CSC/ECE 517 Fall 2016/E1686-Timestamps for students' submissions
Problem Statement
In this project, We will keep track of timestamp records for students' submissions and updates of artifacts (links to google doc/wikipedia/github), reviewers' submissions and edits of peer-reviews and author feedbacks.We will also create a timeline view for each artifact, showing when it was submitted/resubmitted and when it was reviewed. With our work, users (students and instructors) can see:
- when do the authors submit and update their artifacts.
- when do reviewers submit peer-reviews.
- when do reviewees send author feedback.
- when do reviewers update their peer reviews.
Approach
From a perspective (both instructors and students), users should be able to see such a timeline with all the events for each submission of an assignment. The events can be -
- add/edit events of various submission links/files
- Reviews
- author feedbacks
- peer reviews
Each of these artifacts can be edited and updated multiple times. Because we want to track when these artifacts are updated, we have to store their information in the database. We use the table suggested in the problem description “submission_histories”-
- Id :: integer- primary key for this table.
- team_id :: integer ← foreign key to the teams table
- submitted_detail :: string ← details of the event. This can be one of x values.
- Link - if it is a assignment submission link. This can further be of 4 types -
- Google Docs
- Wikipedia
- Github repository
- Any other web link
- Review ids - if the event is a review submission
- Author feedback id - if the event is an add or edit operation on author feedback.
- Peer review id - if the event is an add or edit operation on peer review.
- Submitted_at :: datetime ← to store the timestamp of the event
- Type :: string ← field which distinguish between different types of events - to be used for polymorphism.
- Action :: string - add / edit / delete based on the event.
These are the common components under which each component in the timeline can be classified. Each of these components are explained in detail on how it fits into the timeline and its significance and types listed.
Data stored in submission_histories table
- Google Document, Wikipedia article, Github repository url : All of these are types of links given by the team during their submission. Through the url, we will identify which type of a submission is this, and then create an object of the particular class using the factory pattern. Each of these classes will implement their own “getLastUpdatedTimestamp” method, using the suggested APIs, through which we can get the required timestamp.
- File path and any other web link - These are also a part of main submissions, but since there is no way of tracking changes to these artifacts, we just take the time of the system when the link is added or removed.
- Peer Reviews, Author feedback: Peer reviews and author feedbacks are updated into the submission history table for every edit that takes place. The “updated_at” field acts as the current timestamp for edits in the Peer Reviews and Author feedbacks and the “created_at” field marks the start of these Events.
Data retrieved from existing tables
- Reviews: Timestamp for assignment reviews are taken from “responses” and “response_maps” table. The responses table contains round field and when “is_submitted” flag is true, the submission appears on the reviewee timeline where the “reviewee_id” retrieved from response_maps table. The reviews attached to the respective reviewee are collected and updated_at field gives the submission timestamp which is used on the timeline. Each review is collected and is added to the single Event map.
- Timeline Parameters: Values corresponding to an assignment which are common for a given Assignment are retrieved from the assignments table. And the timeline parameters are obtained by calculating from the created_at which is the start date for the assignment and the field rounds_of_reviews determines the deadline by adding up the value stored in days_between_submission field of the same table. The Events - Start Date, Submission Deadline and Revision Deadline and Final assignment deadline exists for each assignment.
All the components are then added into one single map - Event -> Timestamp which is sorted on timestamp values and is displayed on screen.
