CSC/ECE 517/Spring 2023/E2332 Reimplement Courses (Refactor course.rb)
Team Contact
Team Members
- Kartik Rawool, (unityID:khrawool, GitHub:kartikrawool)
- Ameya Vaichalkar, (unityID:agvaicha, GitHub:ameyagv)
- Vikram Pande, (unityID:vspande, GitHub:vikrampande7)
Mentor
- Kartiki Bhandakkar, kbhanda3
Description of Project
The Course
model stores information about the instructor and institution it belongs to, and it is associated with other models such as User, Assignment, etc. The course model is responsible for completing a variety of tasks, including returning the submission directory for the course, viewing students enrolled in the course, and adding a student to the course, etc.
The CoursesController
is responsible for managing courses. It performs CRUD operations on the Course model. It is responsible for creating, editing, deleting courses, and adding/removing teaching assistants to the course. The controller also returns a list of Teaching assistants associated with the course.
Problem Statement
The problem statement requires us to re-implement the Course model and CoursesController in a Rails application, with the goal of managing courses by allowing admins to create, edit, update, and delete courses, as well as create copies of existing courses. The re-implementation should follow RESTful conventions, including appropriate HTTP response codes and status codes for each endpoint. We are required to write RSpec tests for the Course model and the CoursesController, covering all endpoints and including appropriate HTTP response codes for every method. Tests for the controller will be made compatible with RSwag to generate API documentation and test REST APIs from the Swagger UI.
Implementation
Based on the current functionality of the Course model and controller we have defined the following RESTful endpoints with their Request type which would be implemented in the controller. The Strategy Pattern will be followed in the context of a controller, this pattern can be used to encapsulate the actions that the controller can perform and make them easily extensible and usable.
1. Add TA Mapping Model.
We created a model ta_mapping to map the many to many relationship between Course and Teaching Assistant. The model has the following attributes of course_id and ta_id. Through ta_mapping we can keep track of all the TAs assigned to a Course.
2. Deleting the methods that are irrelevant from the controller.
Reason
A controller's main responsibility is to handle incoming requests and manage the data flow between the model and the view. However, having unused or redundant methods in a controller can make the codebase more complex and difficult to maintain. To simplify the codebase and reduce the risk of errors, we removed a few controller methods from the previous implementation of the course controller to simplify and make the code clean.
Work Done
As we reviewed the courses_controller in the previous implementation, we noticed that several methods were not being used in our current implementation of the course model and controller. To streamline the codebase and eliminate unnecessary code, we decided to remove those methods and clean up the controllers and model. Since this is a reimplementation of the controller, we did not include those unused methods in our new implementation.
method removed | reason |
user_on_team | The functionality to check whether a user is a member of any team is associated with team model, follows Single Responsibility Principle |
action_allowed | The course model class does not have the responsibility to check whether the user has instructor privileges. |
auto_complete_for_user_name | This method provides an autocomplete functionality for searching users by their name in the frontend; as our project is to reimplement the backend, this is not required. |
Controller
Index | Method | Request Type | Description |
---|---|---|---|
1 | index | GET | List all courses |
2 | show | GET | Method to get the course with the given id |
3 | update | PUT | Method to update an existing course and save the changes to the database |
4 | copy | GET | Creates a new copy of an existing course with a new submission directory and saves it to the database |
5 | create | POST | Creates a new course and saves it to database |
6 | delete | DELETE | Method to delete an existing course |
7 | view_tas | GET | Displays all the teaching assistants for the given course |
8 | add_ta | GET | Method to add teaching assistant to a course |
9 | remove_ta | GET | Method to remove teaching assistant from a course |
Model
Index | Method | Description |
---|---|---|
1 | path | Returns the course's submission directory |
UML Diagram
Testing Plan
The Course model and controller will be tested using Rspec. FactoryBot will be utilized to create test fixtures and to build the required models. As the methods in the controller are defined as RESTful endpoints. The test take into consideration that the methods return the correct status codes for the output. Based on the current functionality of Course model and controller we have defined the following test which are implemented to test the reimplemented code of course model and controller.
