CSC/ECE 517 Fall 2009/wiki2 3 pp
Synchronizer Token Pattern - Introduction
Synchronizer token pattern is a well know design pattern (Alur 2003) that is normally used in web application development to control flow sequence.
Normal flow sequence of an application can be disrupted by the following ways like
1. Duplicate Form Submissions
2. Access a page in an out of order fashion via bookmarks
Synchronizer token pattern is listed as one of the "Presentation Tier Refactorings" pattern in the Core J2EE Patterns 2nd edition.
Double Submit Problem
Double Submit Problem is a specific instance of the generic Duplicate form submission. It is a common issue with any web based application that has server side transactions. Submission of POST data more than once could result in undesirable results and hence the name : Double Submit Problem . Generally POST requests contain input data and could change the state of the server. So, submitting a form twice or multiple times could result the server in an inconsistent state.
This problem can occur due to any of the following reasons
(a) User clicks the submit button more than once
(b) User inadvertently pressing a back button on the web browser and resubmitting the same form, thereby invoking the duplicate transaction
(c) User refreshes the page after successful completion of the transaction. This will invoke the previous transaction again on the server side
(d) User invokes the transaction, but stops the transaction after the transaction is completed on the server side but before the response is rendered on the screen and refreshes the page causing the transaction to be duplicated
For instance, assuming an user is checking out a cart from an e-commerce site like amazon.com. User does check-out after paying through credit card and the transaction is successful. For whatever reasons, if user inadvertently refreshes this page, then user's credit card could be charged twice.
Solutions for Double Submit Problem
Multiple solutions are available to handle these scenarios are discussed below.
Client Side Solution - Browser Warning Disabling
User can be warned not to submit again and can be asked to wait for a response after submitting. Web browsers display a warning to the user when the user tries to reissue a POST request. However, refreshing pages loaded using GET request is allowed and no browser warning will be shown.
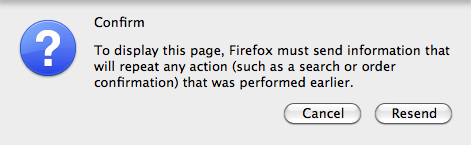
Client Side Solution - Javascript Control Disabling
Client based strategy to this issue is to disable the submit button once the form is submitted. Although this could be an easy solution to implement using javascript, it is not as dependable as server side as it is browser based and can be overridden on the browser.
Server Side Solution - PRG Pattern
POST-REDIRECT-GET idiom or PRG Pattern (Jouravlev 2004) offers a decent solution to this problem. The idiom suggests that a web application should issue a REDIRECT request internally as a response to the initial POST request. This internal REDIRECT will then cause a subsequent GET request as shown in the diagram below:
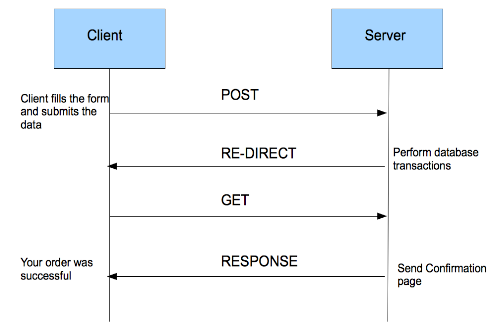
The web browser that receives a redirect response will then add only the redirected GET request instead of adding the original request to its browsing history. Hence this elegantly solves the double submit problem as the redirected request which is being a GET request can be safely refreshed multiple times. Also since the navigational history on the browser will now on contain the idempotent GET requests, user can use back or forward buttons on the browser or bookmark the pages without disrupting the control flow.
Server Side Solution - Synchronizer Token Pattern
Synchronizer token pattern which is explained below can also be applied to this problem as a server side solution.
Synchronizer Token Pattern
Synchronizer token pattern is a well know design pattern (Alur 2003) that is normally used in web application development to avoid duplicate form submissions.