UML Diagram:
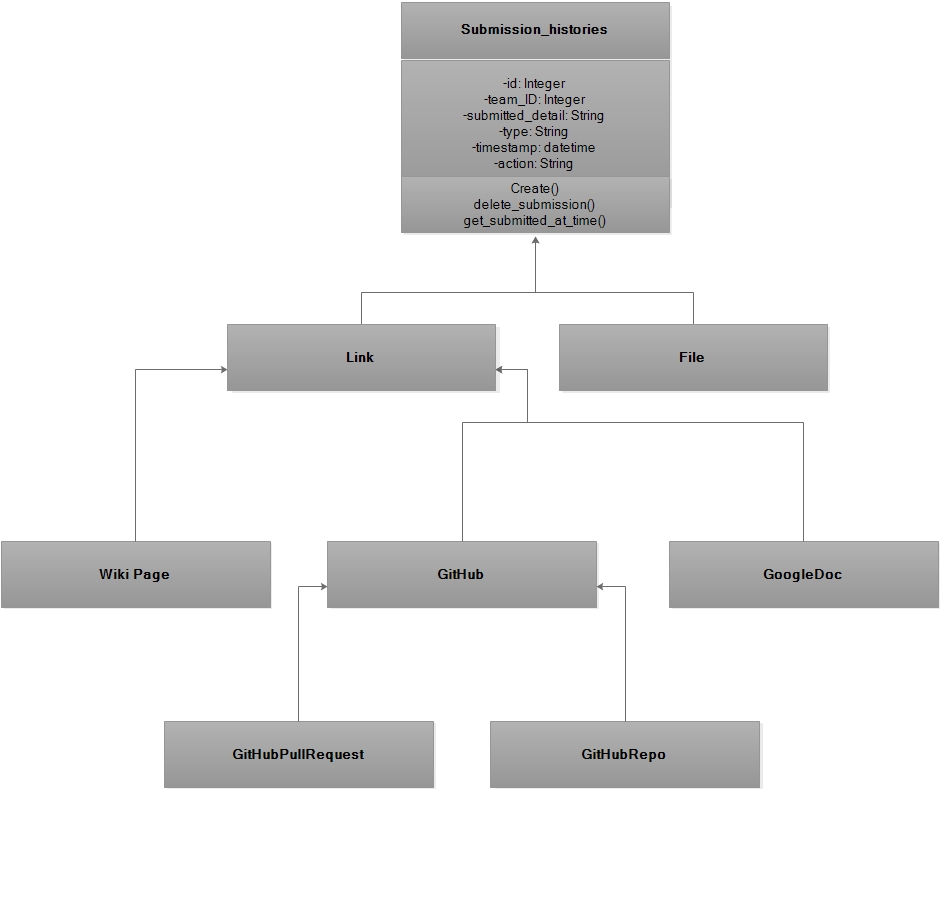
Files Added/Modified
Models
- assignment
Rufus scheduler is an in-memory scheduler for Ruby on Rails applications. It works on threads and not crons, hence it is lightweight and easy to use. However, because it is in-memory, a server shutdown will result in the loss of all scheduled jobs. We used it for its simplicity and because it mapped very well to our requirement. An even better scheduler would be resque scheduler, because it uses a Redis backend.
require 'rufus-scheduler' after_save :record_submission
+private + def record_submission + # whenever a new assignment is added, add a job on each of its deadlines, to update the + # timestamps of teams' submissions. + scheduler = Rufus::Scheduler.singleton + + self.due_dates.each do |due_date| + if due_date.deadline_type.name == 'submission' + scheduler.at(due_date.due_at.to_s, "assignment"=>self) do |job, time, arg| + LinkSubmissionHistory.add_submission(job.opts['assignment'].id) + end + end + end + end
- files_submission_history
+class FileSubmissionHistory < SubmissionHistory + def self.create(link, team, action) + history_obj = FileSubmissionHistory.new + history_obj.submitted_detail = link + history_obj.team = team + history_obj.action = action + return history_obj + end +end
- github_pull_request_submission_history
+class GithubPullRequestSubmissionHistory < GithubSubmissionHistory + def self.create(link, team, action) + history_obj = GithubPullRequestSubmissionHistory.new + history_obj.submitted_detail = link + history_obj.team = team + history_obj.action = action + return history_obj + end + + def get_submitted_at_time(link) + uri = URI(link) + link_path = uri.path + git_user_details = link_path.split("/") + github = Github.new + a = github.pulls.get git_user_details[1], git_user_details[2], git_user_details[4] + return a.updated_at + end +end
- github_repo_submission_history
+class GithubRepoSubmissionHistory < GithubSubmissionHistory + def self.create(link, team, action) + history_obj = GithubRepoSubmissionHistory.new + history_obj.submitted_detail = link + history_obj.team = team + history_obj.action = action + return history_obj + end + + def get_submitted_at_time(link) + uri = URI(link) + link_path = uri.path + git_user_details = link_path.split("/") + github = Github.new + a = github.repos.get git_user_details[1], git_user_details[2] + return a.pushed_at + end +end
- github_submission_history
+class GithubSubmissionHistory < LinkSubmissionHistory + def self.create(link, team, action) + if link.include? "pull" + history_obj = GithubPullRequestSubmissionHistory.create(link, team, action) + else + history_obj = GithubRepoSubmissionHistory.create(link, team, action) + return history_obj + end + end +end
- googledoc_submission_history
+class GoogledocSubmissionHistory < LinkSubmissionHistory + def self.create(link, team, action) + history_obj = GoogledocSubmissionHistory.new + history_obj.submitted_detail = link + history_obj.team = team + history_obj.action = action + return history_obj + end +end
- link_submission_history
