CSC/ECE 517 Fall 2009/wiki2 2 AJAXandMVC
AJAX AND MVC
There are several MVC frameworks that can take advantage of the client-side processing facilities of AJAX. Our aim in writing this wiki page is to consider what parts of the MVC framework (views, controllers?) can migrate part of their functionality to AJAX. In analyzing this problem, we would be considering some server-side languages such as Java, PHP, and Ruby, as well as the .NET framework.
What is MVC ?
Model–View–Controller (MVC) is an architectural pattern used in software engineering. The pattern isolates business logic from input and presentation, permitting independent development, testing and maintenance of each.MVC is often seen in web applications where the view is the HTML or XHTML generated by the app. The controller receives GET or POST input and decides what to do with it, handing over to domain objects (ie the model) which contain the business rules and know how to carry out specific tasks such as processing a new subscription.MVC helps to reduce the complexity in architectural design and to increase flexibility and reuse of code.
What is AJAX ?
AJAX stands for Asynchronous JavaScript and XML.It is a Web development technique for creating web applications. It Makes web pages more responsive by exchanging small amounts of data. It allows the web page to change its content without refreshing the whole page. A web browser technology is independent of web server software. It gives desktop application a feel good factor,by doing behind the scene server communication. A famous example: Google's suggest uses Ajax, and it also helped in making it popular.
Advantages:
Improves the user experience in,
- Analyzing information typed into browser in real time
- Provide a richer experience
- Increases responsiveness of web pages
Improve bandwidth utilization
- Only data which is required is retrieved from the server
How does the magic work??

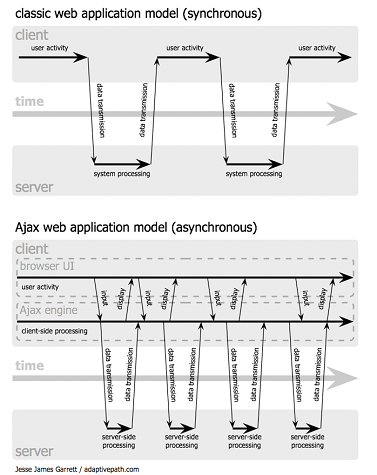
AJAX runs in your browser.
Works with asynchronous data transfers(HTTP requests) between the browser and the web server.
HTTP requests are sent by JavaScript calls without having to submit a form.
XML is commonly used as the format for receiving server data but plain text and some other format may be used as well.
Instead of loading a web page, at the start of the session, the browser loads an Ajax engine — written in JavaScript and usually tucked away in a hidden frame. This engine is responsible for both rendering the interface the user sees and communicating with the server on the user’s behalf.
The Ajax engine allows the user’s interaction with the application to happen asynchronously — independent of communication with the server. So the user is never staring at a blank browser window and an hourglass icon, waiting around for the server to do something.
Every user action that normally would generate an HTTP request takes the form of a JavaScript call to the Ajax engine instead. Any response to a user action that doesn’t require a trip back to the server — such as simple data validation, editing data in memory, and even some navigation — the engine handles on its own. If the engine needs something from the server in order to respond — if it’s submitting data for processing, loading additional interface code, or retrieving new data — the engine makes those requests asynchronously, usually using XML, without stalling a user’s interaction with the application
Technologies used in AJAX.
- JavaScript - JavaScript is a loosely typed object based scripting language supported by all major browsers and essential for AJAX interactions. JavaScript functions in a page are invoked as event handlers when an event in a page occurs such as a page load, a mouse click, or a key press in a form element.
- DOM - is API for accessing and manipulating structured documents. In most cases DOM represent the structure of XML or HTML documents.
- CSS - Allows you to define the presentation of a page such as fonts, colors, sizes, and positioning. CSS allow for a clear separation of the style from the content and may be changed pragmatically by JavaScript.
- HTTP - Understanding the basic request/response interaction model of HTTP is important for a developer using AJAX. You will be
exposed to the GET and PUT method when configuring an XMLHttpRequest and HTTP response codes when processing callback.
Some of the drawbacks:[1]
- AJAX interfaces are substantially harder to develop properly than static pages.
- Pages dynamically created using successive AJAX requests do not automatically register themselves with the browser's history engine, so clicking the browser's "back" button may not return the user to an earlier state of the AJAX-enabled page, but may instead return them to the last full page visited before it. Workarounds include the use of invisible IFrames to trigger changes in the browser's history and changing the anchor portion of the URL (following a #) when AJAX is run and monitoring it for changes.