The Synchronizer Token pattern works as follows: When a conversation starts, the application generates a unique token that is stored in the HTTP session. The token is also embedded in each page generated during the conversation, and every request that is part of the conversation is required to include the token value. When a request arrives on the server, it compares the value of the token in the HTTP session with the value included in the request. If the two values match, the request is allowed to continue processing. If they don’t match, an error is generated informing the user that the conversation has ended or was invalidated. The token is removed from the HTTP session when the conversation ends or the session expires.
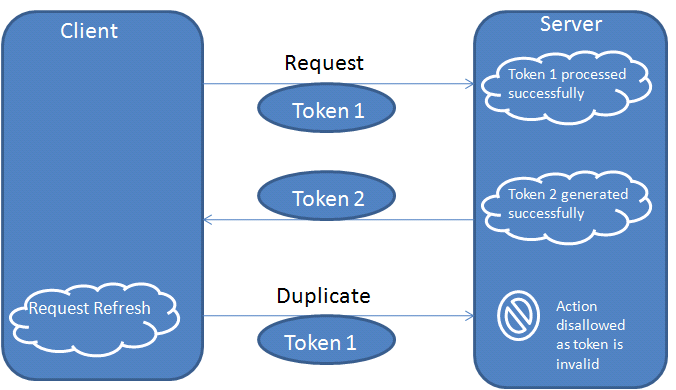
Using POST-REDIRECT-GET avoids accidental double submits of a single request but does not help prevent a user from completing the same business process twice. Such a business process is typically composed of multiple pages spanning several requests. Synchronizer token pattern adds additional safety on top of the POST-REDIRECT-GET idiom by preventing a possibly intentional resubmit of a page. Both the techniques should typically be combined to deliver a complete solution.
The basic idea of Synchronizer Token is to set a token in a session variable before returning a transactional page to the client. This page carries the token inside a hidden field. Upon submission, request processing first tests for the presence of a valid token in the request parameter by comparing it with the one registered in the session. If the token is valid, processing can continue normally, otherwise an alternate course of action is taken. After testing, the token resets to null to prevent subsequent submissions until a new token is saved in the session, which must be done at the appropriate time based on the desired application flow of control. Many web based frameworks provide built-in support for this. However some of the frameworks require serious developer attention whereas some frameworks do provide configurable automatic support. This section will describe Struts and Spring Web Flow provides Web Control Flow.
Struts
Apache Struts provides built in mechanism for handling tokens in org.apache.struts.action.Action class using the saveToken() and isTokenValid() methods. saveToken() method creates a token (a unique string) and saves that in the user's current session, while isTokenValid() checks if the token stored in the user's current session is the same as that was passed as the request parameter.
To do this the JSP has to be loaded through an Action. Before loading the JSP call saveToken() to save the token in the user session. When the form is submitted, check the token against that in the session by calling isTokenValid(), as shown in the following code snippet:
class PurchaseOrderAction extends DispatchAction { public ActionForward load(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { try { //save the token saveToken(request) // rest of the code for loading the form } catch(Exception ex){ //exception } } public ActionForward submitOrder(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { try { // check the token. Proceed only if token is valid if(isTokenValid(request,true)) { //implement order submit functionality here } else { return mapping.findForward("failure"); } } catch(Exception ex){ //exception } } }
This actually what is happening behind the scene in the Action class. saveToken() has logic as below:
HttpSession session = request.getSession(); String token = generateToken(request); if (token != null) { session.setAttribute(Globals.TRANSACTION_TOKEN_KEY, token); }
The method generates a random token using session id, current time and a MessageDigest and stores it in the session using a key name org.apache.struts.action.TOKEN (This is the value of the static variable TRANSACTION_TOKEN_KEY in org.apache.struts.Globals class).
The Action class that renders the form (PurchaseOrderAction.load) invokes the saveToken() method to create a session attribute with the above name. In the JSP, the token needs to be as a hidden form field as follows:
<input type="hidden" name="<%=org.apache.struts.taglib.html.Constants.TOKEN_KEY%>" value="<bean:write name="<%=Globals.TRANSACTION_TOKEN_KEY%>"/>">
The embedded <bean:write> tag shown above, looks for a bean named org.apache.struts.action.TOKEN (which is the value of Globals.TRANSACTION_TOKEN_KEY) in session scope and renders its value as the value attribute of the hidden input variable. The name of the hidden input variable is org.apache.struts.taglib.html.TOKEN (This is nothing but the value of the static variable TOKEN_KEY in the class org.apache.struts.taglib.html.Constants).
