CSC/ECE 517 Fall 2010/ch2 S20 CR
Introduction to Code Reuse
Code reuse is, as the name suggests, reusing an applicable portion of code from a previous project into a new project.The concept of code reuse closely binds with the concept of not "Reinventing the wheel". Simple copy & paste code reuse has been used from the beginning of programming. With the development of computing, code reuse now exists in different facets.
The initial phase of code reuse can be traced to '80s when the higher level programming languages became common. Implementation of math libraries is an example of early code reuse although the codes were OS, language or platform specific. With the development of OO systems code reuse concepts like shared libraries , dynamic link libraries were introduced. Microsoft foundation class(MFC) is another example of wide application of code reuse.
Code reuse can be linked to some OO practices like don't repeat yourself (DRY).
Advantages of Code Reuse
The most evident benefit of code reuse is reduction in programming time thus faster project completion. This in turn translates to saved effort and reduced project cost. Other benefits include:
- If one person or team has already solved a problem, and they share the solution, there's no need to solve the problem again.
- Sharing code can help prevent bugs because the amount of total code that needs to be written is reduced. It is assumed that number of bugs is proportional to code size.
- Expert programmers can utilize their strength to build libraries which can be leveraged by other programmers. For example, A security library can be built by security experts while a user interface which uses the library can let UI experts focus on their tasks.
- Specialized libraries can be tuned for performance, security etc. special cases. For example, a Python application might delegate graphics functionality to a C library for performance.
The following diagram shows improvements in different productivity matrices from a research on code reuse.
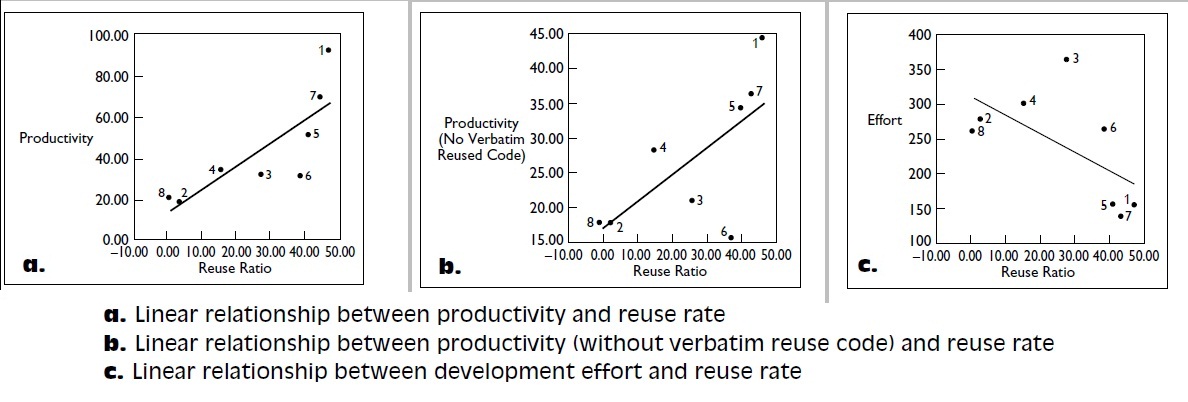
Disadvantages of Code Reuse
Disadvantages are often dependent on the situation and implementation.
- Performance might become a factor depending on the platform and programming language.A library or framework might perform slower than expected.In some situations it might be beneficial to build a specialized one-time solution instead of using a common library.
- Loss of control over third party solutions might cause issues, i.e- they may stop product support. There might also be licensing and liability issues.
- If not well implemented, code reuse can eventually cause code bloat.
Most Common Methods of Code Reuse
Few of the common methods are discussed below.Reference-Types of reuse in Information Technology
Reusing Architectures -
It refers to identifying,analyzing various enterprise architectures in order to lay foundation for developing the software application from scratch.For example in order to develop a web based application we rely on model view controllerarchitecture.
Reusing available Artifacts -
For any software development we can reuse existing document,use cases ,domain-specific models,guidelines.
Reusing Frameworks -
Frameworks are basically reusable set of libraries or classes for a software system.Developer use frameworks in order to build their application easily instead of going into low level details of implementing each and everything.The best example reuse of frameworks is ruby on rails.
Reusing Design Patterns-
Design pattern is a reusable method of addressing a commonly occurring problem in a software design.The best example of reusing design pattern is using abstract classes and interfaces.
Reusing Modules -
Modules are independent,interchangeable component of a software.It represent separation of concern and vastly improve the modularity of the program.Modules can be either object oriented or functional.Because of these features developers can reuse this modules for software development.
Categories of Code Reuse
Coming to implementation of code reuse it can be categorized as follows
- 1.Internal Code Reuse
- 2.External Code Reuse
- 1.Internal Code Reuse
Internal Code Reuse
Suppose a team needs to start a new project.In order to start the project the team can use all possible reusable components that it has built earlier instead of again re-creating them.Such usage of reusable components is referred as Internal Code Reuse.Few such examples of Internal Code reuse are :
- 1.Simple Copy and Paste of the Code
- 2.Using Software Library
- 1.Simple Copy and Paste of the Code
Simple Copy and Paste of the Code
Here we simply try to find the source code that perform the required functionality.We copy and paste the source code into our required code area.But in this case people sometimes try to copy from an external source which is discouraged method and it leads to plagiarism.
