CSC/ECE 517 Spring 2023 - E2309. Refactor Node model and its subclasses
Overview of Expertiza
Expertiza is a learning management system that is available as open source software and was created using Ruby on Rails. It has a wide range of features and capabilities, including the capacity to design assignments, quizzes, assignment teams, and courses. It also includes a comprehensive mechanism for offering peer reviews and comments to other teams and groups of teammates. The files that are largely addressed in this project, such as assignment_node.rb
, course_node.rb
, team_node.rb
, folder_node.rb
, and questionnaire_node.rb
, are essential in executing this functionality.
Description of Project
The following is an Expertiza based OSS project which deals primarily with the Node model. The node model defines a tree structure containing Assignments, Courses_table, Questionnaire Types, Questionnaires, and Teams. The node model class is a superclass for the seven files namely assignment_node.rb, course_node.rb, folder_node.rb, questionnaire_node.rb, questionnaire_type_node.rb, team_node.rb and team_user_node.rb. Most of the methods written in node.rb are implemented by polymorphism in their respective subclass files.
The following changes were made:
1. Method names were made more informative of the method wherever necessary.
2. Some common methods in the subclasses were implemented in different ways. So the methods in the subclasses were refactored so that they are uniformly implemented.
3. Appropriate comments were added to the existing code so that the functionality is easier to understand.
4. A method was created to return true/false based on courses.private.
5. Finally, tests were updated according to the changes made in the methods.
Files Modified
Changes to app/models/assignment_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Redefined self.get method and added two new methods self.get_assignment_query_conditions and self.get_assignments_managed_by_user .
|
The self.get method calls these two methods and creates the database query for getting the assignments. Creating two extra methods and calling them from self.get makes the code readable and uniform among all the files.
|
Commit |
2 | self.get_assignment_query_conditions has all the conditions needed for the database query.
|
It makes it easy to list all the query conditions at one place rather than in one method. | Commit |
3 | self.get_assignments_managed_by_user manages all the user permissions with various conditions.
|
It is better to maintain all the rights and accesses at one place rather than in the same method as self.get .
|
Commit |
4 | Renamed the methods get_max_team_size , get_is_intelligent , get_require_quiz , get_allow_suggestions to get_max_assignment_team_size , get_assignment_is_intelligent , get_assignment_require_quiz get_assignment_allow_suggestions .
|
Adding the word assignment to the methods name makes the methods more informative and adds meaning. | Commit |
5 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/controllers/tree_display_controller.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Renamed the methods that were renamed in assignment_node.rb above
|
The methods tha renamed in assignment_node.rb have to be the same in controller so that when they are called, it does not give an error.
|
Commit |
Changes to app/models/course_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Renamed the method get_survey_distribution_id to get_course_survey_distribution_id .
|
Adding the word course to the method name makes the method more informative and adds meaning to survey distribution id. | Commit |
2 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/models/folder_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Renamed the method get_child_type to get_folder_child_type .
|
Adding the word folder to the method name makes the method more informative and adds meaning to the child type of the folder. | Commit |
2 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/models/node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Changed the method name from get_child_type to get_folder_child_type
|
The method name was changed in the folder_node.rb . Since node.rb is its super class, the method name in it also has to be the same
|
Commit |
Changes to app/models/questionnaire_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Redefined self.get method and added two new methods self.get_questionnaire_query_conditions and self.get_questionnaires_managed_by_user .
|
The self.get method calls these two methods and creates the database query for getting the assignments. Creating two extra methods and calling them from self.get makes the code readable and uniform among all the files.
|
Commit |
2 | self.get_questionnaire_query_conditions has all the conditions needed for the database query.
|
It makes it easy to list all the query conditions at one place rather than in one method. | Commit |
3 | self.get_questionnaires_managed_by_user manages all the user permissions with various conditions.
|
It is better to maintain all the rights and accesses at one place rather than in the same method as self.get .
|
Commit |
4 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/models/questionnaire_type_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/models/team_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to app/models/team_user_node.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Added appropriate comments for all the methods of the file. | Understanding the need of the method: A well-written comment makes it clear about what that method is supposed to do.
