CSC/ECE 517 Fall 2016 E1691 ConvertAssignmentCreationFormtoReactJS
E1691 Convert Assignment Creation Form to ReactJS
This a page to describe a final project for ECE517. In this project we will be converting Assignment creation form to ReactJS.
Expertiza
Expertiza is an Open Source Software project developed by NC State University, funded by the National Science Foundation. It allows the instructor to create assignments as well as modify existing assignments. Students can signup for topics in an assignment and can submit articles, codes, web-sites etc. It is a web application built on Ruby on Rails framework. It also allows students to review the submissions that have been made other students.
React JS
ReactJS is an open source JavaScript library, used to provide a view for data rendered as HTML.It is maintained by Facebook, Instagram and a community of individual developers and corporations. React was created by Jordan Walke, a software engineer at Facebook. He was influenced by XHP, an HTML component framework for PHP. It was first deployed on Facebook's newsfeed in 2011 and later on Instagram.com in 2012. It was open-sourced at JSConf US in May 2013.
Advantages of ReactJS
- It is easy to know how a component is rendered, you just look at the render function. This render function basically implements html divs.
- JSX is a faster, safer and easer JavaScript which makes it easy to read the code of your components. It is also really easy to see the layout, or how components are plugged or combined with each other.
- React can be rendered on the server-side. So you can easily use it in the Ruby on Rails too.
- It is easy to test (easier than the traditional JavaScript or JQuery where you have to test the code in the Developer Tools) and it can easily be integrated with tools like jest which can make testing painless.
- It ensures readability and makes maintainability easier.
- It can be used with any framework such as Backbone.js, Angular.js, as it is only a view layer.
Dynamic Rendering
A client-side dynamic web page processes the web page using HTML scripting running in the browser as it loads. JavaScript and other scripting languages determine the way the HTML in the received page is parsed into the Document Object Model, or DOM, that represents the loaded web page. The same client-side techniques can then dynamically update or change the DOM in the same way.
Shown below is an example of a dynamically rendered webpage. The user is able to enter values, which are reflected in the view immediately after submission, without any redirection or reloading of the webpage. You can also see the timer running on the page, which demonstrates the ability to dynamically update variable values inside the view.
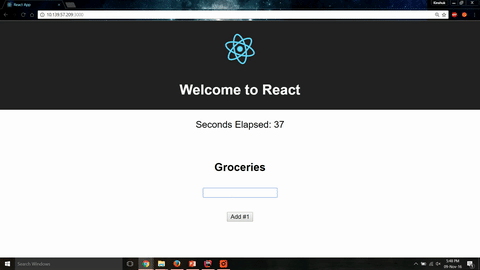
Assignment Creation
Current Implementation
The assignment creation view is currently implemented mostly in HTML and some data validations are done using JQuery. This implementation does not support dynamic view rendering which is essential to improving the user interface and contributing to the fluidity of the overall experience. For example, If the user wants to create a new assignment, clicking the new assignment link will generate a server request and the new view is rendered (url is changed). After filling up all the details required for the assignment creation, save link will again generate a server request which will make the database entry and again render a new view.
Proposed Solution
In the revised implementation, clicking on the New Assignment link generates a drop-down window, which contains the form for creating a new assignment. This form contains the same fields as the original form.
In addition, we have added data validations for two fields. The name of the assignment cannot be left blank, and the 'submission directory' field cannot be left blank or contain any special characters. If either of these conditions is violated, an error message is displayed to the user. Furthermore, these validations are done in the client-side itself, removing the need for a server request to validate the data.
Submitting the form will create a database entry and close the form.
