CSC/ECE 517 Fall 2009/wiki1b 2 SD
Advantages of statically typed vs. dynamically typed languages
Type System
The formal definition of a type system is[1]:
“A type system is a tractable syntactic method for proving the absence of certain program behaviors by classifying phrases according to the kinds of values they compute.”
Type system is the study of types which defines the interaction between various types, the way in which a programming language assigns types. Type systems help detecting errors in the program, provide abstraction, improve the readability of the program, and improve the efficiency of the program [1][2]. Detecting errors related to types in a program is called type checking.
Type Checking
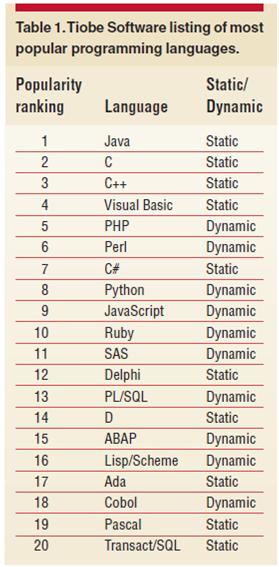
In layman terms type checking is the process of determining errors related to types in the expressions and calculations of a program. There are a few programming languages which are typed and there are a few which are untyped. Among the typed programming languages, based on when the type checking happens, they are either dynamically typed or statically typed. There is another factor used to classify programming languages, that is, the level to which type checking is enforced. Based on the extent to which type checking is enforced, programming languages are either strongly typed or weakly typed.
Therefore, broadly we classify programming languages as:
- Statically typed: Statically typed languages are those languages in which type checking is done at the compile time as opposed to run time. A few examples of statically typed languages are C,C++, C#, Java, FORTRAN, and Pascal, etc.
- Dynamically typed: Dynamically typed languages are those set of languages where type checking is done, unlike static typing, at run time. Examples of dynamically typed languages are Ruby, Prolog, Python, SNOBOL, and Smalltalk etc.
- Strongly typed: Those set of languages where type checking is strictly enforced are called strongly typed languages. Strongly typed languages guarantee type-safety [2]. Examples of strong typing are Lisp dialects, Python, Haskell etc.
- Weakly typed: Weak typing means that the language does implicit type casting. For e.g. consider the following operations:
var a = 5; var b = "string"; var c = a + b; print c;
We know that 5 is an integer and the type of ‘a’ is integer, which is different from the type of ‘b’ which is a string. Therefore, the operation a + b, should not have any value. In a weakly typed programming language, the result might be ‘5string’; this is because ‘a’ is implicitly converted into a string. Examples of weakly typed languages are JavaScript, PHP, Perl etc.
Table 1 shows the list of popular languages and the kind of type checking they enforce[8]. The scenario of the war of fame between the strongly and weakly typed languages can be understood from this table.
Statically typed languages
Static typed programming languages are those in which the variables and their values have types. A variable of type number cannot hold anything other than a number. Types are determined and enforced at compile-time, or declaration-time.
This implies that in statically typed programming languages the variables should be explicitly declaration (or initialization) before they're employed.
Consider the following example:
/*Sample C Code*/ int variable, sum; variable = 5; sum = 10; sum = sum + num;
The above code fragment is an example of how variable declaration in static typed languages generally appears.
There are two main types of static type checking:
- Explicit type decoration: In this kind of type checking the variables, parameters have to be explicitly declared that they are of a given type in the program.
- Implicit type inference: In this kind of type checking the variables are not explicitly decorated with types, but type the programming language automatically decides the type.
Consider the following expression:
(a + string-length(b))
In explicit type decoration it is checked, before the evaluation of the expression, that a is a number and b is a string. Whereas, in type inference by studying the expression it is decided that string-length can only be used on strings therefore b has to be a string and ‘+’ is only used on numbers therefore a has to be a number and string-length(b) also has to be a number.
In the present time Information technology has become a part and parcel of everyone’s life. We cannot imagine a day without a computer. So much are we dependent on the computer that the reliability of software programs is a huge thing. There are many factors which make a program reliable, functional and efficient; one of these factors is static typing. In a statically typed system all the function calls and variables are checked at the compile time and any error detected here is treated as a serious error and the compilation stops and the system does not allow the error to propagate to run-time. With static typing, it is impossible to build a program that would assign a string value to an integer variable. Whereas, in a dynamically typed program, the compiler wouldn’t check for this possibility, and the error would happen at run-time.