- Dynamic web page updates also make it difficult for a user to bookmark a particular state of the application. Solutions to this problem exist, many of which use the URL fragment identifier (the portion of a URL after the '#') to keep track of, and allow users to return to, the application in a given state.
- Because most web crawlers do not execute JavaScript code, publicly indexable web applications should provide an alternative means of accessing the content that would normally be retrieved with AJAX, to allow search engines to index it.
- Any user whose browser does not support JavaScript or XMLHttpRequest, or simply has this functionality disabled, will not be able to properly use pages which depend on AJAX. Similarly, devices such as mobile phones, PDAs, and screen readers may not have support for the required technologies. Screen readers that are able to use AJAX may still not be able to properly read the dynamically generated content. The only way to let the user carry out functionality is to fall back to non-JavaScript methods. This can be achieved by making sure links and forms can be resolved properly and do not rely solely on AJAX. In JavaScript, form submission could then be halted with "return false".
- The same origin policy prevents some AJAX techniques from being used across domains, although the W3C has a draft of the XMLHttpRequest object that would enable this functionality.
- AJAX opens up another attack vector for malicious code that web developers might not fully test for.
- AJAX-powered interfaces may dramatically increase the number of user-generated requests to web servers and their back-ends (databases, or other). This can lead to longer response times and/or additional hardware needs.
- User interfaces can be confusing or behave inconsistently when normal web patterns are not followed.
- Due to multiple dependent asynchronous requests, you can’t rely on any order of operations in classical AJAX models.
How does AJAX fit with MVC ?
Ajax functionality needs to be implemented first in the view in terms of the call made and then in controller for handling the call . The view should have the call to the Ajax action which is implemented in controller.
MVC Controller needs to have a ajax-action class to take whatever action is required on the business model in model. Action methods can do things like render different views, render a portion of the user interface defined within a partial view as opposed to the full page. It can be consider that any behavioral JavaScript as part of the Controller, including attaching events as well as sending HTTP requests.
AJAX tag libraries [2] can be to fit ajax into any framework.
Moreover, there has been AJAX MVC[3] used for much smoother integration of ajax into mvc framework.
AJAX IN RAILS
Rails provide support for AJAX in the form of helper methods. There is no need to add JavaScript code in the view templates. Instead, the helper methods create the JavaScript code in the HTML page derived from the view templates. One of the ways this could be understood is through an example [4] of link_to_remote() method which can be used to get the latest time from a server and display it on a web page.Here is a sample view template.
SERVER SIDE CODE
<html> <head> <title>Ajax Demo</title> <%= javascript_include_tag "prototype" %> </head> <body> <h1>What time is it?</h1> <div id="time_div"> I don't have the time, but <%= link_to_remote( "click here", :update => "time_div", :url =>{ :action => :say_when }) %> and I will look it up. </div> </body> </html>
What this view does this is that it gives a link to display the current time. The current time would be fetched in the background without refreshing the browser. Now, there are two main helper methods to do this job. One of them is javascript_include_tag() that includes the Prototype JavaScript library. This is included with Rails package and is a basic requirement to make use of AJAX functionality. The other is link_to_remote() call that talks to the remote server when a request for current time is made. Here is a brief explanation of the parameters used in this method:
- click_here : this is the text for displaying the link
- time_div : it is the id of the HTML DOM element that would have the current time content
- url : refers to the server side action and it is say_when in this case
The role of the index action is to render the index.html file which displays the ‘before’ view. The controller is named demo and is coded as,
SERVER SIDE CODE
class DemoController < ApplicationController def index end def say_when render_text "<p>The time is <b>" + DateTime.now.to_s + "</b></p>" end end
When “click here” is clicked on we would see the ‘after’ view which is shown above. What happens over here is that when a user clicks on “click me” an XMLHttpRequest is created by the browser and sent to the server where in the server invokes the say_when action and renders the HTML response fragment containing the current time. When the client side JavaScript receives the response it replaces the contents of the div with an id of time_div.Besides the above example, there are other AJAX helper methods like the form method, i.e., form_remote_tag(). Here, is a prototype for it.
<%= form_remote_tag :url => { :action => 'create' }, :update => ‘ajax_result’ %> <%= render :partial => ‘form’ %> <%= submit_tag "Create" %> <%= end_form_tag %> <div id="ajax_result"></div>
Ajax in Ruby has immense potential in its application and can add to the efficiency as well as to the looks of a web template. In order to get a more in depth foot in Ajax on Rails , there is a very popular book “Ajax on Rails” by Scott Raymond and published by O’Reilly.
