CSC/ECE 517 Fall 2009/wiki2 8 RP
Reflection Oriented Programming
This wiki throws light on what Reflection Oriented programming is all about. It deals with the need for such dynamic aspect and how it is useful in satisfying the practical needs. It further highlights the importance and the limitations of reflection oriented programming languages.
Definition
Reflection is a process of reasoning about and acting up on by itself [1]. Throwing more clarity, we can presume reflection as a computer code which can be dynamically modified in the process of execution. The dynamic modification depends on the code features and its run time behavior. A system which exhibits such reflective behavior is called as reflection oriented system.
Reflection oriented programming – Insights
Reflection
Reflection is the process of converting a programmatically expressed value into a component of interpreter state [2]. Reflection allows program entities to discover things about themselves through introspection.
In the case of usual programs the information about itself (i.e the program) is lost as soon as it finishes compilation, but in reflection the program (object) needs to have information about its structures. This is done by storing it in the form of metadata.
There are two types of reflection based on its dynamic nature. Few programs support reflection during compilation. They are called as compile time or static reflection. Run time reflections are those which are carried on during run time and its the usual form of reflections.
Example of Reflections:
A simple ruby code does enough to understand the concept behind reflections
puts 1.class # print the class of 1 >> Fixnum puts 123456789012345.class >> Bignum
The above code dynamically finds out the class. Thus reflection is done in ruby code to carry out operations during run time with meta programming enabled.
Reflection Oriented Program as paradigm
Reflection oriented paradigm is a new paradigm used as an extension to the object oriented paradigm. It adds various features meeting the need of various applications and its very flexible. Its a dynamic run time paradigm unlike object oriented and procedure oriented model. In this paradigm objects can be varied and modified according to the needs during the run time rather than creating static set of codes with a predetermined set of operations.
The dynamic decision in this paradigm is decided based up on the data being processed and the manipulation performed on those data sets. Here is where the knowledge about its own structure plays a crucial role. On considering an example wherein a program needs to change dynamically on various sets of data (find an instance of that data or length), intercepting the interpreters becomes important. In these cases the program should consist of codes just to identify the data and to decide the operations to be performed on them.
Concepts
The concept of reflection oriented programming is based upon the extension of object oriented program to dynamically shape the program based on its data. All the computations can be divided in to two categories:
- Atomic
- Compound
Atomic refers to when the computation is completed in a single logical step whereas compound refers to the computation that requires the completion of sub computation
To make the concept work as described earlier a new entity called meta information(information about information) is introduced.It takes into account of the details contained in the meta level.It acts as a storage wherein it contains the meta information. It changes as the state changes and its designed to hold the meta information of the current state.On encountering data it derives the information of the state, processes it and triggers the state change. These operations are performed during run time.
Reflectives
A reflective does the job of describing the behavior of meta information. The current state of meta information is reflected by the instances of reflectives or objects of reflectives.
Reflectives can be broadly classified in to two categories.
- Simple reflective and
- Control reflective.
Simple reflective contains the computational concepts whereas control reflectives contains concept related to flow and control.
There is a subcategory of simple reflectives that is designed to contain the meanings of the expressed values. It is called as the value reflectives. The common notation for expressing relative is <reflective>.Numerous examples of reflectives can be found here.
Illustration
Below is an illustration of the concept for reflection in ruby. Here the class person has a function defined which is used to display the name. An object is created to the class Person and we use obj.send to send the object to the function. This shows the dynamic behavior in ruby
class Person def hello (name) puts "hello, #{name}" end end p = Person.new p.send(:hello, "Sam")
List of Reflection Oriented Programming Languages
The list of reflection oriented programming languages includes programming languages which can support and implement reflection i.e. dynamically typed languages like Smalltalk,etc., scripting languages like PERL, etc.
