CSC/ECE 517 Fall 2009/wiki1a 11 f1,
Ruby and Python are both scripting languages whose popularity has sky rocketed in recent years. Both languages are High-Level, Garbage-collected, and Dynamically-typed. Both provide an interactive shell, standard libraries, and persistence support. So, what are the differences?
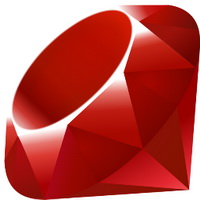

Points of comparison:
- Language Features: Ruby vs Python
- Programming environments
- Features exclusive to each of Ruby and Python
- Advantages of Ruby/Python over statically typed languages such as Java
- Project environments suited to each
Language Features: Ruby vs Python
Access Protection
Ruby supports private, protected and public types of access (like java)to the elements of a class. By default all methods are public except the initialize method and all instance variables are private. One of the differences of Ruby compared to Python is that Ruby keeps all of its instance variables completely private to the class and only exposes them through accessor methods (attr_writer, attr_reader, etc).
Python's property descriptors are similar, but come with a trade-off in the development process. If one begins in Python by using a publicly exposed instance variable and later changes the implementation to use a private instance variable exposed through a property descriptor, code internal to the class may need to be adjusted to use the private variable rather than the public property. Ruby removes this design decision
Functions and methods
In Ruby, the part which is different from Python is the fact that all operations are messages to objects. There are no separate functions and methods; all of them are methods.
Ruby:
string = 'Hello world' puts string.count('o'), string.length # prints 2, 11
In Python, there are separate methods and functions as shown in the example below.
Python:
string = 'Hello world' print string.count('o'), len(string) # prints 2, 11 – why not string.len()?
Ruby has reference to class in class body
Ruby:
class MyClass initialize_magick() end
Rubys variant is cleaner, as the magic stuff is done in the class definition, so you see that it’s being done when you look at the class.
Python:
class MyClass: pass initialize_magick(MyClass)
But it’s really not a big deal, because calling the initialise method after the class or as a decorator is really not a major drawback.
Self Reference
In Python, one needs to write self as the first parameter of a method definition (alike Perl). Furthermore, Python doesn’t require the variable name to be self. In Ruby, self is automatically available in a similar fashion as in C++.
Additionally, the method call self.method can be shortened to method, as self is the default receiver.
Ruby continuations vs Python Generators
Continuation is a "pointer" to the current position in your program, including calling stack and all variables. You can reuse that pointer to "go back in time" when needed. Continuations are useful when it comes to usecases.
def loop cont=nil for i in 1..4 puts i callcc {|continuation| cont=continuation} if i==2 end return cont end
Python doesn't support full continuations or even co-routines; instead it supports "generator" functions which create a kind of limited co-routine. A generator in python is implemented as a special syntax for creating an instance of an iterator object, which returns the values returned by the "function" definition you provide. Python generators are easy and clean. The equivalent code for the above code segment, in python is
from generator_tools import copy_generator def _callg(generator, generator_copy=None): for _ in generator: # run to the end pass if generator_copy is not None: return lambda: _callg(copy_generator(generator_copy)) def loop(c): c.next() # advance to yield's expression return _callg(c, copy_generator(c)) if __name__ == '__main__': def loop_gen(): i = 1 while i <= 4: print i if i == 2: yield i += 1 c = loop(loop_gen()) print("c:", c) for _ in range(2): print("c():", c())
Indentation
Python uses whitespace indentation, rather than curly braces or keywords, to delimit statement blocks (a feature also known as the off-side rule). An increase in indentation comes after certain statements; a decrease in indentation signifies the end of the current block. On the other hand, ruby doesn't need any indentation although it can be optionally used for clarity.