When the client submits the form, the hidden field is also submitted. In the Action that handles the form submission i.e. PurchaseOrderAction.submitOrder (which most likely is different from the Action that rendered the form), the token in the form submission is compared with the token in the session by using the isTokenValid() method. The method compares the two tokens and returns a true if both are same. Be sure to pass reset=”true” in the isTokenValid() method to clear the token from session after comparison. If the two tokens are equal, the form was submitted for the first time. However, if the two tokens do not match or if there is no token in the session, then it is a duplicate submission and handle it in the manner acceptable to your users.
If the form is spanned across the multiple pages, then every time the form is submitted before going from one page to another. You definitely want to validate token on every page submission. However you also want to allow the user to traverse back and forth using the browser back button until the point of final submission. If the token is reset on every page submission, the possibility of back and forth traversal using the browser button is ruled out. The solution is not disabling back button (using JavaScript hacks) but to handle the token intelligently. This is where the reset parameter is useful. The token is initially set before showing the first page of the form. The reset parameter is false for all the boolean) isTokenValid() invocations except in the Action for the last page. The last page uses a true value for the reset argument and hence the token is reset in the isTokenValid() method. From this point onwards you cannot use back button to traverse to the earlier form pages and successfully submit the form.
Pros and Cons - Token generation and checking support is built in by struts. Although the above approach is good, it requires application developer to add the token checking method pair – saveToken() and isTokenValid() in methods rendering and submitting the sensitive forms respectively. Since the two tasks are generally performed by two different Actions, the pairs need to be identified and added manually.
However there is another problem with this implementation. HTTPSession can be looked as a HashMap which holds the key-value pair. No duplicate keys are allowed. Struts uses a fix key (Globals.TRANSACTION_TOKEN_KEY) to store the token in the session. This means that at any time only one token can be stored in the session. This prohibits users from opening new windows and having multiple conversations at one time. Because as soon the new window is loaded, new token gets generated overwriting previous window's token in the session. So if the first form in submitted, server would not be able to process as the token in the session and in the request would not match. This is a serious limitation. In order to achieve multiple windows using the struts, developers are required to modify the struts implementation and instead of keeping token directly in the session, it would be stored in a separate HashMap with key as window_id and value as token and this HashMap needs to be stored in the session with the key as Globals.TRANSACTION_TOKEN_KEY. All the places where the session is accessed to retrieve the token are required to be modified and instead of token reading directly from session now it needs to read from the this inner HashMap. This is not only tedious but extremely problematic because then you can not simply upgrade to newer versions of struts because you have customized it. Before adapting newer versions of struts, same modifications as explained above are required.
Next framework provides automatic support for generating and checking token thus developers do not have to worry about forming the pairs. Also it provides multiple windows feature as well.
Spring Web Flow
Spring Web Flow was designed with the intention to help developers implement complex conversations within web applications. Spring Web Flow acts as a controller component in the MVC triad and integrates into hosting web MVC frameworks. It serves as an application controller and handles the screen navigation by coordinating the flow of a business process.
Spring Web Flow captures business processes or conversations in modules called flows. A flow is a blueprint for the interaction a user can have with a web application; it reacts to user events to drive the process to completion. You can look at a flow as a simple manifestation of a finite state machine (FSM), consisting of a number of states that define the activities to execute while progressing through the flow. A state can allow a user to participate in the flow, or it can call business services. The flow can move from one state to another using transitions triggered by events. As a common practice business processes are defined using UML state diagrams, and Spring Web Flow flow definitions use a similar model. The following screenshot shows a Spring Web Flow flow definition that mirrors the process definition in Figure:
Given the navigational rules set out in a flow definition, Spring Web Flow automatically takes care of navigational control. Using web continuations, Spring Web Flow can guarantee stable, predictable behavior of a web application even when the user uses the browser’s Back, Forward, or Refresh buttons; revisits bookmarked pages; or opens multiple windows in the same conversation. The POST-REDIRECT-GET idiom will also be automatically applied, without any need for developer intervention.