The following is a comprehensive list of the reflection oriented programming languages:
- APL
- Befunge
- Curl
- Delphi
- Eiffel
- Io
- JAVA(java.lang.reflect)
- Lisp
- Logo
- .NET Framework
- Objective-C
- Perl
- PHP
- Python
- Ruby
- Smalltalk
- Tcl
Examples of Reflection Oriented Languages
Example in JAVA
In JAVA, reflection is implemented by using the Java package, java.lang.reflect:
/* In the following example in JAVA, identification of an array using reflection is demonstrated. */ import java.lang.reflect.*; public class FindIfArray { public static void main(String str[]){ int[] arr = {1,9,8,7}; String strg="Hello"; Class cl_arr= arr.getClass(); Class cl_strg= strg.getClass(); if(cl_arr.isArray()) System.out.println(" arr is an array "); else System.out.println(" arr is not an array "); if(cl_strg.isArray()) System.out.println(" strg is an array "); else System.out.println(" strg is not an array "); } }
The output of this above program will be as follows:
C:\Reflection\javac FindIfArray.java C:\Reflection\java FindIfArray arr is an array strg is not an array
Example in C#
To implement reflection in C#, the namespace System.Reflection is used.
The System.Reflection namespace contains classes and interfaces that provide a managed view of loaded types, methods, and fields, with the ability to dynamically create and invoke types7.
using System; using System.Reflection; public class MyClass { public virtual int AddNumb(int numb1,int numb2) { int result = numb1 + numb2; return result; } } class MyMainClass { public static int Main() { Console.WriteLine ("\nReflection.MethodInfo"); // Create MyClass object MyClass myClassObj = new MyClass(); // Get the Type information. Type myTypeObj = myClassObj.GetType(); // Get Method Information. MethodInfo myMethodInfo = myTypeObj.GetMethod("AddNumb"); object[] mParam = new object[] {5, 10}; // Get and display the Invoke method. Console.Write("\nFirst method - " + myTypeObj.FullName + " returns " + myMethodInfo.Invoke(myClassObj, mParam) + "\n"); return 0; } }
In this code:
- Firstly, we obtain the type information and
myTypeObj
will have the information aboutMyClass
- Secondly,
by using the MethodInfo myMethodInfo = myTypeObj.GetMethod("AddNumb");
,we can get the method's information.
- Thirdly, the following code will invoke the
AddNumb
method:
myMethodInfo.Invoke(myClassObj, mParam); //In this example, we will use the typeof keyword to obtain the //System.Type object for a type. Public class MyClass2 { int answer; public MyClass2() { answer = 0; } public int AddNumb(intnumb1, intnumb2) { answer = numb1 + numb2; return answer; } }
Hence, in this way we can use reflection to obtain details of an object, method, and create objects and invoke methods at runtime.
Importance of Reflection Oriented Programming Languages
Reflection is a advanced feature which helps applications work better in the following ways6:
- Dynamic nature
It can be used for modifying or observing a program execution at run time.
- Features can be extended
An application can use external, user-defined classes by generating instances of extensibility objects using their fully-qualified names.
- Class Browsers and Visual Development Environments
A class browser must be capable of enumeration of the class members. Visual Development Environments are at an advantage from making use of type information available in reflection to help the developer in writing code correctly.
- Debuggers and Test Tools
Debuggers must be able to inspect the private members on the classes. Test harnesses can make use of reflection to systematically call a discoverable set of API's defined on a class, to insure a high level of code coverage in a test suite.
- Adaptability to environment & elimination of hard-coding
Consider an application which makes use of two different classes X and Y interchangeably to do similar operations. Without reflection-oriented programming, the application might be hard-coded to call method names of class X and class Y. However, by making use of the reflection-oriented programming paradigm, the application can be designed and written to utilize reflection in order to invoke methods in classes X and Y without hard-coding method names.
- Reflection can be used in aspect-oriented programming.
Aspect-oriented programming is all about enabling the programmer to concisely address functionality that may crosscut the actual implementation of their application 9.
Limitations of Reflection Oriented Programming Languages
There are certain limitations which hinder the usage of the reflection oriented programming languages with the major drawback being performance issues6.
- Performance Overhead
Operations involving reflections have slower performance than the non-reflective counterparts.
