CSC/ECE 517 Fall 2010/ch7 7b vk
Information Expert Pattern is one of the GRASP patterns which states the following:
Assign a responsibility to the information expert: the class that has the information necessary to fulfill the responsibility.[1]
Object Oriented Design
Object oriented design is a process of building a system of interacting objects to solve a given software problem. It can be otherwise defined as, identifying requirements and creating a domain model, then adding methods to the software classes, and defining the messaging between the objects to fulfill the requirements. But, deciding what methods belong where, and how the objects should interact, is critically important in the designing process and is something non-trivial.
Design Patterns
Experienced Object-oriented developers build up a repertoire of both general principles and idiomatic solutions that guide them during Object oriented design phase of the software. The principles and idioms, if codified in a structured format describing the problem and solution, and given a name, may be called as Design patterns.
The benefits of design patterns include [2] :
- Code is more accessible and understandable.
- Use of common vocabulary to help collaboration among developers.
- Helps people understand the system more quickly.
- Easier to modify system.
GRASP
General Responsibility Assignment Software Patterns (or Principles), popularly known as GRASP, are a set of guidelines for assigning responsibility to software classes and objects in object oriented design. Information Expert Pattern is one of the very basic five GRASP Patterns: Information Expert, Creator, High Cohesion, Low Coupling and Controller. The Information Expert pattern is a solution to the problem of determining which object should assume a particular responsibility. During design, we make choices about the assignment of responsibilities to classes. Information Expert helps us decide, once we know the task (responsibility), which class to make responsible for carrying out the task.
Our focus in this article will be Information Expert Pattern. Information Expert Pattern is also popularly known as Expert Pattern.
Responsibilities and Methods
Separation of Responsibilities among various classes is a crucial task in the process of object-oriented design. Responsibilities of each object defines its behavior in the system. Hence, translating Responsibilities into methods of an object is quite a challenging task.
The OMG in its UML standard specification defines a responsibility as "a contract or obligation of a classifier". These responsibilities are of two types. [3]
- doing
- knowing
Doing responsibilities of an object include:[4]
- doing something itself, like constructing an object or doing a calculation
- initiating action in other objects by passing messages
- controlling and coordinating activities in other objects
Knowing responsibilities of an object include:
- knowing about private encapsulated data
- knowing about related objects
- knowing about things it can derive or calculate
The challenge here is to translate these responsibilities into methods and assigning them to an appropriate class such that the design has low coupling and high cohesion. Information Expert Pattern helps the designers in this aspect.
Information Expert Pattern
Information Expert pattern can be simply defined as follows: [5]
Pattern Name : Information Expert Pattern
Problem : What is the basic principle by which we can assign responsibilities to objects?
Solution : Assign a responsibility to the class which has the information needed to fulfill it.
The Shopping Cart Example
Let us consider a simple Shopping Cart example, to demonstrate what exactly Information Expert Pattern guides us.
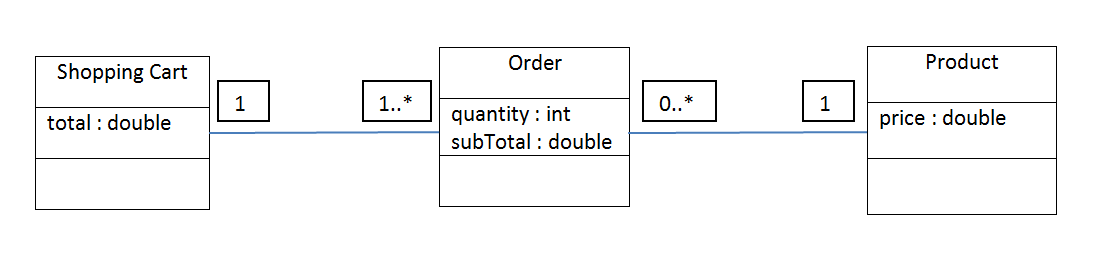
A Shopping Cart can hold one or more Orders, and an Order refers just to a single product. The simple business rules regarding the entities, Shopping Cart, Order and Product are as follows:
1. The Shopping Cart total is sum of all Order subTotals.
2. Order subTotal is calculated by multiplying the price of the Product with the ordered quantity.
Now, given the simple business rules, lets design the classes.
public Class Product{ private String productName; private double price; public double getPrice(){ return price; } public void setPrice(double price){ this.price = price; } }
public Class Order{ private Product product; private int quantity; public product getProduct(){ return this.product; } public void setProduct(Product p) { this.product = p; } public int getQuantity() { return this.quantity; } public void setQuantity(int q) { this.quantity = q; } }
public class ShoppingCart { private List orders = new ArrayList(); public void addOrder(Order o) { this.orders.add(o); } public List getOrders() { return this.orders; } }
As we can see, each of the classes have right properties and dependencies:
1. A Product has an attribute 'price'.
2. An Order is composed of a Product and has a product 'quantity'.
3. The Shopping Cart has one or more orders.
4. The Shopping Cart total is calculated by computing all 'Order's subTotal and adding them up.In our implementation these orders are stored in an ArrayList, which can be traversed and each subTotal for the corresponding Order can be added to compute the final 'total'.
5. Order subTotal is calculated by multiplying the price of each Product with the product quantity.
In the above example, we only have various get and set methods but, we actually did not assign any responsibilities to any of the classes.
Now, we will seek the help of Information Expert Pattern to assign responsibilities to most appropriate classes.