+class LinkSubmissionHistory < SubmissionHistory + + def self.create(link, team, action) + # lets assume link of type http://docs.google.com or http://wiki.expertiza.ncsu.edu or http://github.com/goeltanmay/ + if link.include? "docs.google.com" + history_obj = GoogledocSubmissionHistory.create(link, team, action) + elsif link.include? "wiki.expertiza.ncsu.edu" + history_obj = WikipediaSubmissionHistory.create(link, team, action) + elsif link.include? "github.com" + history_obj = GithubSubmissionHistory.create(link, team, action) + else + # some random link has been given + history_obj = LinkSubmissionHistory.new + history_obj.team = team + history_obj.submitted_detail = link + history_obj.action = action + end + return history_obj + end + + def self.add_submission(assignment_id) + @assignment = Assignment.find_by_id(assignment_id) + teams = @assignment.teams + teams.each do |team| + submitted_links = team.hyperlinks + submitted_links.each do |link| + submission_history = SubmissionHistory.create(team, link) + timestamp = submission_history.get_submitted_at_time(link) + submission_history.submitted_at = timestamp + begin + submission_history.save + rescue + @error_message = "No new updates" + end + end + end + end +end
- submission_history
+class SubmissionHistory < ActiveRecord::Base + belongs_to :team + validates_uniqueness_of :submitted_detail, scope: [:team_id, :submitted_at] + + def self.create(team, link) + # for file, the link will be? - put this as default condition + # determine whether this is a file or a link + existing_submission = SubmissionHistory.where(["team_id = ? and submitted_detail = ?", team, link]) + if existing_submission.size>0 + action = "edit" + else + action = "add" + end + + if link.start_with?("http") + history_obj = LinkSubmissionHistory.create(link, team, action) + else + history_obj = FileSubmissionHistory.create(link, team, action) + end + return history_obj + end + + def self.delete_submission(team, link) + # just add a delete record + if link.start_with?("http") + history_obj = LinkSubmissionHistory.create(link, team, "delete") + else + history_obj = FileSubmissionHistory.create(link, team, "delete") + end + return history_obj + end + + def get_submitted_at_time(link) + return Time.current + end + +end
- wikipedia_submission_history
+class WikipediaSubmissionHistory < LinkSubmissionHistory + def self.create(link, team, action) + history_obj = WikipediaSubmissionHistory.new + history_obj.submitted_detail = link + history_obj.team = team + history_obj.action = action + return history_obj + end + + def get_submitted_at_time(link) + uri = URI(link) + wiki_title = uri.path + wiki_title.slice! "/index.php/" + url = URI.parse('http://wiki.expertiza.ncsu.edu/api.php?action=query&prop=info&titles='+ wiki_title +'&format=json') + req = Net::HTTP::Get.new(url.to_s) + res = Net::HTTP.start(url.host, url.port) {|http| + http.request(req) + } + hash= JSON.parse res.body + id = hash["query"]["pages"].keys + return hash["query"]["pages"][id[0]]["touched"] + end +end
Controllers
- submitted_content_controller
+ #create timeline for generalized map to just loop in the html + @timeline = Hash.new() + #Get timeline entries from submission_histories table + @submission_history = SubmissionHistory.where(team: @participant.team.id).order(:submitted_at) + #reviews + @review_maps = ResponseMap.where(reviewee_id: @participant.team.id) + @review_maps.each do |map| + if "ReviewResponseMap".eql?map.type + @response = Response.find_by(map_id: map.id) + @timeline[@response.updated_at]={:heading => map.type.chomp('ResponseMap') , :description => , :color => 'green'} + end + end + #feedbacks + @feedback_maps = ResponseMap.where(reviewer_id: @participant.id) + @feedback_maps.each do |map| + if "FeedbackResponseMap".eql?map.type + @response = Response.find_by(map_id: map.id) + @timeline[@response.updated_at]={:heading => map.type.chomp('ResponseMap') , :description => , :color => 'info'} + end + end + @submission_history.each do |submission| + @timeline[submission.submitted_at]={:heading => submission.type+' '+submission.action, :description => submission.submitted_detail} + end + @assignment.due_dates.each do |due_date| + @timeline[due_date.due_at]={:heading => ' Due Date ', :description => due_date.deadline_type.name+' deadline', :color => 'danger'} + end
