CSC/ECE 517 Fall 2010/ch3 3b sv
Multiple Inheritance and Mixins
The concept of Inheritance and its working is explained in most of the Object Oriented Programming (OOP) overviews. But a very few of these OOP overviews discuss alternatives when Inheritance fails to be the best design choice for an OO application. The main focus of this topic is to introduce the case where Inheritance proves to be a bad design option and discuss the concepts which are practiced to overcome it. The discussion starts with brief description of what Inheritance is and how it works. The classic scenario of the deadly Diamond problem is mentioned to discuss why exactly inheritance is criticized. The potential alternatives to solve this problem are introduced. We look at the merits and demerits of these alternatives. This topic discusses Mixins more closely.
[This chapter adds to the coverage of chapter 2f (Fall 2010) and related topics from Fall 2007 which can be found at the mediawiki website [[1]]]
Inheritance in OOP Design
Inheritance is a concept of OOP that facilitates reuse and compartmentalization of code by creating objects (which are collections of attributes and behaviors) that can be derived from existing objects. The deriving object (class) is termed as the child class/sub-class/derived class, and, the object (class) whose methods are being used is called the parent class/super-class/base class. This relationship between the super and sub-classes arising due to inheritance creates a hierarchy amongst the classes. Inheritance is termed as Single Inheritance if there is only one base class. Languages like C++,Java,Ruby,Python and SmallTalk support inheritance.
Single inheritance creates a Is-A relationship amongst the classes. Consider the concept of single inheritance using class extension feature:
class Animal{ //... } class Reptiles extends Animal{ //... }
In the above example, the Reptiles class is a specialization of Animals class and derives some/all of its attributes and/or methods from the Animal class.
The next section briefly discusses the alternatives of Inheritance used in various OO languages.
Definitions
Multiple Inheritance
If a child class inherits behaviors and attributes from two or more parent classes, it is termed as Multiple Inheritance. Languages supporting this feature include C++, Perl and Python. The main advantage of Multiple Inheritance is that it enables an user to combine independent (and not so independent) concepts (classes) into a composite concept called the derived class. For instance, given a set of interaction base classes and a set of base classes defining display options, the user can create a new window by selecting a style of window interaction and appearance from the given base classes.
Suppose there are N concepts, each of which can be combined with one of M other concepts, N+M classes are required to represent all the combined concepts. The situation with N = M = 1 is Single Inheritance. Examples with N>=2 and M>=2 are common and Multiple Inheritance plays an important role in such situations by avoiding replication.
The following figure depicts the use of multiple inheritance:
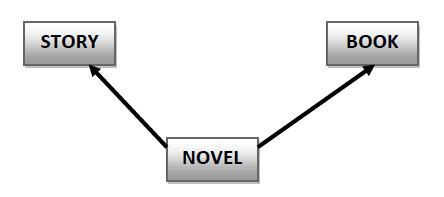
A novel has a certain number of chapters, characters and other properties that can be thought as features of class STORY. It also has features of class BOOK like publisher, a price, a weight and an ISBN number. Thus, Class NOVEL should inherit from both.
Following is the code snippet showing the above mentioned example:
class STORY{ //... } class BOOK{ //... } class NOVEL : public STORY, public BOOK{ //... }
Interfaces
Java designers cut the features of multiple inheritance and operator overloading from C++ to keep the language simple, object oriented and familiar. Interfaces were introduced in Java to simulate multiple inheritance. Interfaces (defined using the keyword interface) are abstract type containing only the method signatures and constant declarations. A class which implements an interface does so by implementing all its methods. Thus, interfaces act as templates.
Here's the example showing how interfaces are used in Java:
interface Bird { public void fly(); } public class Eagle implements Bird { public void fly() { System.out.println(“Eagle flies”); } public static void main(String args[]) { Bird bird = new Eagle(); bird.fly(); } } public class Duck implements Bird { public void fly() { System.out.println(“I cannot fly!!”); } public static void main(String args[]) { Bird bird = new Duck(); bird.fly(); } }
In the above example, the interface Bird has a method fly(). The Eagle class and the Duck class implement this method in different ways. Since each class making use of the interface has to implement all the methods of the interface, the methods in the interface can be implemented in different ways by different classes.
