CSC/ECE 517 Fall 2010/ch3 3d AI
Introduction
Aspect Oriented Programming 1 is a programming style which isolates the business logic of the program from its secondary or supporting functions. The basis of the aspect oriented programming is to add a third dimension to pre-existing dimensions of the Procedural Programming and Object Oriented Programming, by describing aspects that cross-cut the structure of the program.
Overview
If we look at the programming field from a distance we’ll generally divide programming languages into two main camps: procedural programming(POP) and object-oriented programming (OOP). Like all programming techniques these were invented as ways to structure programming to achieve conceptual separation of components. This separation is obtained by dividing programs into sub-structures of some kind so that we can think and program each one as a reasonably separate and autonomous entity.
When using POP we divide our program into separate functions or procedures and compose the program flow by redirecting it from one to another in what is essentially a linear structure. With OOP a second dimension is added as we structure our code into encapsulated units linked to each other. The control flow is now defined dynamically by the object structure and polymorphism. Aspect Oriented programming tries to add a third dimension to this structure by describing so called aspects that cross-cut the structure of the program.
Motivation
Traditional programming designs had poor modularity due to crosscutting dependencies. Especially in OOP each aspect is scattered by virtue of the function being spread over numerous unrelated functions or modules which maybe using its functionality, this means changing logging in one function requires modifying all the modules using this functionality, this works on the logic that the users knows/maintains a log of all the functions that use the functionality of the aspect Example.
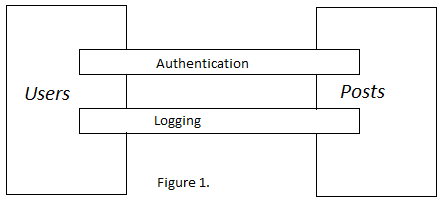
Let us consider the example all know and love, Twitter. The model for which is shown in figure 1. If this model were to be implemented in an Object Oriented manner then the security aspect like authentication would have been scattered by virtue of a function called a logging, which is used by both the Users and Posts, a database transaction should encapsulate the operation in order to prevent accidental data loss Also, this operation should be logged onto the system log. We already see that authentication together with logging and transactions are now exhibiting cross-cutting concerns. Now consider the scenario in which the security specifications change , then in order to complete these changes this would require a major effort as the security-related operations are scattered across numerous methods.
Aspect Oriented Programming
Aspect Oriented Programming aims to solve this problem by including the concept called as aspects 3. Aspects are stand-alone modules used to express cross-cutting concerns 4. Figure demonstrates how our twitter module now looks with the inclusion of aspects. In Java, the aspects contain advice and intertype declarations 9.
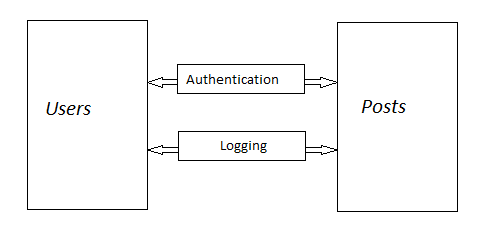
In our example our authentication module can include a security check for the users before he/she can access the posts posted by himself and his/her friends.
Key Concepts
Join Points: A well defined location at a point in the execution of a program.
PointCut: A set of join points
Advice: Code designed to run automatically at all join points in a particular pointcut. It can be marked as before, after or around i.e. in place of the join points in the pointcut.
Lexical Introduction: Adding functionality to a class in place as opposed to extending it.
AspectR
Aspect Oriented Programming in Ruby is known as AspectR. 2 Aspects such login, security login, database session, transactions need to be done at the start of each method. If for example an application starts a session at the beginning of the application start and releases the resource only at the termination. A lot of resources are wasted and also may cause starvation of other applications. Hence we need frequent access to resources every time a needy method is called. Implementing this logic inside the method makes the aspect code repeated and cluttered all over the application source. To avoid this problem Aspect oriented programming can be adopted into the Ruby applications6. Where all the aspect oriented functionality can be placed inside one behaviour. This behaviour can then be called before and after each method invocation. Thus making changes in aspect behaviour easy 7. Aspect oriented programming in Ruby can be implemented using Aquarium framework.
Creating a Simple Aspect in Ruby
Let us understand how easily an aspect behavior can be setup.
Setup
Install Aquarium gem from rubyForge website or alternatively use "gem install aquarium".
Example
Depending on the requirement of the application the aspect behavior may be changed from the one below. The snippet below shows the skeleton of a typical Aspect definition 8.
class Aspect_Name include Aquarium::DSL before :calls_to => :all_methods, :in_types => /Service$/ do |join_point, object, *args| #Do Aspect Work Like Creating Session, loggin, transaction, security etc end after :calls_to => :all_methods, :in_types => /Service$/ do |join_point, object, *args| #Do Aspect Work Like Ending Session, loggin, transaction, security etc end end
Before advice tells what work needs to be done before the method is called.
After advice tells what work needs to be done after the method ends.
Another way of implementing is using Around Advice.
class Aspect_Name include Aquarium::DSL around :calls_to => :all_methods, :in_types => /Service$/ do |join_point, object, *args| #Do Aspect Work Like Creating Session, loggin, transaction, security etc result = join_point.proceed #Do Aspect Work Like Ending Session, loggin, transaction, security etc result # block returns the result of the proceed. end end
Aspect Oriented programming vs Object Oriented Programming
Aspect oriented programming is not a complement to Object Oriented programming. It coexists together 3. AOP tries to lessen the limitations of traditional object oriented programming like cross-cutting in code, dependency in code change in repetitive methods which deal with aspects like logging, authentication and sessions.
Summary
Aspect oriented Programming is a programming technique which has added a third dimension to programming languages, which is a significant improvement over Object Oriented Programming as it lets the programmer focus on working on the business logic in times when he has to change his line of thinking mid-way. All this without having to to be worried about his aspect being scattered through the code as all his new code can be incorporated in aspects.
References
- [6]: AspectR sourceforge
- [7]: Aspect the Ruby way
- [8]: Ruby Forge