Views
- submitted_content/_main.html.erb
+ <%if !@timeline.nil? %> + <%= render partial: 'submitted_content/submission_history', locals: {timeline: @timeline, origin: } %> + <%end%> +
- submitted_content/_submission_history.html.erb
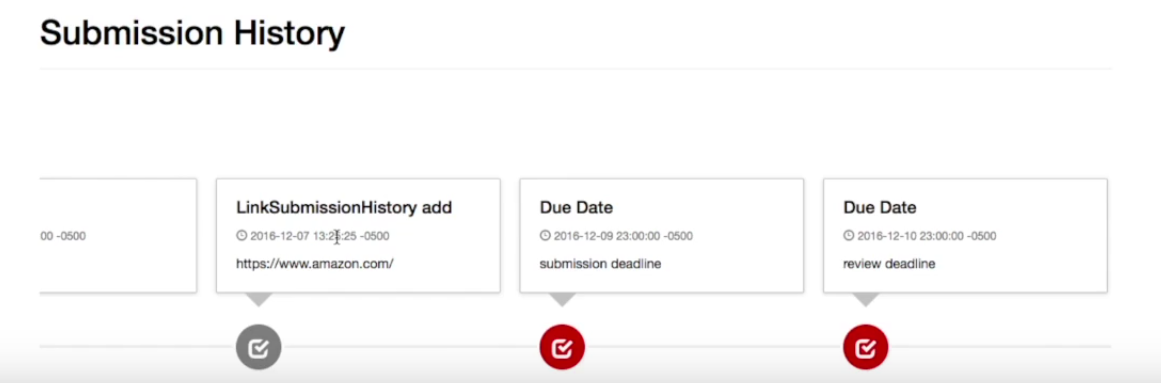
Testing
- Unit Testing of controllers
This involves unit testing of following controllers: TeamsController, because we will have to change some methods.
- Unit Testing of Models
This involves writing rspec test for all the newly created models like submission_history, google_doc_submission_history, etc. Tests for team model are already written.
- models/github_pull_request_submission_history_spec.rb
+require "rails_helper" + +describe GithubPullRequestSubmissionHistory do + describe "get_submitted_at_time" do + it "should return the last time a github pull request was updated" do + history_object = GithubPullRequestSubmissionHistory.new + time_stamp = history_object.get_submitted_at_time("https://github.com/prerit2803/expertiza/pull/1") + expect(time_stamp).not_to be_empty + end + end +end
- models/github_repo_submission_history_spec.rb
+require "rails_helper" + +describe GithubRepoSubmissionHistory do + describe "get_submitted_at_time" do + it "should return the last time a github repo was pushed" do + history_object = GithubRepoSubmissionHistory.new + time_stamp = history_object.get_submitted_at_time("https://github.com/prerit2803/expertiza/") + expect(time_stamp).not_to be_empty + end + end +end
- models/link_submission_history_spec.rb
+require 'rails_helper' + +describe LinkSubmissionHistory do + describe "add submission" do + it "should add GithubRepoSubmissionHistory" do + assignment = build(Assignment) + assignment.save + assignment_team = build(AssignmentTeam) + assignment_team.parent_id = assignment.id + assignment_team.submit_hyperlink("https://github.com/prerit2803/expertiza") + assignment_team.save + expect_any_instance_of(GithubRepoSubmissionHistory).to receive(:get_submitted_at_time) + LinkSubmissionHistory.add_submission(assignment.id) + end + + it "should add GithubPullRequestSubmissionHistory" do + assignment = build(Assignment) + assignment.save + assignment_team = build(AssignmentTeam) + assignment_team.parent_id = assignment.id + assignment_team.submit_hyperlink("https://github.com/prerit2803/expertiza/pull/1") + assignment_team.save + expect_any_instance_of(GithubPullRequestSubmissionHistory).to receive(:get_submitted_at_time) + LinkSubmissionHistory.add_submission(assignment.id) + end + + it "should add WikipediaSubmissionHistory" do + assignment = build(Assignment) + assignment.save + assignment_team = build(AssignmentTeam) + assignment_team.parent_id = assignment.id + assignment_team.submit_hyperlink("http://wiki.expertiza.ncsu.edu/index.php/CSC/ECE_517_Fall_2016/oss_E1663") + assignment_team.save + expect_any_instance_of(WikipediaSubmissionHistory).to receive(:get_submitted_at_time) + LinkSubmissionHistory.add_submission(assignment.id) + end + end +end
- models/submission_history_spec.rb
