CSC/ECE 517 Fall 2024 - E2489. Create a UI for Assignment Edit page "Etc" tab in ReactJS
Expertiza
Expertiza is an open-source, web-based application built on Ruby on Rails. Its main purpose is to provide flexible, interactive assignment management tools, tailored to educational settings. With Expertiza, instructors can create and customize assignments, specifying topics and tasks that students choose based on their interests. This system not only supports varied, self-guided learning experiences but also encourages active student engagement through choice.
The platform is designed to support teamwork, enabling students to collaborate on projects, which aligns well with team-based learning approaches. One of Expertiza’s most notable features is its peer review system. This system lets students evaluate each other’s work, fostering skills in critical thinking, feedback, and self-reflection that are beneficial in both academic and professional contexts.
The review feature on Expertiza is versatile, accepting multiple file formats, URLs, and even content from external sources like wiki pages. This adaptability makes Expertiza suitable for handling a wide variety of assignments and multimedia projects, fitting well with different teaching styles and course needs. Overall, Expertiza is a solid, comprehensive platform that enhances assignment management, collaborative learning, and peer-to-peer interactions within educational settings.
Problem Statement
The "Etc" tab on the Assignment Edit page of the Expertiza application is due for a redesign using ReactJS. Currently, this section, which houses various miscellaneous settings, is cluttered and outdated. It lacks intuitive features that would make it easier for users to configure assignment options efficiently. Enhancing this tab aims to improve navigation, layout, and overall aesthetics while retaining its existing functionalities.
A revamped UI should organize settings into clear sections using collapsible panels or tabs, which can help users find specific options quickly without having to scroll through a long list. Introducing tooltips or information icons next to less common settings can also offer helpful context, especially for new users.
Visually, the redesign should use a modern color scheme and flat design elements consistent with the rest of the Expertiza application, giving the tab a cohesive and polished appearance. Replacing text-heavy fields with toggles, dropdowns, or sliders can also streamline the interface, making it more user-friendly. Following ReactJS best practices, the improved "Etc" tab would offer a responsive, engaging, and intuitive interface that simplifies the management of additional assignment configurations and aligns well with the overall platform design.
Introduction
This project aims to upgrade the user interface of select parts of the Expertiza application by utilizing modern front-end tools like ReactJS and Typescript. The focus is on redesigning key pages to make them more intuitive, responsive, and easier for users to navigate. By revamping these elements, we aim to solve current usability challenges, simplify the user journey, and create a unified visual style throughout the platform. The enhanced interface will help users handle assignments and tasks more effectively, aligning with contemporary design principles and elevating the overall experience.
Design
Frontend (React & TypeScript)
The new "Etc" tab interface for the Assignment Edit page in Expertiza will be developed using **React** and **TypeScript**. By incorporating TypeScript, this project aims to create a user interface that is not only visually modern but also highly maintainable and type-safe. TypeScript’s built-in type-checking feature will catch errors during the coding process, significantly reducing issues that might otherwise arise at runtime. This will help streamline debugging and ensure a cleaner, more efficient codebase, ultimately improving both the functionality and longevity of the application.
Component Structure
Main Container Component (`EtcTab`)
- The `EtcTab` component will act as the main container for the "Etc" tab, organizing and rendering individual task components in a grid or card layout.
- It will house all task-specific components (`AddParticipantButton`, `CreateTeamsButton`, `AssignReviewerButton`, etc.) and ensure layout responsiveness.
Task Button Components
- AddParticipantButton
A button component that, when clicked, triggers a modal or redirects to the participant addition form.
- CreateTeamsButton
A button component to initiate the team creation process, either by redirecting to a dedicated page or displaying a modal with relevant options.
- AssignReviewerButton
A button component that opens the reviewer assignment view or triggers actions related to reviewer management.
- ViewSubmissionsButton
A button allowing users to view all assignment submissions, either by navigating to a submissions page or displaying them in a modal.