Other Differences
Feature | Ruby | Python |
---|---|---|
Higher-Order Functions | Implemented with procedure objects | Implemented as lambda expressions |
Arrays and hashes | supports Arrays and associative arrays (Hash) | has Lists and Tuples which are the same as arrays. |
Memory management | has a mark and sweep garbage collector | has a reference counting garbage collector. |
Parallel assignment | claims to do Operating System independent threading. | Threading available on many common platforms with some threading support |
Package support | Not available | Available
|
Programming Environments
Development Environments
Both languages provide interactive shells (type "python" or "irb") and high-level persistence support. Multiple IDEs (Integrate Development Environments) are available for Python, including GUI (Graphical User Interface) debuggers. A gdb-style debugger is available for each language. Python has a RefactoringBrowser, BicycleRepairMan. RubyLanguage now has a RubyRefactoringBrowser
Both languages are supported by Emacs modes. Python can be used as an elisp replacement - see Pymacs. The current Ruby implementation is closely tied to Unix, making Windows performance and ports to new platforms problematic. There is an Eclipse IDE for Ruby. RubyCocoa adds support for Ruby to ProjectBuilder/ExCode on MacOsx.
Web Programming Environments
Both Ruby and Python have a large number of web programming environments/ application frameworks. They are exhaustively listed and compared here. For brevity, we compare ruby's Ruby on Rails with python's Django. The need was to compare two frameworks which were developed independent of each other and not based or influenced on the other. Hence the choice of django was made, as other web python frameworks like pylons and web2py are based on rails.
Django is a complete Python web application framework while rails is an agile web programming environment built in Ruby which greatly simplifies MVC (Model-View-Controller) framework development. Ruby OO programming topics in conjunction with various Rails recipes are considered a much lighter, simpler and faster development experience than other current enterprise frameworks provided today.
The table below compares them.
Feature | Ruby on Rails | Django |
---|---|---|
Language | Ruby | Python |
Ajax | Prototype, script.aculo.us | Yes |
MVC framework | ActiveRecord, Action Pack | Yes |
MVC Push/Pull | Push | Push |
i18n & l10n? | Localization, Plug-in | Yes |
ORM | ActiveRecord | Django ORM |
Testing framework(s) | Unit Tests, Functional Tests and Integration Tests | Yes |
DB migration framework(s) | Yes | No (plugin exists, might be merged into trunk when more stable and feature complete) |
Security Framework(s) | Plug-in | ACL-based |
Template Framework(s) | Yes | Yes |
Caching Framework(s) | Yes | Yes |
Form Validation Framework(s) | Yes | Yes |
Features exclusive to each of Ruby and Python
Python Advantages
- Python has multiple inheritance
- Python has docstrings : Docstrings makes it possible to attach documentation directly to the classes and methods. That’s a nice documentation plus, and makes things like the Python interpreters help() function really useful.
Ruby Advantages
- Ruby blocks are much more powerful than Python lambdas and they are integrated pervasively into the language. They also allow interaction between the block and the container.
- Metaprogramming (modifying classes on the fly) is easier in Ruby than in Python. Interestingly, in the Ruby community this is considered a powerful feature and used frequently, whereas the Python community tends to think of it as a last resort.
- Ruby is very good at creating domain-specific mini-languages. This is a consequence of the easy metaprogramming and optional parentheses on function calls.
Advantages of Ruby/Python over statically typed languages such as Java
The term Dynamic language refers to high level programming language that allows the programmer to modify the code during run time. The modifications may include addition of new blocks of code or modifications to objects during execution or changing the type of objects. Statically typed languages such as Java or C# have these operations performed during compile-time as opposed to run-time. further Ruby and Python are dynamically typed; type checking is performed at run-time and typing isn't enforced as strictly as it is in a statically typed language. For example, if an object being passed as an argument to a function doesn't match the type of the argument, but does have a method that matches what is being called, program execution runs normally. This isn't the case in a statically typed language where programmers should declare the types they intend a method or function to use and the compiler will not permit the programmer to ignore this type.
Advantages of a dynamic language are many.