Modules
A module is defined as a component which regroups similar things. Modules differ from classes as modules are just names to regroup things which we think are similar, whereas, classes consist of variables and methods describing the state and the behavior of an entity [1]. A module "protects" the names it contains. The primary benefits of using modules are:
- The code is more organized.
- The risk of name clashes is eliminated as modules provide namespace facility.
- Mixins can be implemented using modules.
Unlike classes, objects can neither be created nor sub-classed based on modules. Instead, the functionality of a particular module can be specified to be added to a particular class or object.
Following is an example code snippet showing the use of Modules in Ruby: [7]
module takes_courses (parameter) def takes puts "student #{parameter} takes course csc517" end end class students include takes_courses -----> makes the module methods accessible def student_name( param ) puts "student_name = #{param}" end end student = students.new #In class student.student_name 'john' #student name = John # In Module student.takes #student John takes course csc517
In the above example, a module called takes_courses is defined. This module contains a method called takes which prints the name of the student who has taken the course csc517. The class called students includes the module takes_courses. A new student object is created. This object calls a method (student_name) defined in the class. The output would be "student_name = John ". The same student object is used to call the method takes in the module. This outputs "student John takes course csc 517".
Mixin
Mixins are one of the defining features of Ruby that are closely related to Duck typing. Duck typing is a technique that allows usage of objects to respond to a certain set of method calls interchangeably. Mixins are a group of methods that are not yet attached to any class. They exist in a module, and are useful only when they are included in a class. Mixin thus behaves as an interface with implemented methods.
Mixins cannot be instantiated, but, provide functionality to be inherited by a subclass. Ruby supports only single inheritance. So, mixins prove to be of great importance in Ruby as they simulate multiple inheritance capability in Ruby which allows classes to inherit functionalities from two or more mixins [2],[3],[4],[6].
An Enumeration is a type of mixin module which can be used by all the classes. It does not depend on the language syntax. It is defined as “ an operation that is performed on elements of a set”.
Example of enumeration is: [34, 56, 76, 96].each {|a| puts a} Here, Each element in the array is printed.
Following is an example showing the use of mixins: [8]
module Draw def draw_shape(param) puts "draw #{param}" end end class ParentClass def shape puts "shape is circle" end end class Circle < ParentClass include Draw end f = Circle.new f.shape f.draw_shape "circle"
In the above example, a subclass circle inherits the methods defined in the parent class.The class circle also includes the drawModule. It can access the methods in the module.A new circle object is created which can access the methods in parent_class as well as the methods defined in the module. The output would be i)shape is a circle ii) draw circle
Traits
A trait can be considered as a collection of methods which serves as a simple model for structured object oriented programs [5]. The main difference between mixins and traits lies in the way both are composed. Mixins are composed using only the inheritance operation, but, traits can include symmetric sum, aliasing and method exclusion. Trait is not an abstract type and implements methods.
With Traits, classes are organized in a single inheritance hierarchy. Traits are basically used to specify incremental differences in the behavior of sub- and super-classes. Traits are supported in programming languages like Fortress,Perl,SmallTalk and Joose framework for JavaScript.
Traits can be considered as a combination of interfaces that are abstract and their implementation. Here's a Scala trait representing objects that can be compared and ordered:
trait Ord { def < (that: Any): Boolean def <=(that: Any): Boolean = (this < that) || (this == that) def > (that: Any): Boolean = !(this <= that) def >=(that: Any): Boolean = !(this < that) } class Date extends Ord { def < (that: Any): Boolean = /* implementation */ } // can now write myDate >= yourDate
As we can see in the above example, Date only needs to implement <. It gets the other operators for free.[9]
Diamond Problem : Loophole in Multiple Inheritance
With single inheritance, classes cannot be classified into the branches of the hierarchy and hence, is limiting. Multiple inheritance overcomes this and supports complex relationship models.