Refactoring using Information Expert Pattern
We have two responsibilities to be assigned, which are:
1. The 'total' for the shopping cart has to be computed : we see that class ShoppingCart has the whole list of orders and hence as per Information Expert Pattern, the information expert is the class ShoppingCart.
2. The 'subtotal' for each order has to be computed: we see that class Order has reference to the product and its quantity and hence according to Information Expert Pattern, the information expert is the class Order.
public class Order { private Product product; private int quantity; public void setProductAndQuantity(Product p, int q) { this.product = p; this.quantity = q; } public double calculateSubTotal() { return this.product.getPrice() * this.quantity; } }
public class ShoppingCart { private List orders = new ArrayList(); public void addOrder(Order o) { this.orders.add(o); } public double calculateTotal() { double total = 0; Iterator it = this.orders.iterator(); while (it.hasNext()) { Order current = (Order) it.next(); total += current.calculateSubTotal(); } return total; } }
By refactoring using Information Expert Pattern, we'll enhance the encapsulation and hence the design will be more robust, as we can place constraints pertaining to any of these responsibilities very easily in their respective Information Expert classes.
The above example captures the essence of Information Expert Pattern. We should observe that though, we assigned the responsibility to the Information Expert, which has the required information to fulfill it, the task is often accomplished by collaborating with several other classes. This implies there are many 'partial' information experts collaborating in fulfilling the assigned responsibility.
In our example, since the class ShoppingCart has the list of orders, Expert Pattern suggests us to assign the responsibility of calculating the total to it. But, this task needs collaboration of three classes of objects as illustrated by the following figure.
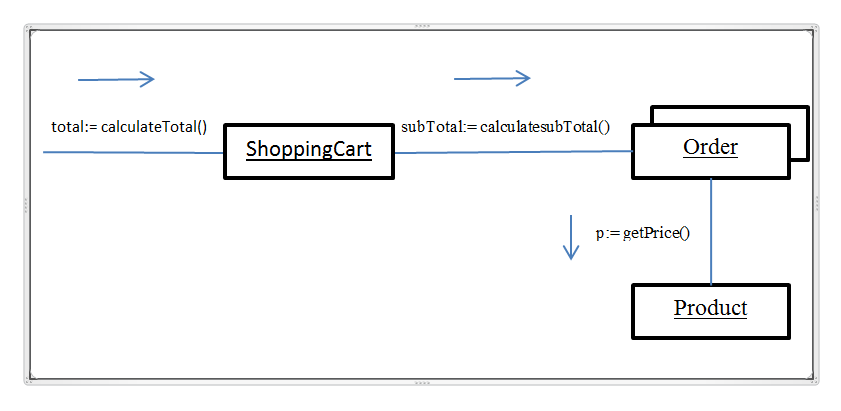
Real World Analogy
Like many concepts in Object technology, Information Expert Pattern has a real world analogy.
Generally, we assign a responsibility to an individual who has necessary information to fulfill the given task.
For example, in a business, who should be responsible for finding out the net worth of the company? The person who has all the necessary information to create it - perhaps the chief financial officer. The company's chief financial officer may ask his sub-ordinates or accountants to generate reports on assets and liabilities. As all the information is generally spread out across many individuals, it is essential to collaborate to accomplish a given task.
Similarly, in case of software objects, though Information Expert is primarily responsible for an assigned responsibility, very often it needs to collaborate with several other classes to fulfill a given task.
Benefits
- Expert Pattern improves the Information Encapsulation, since objects use their own information to fulfill a task. This supports Low coupling, as objects do not have to rely on external objects to fulfill a given task, and hence leads to more robust and maintainable systems.
- Behavior is distributed across several classes, which are 'partial' information experts, which leads to "lightweight" cohesive class definitions that are easier to understand and maintain. High cohesion is usually supported.
Contraindications
There are situations where solutions suggested by Expert pattern are undesirable, usually due to coupling and cohesion issues.
For example, who should be responsible to save 'ShoppingCart' in the database? As per Expert suggestion, we can argue that the class 'ShoppingCart' should take the responsibility to save the 'ShoppingCart' object, as all the information needed is present in the 'ShoppingCart' object.
This suggests that, each class should have it own services to save itself in the database. But, this leads to problems in coupling, cohesion and duplication. The class should now contain logic related to database handling, such as JDBC and SQL or MySQL etc., But, the class no longer reflects the actual intended abstraction "ShoppingCart". It rather than being coupled only with domain objects, now it is also coupled with the database subsystems, which lowers its cohesion.
This problem indicates the violation of basic architectural principles: design for separation of major system concerns. The application logic and database logic should be different system concerns and hence should be at different places. Even though Expert suggests that the database logic should be handled by each class, it is a poor design.
See also
More GRASP Patterns
Separation of Responsibility
References
[1] [CS4233 Lecture 7 Mindjet Mindmanager 2007] http://web.cs.wpi.edu/~gpollice/Maps/CS4233/Week34/CS4233%20Lecture%207/index.html
[2] [Dale Skrien. Object-Oriented Design Using Java. pp. 196-197 2005 ]
[3] [OMG 2001] http://www.omg.org/spec/UML/2.1.2/
[4] [GRASP: Designing Objects with Responsibilities Harshjagadeesan 2009] http://www.slideshare.net/harshjegadeesan/lecture-responsibility-driven-design-presentation
[5] [Hongjun Su 2006]
http://cs.armstrong.edu/hsu/Course/CSCI3321/PracticalSoftwareEngineering/html/ch10s04.html