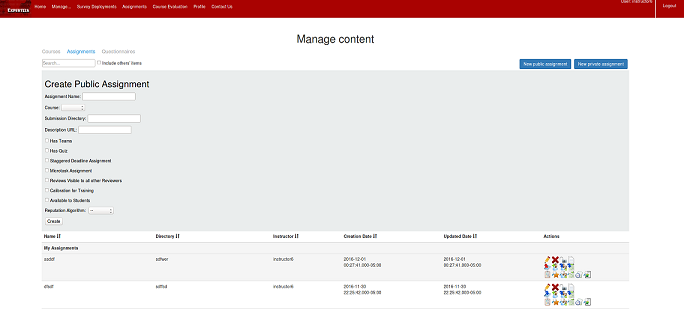
Changed files
- apps/assets/javascripts/tree_display.jsx.erb
- apps/controllers/assignments_controller.rb
- apps/controllers/tree_display_controller.rb
Details
We created a new React class NewAssignmentForm which dynamically creates and displays the form for New assignment. This class the following methods:
getInitialState function(){} - Here we identify the form parameters that will be sent to the database when a new assignment is created. handleNameChange function(e){} - The following methods get triggered on change of the form fields and capture/update the state variables handleCourseChange: function(e){} -//- handleSubChange: function(e){} -//- handleDescChange: function(e){} -//- handleTeamChange: function(e){} -//- handleQuizChange: function(e){} -//- handleDeadlineChange: function(e){} -//- handleReviewsChange: function(e){} -//- handleCalibrationChange: function(e){} -//- handleAvailabilityChange: function(e){} -//- handleReputationChange: function(e){} -//-
directoryValidate: function(e){} - this method validates that there is valid directory entered nameValidate: function(e){} - this method validates that a valid project name isentered
handleCreateAssignment: function(e) {} - this method sends ajax request with the parameters from the filled form.
handleGetCourses: function() {} - this method is triggered when a course selection drop down is clicked. It sends a jQuery request and gets all the courses based on the instructor role
render: function(){} - this method renders the form components
In assignment_controller.rb we edited create method:
@assignment_form.assignment.instructor_id = current_user.id -- We now pass current instructor to the assignment form.
In tree_display_controller.rb we added new method:
def get_courses_node_ng -- This method returns the course list array of hashes based on the instructor chosen. This logic is similar to the helper method course_options
Testing plan
1. Log in as an instructor and check that creating a new assignment works as expected
2. Log in as an instructor and check that the assignment could be created successfully and all the entered options have been preserved
Editing Assignment
Current Implementation
Similar to New assignment creation page Edit page does not support dynamic view rendering which is essential to improving the user interface and contributing to the fluidity of the overall experience. For example, If the user wants to edit the name of the assignment or change number of slots they have to click edit button. It will generate a server request and the new view is rendered (url is changed). After filling out all the details that changed, save link will again generate a server request which will make the database entry and again render a new view.
Proposed Solution
In the revised implementation, as the edit button is clicked a dynamic drop down form will be displayed. Saving the details will make the database entry. Except for the database update, all the processing is done on the client side itself, leading to a decrease in the number of server requests.
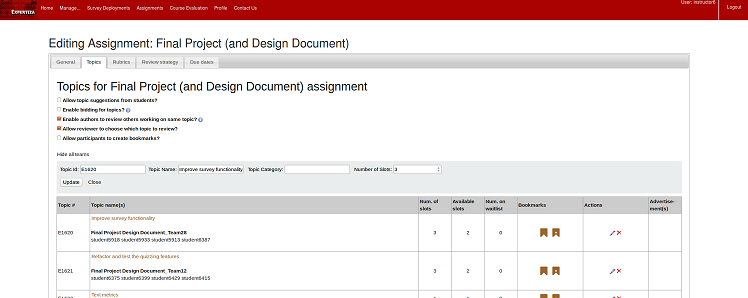
Files to be changed
- apps/views/tree_display/list.html.erb
- apps/views/assignments/edit.html.erb
- apps/views/assignments/edit/_general.html.erb
Testing plan
1. Log in as an instructor and check that editing an assignment works as expected
2. Log in as an instructor and check that an assignment could be successfully edited and all the existing functionality is preserved