But multiple inheritance has the following problems:
- It is possible that a class inherits multiple features with the same name.
- There can be more than one path to a given ancestor from a class (known as the diamond problem or fork-join inheritance)
- Encapsulation of inheritance: Does a client or inheritor of a class depend on the inheritance structure of that class?
- Common ancestor duplication problem: Should common ancestor be duplicated or not?
- Duplicate parent operation invocation problem: How is this problem of Multiple inheriting from two classes that both invoke the same parent operation on a common ancestor can cause duplicate invocation of this parent operation dealt with?
The conflicting-features problem can be resolved by permitting renaming or by having a linear class hierarchy. The diamond problem is an ambiguity arising when two classes B and C inherit from class A, and both B and C are inherited by class D. If both classes B and C override the definition of a method inherited from A, then, when class D inherits this same method, conflict arises if D should use the method from B or C.
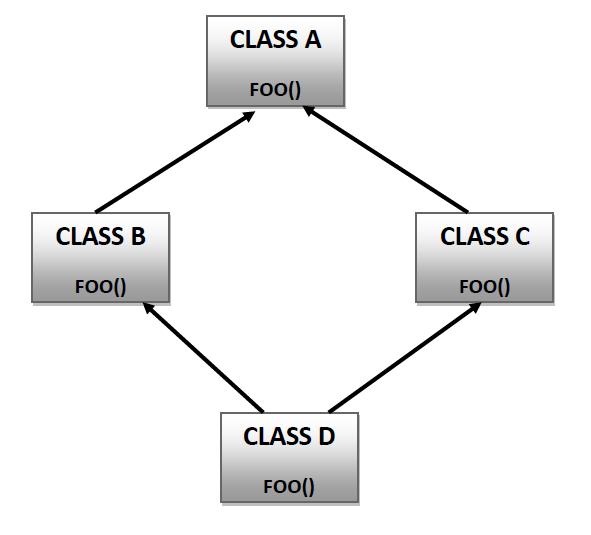
In C++, Virtual inheritance was proposed as one solution for class D to inherit only one copy of A's methods. But object initialization is a problem when we have only one copy. Another consequence of diamond problem is that multiple inheritance interacts poorly with modular type-checking of multiple dispatch.
Java makes use of the concept of interfaces to simulate Multiple Inheritance and the responsibility of implementing the functions defined in the interfaces lies with the classes making use of the interface. Similarly Ruby uses the concept of mixins and SmallTalk and Perl use Traits. But each solution has its own pros and cons.
How mixins solve the Diamond Problem
Ruby supports only single inheritance, i.e; a given class can inherit from only one parent class. But it is allowed to mixin many modules into a class. Moreover, modules are not permitted to inherit from other modules or classes. As these two conditions have to be always followed, there will be no "diamond" inheritance situation as every class is guaranteed to have a single parent class in case of inheritance.
As mentioned above, modules can mixin modules. So the following situation (hierarchy) may arise:
module P module Q module R class X
This hierarchy resembles Diamond Problem. But Ruby's method look-up flow [2] guarantees that mixin modules are searched before the parent class. Thus, the order of mixing in is significant in Ruby which guarantees that the module/class to be searched is unambiguous. Hence, the diamond problem is avoided.
Though mixins avoid diamond problem, they have a few disadvantages too. The following section does a comparison between the concepts, namely, Multiple Inheritance, Interfaces and Mixins, mentioned above.