Code readability: Comments help to break up large blocks of code and make it easier to understand what is happening in each section. Maintenance and updates: When the developer re-visits the code that was written months or years ago, it can be difficult to remember how each method works. Team Work: While working with a team, comments to methods can help other coders understand your code and can facilitate collaboration. |
Commit |
Changes to spec/models/course_node_spec.rb
# | Change | Why? | Commit Link |
---|---|---|---|
1 | Changed the method name from get_survey_distribution_id to get_course_survey_distribution_id in the test case where it is used
|
Any changes made to the name of methods in the files should also be reflected in the test cases for them to pass successfully. If a method name is changed in a file and the same is not updated in the test case, the test case will fail | Commit |
Testing
Everything in the testing segment was beyond the scope of our work. However, we wanted to validate our code through RSpec testing before merging into the main
Expertiza branch. In the second submission phase, we plan to further enhance the testing suite of the auth_controller.rb
and password_retrieval_controller.rb
through factories and fixtures.
Test Plan - Manual/System Test Cases
Along with the unit tests that we have written to test our files, we conducted a few system tests manually to verify the functionality works as expected. The password_retrieval_controller.rb
is difficult for us to test because we don't have access to the email used to make the sample account, but the auth_controller.rb
is very testable. Below is the tests we completed listed in the order of completion.
1) Test instructor login & redirect to home page
When navigating to the expertiza website, our branch correctly displayed the login page as shown below.
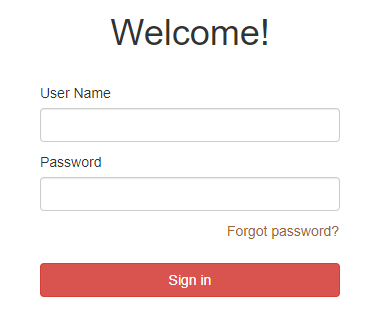
After typing in the sample login credentials of "instructor6" and "password," the user is then correctly logged in and redirected to the home page for instructors shown below.
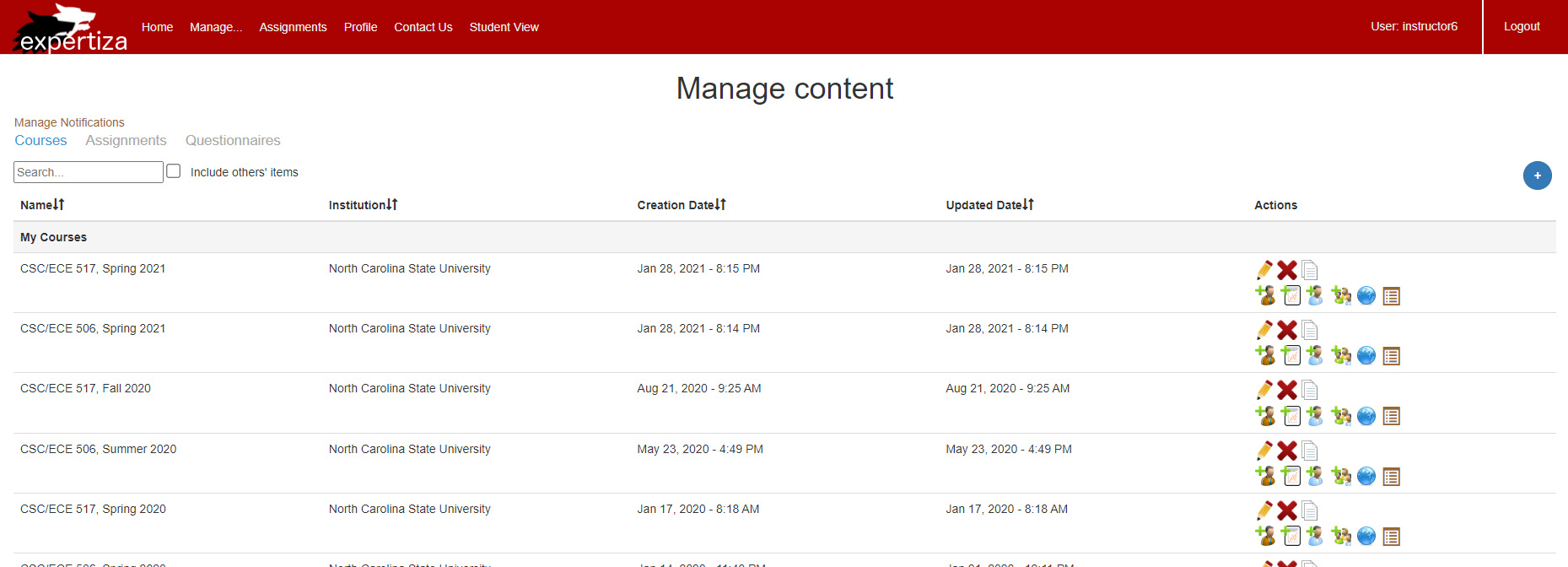
2) Test instructor logout & redirect to login page
Then, when the user clicks the logout button, they are correctly logged out and redirected once again to the login page as shown below.
