CSC/ECE 517 Fall 2024 - E2475. Reimplement student task view
Expertiza Background
Expertiza is an open-source, web-based application built on Ruby on Rails. Its main purpose is to provide flexible, interactive assignment management tools, tailored to educational settings. With Expertiza, instructors can create and customize assignments, specifying topics and tasks that students choose based on their interests. This system not only supports varied, self-guided learning experiences but also encourages active student engagement through choice.
The platform is designed to support teamwork, enabling students to collaborate on projects, which aligns well with team-based learning approaches. One of Expertiza’s most notable features is its peer review system. This system lets students evaluate each other’s work, fostering skills in critical thinking, feedback, and self-reflection that are beneficial in both academic and professional contexts.
The review feature on Expertiza is versatile, accepting multiple file formats, URLs, and even content from external sources like wiki pages. This adaptability makes Expertiza suitable for handling a wide variety of assignments and multimedia projects, fitting well with different teaching styles and course needs. Overall, Expertiza is a solid, comprehensive platform that enhances assignment management, collaborative learning, and peer-to-peer interactions within educational settings.
Project Description
In the Expertiza application, the student task view acts as a central hub where students can access crucial assignment information, manage submissions, and monitor their progress. This feature consolidates all assignment details in one place, including the assignment title, stage deadlines (for submission, review, and feedback), and individual progress updates. By organizing information clearly, the task view helps students stay on top of their responsibilities, making it an essential aspect of the Expertiza experience.
Our goal with this reimplementation is to redesign the student task view using modern frontend technologies like React JS and TypeScript, enhancing the app’s performance and responsiveness. These technologies will enable us to build a more intuitive, interactive interface with real-time updates, allowing students to see any changes in deadlines, submission statuses, or feedback availability instantly. This upgrade focuses on usability, aiming to deliver a smooth, user-friendly experience that helps students efficiently manage their academic tasks.
The project highlights three key features to meet this goal:
- Task Details View: This feature will present key assignment details such as the title, submission and review due dates, progress status, and any earned badges. By providing a straightforward overview, students can quickly understand their current status and tasks without navigating through multiple pages.
- Task Interaction: Aiming to simplify task interactions, the new design will feature accessible options for submitting work, requesting revisions, and accessing feedback from instructors or peers. Actions will be streamlined to minimize steps, making assignment management easier for students. Improved navigation will also allow students to switch seamlessly between different sections within the task view.
- Timeline Visualization: The new design will integrate a visual timeline, clearly marking assignment deadlines and review stages. This timeline will highlight key due dates and milestones, enabling students to track their progress visually and plan for upcoming tasks. By seeing the assignment flow in a visual format, students can better manage their time and stay on track throughout the assignment cycle.
This reimplementation not only improves the student task view’s design and usability but also creates a more organized and supportive environment, ultimately enhancing the overall student experience in Expertiza.
Reimplementation
The StudentTaskView.tsx file includes a React component called StudentTask. This component handles the display of the student task view, showing assignment information, deadlines, and interactive options that vary depending on user permissions. It imports essential modules and functions from React along with custom utility files.
- At the top of the page, a bold title welcomes the user with the assignment name, "OSS Project & Documentation." Just below it, a green notification bar provides students with straightforward instructions on their next steps.