Tests
Course Controller
Test No. | Description |
---|---|
1 | Tests if create method returns a 201 Created status code if the course is successfully created. |
2 | Tests if create method returns a 422 Unprocessable Entity status code if the course cannot be created due to validation errors. |
3 | Tests if the delete method returns a 200 OK status code if the course is successfully deleted. |
4 | Tests if the delete method returns a 404 Not Found status code if the course cannot be found. |
5 | Tests if the update method returns a 200 OK status code if the resource is successfully updated. |
6 | Tests if the update method returns a 422 Unprocessable Entity status code if the course cannot be updated due to validation errors. |
7 | Tests if the copy method return 200 OK status code when new course is created successfully. |
8 | Tests if the view_tas method returns 200 OK status code along with the list of TAs for the course. |
9 | Tests if the add_ta method returns a 200 OK status code if a TA added successfully to the course. |
10 | Tests if the add_ta method returns a 422 Unprocessable Entity status code if a TA cannot be added to the course. |
11 | Tests if the remove_ta method returns a 200 OK status code if the ta is successfully removed from the course. |
12 | Tests if the remove_ta method returns a 404 Not Found status code if the ta cannot be found. |
Course Model
Test No. | Description |
---|---|
1 | Tests if the model validates presence of name |
2 | Tests if the model validates presence of directory_path |
3 | Tests if path method raises an error when there is no associated instructor. |
4 | Tests if path method returns a directory when there is an associated instructor. |
Swagger UI Courses
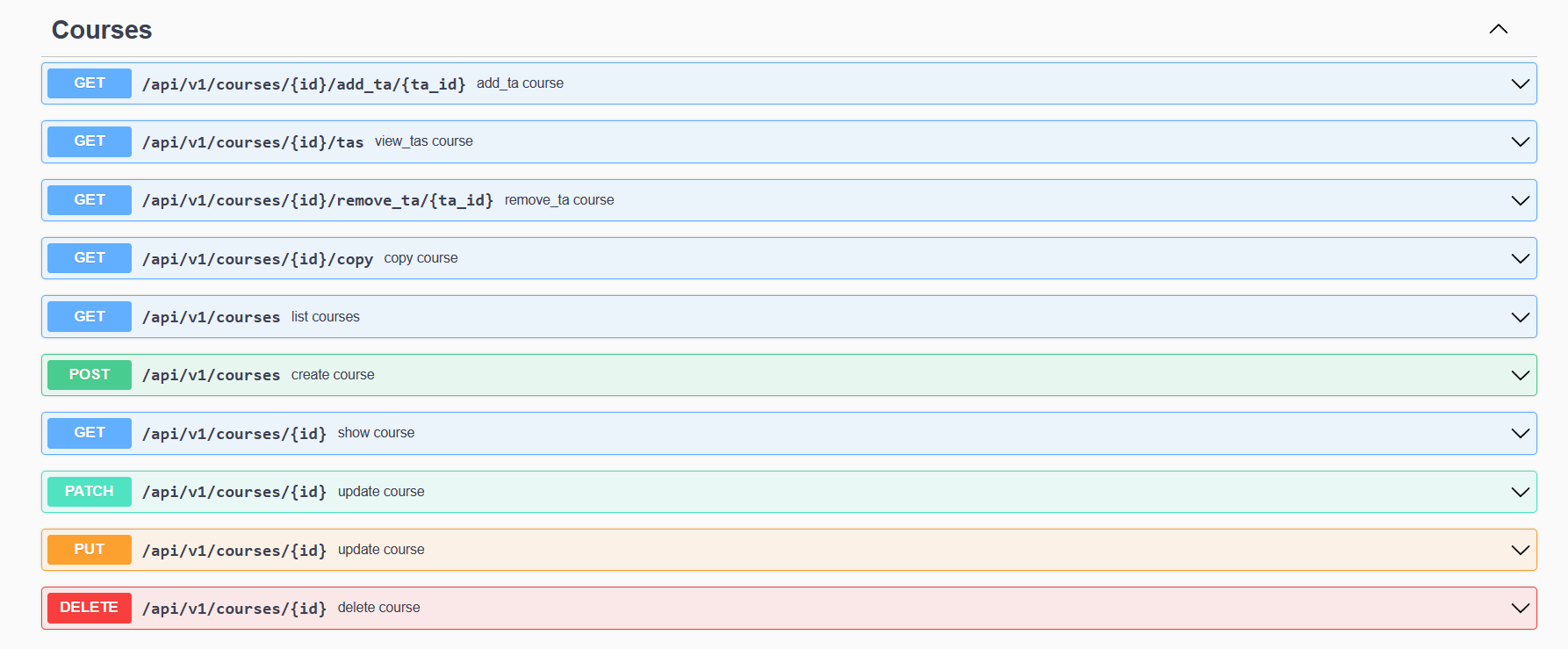
Relevant Links
- Github Repository: https://github.com/ameyagv/reimplementation-back-end
- Pull Request: https://github.com/expertiza/reimplementation-back-end/pull/36
- Video Link: https://youtu.be/22wExrVX15o
Contributors to this project
- Kartik Rawool, (unityID:khrawool, GitHub:kartikrawool)
- Ameya Vaichalkar, (unityID:agvaicha, GitHub:ameyagv)
- Vikram Pande, (unityID:vspande, GitHub:vikrampande7)