CSC/ECE 517 Fall 2010/ch4 4d PR
Namespaces is a set of names which are bound together to form unique identifier in order to resolve conflict with same names from different context. Namespaces give you control over the visibility of the properties and methods that you create in a program.
Overview
A namespace is a way to keep names organized. Lets use an analogy to file system in the computer which uses directories and sub-directories. Namespaces in this context could be viewed as the path to a file in the directory/sub-directory which can uniquely identify each file. User can create more than one files with same name in different directories or sub-directories as they are in different namespaces.[1] Another advantage of namespace is logical grouping of source code.
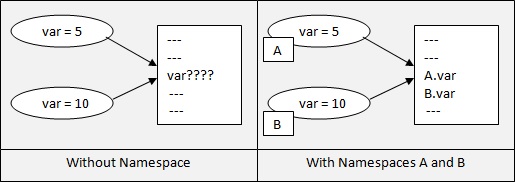
Above figure depicts the use of namespace in programming language. The left part of the diagram shows variable "var" used by two different contexts. Now, the context which wants to use the variable "var" should have some means of distinguishing between its declarations. This is where namespaces comes into account. As shown in the second part of the diagram each variable "var" can now be identified independently.
Types of Namespaces
Different languages support namespace either using implicit types or explicitly by using keyword 'namespace'.[2]
Implicit Namespaces
Implicit Namespaces are the abstraction defined by language itself in order to resolve name conflicts. Some of the constructs of implicit namespaces in different languages are interfaces, packages, scope, attributes,closure[3] etc.
Blocks
Blocks are often used as an abstraction to resolve naming conflicts. In many languages blocks determine scope of the variables. Hence a variable declared in a particular block has its scope within the block. Hence, we can think of a block as a namespace.[4]
Below is the example that shows how a block resolves naming ambiguities.
main() { int i=5; ------ ------ { int i=10; ------ ------ //printing I here gives value equal to 10 } //printing I here gives value equal to 5 }
The above program depicts the concept of global and local variable initialization which can be viewed as a example of implicit namespace handled by programming language constructs. Disadvantage of this type of namespace is that programmer has to keep track of all variables in program and their namespaces.
Objects
In JavaScript, a namespace is actually an object which is used to reference methods, properties and other objects.Objects are all we need to create namespaces in Javascript.
Below is an example of a modules in Javascript:
var Val = { Hello: function() { alert("Hello...!!!"); }, Goodbye: function() { alert("Goodbye...!!"); } } // In a nearby piece of code... Val.Hello(); Val.Goodbye();
The above example shows the use of object to reference the methods in its namespace. Hence the functions above are called using the object.[5]
Packages
Packages can be used as a mechanism to organize classes into namespaces which are mapped to file system. A unique namespace is provided by each package which gives flexibility to define same identifier name over different packages.
Below is an example of a package in Java:
package A; class A1 { int var = 4; } package B; class B1 { int var = 12; }
The above code snippet shows how a package can behave as a namespace in some programming language. Here, package A and package B are two different namespaces with variable var in each of them.[6]
Modules
In some languages, different constructs are used to identify namespaces. Languages like Ruby maintains namespace at higher level of abstraction known as modules which can be thought to be similar to packages in Java.
Below is an example of a modules in Ruby programming language:
module Trig PI = 3.141592654 def Trig.sin(x) # .. end def Trig.cos(x) # .. end end module Moral VERY_BAD = 0 BAD = 1 def Moral.sin(badness) # ... end end require 'trig' require 'moral' y = Trig.sin(Trig::PI/4) wrongdoing = Moral.sin(Moral::VERY_BAD)
The above code snippet shows the use of modules in a form of namespace in Ruby language.The methods are referenced using the name of the module as shown in the code. Hence there is no clash on method name 'sin'.[7]
Attributes
XML namespaces provide method for qualifying element and attribute names by associating them with namespaces identified by unique Uniform Resource Identifier URI references. For example, Id could be in both vocabularies, employee and student, hence we can provide uniqueness for each vocabulary using namespaces to avoid conflicts.
A namespace is declared using the reserved XML attribute xmlns, the value of which must be an Internationalized Resource Identifier (IRI), usually a Uniform Resource Identifier (URI) reference.
Below is an example of a attributes as a namespace in XML:
<root xmlns:fruits="http://www.w3schools.com/fruits" xmlns:furniture="http://www.w3schools.com/furniture"> <fruits:table> <fruits:tr> <fruits:td>Apples</fruits:td> <fruits:td>Bananas</fruits:td> </fruits:tr> </fruits:table> <furniture:table> <furniture:name>Sofa</f:name> </furniture:table> </root>
The above XML creates the namespace "fruits" and "furniture" in the attribute of xmlns in XML. This way we define namespaces in XML.[8]
Explicit Namespaces
Namespaces can be explicitly manipulated and composed, making it quite a simple matter to combine, rename and compose packages or modules. Hence some languages use keyword "namespaces" for declaring namespaces. For example, C, CPP, PHP, C#. Below is the CPP code which depicts use of namespace keyword.
namespace Square{ int length = 4; } namespace Rectangle{ int length = 12; int breath = 8; } int main () { cout << Square::length << endl; //output 4 cout << Rectangle::length << endl; //output 12 return 0; }
The above code snippet tells us the use of explicit namespace by using the keyword "namespace" in C++. It defines length in two different namespaces using the keyword namespace. When the length is referenced it is referenced using the name of the namespace.[9]
Similarly, PHP uses the namespace keyword to distinguish between identifiers.Below is an example of PHP using namespace keyword:
<?php Namespace A; Const NAME = ‘this is constant’ Function FUN() { ----- ----- return _MYVARIABLE_; } Class C { function print_this() { ------ ------ return _THIS_METHOD_; } } ?>
The above example denotes the use of namespace keyword in PHP language. Hence in the code const NAME,function fun() and class C are defined in Namespace A. Therefore, they are referenced using the namespace A.[10]
Similarly, C# also uses namespace keyword to distinguish names. Below is the code of C# showing use of namespace keyword.
namespace A { public class A1 { static void Main() { B.B1.welcome(); } } } namespace B { public class B1 { public static void welcome() { Console.WriteLine("Welcome"); } } }
The above code snippet shows the use of namespace keyword in C# language. Here, function main in namespace A calls to function 'welcome' from namespace B by referring its namespace_name.class_name.function_name.[11]
Conclusion
Every programming language support namespaces at different abstraction level. They are not just containers but designed to allow simple,fast and efficient usage and understanding of the code. Namespaces actually provide luxury to declare multiple variable with same name which in turn protect code with name clashes. Using namespace generally gives a better structure to program which makes easier to maintain when the code length increases. In summary, different languages have different methods of providing namespace functionality be it explicit or implicit to provide better coding experience to the user.
References
References
- NameSpace
- Explicit Namespaces
- Closures
- Code blocks, execution frames, and namespaces
- Namespaces in Javascript
- Namespace vs. Packages
- Ruby Modules and Mixins
- XML Namespace
- Namespace in C++
- PHP Manual by Mehdi Achour, Friedhelm Betz, Antony Dovgal, Nuno Lopes, Hannes Magnusson, Georg Richter, Damien Seguy, Jakub Vrana
- Namespaces in C#