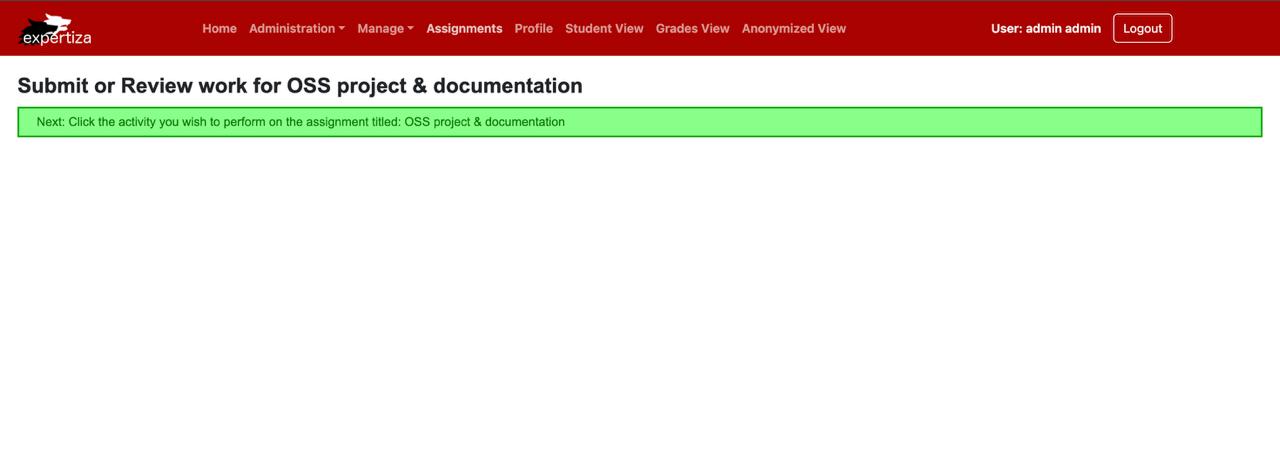
- In the second image, the view expands to show various options available for students to manage their assignments. Links such as "Your Team," "Your Work," "Others' Work," and "Your Scores" are listed, each accompanied by a brief description to clarify available actions. This layout enables students to navigate easily between sections in their assignment workflow, enhancing the platform’s usability.
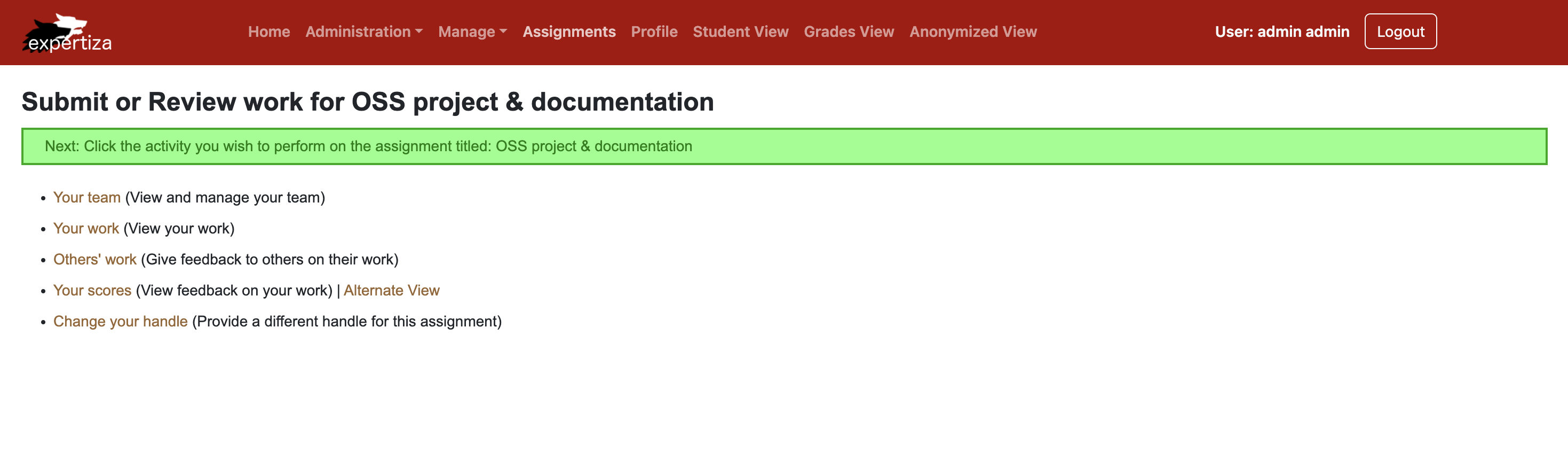
- In this image, alongside the activity navigation links, there's a new option titled "Send Email to Reviewers." This feature allows students to easily connect with their reviewers, streamlining communication. Its strategic placement ensures quick access, making the process more efficient.