Comparison
Mixins and Interfaces
FEATURE | MIXINS | JAVA INTERFACES |
---|---|---|
Maintainability | In Ruby, modules have the implementation of the methods. So all the classes who “include” the module get the same method and its functionality. The definition of the method can change at run time. | Java forces the classes using the interfaces to implement the methods defined in the interface. This might lead to code duplication if two classes have similar functionality. Secondly, changes have to be made at both places if the method changes in future. |
Size of the Code | The class/objects automatically get access to the methods of the included module(s). | If a class inherits multiple interfaces, it is forced to implement unnecessary, and sometimes, duplicate code which makes the code grow in size. |
Readability | The module and the class using the module can be in different files. Hence, the code is less readable. So mixins are beneficial for individual or small teams. For bigger teams, documentation becomes an important criterion. | The code is more readable and explicit as the implementation of a method can be found at a place. |
Naming Conflicts | Modules provide the facility of namespace. So it is possible for a class to inherit two or more methods of same name from different modules. | Since the class itself has to provide the implementation of the methods, inheriting methods of same names from different interfaces will signal an error message. |
Mixins and C++ Multiple Inheritance
ADVANTAGES | DISADVANTAGES | |
---|---|---|
MIXINS | 1. Avoids complex features like virtual base classes. 2. Code is less verbose. 3. Modules can be made aware of who included them. |
1. In case of naming conflicts while mixing multiple modules, notification of the error may be incorrect. 2. Ruby is used for implementation inheritance and finds very less use in inheritance implementation. 3. Since it supports non-virtual classes, getting two copies of the base class requires odd code. |
C++ MULTIPLE INHERITANCE | 1. Naming conflicts point to the proper error normally. 2. Can be used for both interface and implementation inheritance. 3. Supports virtual base classes. |
1. C++ classes do not have any knowledge of who inherited them. 2. Poses diamond problem |
In the classic scenario of diamond problem [ Figure 1 ], Ruby simplifies the inheritance to look like D<C<B<A. In C++ Multiple Inheritance, C::foo cannot call B::foo, but with Ruby mix-ins,this is possible.
Conclusion
As we saw, each of the above approaches- Multiple Inheritance, Interfaces, Mixins and Traits - have their own pros and cons. Each one tries to solve the deadly Diamond problem in a different way, but, no one solves it completely. Traits have the disadvantage that they do not allow states. Different languages try to deal with this problem in a different way. JavaFX technology has introduced mixins too.
As mentioned in the Sun Microsystem website [[3]], developers have a new style of class inheritance: a mixin. A mixin is a type of class that can be inherited by a subclass, but it is not meant for instantiation. In this manner, mixins are similar to interfaces in the Java programming language. However, the difference is that mixins may actually define method bodies and class variables in addition to constants and method signatures.
Thus, we can conclude that it is the responsibility of the programmer to make the choice of which mechanism fits the best depending upon the task under consideration.
References
[1] http://ruby-doc.org/docs/ProgrammingRuby/html/tut_modules.html
[2] http://juixe.com/techknow/index.php/2006/06/15/mixins-in-ruby/
[3] http://ruby.about.com/od/beginningruby/a/mixin.htm
[4] http://stackoverflow.com/questions/533631/what-is-a-mixin-and-why-are-they-useful
[5] http://en.wikipedia.org/wiki/Trait_%28computer_science%29
[6] Dave Thomas,Andy Hunt and Chad Fostler: Programming Ruby: The programmatic programmer’s guide Second Edition [4]
[7] http://codeshooter.wordpress.com/2008/09/27/including-and-extending-modules-in-ruby/
[8] http://www.innovationontherun.com/why-rubys-mixins-gives-rails-an-advantage-over-java-frameworks/
[9] http://hestia.typepad.com/flatlander/2009/01/scala-for-c-programmers-part-1-mixins-and-traits.html
See Also
[1] http://www.oreillynet.com/ruby/blog/2007/01/digging_deep_mixing_it_up_or_i_1.html Article from O'Reilly's website which discusses mixins at depth
[2] http://www.parashift.com/c++-faq-lite/multiple-inheritance.html#faq-25.8
[3] http://www.juixe.com/techknow/index.php/2006/06/15/mixins-in-ruby/