AJAX IN PHP
PHP supports JSON encoding by default, this allows you to pass complex data types back and forth between PHP and Javascript fairly easily.Consider this simple example.[5]
To demonstrate the AJAX PHP connection we will create a very simple form with 2 input fields. In the first field you can type any text and we will send this text to our PHP script which will convert it to uppercase and sends it back to us.
CLIENT SIDE CODE
<script> // Get the HTTP Object function getHTTPObject(){ if (window.ActiveXObject) return new ActiveXObject("Microsoft.XMLHTTP"); else if (window.XMLHttpRequest)//catches the response from the server return new XMLHttpRequest();//this object is for AJAX PHP communication else { alert("Your browser does not support AJAX."); return null; } } // Change the value of the outputText field function setOutput(){ if(httpObject.readyState == 4){ document.getElementById('outputText').value = httpObject.responseText; } } // Implement business logic function doWork(){ httpObject = getHTTPObject(); if (httpObject != null) { httpObject.open("GET", "upperCase.php?inputText=" +document.getElementById('inputText').value, true); httpObject.send(null); httpObject.onreadystatechange = setOutput; } } </script> <form name="testForm"> Input text: <input type="text" onkeyup="doWork();" name="inputText" id="inputText" /> Output text: <input type="text" name="outputText" id="outputText" /> </form>
SERVER SIDE CODE
Server side functionality is very simple compared to the client side. In the PHP code we just need to check the $_GET super-global array. Afterwards convert it to uppercase and echo the result.
<?php if (isset($_GET['inputText'])) echo strtoupper($_GET['inputText']); ?>
AJAX IN .NET Framework
ASP.NET AJAX is the free Microsoft AJAX framework for building highly interactive and responsive web applications that work across all popular browsers. The ASP.NET AJAX framework includes Server-Side ASP.NET AJAX, Client-Side ASP.NET AJAX, the AJAX Control Toolkit, and the jQuery library. ASP.NET AJAX enables developers to choose their preferred method of AJAX development, whether it is server-side programming, client-side programming, or a combination of both.ASP.NET AJAX server-side controls such as the ScriptManager, UpdatePanel, and the UpdateProgress control to add AJAX functionality to an ASP.NET application without writing any JavaScript. For example, the UpdatePanel control enables you to update a portion of an ASP.NET page without requiring you to reload the entire page. The ScriptManager control enables you to manage browser history in an AJAX application by updating the browser back button after an AJAX request.
If you prefer to work directly with JavaScript then you can take advantage of client-side ASP.NET AJAX. The client-side ASP.NET AJAX Library provides a foundation for building rich client-side applications. The library simplifies cross-browser development of client-side applications. For example, the library enables you to call web services and create components and controls -- all through pure client-side JavaScript code.
One cool thing: AJAX Extensions for .NET No need to modify server-side code No javascript functions need to be added AJAX can be enabled/disabled by changing one line of code (“Script Manager”)
Its as simple as 1 2 3 :)
- “Drag and Drop” Script Manager Control onto your form
- Place “Update Panels” around content you wish to have async updates for
- Add triggers to the update panels to tell them which events to update on.
AJAX IN JAVA
Java technology and AJAX work well together. Java technology provides the server-side processing for AJAX interactions. It can provide this through servlets, JavaServer Pages (JSP) technology, JavaServer Faces (JSF) technology, and web services. The programming model for handling AJAX requests uses the same APIs that you would use for conventional web applications. JSF technology can be used to create reusable components that generate the client-side JavaScript and corresponding server-side AJAX processing code.
Consider a simple example [6] where AJAX and java servlets interact.HTML page generated in JSP technology contains an HTML form that requires server-side logic to validate form data without refreshing the page. A server-side web component (servlet) named ValidateServlet will provide the validation logic.
Ajax interaction as they appear in Figure:
- A client event occurs.[7]
- An XMLHttpRequest object is created and configured. [8]
- The XMLHttpRequest object makes a call.[9]
- The request is processed by the ValidateServlet.[10]
- The ValidateServlet returns an XML document containing the result.[11]
- The XMLHttpRequest object calls the callback() function and processes the result.[12]
- The HTML DOM is updated.[13]
Conclusion
Rich Internet applications (RIA) are web application that approximate the look and feel and usability of desktop application. It adds performance and rich GUI. AJAX enables RIA, which uses client-side scripting to make web applications more responsive. Ajax application separates client-side user interaction and server communication, and run them in parallel, reducing the delays of server-side processing normally experienced by user.
MVC frameworks in various languages like PHP, Rails, .NET etc provides well defined support to implement AJAX functionality. This article provided a brief overview of this support in Java, .NET, Rails and PHP.