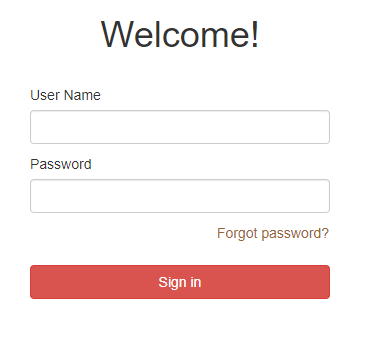
3) Test incorrect login
When the user attempts to login but enters the incorrect login information of "instructor6" and "incorrect," the user is shown the error message and then correctly redirected to the forgot password page shown below.
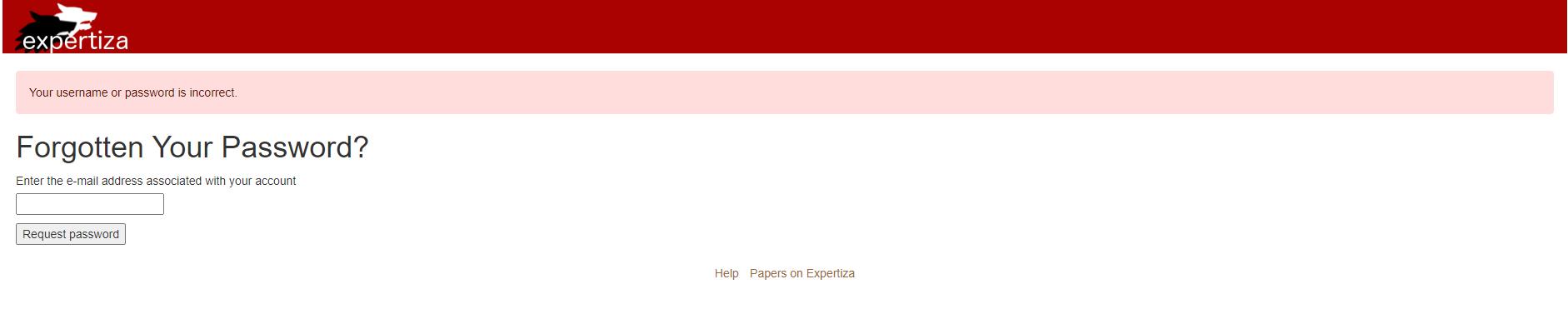
Automated Testing of course_node.rb
After refactoring the code for course_node.rb
, all the test cases passed for it.