To Err is human and humans write computer programs, therefore, static typing helps us write bug free programs. It is nice to know that the compiler is there to point out mistakes in the program. Static typing also helps us make modifications to existing code. If a program does not compile, we know there is a problem in the code and we need to modify it. It also helps us use the code of other programmers, because there is a protocol on how functions should be used [4].
In the statically typed programming language communities, there is a saying that “if it compiles, it works”, this the level of reliability that static typing provides.
The advantages of a statically-typed programming language are that they allow errors to be detected earlier at the compile time, enforce disciplined programming styles, better documentation in the form of type signatures, provides more opportunities for compiler optimizations, generate an efficient object code, and a better design time developer experience [3][6].
There are also few disadvantages about static typing, according John Ousterhout [6]:
“Statically typed systems programming languages make code less reusable, more verbose, not safer, and less expressive than dynamically typed scripting languages”.
Dynamically typed languages
The term dynamically typed programming language refers to a class of programming languages that perform a number of common runtime characteristics that are done by a statically typed language during compilation [9].
A dynamically typed programming language has one or more of the below mentioned characteristics:
- Dynamic typing i.e. variables need not be declared before they are used
- Interpretation by translation into an intermediate representation or machine code dynamically and then executed immediately.
- Runtime modification ability.
According to Blackfriars’ Howe "dynamic Languages are not new" [8]. He emphasized that languages such as APL and LISP were developed in the late 1950’s. Since then there have been many such programming languages, a few of them are ABAP, Groovy, SAS and Tcl – with a few of them becoming widely used [8]. The most popular dynamic programming languages according to [8] are PHP, PERL, Python, JavaScript and Ruby
Consider the following example :
/* Sample Python code */ num = 10; // directly using the variable
In the above code fragment, we have directly assigned the variable num the value 10 before initializing it. This is characteristic to dynamic typed programming languages.
The main advantage of the dynamically typed programming languages is that a programmer doesn’t have the need to initialize variables, which is an add-on to many programmers as stated in the above example. Hence, these languages are called the "kings of code generated at run time" [11]. This feature can be used to fix parts of the language, improve parts of the language and grow it.
Because of their flexibility and malleability, dynamic programming languages are often in “exploratory” programming [9] – "a programming style in which programs are developed in an evolutionary fashion, often utilizing integrated development tools that are part of the system itself." [9]
With dynamically typed languages, programs must define variables but need not explicitly declare them before they are used. Because of this feature dynamic typing eliminates the need to write as much code. This flexibility is liked by many programmers as they can use the variables as they wish without declaring them [8].
Dynamically typed languages provide much more freedom than statically typed languages, in a sense; they seem to be “closer to how real life works”. Productivity is said to be larger with a dynamic programming language[10]. Errors are detected at run time in dynamic programming. This is why run-time matters a lot in the dynamically typed programming languages whereas in statically typed programming languages compile time is important.
A very good example of the advantage of a dynamically typed programming language can be illustrated by demonstrating dynamic finders which are convenient methods provided by Rails which allows a user to find records by their attribute values which concatenates Find_By or Find_All_By with the column names to be filtered[5].
So instead of creating and implementing a method as follows (example from Castles Active Record):
1: public static Card[] Find_By_CardType_And_ExpirationDate(int cardTypeId, DateTime expirationDate) 2: { 3: return FindAll(Expression.And(Expression.Eq("CardTypeId", cardTypeId), 4: Expression.Eq("ExpirationDate", expirationDate))); 5: } 6: 7: ... 8: 9: Card[] cards = Card.Find_By_CardType_And_ExpirationDate(cardTypeId, expirationDate);
The next step to be taken by the programmer is to call the non-existent method which contains the column names and rails will generate the method dynamically [5] .
1: @cards = Card.find_all_by_cardType_and_expirationDate(cardTypeId, expirationDate)
Unlike static languages, Ruby doesn’t need to check if this method exists at the time of compilation. Instead, it throws a method missing exception if the method is not found and provides an option to define or override the method. To provide the Dynamic Finder Functionality, Rails uses regex logic as shown below to parse the method that was passed in method_missing override and then uses the tokens generated dynamically to execute it [5].