Typically, a web flow will define a process spanning multiple requests into the web application. While completing the process, the user interacts with the application through several different pages, accumulating data along the way. It is the responsibility of a flow execution repository to maintain all data associated with a flow execution in between separate requests participating in that flow execution.
Spring Web Flow provides multiple types of repository implementations where the control flow behavior is predefined. Depending on the requirements developer can configure to use a particular implementation of a repository. All of these implementations implements FlowExecutionRepository interface.
- Line 5: To adequately manage flow executions, a flow execution repository needs to assign a unique key to each flow execution. Using generateKey(flowExecution), a new FlowExecutionKey will be generated for a freshly launched flow execution. When an existing flow execution, with a key already assigned, needs to be persisted again, getNextKey(flowExecution, previousKey) generates the next key to use. This implies that a new key can be obtained every time a flow execution needs to be stored in a repository, allowing the repository to potentially change the key every time. FlowExecutionKey objects can be marshaled into a string form using their toString() method. This string form will be embedded in HTML pages and later travels back to the server using the _flowExecutionKey request parameter. Using parseFlowExecutionKey(encodedKey), you can unmarshal a string form of a flow execution key back into its object form.
- Line 12: To make sure all access to a flow execution object occurs in an orderly fashion, a flow execution repository provides a FlowExecutionLock. A flow execution needs to be locked before it is manipulated and unlocked afterward to ensure that all processing done by a flow execution is serialized: the next request is only processed when the previous one completed processing.
- Line 15: Finally, the FlowExecutionRepository interface provides methods to store FlowExecution objects in the repository (putFlowExecution(key, flowExecution)), obtain them from the repository getFlowExecution(key)), or remove them from the repository (removeFlowExecution(key)). Before using any of these methods, the flow execution needs to be locked in the repository.
Figure 6 represents all the built in repository implementations provided by Spring Web Flows. An appropriate implementation can be chosen by using:
<flow:executor id="flowExecutor" registry-ref="flowRegistry" repository-type="simple"/>
In above example simple repository will be used. When using classic Spring bean definitions instead of the Spring Web Flow configuration schema, set the repositoryType property to value SIMPLE:
<bean id="flowExecutor" class="org.springframework.webflow.config.FlowExecutorFactoryBean"> <property name="definitionLocator" ref="flowRegistry"/> <property name="repositoryType" value="SIMPLE"/> </bean>
Simple Repository
The SimpleFlowExecutionRepository guards access to the flow execution by changing the flow execution key for every request thereby imitating the use of a synchronizer token. The methods getNextKey(flowExecution, previousKey) always returns a new key that is different from the previous key. A user can no longer access the flow execution using browser navigation since the request is rendered as stale. The use of Back button on the browser to access history is completely disabled and a user may get an error. When the conversation ends the same situation will occur. When the conversation ends Spring Web Flow will clean up the conversation state and prevent the user form resuming the terminated conversation or to cause duplicate submits.
Pros and Cons - The single most important benefit of using the simple repository is that it only maintains a single copy of the FlowExecution data. These results in very low memory requirements, making this repository type ideal for environments where memory resources are scarce or load requirements are very high.
A downside is that it does not support use of the browser Back button or navigation history and generates an exception if Back button usage is detected. This kind of strict no-Back-button policy enforcement is typically not suited for Internet facing applications. It can be ideal, however, in intranet settings, where you might be able to deploy a custom browser that can completely disable the browser navigational aids.