- ViewScoresButton
A button component for viewing assignment scores, either showing a summary in a modal or linking to a detailed scores page.
- ViewReportsButton
A button component for accessing assignment reports, providing users with an overview of feedback and performance metrics.
- ViewDelayedJobs
A button component for viewing delayed background jobs associated with the assignment
Styling
The UI will be styled using CSS or CSS-in-JS (e.g., `styled-components`) for a cohesive and visually appealing layout.
Grid or Card Layout
- Task buttons will be displayed in a grid or card format, providing a clear and organized view.
- Each button will use color coding and icons for better readability and easy navigation.
Responsive Design
- The UI will adapt to various screen sizes, optimizing for both desktop and mobile devices to ensure a consistent user experience.
- Components will have distinct styles with hover effects and smooth transitions to enhance interactivity.
Use Case Diagram
For this project, creating a use case diagram for the "Etc" tab on the Assignment Edit page in Expertiza could highlight various interactions with the UI. Here's a general outline of possible actors and use cases:
Actors:
- Instructor/Admin - Primarily interacts with the UI to manage assignment-related tasks.
Use Cases:
- Add Participants - Instructor can add students or groups to the assignment.
- Create Teams - Instructor creates teams within the assignment.
- Assign Reviewers - Instructor assigns reviewers to specific submissions.
- View Submissions - Instructor views all submissions related to the assignment.
- View Scores - Instructor views scores assigned to each submission.
- View Reports - Instructor can view detailed reports, such as peer reviews and grade distribution.
- View Delayed Jobs - Instructor checks for delayed background jobs associated with the assignment, providing visibility into any tasks still pending or scheduled in the system.
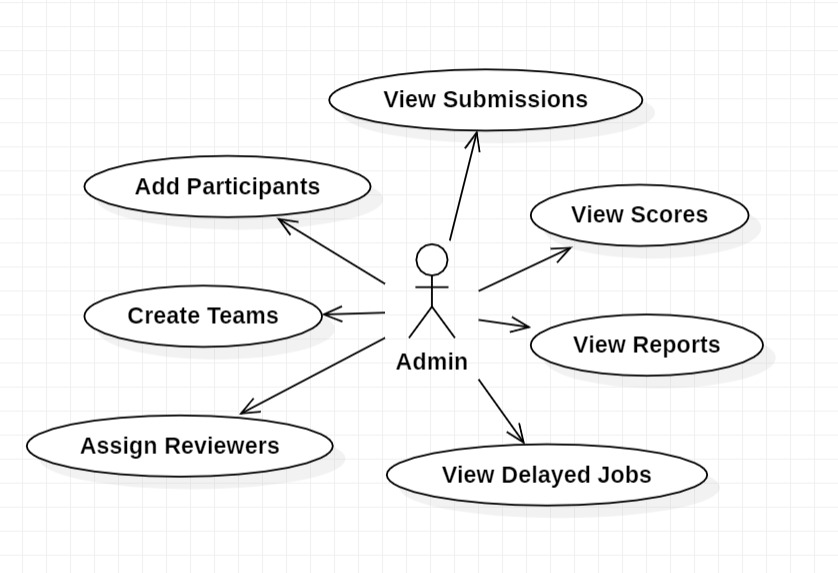
Plan for Reimplementation
The reimplementation of the "Etc" tab will follow a systematic approach using ReactJS and TypeScript, emphasizing maintainability, scalability, and user experience. Each action in the "Etc" tab will be handled as distinct tasks, ensuring modularity and a clear separation of concerns. The following plan outlines the key actions and methods for the new interface:
Add Participant Action
The `AddParticipantAction` component will be responsible for handling the addition of new participants. It will collect participant information through a form, perform front-end validations, and send a POST request to the backend API. TypeScript will ensure type safety, while error handling will manage invalid or missing input data. Upon successful addition, the new participant's details will be displayed in the UI, updated in real-time.