1: def method_missing(method_id, *arguments) 2: if match = /find_(all_by|by)_([_a-zA-Z]\w*)/.match(method_id.to_s) 3: # find... 4: elsif match = /find_or_create_by_([_a-zA-Z]\w*)/.match(method_id.to_s) 5: # find_or_create... 6: else 7: super 8: end 9: end
Statically typed vs. Dynamically typed languages
It has always been a war of arguments between developers regarding the statically typed languages versus the dynamically typed languages.
The developers using the statically typed programming languages argue that these type are the best because of their faster execution speed i.e. high runtime efficiency, low probability of errors, and probability of detecting errors at an early point of execution of the program.
On the other hand, supporters of dynamically typed programming languages argue that the static typing is less interactive, highly rigid and that the softness of the dynamically typed languages make them suitable for prototyping systems.
Let us take JAVA and PYTHON for comparison between the statically typed language and dynamically typed language[12].
JAVA |
PYTHON |
---|---|
class A { public static void main(String args[]) { char a; int i; i=10; int j; j=a+i; } } When the above coding is executed, an following error is displayed. A.java:9: incompatible types found : java.lang.String required: int j=a+i; ^ 1 error Hence, in a statically typed language, errors can be detected earlier at the time of compilation. |
Consider the following example : 1. x = "Wikipedia and Python are awesome" 2. if x % 2 == 0: 3. print "x is even" During compile time, PYTHON does not detect the error in line 2. |
VARIABLE DECLARATION : |
VARIABLE DECLARATION: In Python, there is no need to declare any variables explicitly. |
VERBOSE: public class HelloWorld { public static void main (String[] args) { System.out.println("Hello, world!"); } } |
CONCISE: PYTHON is very concise i.e. it can be coded by using fewer number of words. e.g. print "Hello, world!" |
HANDLING MULTIPLE CLASSES:
If there are multiple classes in a program, each class defines its own file. |
HANDLING MULTIPLE CLASSES: If there are multiple classes in a program, all the classes can be defined in just one file. |
EXCEPTION HANDLING:
When an exception arises, it must be thrown and caught by using the try - catch - throw concept. |
EXCEPTION HANDLING: Exceptions are thrown and caught automatically. Explicit declaration is not necessary. |
STRING HANDLING CAPABILITY: |
STRING HANDLING CAPABILITY: Python handles strings efficiently. |
Conclusion
There are developers who support both the types of languages. No one can ever say that one type of a language is better than the other. A mixed approach of usage of both the types of languages can be considered to be a valuable approach & helps build a good software.Some prefer dynamically typed programming languages for simplicity whereas on the other hand some prefer statically typed programming languages as they believe static typing is an important requirement [6].
The performance gap between dynamically typed and statically typed programming languages has been reducing over the last few years, largely due to improvements surrounding JIT compilation – the difference in speed between dynamically typed language with and without a JIT compiler is on an average a factor of 3 to 5. Hence a final decision cannot be made easily regarding a better typed language and so, programming languages shall be chosen by need, and then the choice is up to the programmer [6].
References
[1] Pierce, B. C., & NetLibrary, I. (2002). Types and programming languages. Cambridge, Mass.: MIT Press.
[2] http://www.ljosa.com/~ljosa/teaching/cs162/lectures/L7%20-%20Types.pdf
[3] Martin Abadi et. al, Dynamic Typing in a Statically Typed Language, ACM Transactions on Programming Languages and Systems, Vol. 13, No, 2, April 1991.
[4] http://gnuvince.wordpress.com/2008/02/15/static-typing-%E2%88%A7-dynamic-typing/
[5] “Agile Web Development with rails" by Dave Thomas, David Heinemeier Hansson, Andreas Schwarz (topic : Dynamic Finders)
[6] Erik Meijer and Peter Drayton, Static Typing Where Possible, Dynamic Typing When Needed: The End of the Cold War Between Programming Languages
[7] http://articles.sitepoint.com/article/typing-versus-dynamic-typing
[8] http://dsonline.computer.org/portal/cms_docs_computer/computer/homepage/Feb07/COM_012-015.pdf
[9] http://research.sun.com/techrep/2007/smli_tr-2007-168.pdf
[10] http://robertsundstrom.wordpress.com/2009/06/26/the-dynamics-of-dynamic-programming-languages/
[11] http://gnuvince.wordpress.com/2008/02/15/static-typing-%E2%88%A7-dynamic-typing/
[12] http://www.ferg.org/projects/python_java_side-by-side.html