Single Key Repository
The single key repository is a variation on the simple repository called as SimpleFlowExecutionRepository. It will be configured to keep the flow execution key constant for the entire flow execution. Instead of changing the flow execution key on every request the single key repository maintains a single key for every flow execution and hence it supports the use of Back button. Although, since it maintains only a single snapshot of the flow execution it does not support the use of Back button. When single key repository is used in combination with “always redirect on pause”, all pages of the flow will appear to have been served from exactly the same URL. This is caused by the redirect Spring Web Flow issues before rendering a view. This redirect causes a flow execution refresh. Since the flow execution key remains constant for the entire flow execution when using the single key repository, all pages of the flow will appear to have been served from a URL like this:
http://server/springbank/flows.html?_flowExecutionKey=12345
The browser will not notice that you are actually navigating from one page to another, because every page of the flow has exactly the same URL. The end result is that the browser does not build up a navigation history, making the Back button useless. If you click the Back button, you end up on the last page before the flow execution started! Just like all other repository implementations, the single key repository will also remove a flow execution when it ends. This prevents a user from jumping back into a terminated conversation or causing a double submits.
Pros and Cons - Like the simple repository, the single key repository has an important benefit of using very little memory (since it only stores a single flow execution snapshot). This makes it ideal for high-load or low-memory environments. Although the simple repository completely disallows use of the Back button, the single key repository tricks the browser into not accumulating any browsing history inside the flow execution. This makes going back in the flow using the Back button impossible. This compromise, unlike the strict rules enforced by the simple repository, is typically acceptable for Internet applications.
The Continuation Repository
Continuation Repository is a powerful execution repository provided by Spring Web Flow implemented by the ContinuationFlowExecutionRepository class. As suggested by the name the continuation repository manages flow executions using a web continuations algorithm which are an elegant way to deal with complex navigation in web applications. They allow web applications to respond correctly if the user uses the browser Back button or navigation history or even if the user opens multiple windows on a single conversation against which there is no built in mechanism provide by struts. Struts framework required modification in order to support multiple windows on a single conversion. The continuation repository will take a snapshot of the FlowExecution object at the end of every request that comes into the flow execution. In other words, the “game” is saved at the end of every request. Each continuation snapshot has a unique ID, which is part of the flow execution key. When a request comes in, Spring Web Flow restores the flow execution from the identified continuation snapshot and continues processing. Figure 8 shows this graphically.
- The first request that comes into the flow executor does not contain a flow execution key and hence the flow executor launches a new flow execution for this simple three-state flow. The flow progresses from the first state to a view state which is the second state and then pauses. The flow execution repository takes a snapshot of the FlowExecution and a unique key is assigned. The control is then returned to the Browser. This key is embedded in the rendered view to make sure a next request can submit it again. At the end of the first request, the continuation repository contains a flow execution continuation snapshot, snapshot1, indexed by key1.
- The second request coming into the flow contains key1 as a flow execution key and the response to this request, the flow executor restores the flow execution from the identified continuation, snapshot1. The flow resumes processing and pauses after moving to third state. At this point, the flow execution repository takes another snapshot of the FlowExecution and assigns it a new unique key, which is embedded in the rendered view. The second request caused a second continuation snapshot, snapshot2, to be stored in the repository, indexed using key2. At this point the first snapshot is still present which allows the user to click the Back button and jump back to the previous request to continue from that point onward. Opening a new browser window on the same conversation would allow the user to continue from the current snapshot (snapshot2) independently in each window.
- The third request continues from the continuation identified using key2. In this case, the flow resumes processing and terminates by reaching an end state. As a consequence, the flow execution, and all its continuation snapshots, will be removed from the repository. This prevents double submits, even when using web continuations: if the user clicks the Back button to go back to request two, an error will be produced because the identified continuation snapshot (snapshot2) is no longer available. It was cleaned up along with all other snapshots when the third request terminated the overall conversation. To be able to associate continuation snapshots with the governing logical conversation, Spring Web Flow needs to track both a continuation snapshot ID and the unique ID of the overall conversation. Both of these IDs are embedded in the flow execution key, which consists of two parts:
flows.htm?_flowExecutionKey=_c<conversation id>_k<continuation id>
- The conversation ID is prefixed using _c, followed by the continuation ID prefixed with _k. The conversation ID always remains constant throughout a conversation, while the continuation ID changes on every request.