Consider the following java code9:
public int accessSame(int loops) { m_value = 0; for (int index = 0; index < loops; index++) { m_value = (m_value + ADDITIVE_VALUE) * MULTIPLIER_VALUE; } return m_value; } public int accessReference(int loops) { TimingClass timing = new TimingClass(); for (int index = 0; index < loops; index++) { timing.m_value = (timing.m_value + ADDITIVE_VALUE) * MULTIPLIER_VALUE; } return timing.m_value; } public int accessReflection(int loops) throws Exception { TimingClass timing = new TimingClass(); try { Field field = TimingClass.class. getDeclaredField("m_value"); for (int index = 0; index < loops; index++) { int value = (field.getInt(timing) + ADDITIVE_VALUE) * MULTIPLIER_VALUE; field.setInt(timing, value); } return timing.m_value; } catch (Exception ex) { System.out.println("Error using reflection"); throw ex; } }
In order to study the performance issues, the program above is called repeatedly with a large loop count.
An average field access time is calculated, and during this, the first call has not been included. Hence, the initialization factor has not been considered as a factor in the results. In this performance test, a loop count of 10 million for each call has been considered and this execution has been performed on a 1GHz Pentium III system.
In the Figure 19: the timing results for three different Linux JVM's(with default settings for each JVM) have been displayed.
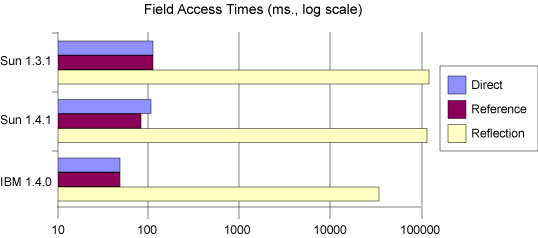
The X-axis, i.e. the logarithmic scale, displays the full range of times. The following observations can be made from the performance test and the above figure:
- In the first 2 cases, the execution time using reflection is 1000 times greater than accessing directly.
- The IBM's JVM has worked considerably better when compared to the Sun JVM's but the reflection method has taken more than 700 times as long as the other methods.
- We can observe that there were no notable differences in the times between the other two methods on the other JVM, though the IBM JVM ran these almost 2 times faster as the Sun JVM's.
- Probably, this difference shows the specialized optimizations used by the Sun Hot Spot JVMs, which tend to do poorly in certain simple benchmarks.
- Security Restrictions
Reflections require a run time permission which may not be present when running under a security manager.
- Exposure of Internals
Since reflection allows code to perform operations that would be illegal in non-reflective code, such as accessing private methods and fields, the use of reflection can result in unexpected side-effects, which may cause the code to become dysfunctional and may destroy portability. Reflective code breaks abstractions and therefore may change behavior with upgrades of the platform.
Conclusion
Reflection is a wide-ranging topic that has been studied independently in various areas of science in general, as well as the field of computer science particularly. Even in the sub-area of programming languages, it has been applied to a variety of paradigms, especially the logic, functional and object oriented ones[8].
With a few exceptions, performance problems of reflection oriented programming languages are rarely addressed and never solved completely in the research of reflection. The best way to overcome this performance problem of reflection is to use less amount of reflection oriented coding in the programming languages.
But to make reflection oriented languages accepted by a wider community we have to prove that the implementation of reflection can take place in a way which does not affect performance too much.
References
- Conference on Object Oriented Programming Systems Languages and Applications : Reflection in an object-oriented concurrent language
- Jonathan M. Sobel Daniel P. Friedman : An Introduction to Reflection-Oriented Programming
- .NET Framework Class Library : System.Reflection Namespace
- Package : java.lang.reflect
- Reflection
- Trail: The Reflection API
- Reflection in C#
- Reflection in logic, functional and object-oriented programming: a Short Comparative Study (1995) by François-Nicola Demers , Jacques Malenfant
- Java Programming Dynamics Part 2: Introducing reflection
- Aspect-Oriented Programming using Reflection and Metaobject Protocols: Gregory T. Sullivan, Artifcial Intelligence Laboratory, Massachusetts Institute of Technology