Using Software Library
It is the most common example of code reuse.Suppose we want certain manipulating functions like date,time,string or functions related to interfacing of an external database with our program we use software libraries.These libraries are either created by us or these comes as external library in the languages.The code reuse in various languages are.
- Examples of code reuse in C/C++
If we want to use all string functionality we can include string header and call the required method in our code area.
#include "stdio.h" #include "string.h" //Inclusion of string header file int main() { if (strcmp("ncsucsc517","ncsucsc517")== 0) //strcmp is function provided by string library which compares two string. { printf("the string are same"); } return 0; }
We can also create Macro and reuse it.Following example clearly explains it.
#include "stdio.h" #define Cubeof(x) x*x*x //Here at first we define a Macro that calculates the cube of a number int main() { int input = 6; printf("\nCubeof(x)=%i",Cubeof(input)); //Here we reuse the Macro "Cubeof" return 0; }
In addition to above C++ also provides Standard Template Libraries(STL).Few STL libaries are
1.Container Library - Bitset,Dequeue,List,Map,Vector
2.Iterator Library - Iterator
- Examples of code reuse in Java
In java we use Package for code reuse.A Package is a collection of related files with a particular functionality and usability.Generally a package contains classes,interfaces etc.For example if we want to print todays date we can use Date Class provided by java.util package.Sample example illustrates it.
The example of a class inside a package.
java.util.Date today = new java.util.Date();//java.util is a package which contains Date Class System.out.println("Todays Date is "+today);
The example of an Interface inside a package.
interface powerOf { public void squareOf(int number); } public class calculator implements powerOf { public void squareOf(int number) { int result; result = number * number; System.out.println("The square of the number is"+result); } public static void main(String args[]) { calculator obj = new calculator(); obj.squareOf(20); } }
- Examples of code reuse in Ruby
In Ruby the code reuse is mainly done through usage of modules and mixins . The example of Module is
module Power NUMBER = 5 def Power.Square(x) return x*x end def Power.Cube(x) return x*x*x end end cube = Power.Cube(Power::NUMBER) puts cube
The example of Mixin is
class Calculator include Power //We have included the module created above def square_of_number number puts Power.Square(number) //Reusing the code end end obj = Calculator.new obj.square_of_number 20
External Code reuse
External code reuse refers to the usage of a Third party Licensed Tool ,applications or framework.Either they come with certain cost or they are open source.
Some of the examples of external code reuse are
Frameworks
Ruby on Rails (RoR) is an open Source Web Application framework.It is used for rapid creation of web application and it uses agile methodologies.It provides various packages like
1.ActiveRecord
- It is used as object relational mapping with database
- It is used as object relational mapping with database
- It provides webservice
- It provides webservice
- It splits the response to a web request into two part.One goes to controller and another goes to view
- It splits the response to a web request into two part.One goes to controller and another goes to view
- It is the collection of utility classes and standard library extensions
- It is the collection of utility classes and standard library extensions
- It acts as middle man between model and view.It routes the request of view to model by acting on request like save or update.Also it renders data from model to view.
Dont Repeat Yourself (DRY) Principle
In the field of software engineering we consider every code repetition as evil.Developers avoid repeating a piece of code in more than one place.So they strictly follow DRY Principle which states that
"Every piece of knowledge must have a single, unambiguous, authoritative representation within a system." By: Andy Hunt and Dave Thomas.
This principle helps maintain the code very easily.Few examples from Ruby on Rails are.
1.Generators - Rails provides bunch of generators in order to generate the skeleton of the application.It saves lot of time and allow more cleaner way of developing things.
2.Migrate Tools- Suppose a developer wants to change the database structure he or she can use rake::migrate tools in order to create new migration script.The changes get reflected in database as well as in model definition.
3.Partial render - Rail view component provides partial rendering mechanism that helps developer to define overall layout just once and which can be included in several pages layout.
For Further Study
[1] Jones, T. Capers , Reusability in Programming: A Survey of the State of the Art, IEEE Transactions on Software Engineering,Issue Date: Sept. 1984 SE-10 Issue: 5 ,P: 488 - 494 ,ISSN: 0098-5589, Digital Object Identifier: 10.1109/TSE.1984.5010271
[2] Stefan Haefliger, Georg von Krogh, Sebastian Spaeth , Code Reuse in Open Source Software , MANAGEMENT SCIENCE ,Vol. 54, No. 1, January 2008, pp. 180-193 , DOI: 10.1287/mnsc.1070.0748
[3] Victor R. Basili, Lionel C. Briand, Walcélio L. Melo. , How reuse influences productivity in object-oriented systems , Communications of the ACM archive, Volume 39 , Issue 10 (October 1996) Pages: 104 - 116, Year of Publication: 1996 , ISSN:0001-0782
See also:
advantage and disadvantages of code reuse
Tips for Effective Software Reuse
Article:Code Reuse Gets Easier
Code Refuse(A good article portraying a scenario where code reuse is misunderstood)
Why Software Reuse has Failed and How to Make It Work for You