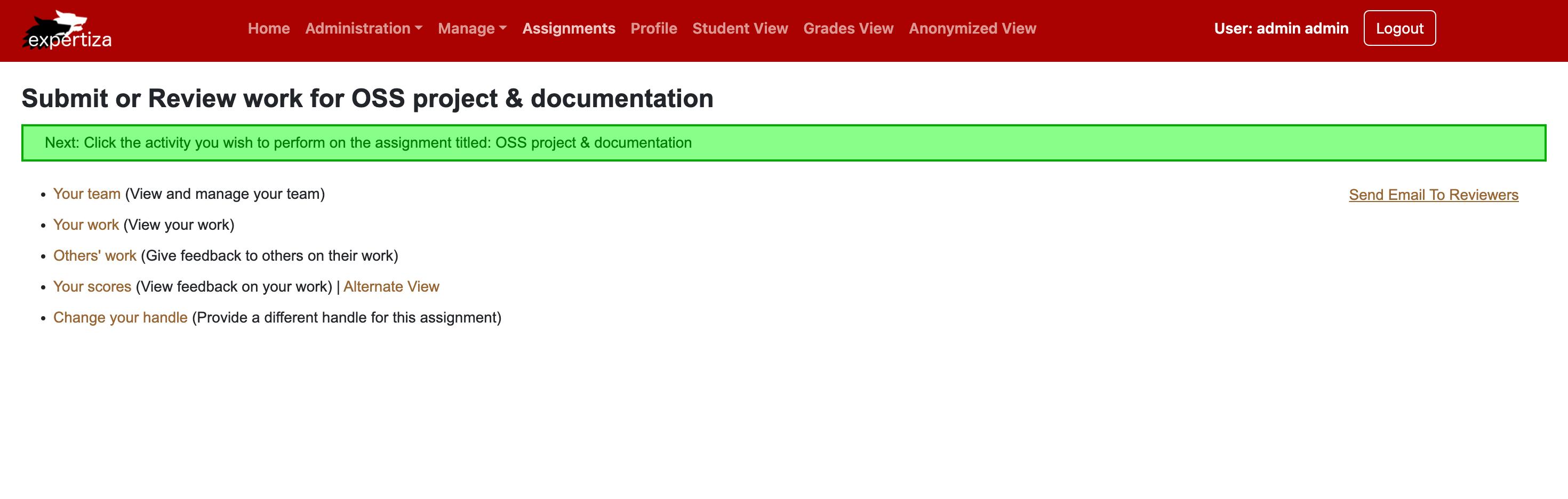
- This shows the same layout but also includes a visual timeline of past deadlines at the bottom. Key dates, like submission and review deadlines, are marked with red dots and labeled for clarity. This timeline feature gives students an easy, visual way to track their progress and stay on top of important deadlines, enhancing the overall user experience.