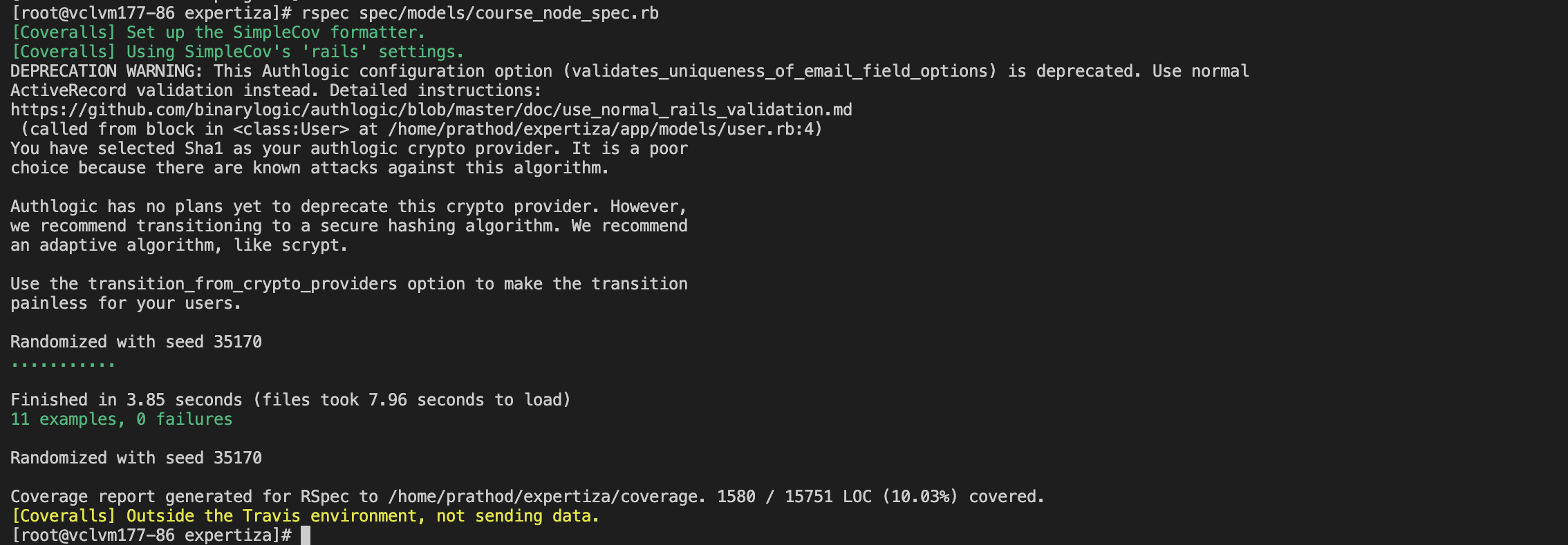
Automated Testing of questionnaire_node.rb
After refactoring the code for questionnaire_node.rb
, all the test cases passed for it.
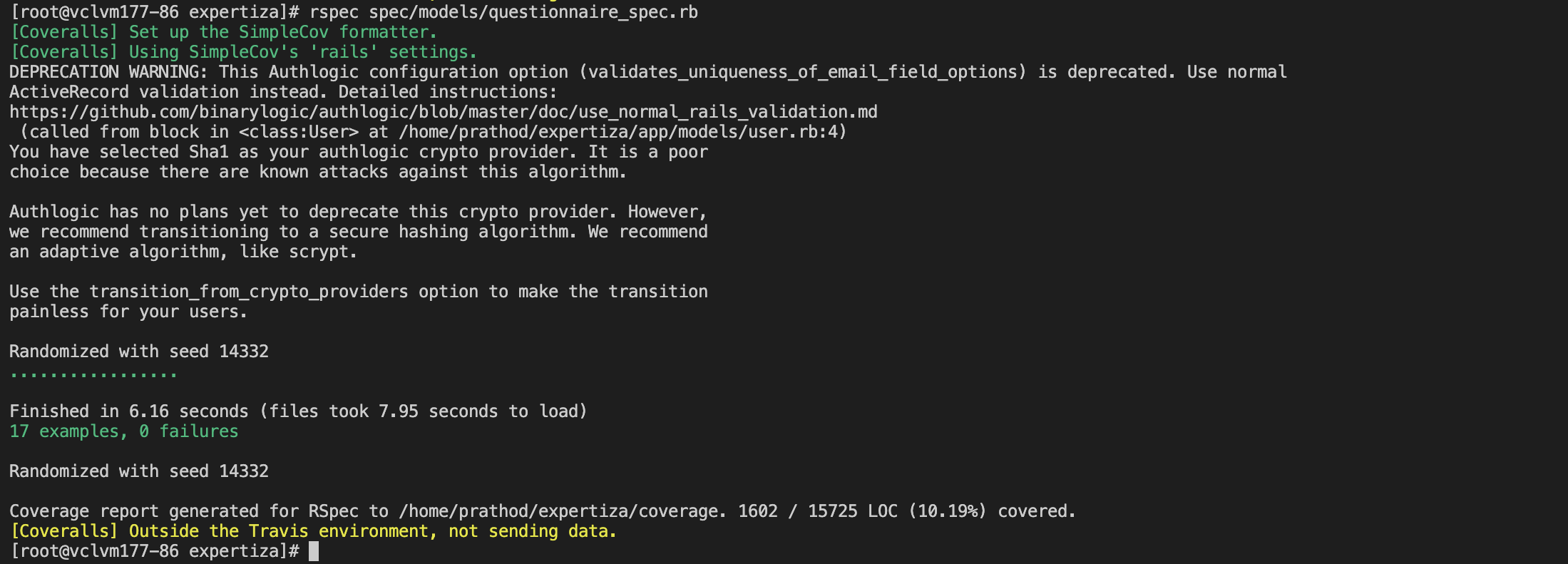
Automated Testing of team_node.rb
After refactoring the code for team_node.rb
, all the test cases passed for it.