+require 'rails_helper' + +describe SubmissionHistory do + describe "create method" do + it "should create link submission history" do + assignment_team = build(AssignmentTeam) + link = "https://www.youtube.com/watch?v=dQw4w9WgXcQ&ab_channel=RickAstleyVEVO" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(LinkSubmissionHistory) + end + + it "should create github link submission history" do + assignment_team = build(AssignmentTeam) + link = "https://github.com/prerit2803/expertiza" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(GithubRepoSubmissionHistory) + end + + it "should create github link submission history" do + assignment_team = build(AssignmentTeam) + link = "https://github.com/prerit2803/expertiza/pull/1" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(GithubPullRequestSubmissionHistory) + end + + it "should create wikipedia link submission history" do + assignment_team = build(AssignmentTeam) + link = "http://wiki.expertiza.ncsu.edu/index.php/CSC/ECE_517_Fall_2016/oss_E1663" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(WikipediaSubmissionHistory) + end + + it "should create google doc link submission history" do + assignment_team = build(AssignmentTeam) + link = "https://docs.google.com/document/d/1fI5KbPXCJ8dSUyLynnqC__A3MtZ47enNfMcki3Vf3uk/edit" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(GoogledocSubmissionHistory) + end + + it "should create file submission history" do + assignment_team = build(AssignmentTeam) + link = "/user/home/Expertiza_gemfile" + submission_history = SubmissionHistory.create(assignment_team, link) + expect(submission_history).to be_a(FileSubmissionHistory) + end + end + + describe "delete method" do + it "should add a row to submission history table with action as delete" do + assignment_team = build(AssignmentTeam) + link = "/user/home/Expertiza_gemfile" + submission_history = SubmissionHistory.delete_submission(assignment_team, link) + expect(submission_history.action).to eq("delete") + end + + it "should add a link submission history with action as delete" do + assignment_team = build(AssignmentTeam) + link = "https://docs.google.com/document/d/1fI5KbPXCJ8dSUyLynnqC__A3MtZ47enNfMcki3Vf3uk/edit" + submission_history = SubmissionHistory.delete_submission(assignment_team, link) + expect(submission_history.action).to eq("delete") + end + + it "should add a row to submission history table with action as edit" do + assignment_team = build(AssignmentTeam) + link = "/user/home/Expertiza_gemfile" + submission_history = SubmissionHistory.create(assignment_team, link) + submission_history.submitted_at = Time.current + submission_history.save + + submission_history2 = SubmissionHistory.create(assignment_team, link) + submission_history2.submitted_at = Time.current + submission_history2.save + expect(submission_history2.action).to eq("edit") + end + end +end
- models/wikipedia_submission_history_spec.rb
+require "rails_helper" + +describe WikipediaSubmissionHistory do + describe "get_submitted_at_time" do + it "should return the last time a wiki page was updated" do + history_object = WikipediaSubmissionHistory.new + time_stamp = history_object.get_submitted_at_time("http://wiki.expertiza.ncsu.edu/index.php/CSC/ECE_517_Fall_2016/oss_E1663") + expect(time_stamp).not_to be_empty + end + end +end
- UI Testing
- Login as student/instructor.
- Go to Assignments Home Page.
- Here you will find a timeline with several tags like submission, review, resubmission, rereview.
- Also, There will be a tags for the authors submitting and updating their artifacts, reviewers submitting peer-reviews, reviewees sending author feedback and reviewers updating their peer reviews.
- As UI would be modified a lot, more testing part would be added after the implementation.