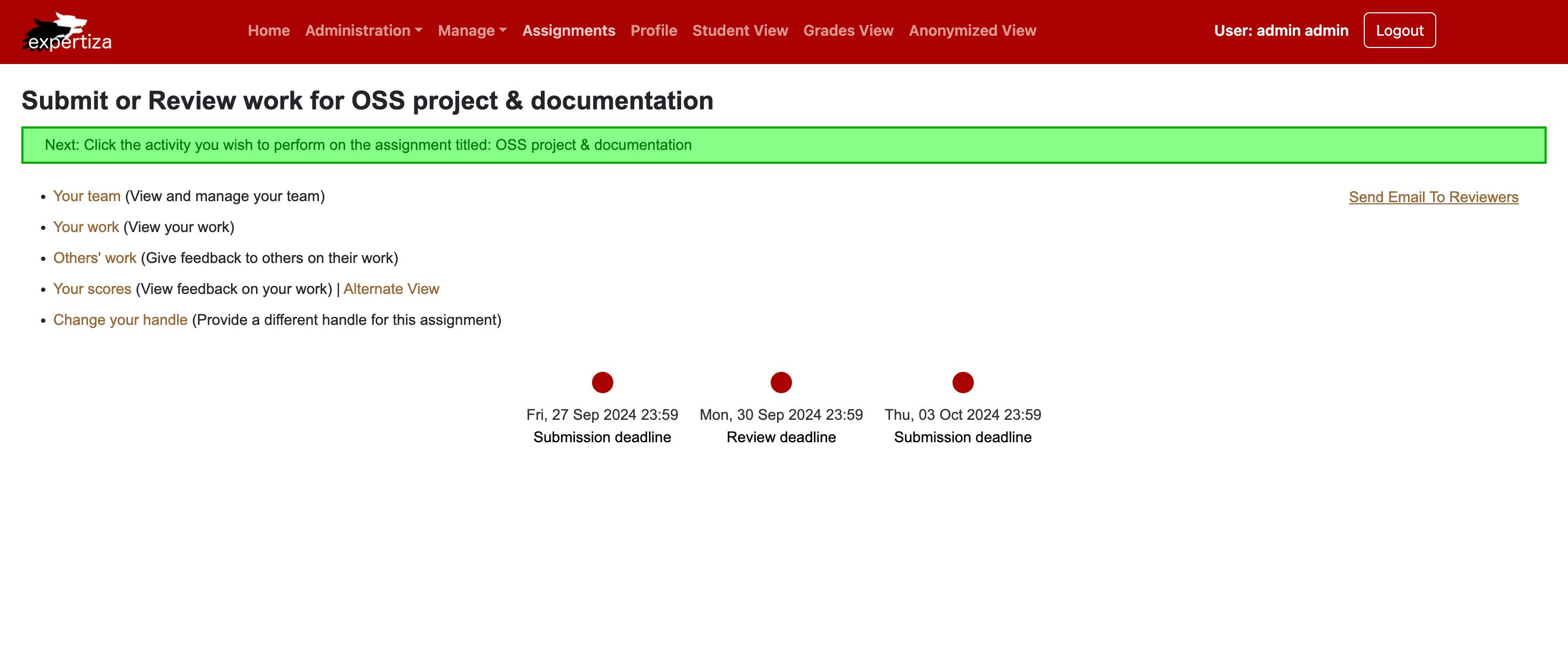
- The timeline includes additional checkpoints showing approaching deadlines for submission and peer review rounds, with each milestone clearly labeled by due date and type, like "Submission Deadline" or "Round 1 Peer Review." This setup lets students easily see their upcoming tasks at a glance.
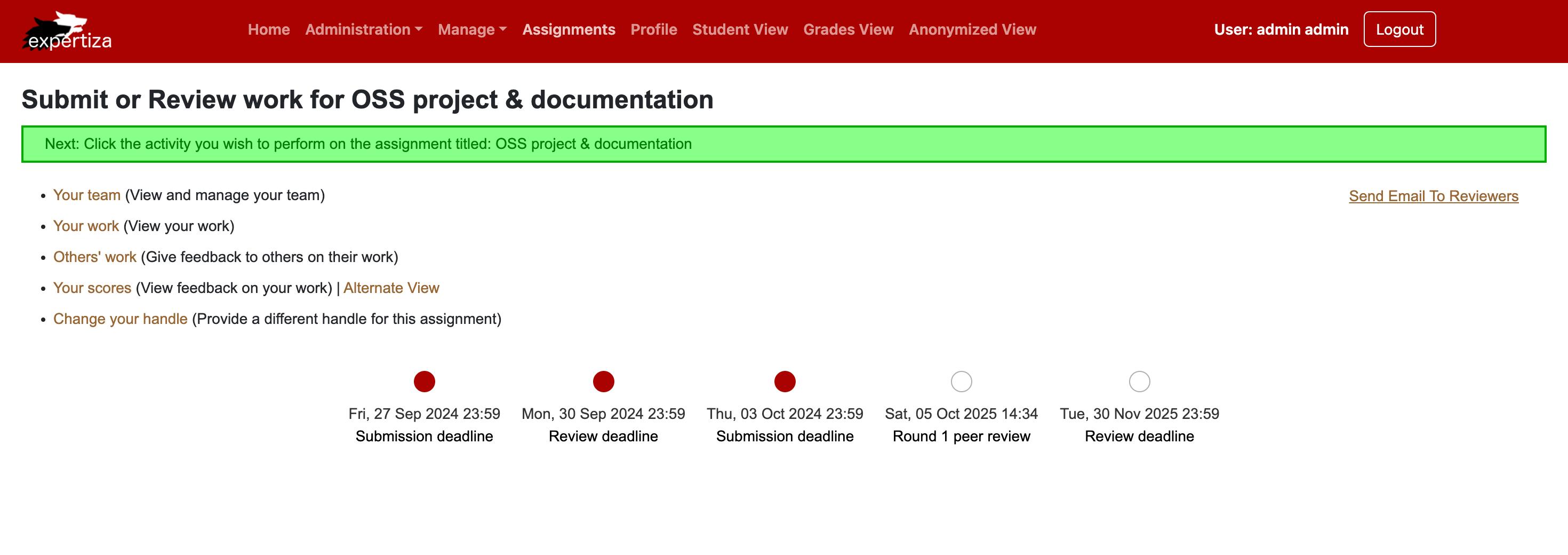
- In this image, the layout includes the footer, i.e., navigation links at the bottom for "Back," "Help," and "Papers on Expertiza," giving students quick access to extra resources. This extended timeline supports assignment management across a full semester or project cycle, improving both clarity and usability.
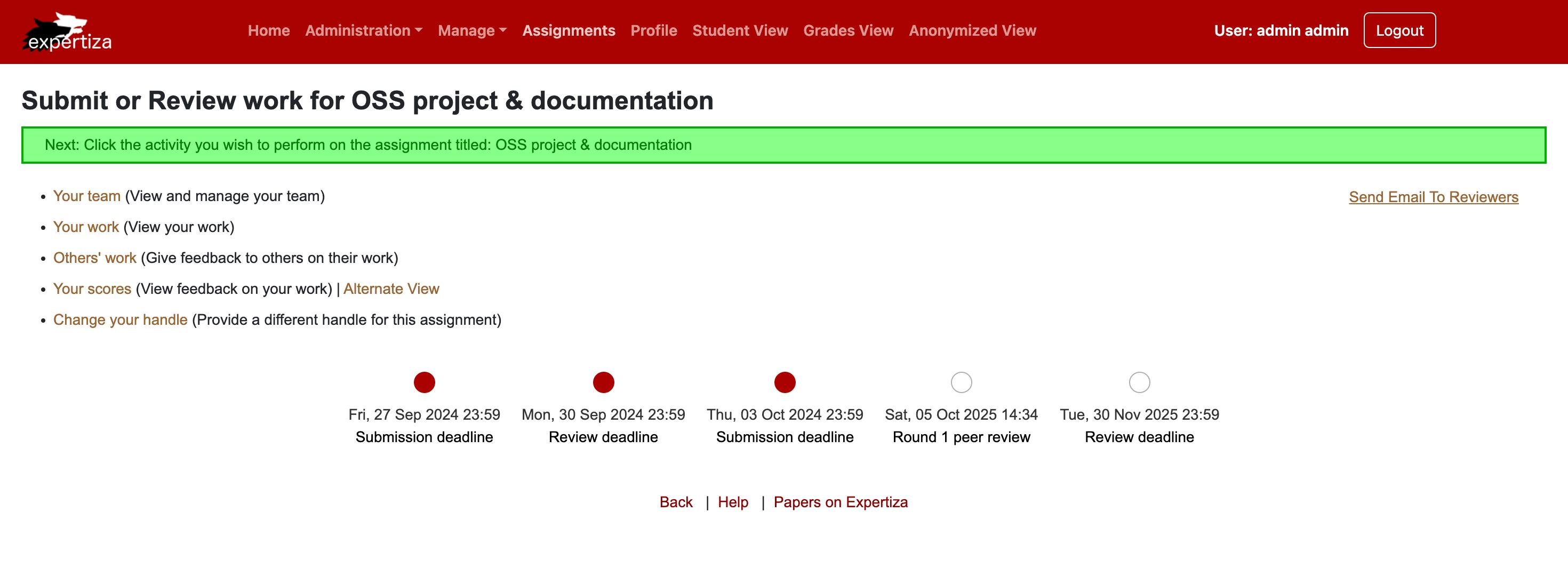
List of files added/updated
- src/App.tsx
- src/pages/StudentTasks/DummyData.json
- src/pages/StudentTasks/StudentTask.css
- src/pages/StudentTasks/StudentTask.tsx
Code Snippets
- DummyData.json :
The DummyData.json file supplies mock data for the StudentTask component, outlining the assignment name and key timeline milestones. The "assignmentName" field holds a string with the assignment title, "OSS project & documentation." The "timeline" field is an array of milestone objects, each featuring a "date" field for the deadline date and time, along with a "label" field indicating its purpose, such as "Submission deadline" or "Round 1 peer review." This data is essential for visualizing assignment milestones within the StudentTask component, providing users with a clear view of important deadlines.