- Dynamic languages are compatible over many platforms.
- Dynamic languages emphasize on the code instead of the structure/ architecture of code. So coding is easier when you don't have to worry about syntax errors and adhering to the language specifications.
- Dynamic code can be written much faster than static code.
- Metaprogramming is easier in a dynamic language. Dynamic languages are suitable if you are working on a template system, or a Domain Specific Language (DSL), a Code Generator, a Parser or other type of application that can build applications. While Static languages can do these things, it takes a lot more effort to achieve it when compared to a dynamic language.
However, a word of warning when starting out using a dynamic language: Dynamic languages put more of a responsibility on the developer when coding. Instead of having a structured syntax that makes sure that the programmer does not make blatant errors, the onus is on the programmer himself. Also, Dynamic languages have a lot of built in shortcuts that ends up revealing code that is not really readable for a beginner.
To aid in understanding how Ruby (or Python) are better than a static language such as Java, consider the examples below:
Purely Object Oriented
- Ruby does not have primitives. everything, including integers are full fledged objects.
- Ruby:
0.zero? This evaluates to a true 1.zero? This evaluates to a false
Typed features
- With Static typing in Java and C#, programmers should declare the types they want a method or function to use while with Ruby you don't declare types for variables or functions. In Ruby objects are strongly and dynamically typed.
- Java:
int val1=5; String value=String.valueOf(val1); if(value.equals("5"))
- Python:
val1=5 value=str(val1) if value == "5"
Verbosity
- Statically typed languages tend to be more verbose.
- Java:
import java.io.*; BufferedReader file1=new BufferedReader(new FileReader(Filename));
- Python:
file=open(Filename);
Relaxed syntax
- Ruby and Python are flexible with respect to syntax. For example,
- Ruby:
cat = Cat.new cat = Cat.new() cat = Cat.new();
More compact code
- Java:
for (int i = 0; i < 100; i++) {}
- Ruby:
100.times { |i| }
Default Arguments
- Ruby and Python allow you to define default values to method arguments while Java does not.
- Ruby:
def calc_cube(x=2,y=3) val=x**y end
Checked Exceptions
- Checked exceptions force every method to deal with (catching or throwing) all exceptions that its child calls or may call.
- Java:
- If in the program, a method calls run as follows, A1() -> A2() -> A3() -> A4() and if A4() throws an Exception, and it is caught by A1(), then A2(), A3() must also throw the same Exception.
- Python:
- Exceptions propagate upwards and A2() and A3() do not need to throw the Exception.
Duck Typing
- Ruby doesn't care about an object's class, just whether it has a method of the name used in the method call. For this reason, the dynamic approach has earned the name duck typing.
- Ruby:
class Duck def sound puts "Quack" end end Class Frog def sound puts "Croak" end end def noises(duck) duck.sound end duckA=Duck.new frogB=Frog.new noises(frogB)
- Result:
"Croak"
Project Environments Suited to Each
Python is useful for rapid prototyping, Web Scripting, XML processing, database applications, GUI applications, scientific computations. Python can be used for large and complex software systems. YouTube, for instance, runs mainly on Python, and it is preferred language at organizations including Google, NASA and Industrial Light and Magic. Specialized Python libraries and frameworks exist for scientific programming, data manipulation, Web services, XML interchange and many other things.
Ruby is useful for typical scripting language applications such as text processing and middleware programs. It is suitable for small, ad-hoc scripting tasks that previously may have been solved with Perl. Ruby has first-class regular expressions, which makes text processing scripts easy to write. Ruby is also suitable for larger software systems. It’s most successful application is in the Ruby on Rails web framework. Websites like Twitter and Hulu use the Ruby on Rails web development framework in production environments.
Ruby can also be used as a high-level API wrapper (or domain-specific language) around some C library. Other uses for Ruby is in test/behavior driven development and when you need to create an "internal" domain-specific language.