The continuation repository is the default repository in Spring Web Flow. You can explicitly configure a flow executor to use the continuation repository by specifying continuation as repository type (or CONTINUATION when using the FlowExecutorFactoryBean). The continuation repository has one additional property that can be configured: the maximum number of continuation snapshots allowed per conversation. Using a first-in-first-out algorithm, the oldest snapshot will be thrown away when a new one needs to be taken and the maximum has been reached. Here is a configuration example:
<flow:executor id="flowExecutor" registry-ref="flowRegistry"> <flow:repository type="continuation" max-continuations="5"/> </flow:executor>
The same configuration using classic Spring bean definitions follows:
<bean id="flowExecutor" class="org.springframework.webflow.config.FlowExecutorFactoryBean"> <property name="repositoryType" value="CONTINUATION"/> <property name="maxContinuations" value="5"/> </bean>
By default, a maximum of 30 continuation snapshots per conversation are maintained. In practice, this is equivalent with an unlimited number of snapshots, since it allows a user to backtrack 30 steps in the browsing history—more than any normal user would ever do. Constraining the number of continuation snapshots is important to prevent an attacker from doing a denial of service attack by generating a large amount of snapshots.
Pros and Cons - The continuation repository allows you to have completely controlled navigation, while still allowing the user to use all of the browser navigational aides. This promise is a very compelling indeed and is the reason why this is the default repository used by Spring Web Flow. The most important downside of the continuation repository is the increased memory usage caused by the multiple continuation snapshots that are potentially maintained for each flow execution. By appropriately configuring the maxContinuations property, you can control this, however, making the continuation repository ideal for most web applications.
The Client Continuation Repository
The last type of flow execution repository provided by Spring Web Flow is the client continuation repository, implemented by the ClientContinuationFlowExecutionRepository class. As the name suggests, the client continuation repository also uses a web-continuations–based approach to flow execution management, similar to the default continuation repository. The difference between the two is in where they store the continuation snapshots. The default continuation repository stores continuation snapshots on the server side, in the HTTP session (using the SessionBindingConversationManager). The client continuation repository stores the continuation snapshots on the client side, avoiding use of any server-side state. To make this possible, the client continuation repository encodes the entire continuation snapshot inside the flow execution key. Here is an example of a hidden field in an HTML form, containing a flow execution key generated by the client continuation repository:
<input type="hidden" name="_flowExecutionKey" value="_cNoOpConversation id_krO0ABXNyAGFvcmcuc3ByaW5nZnJhbWV3b3JrL \ ndlYmZsb3cuZXhlY3V0aW9uLnJlcG9zaXRvcnkuY29udGludWF0aW9uLlNlcmlhbGl6 \ lvbi5GbG93RXhlY3V0aW9uQ29udGludWF0aW9ujvgpwtO1430CAAB4cHoAAAQAAAAM- \ ... L3VDqmZZMURBDF7-I73_3g1IND_5AqBLBJQ14z4tUZQRPZFvz_gzBNwW37Z9uff3bRv \ V1SH-1h7xERqN7--u0Td_Y_VVNH6uJkmYYGLgpLzRWn1XHSxuS5tbgYniCt6hNPkGG3 \ OxFhQME1Wdb1B0jLVgMeee364-3eNtM_MW9xgp9c3n8v4NL2FNyjEbHcNZxcvqBbj8i \ 7lZtyOSeXy_0XxBYpbwUkAAABeA.."/>
The continuation ID is a base-64–encoded, GZIP-compressed form of the serialized FlowExecution object.
Pros and Cons - The client continuation repository is a remarkable specimen. It has the major advantage of not requiring any server-side state. This has important benefits in terms of scalability, failover, and application and server management. Additionally, since this repository uses web continuations, it has complete support for use of the browser’s Back button. There is a high cost to pay however:
- By default, the client continuation repository does not use a real conversation manager. As a consequence, it cannot properly prevent double submits and does not support the conversation scope. These issues can be resolved, however, by plugging in a real ConversationManager.