Create Teams Action
The `CreateTeamsAction` will enable users to group participants into teams. This action will include a user-friendly interface for selecting participants and defining team sizes or names. The component will handle API requests to create and save team data. The state will be managed using React’s Context API, ensuring all updates reflect instantly across the interface. Type checking with TypeScript will prevent errors during team creation, and users will receive feedback on the success or failure of the action.
Assign Reviewer Action
The `AssignReviewerAction` will facilitate the reviewer assignment process. It will provide a list of available reviewers and allow users to select and assign them to participants or teams. The action will validate reviewer availability and participant eligibility before sending the assignment request to the backend. Error handling will address conflicts, and the UI will display the updated reviewer assignments in a structured list or table.
View Submissions Action
The `ViewSubmissionsAction` component will retrieve and display all submissions related to the assignment. It will fetch submission data from the backend using API calls and present it in a sortable and filterable table. Users can view individual submission details, and TypeScript interfaces will ensure consistent data structures. This action will improve user access to assignment submissions, providing a streamlined review experience.
View Scores Action
The `ViewScoresAction` will offer a summary of assignment scores. It will make GET requests to the backend API to fetch scores data, displaying it in an organized format. The component will include options for filtering scores by participant, team, or date. Type safety will be enforced using TypeScript, reducing the likelihood of data-related errors. The action will focus on delivering clear and concise score information to users.
View Reports Action
The `ViewReportsAction` will handle the retrieval and display of assignment reports. It will connect with the backend to pull comprehensive reports data, which will be shown in a user-friendly format with options for sorting and exporting. This action will provide users with valuable insights into assignment performance, feedback, and trends, leveraging the structured data from the backend API.
View Delayed Jobs Action
The `ViewDelayedJobsAction` allows instructors to check for delayed background jobs related to the assignment, providing visibility into tasks that are pending or scheduled in the system. This feature helps track jobs like notifications and score calculations, ensuring transparency and easy monitoring.
This structured approach will ensure a cohesive and efficient reimplementation of the "Etc" tab, enhancing both the usability and functionality of the Expertiza application. Each action and method is designed with a focus on modularity, data integrity, and user feedback, leveraging modern development practices with React and TypeScript.
Design Principles
Single Responsibility Principle (SRP)
Each React component will have a single responsibility, focusing on one specific part of the UI or functionality. For instance:
- The AddParticipantButton component handles only the participant addition functionality.
- The CreateTeamsButton component manages the team creation process independently. This separation of concerns makes the code easier to understand, test, and maintain.
Open/Closed Principle
Components and functions in this project will be designed to be open for extension but closed for modification. For example:
- The EtcTab container component will be built to accommodate additional task buttons (e.g., new features) without modifying its core logic.
- New task buttons can be added as separate components, extending the UI without altering existing components.
DRY Principle
The DRY (Don’t Repeat Yourself) principle will be applied throughout this project to reduce code duplication and enhance maintainability. Reusable components like `TaskButton`, centralized API service functions, shared state via React’s Context API, and utility modules will ensure that common logic is abstracted and reused across the application. This approach will streamline the code, making it consistent, easier to update, and more scalable, while also improving readability and reducing the risk of errors. By following the DRY principle, we aim to create a clean and efficient codebase for the "Etc" tab interface.
Conclusion
This design document introduces a step-by-step plan for revamping the "Etc" tab on Expertiza's Assignment Edit page, aiming for a user interface that's smooth and straightforward. Using React and TypeScript, the goal is to simplify administrative tasks—like managing participants, setting up teams, and assigning reviewers—so they’re easier and faster to handle. A clean and modular component design lies at the heart of this solution, making it not only simple to maintain but also flexible enough for future upgrades. Prioritizing responsive design and performance boosts ensures that users enjoy a consistent and efficient experience across different devices.
Team
Mentor:
Kashika Malick (kmalick@ncsu.edu)
Students:
Pankhuri Priya (ppriya2@ncsu.edu)
Sreeja Nukarapu (snukara@ncsu.edu)
Navyatej Tummala (ntummal3@ncsu.edu)