculate_quiz_scores
Change name of json_generator
json_generator method should be generate_json
Also,there needs to be a method comment saying what the parameters are. We have added the method comments with parameter information as seen below.
Refactor send_post_request
“In (send_post_request
), there are references to specific assignments, such as 724, 735, and 756.
They were put in to gather data for a paper published in 2015. They are no longer relevant and should be removed.
send_post_request is 91 lines long, far too long”
We observed that encryption of request being sent to evaluate Algorithm and decryption of response is written in (send_post_request
) only that is why send post is 91 lines long.
We have created two separate methods
(encrypt_request
)
(decrypt_response
)
In the image below we can see that we have separated Encryption functionality from (send_post_request
) to (encrypt_request
)
On the similar lines we have separated Decryption functionality from (send_post_request
) to (decrypt_response
)
(encrypt_request
) has been refactored as follows
(decrypt_response
) has been refactored as follows
Fixed spelling of dimention
Updating client method
We changed the class variables in the code to instance variables because we realized that the send_post_request method and client method were the only methods using the class variables. A client.html.erb file was used to call our controller to fill out a form for send_post_request which set values of class variables then immediately redirected control to the client method which set the instance variables to those class variables. The instance variables were then used in the rest of the client.html.erb file.
The process of setting class variables to instance variables with a function was redundant, so we removed simply set the instance variables within the send_post_request method and took them out of the client method.
The client method did have a few actions that it completed besides setting class variables which we have left in the method until we can find a solution to replacing them.
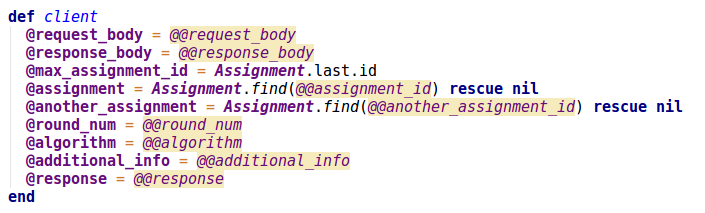
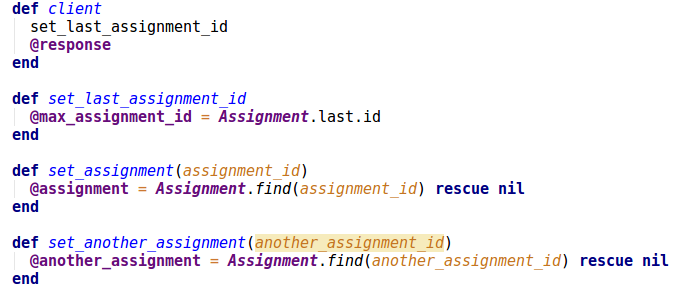
The reason why we kept the client method's name is because the routes.rb file specifies the client method in the code and we haven't fully tracked down the impact of that yet.
Testing
Test Plan
There was no existing tests available for the Reputation web service controller.
Since we are refactoring it, Our testing plan is to have an automated testing to ensure that the refactoring such as splitting a method into separate methods does not affect the existing implementation.
We also added steps for the manual testing to make sure the page loads as expected after the refactoring.
For the automated testing, We are using Rspec tests.
RSpec
We have tested the two new methods created during refactoring and also a renamed method.
The test coverage has increased a bit by 0.6% to 44%.
The tests we created can also be manually run with command
rspec spec/controllers/reputation_web_controller_spec.rb.
Manual UI Testing
The steps to test our branch are below, but they are failing right now due to errors that are in other files of the beta branch.
To test our program you must login to the site here: http://152.7.98.78:8080/
Username: instructor6 Password: password
Then the page "Manage content" doesn't show up, go to Manage... -> Assignments
Then on the CSC 517, Fall 2017 Row, click on "Add Participants" in the actions column. This is also indicated by a blue shirted person.
Once in there, copy on of the student names (such as student7487) and then go to Manage... -> Impersonate User and enter their name (student7487) into the text field.
After impersonating them, you should be able to see their assignments. Click on any of their assignments such as "Program 1", then click on "Alternate View" to the right of "Your Scores".
Modified Files
reputation_web_service_controller.rb
New Files
reputation_web_service_controller_spec.rb
Team Information
Project Mentor:
Saurabh Shingte
Project Members:
Abhishek Gupta
Skieler Capezza
Ummu Kolusum Yasmin Ahamed Adam