- Because of the long flow execution key, applications are limited to using POST requests. This also rules out applying the POST-REDIRECT-GET idiom using “always redirect on pause”.
- Exchanging a relatively large flow execution key between the client and server on every request consumes quite a bit of bandwidth, potentially making the application slower for users on slow connections.
- Storing state on the client has security implications. An attacker could try to reverse engineer the continuation snapshot, extracting sensitive data or manipulating certain data structures. The ClientContinuationFlowExecutionRepository was designed for extensibility, however, allowing you to plug in continuation snapshot encryption. The client continuation repository provided by Spring Web Flow is an interesting experiment. Future versions of Spring Web Flow will certainly investigate this idea in more depth, for instance, in trying to manage conversations using state stored in client-side cookies. For now, make sure you understand the consequences of using the client continuation repository before deciding to use it.
Selecting a Repository
The matrix shown in Figure makes it easy to pick the correct repository depending on whether or not you need to support browser Back button usage and on the memory constraints of the deployment environment.
In general, though, most applications are best served using the continuation repository. Since this is the default repository in Spring Web Flow, you don’t need to configure anything. Only select a different repository type if you have real requirements or constraints that force you away from the default repository. So unlike struts the complete token management and checking is done automatically without developer having to worry about pairing issues.
Ruby on the Rails
Rails introduced the concept of form authenticity tokens in Rails 2.0. These tokens are designed to block naive attempts to call Rails controller methods from outside of views rendered by Rails. Such attacks are called as Cross-site request forgery (CSRF). Form authenticity tokens are one-time hashcodes that are generated as a hidden parameter for any form that is rendered by Rails. When the form is submitted, the hashcode is passed as a hidden parameter to the Rails controller, and Rails validates this hashcode to ensure that the form submission came from a view generated by Rails. This provides a measure of security against naive attempts to submit the form from other clients, since they will not have the proper hashcode needed to pass the Rails authenticity filter for the form submission. Only HTML/JavaScript requests are checked, so this will not protect application against XML API . Also, GET requests are not protected as these should be idempotent anyway.
When a form is rendered using form_tag or form_for, the hidden authenticity_token is placed right after the <form> tag automatically.
This feature is turned on with the protect_from_forgery method, which will check the token and raise an ActionController::InvalidAuthenticityToken if it doesn‘t match what was expected. You can customize the error message in production by editing public/422.html. A call to this method in ApplicationController is generated by default in post-Rails 2.0 applications. The token parameter is named authenticity_token by default. If you are generating an HTML form manually (without the use of Rails’ form_for, form_tag or other helpers), you have to include a hidden field named like that and set its value to what is returned by form_authenticity_token. Same applies to manually constructed Ajax requests. To make the token available through a global variable to scripts on a certain page, you could add something like this to a view:
<%= javascript_tag "window._token = '#{form_authenticity_token}'" %>
In order to enable Request forgery protection:
config.action_controller.allow_forgery_protection = false
Above configuration will turn off the protection for the whole application. If you want to turn it off only for a single controller then:
class FooController < ApplicationController protect_from_forgery :except => :index # you can disable csrf protection on controller-by-controller basis: skip_before_filter :verify_authenticity_token end
Valid Options:
- only/:except - Passed to the before_filter call. Set which actions are verified.
As you can see there is not really a sophisticated approach built in into the Rails 2.0 as like that of Spring Web Flows for handling Web Control Flows. We expect Rails to get mature over the next few years and add more features like provided by Spring Web Flows.
References
[1] Alur, Deepak, John Crupi, and Dan Malks. 2003. Core J2EE patterns: Best practices and design strategies. 2nd ed. Santa Clara: Sun Microsystems Press.
[2] Redirect After Post, Michael Jouravlev, August 2004
[3] http://www.javaworld.com/javaworld/javatips/jw-javatip136.html?page=1
[4] Synchronizer Token using Struts
[5] Struts implementation of Synchronizer Token
[6] Spring Web Flow