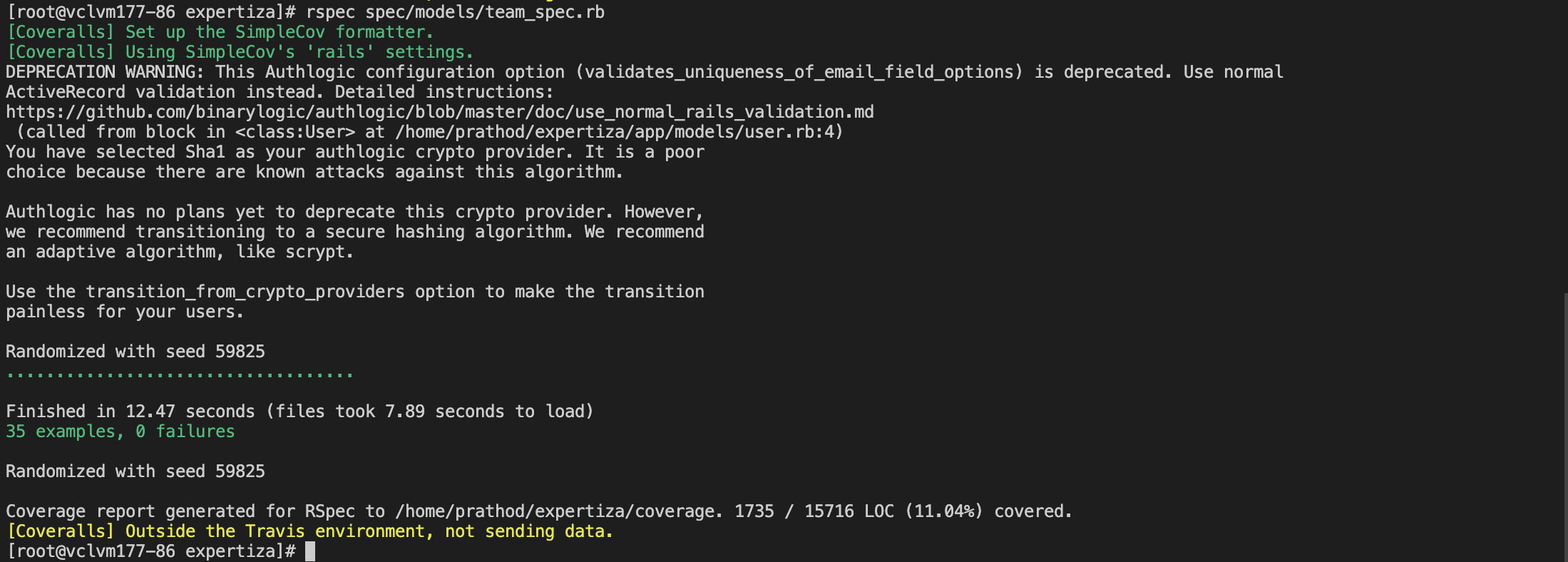
Relevant Links
- Github Repository: https://github.com/palash27/expertiza/
- Pull Request: https://github.com/expertiza/expertiza/pull/2558
- VCL Server: http://152.7.177.86:8080/
Credentials
Username: instructor6
Password: password
Contributors to this project
- Palash Rathod (unityid: prathod, github: palash27)
- Neha Kale (unityid: nkale2, github: nehakale8)
- Vansh Mehta (unityid: vpmehta2, github: vanshmehta-7)