{ "assignmentName": "OSS project & documentation", "timeline": [ { "date": "Fri, 27 Sep 2024 23:59", "label": "Submission deadline" }, { "date": "Mon, 30 Sep 2024 23:59", "label": "Review deadline" }, { "date": "Thu, 03 Oct 2024 23:59", "label": "Submission deadline" }, { "date": "Sat, 15 Nov 2024 14:34", "label": "Round 1 peer review" }, { "date": "Tue, 10 Dec 2024 23:59", "label": "Review deadline" } ] }
- StudentTask.tsx :
The StudentTask.tsx file is a functional React component that serves as the main interface for managing student assignments within the app. Built with TypeScript to improve type safety, it starts by importing essential dependencies, including React, relevant CSS for styling, and a DummyData.json file containing sample assignment and timeline data. Key interfaces, such as TimelineItem, are defined to enforce a consistent structure and validate the data types used.
Within the component's logic, it retrieves the assignment name and timeline details, sets the current date, and includes a function called getStatusClass that determines the status of each timeline item based on its deadline. Additionally, it provides an email feature that lets users send reminders to reviewers. The render method outputs a well-structured layout with a header, user instructions, an email button, a list of assignment-related actions, and an organized timeline showing each deadline alongside its status. The footer offers navigation links for further support. In essence, StudentTask.tsx enhances user experience by integrating interactivity with a clear presentation, allowing students to effectively keep track of their assignments and deadlines.
import React from 'react'; import './StudentTask.css'; import dummyData from './DummyData.json'; interface StudentTaskProps { assignmentName: string; } interface TimelineItem { date: string; label: string; } const StudentTask: React.FC = () => { const assignmentName = dummyData.assignmentName; const items: TimelineItem[] = dummyData.timeline; const currentDate = new Date(); const getStatusClass = (date: string) => { const deadline = new Date(date); if (currentDate > deadline) return 'past-deadline'; else return 'approaching-deadline'; }; const handleSendEmail = () => { window.location.href = "mailto:reviewers@example.com?subject=Review Reminder&body=Please review the submission for Program 2."; }; return ( <div className="student-task-container"> <h1>Submit or Review work for {assignmentName}</h1> <div className="instruction"> Next: Click the activity you wish to perform on the assignment titled: {assignmentName} </div> <div style={{ position: 'relative'}}> <button onClick={handleSendEmail} style={{ position: 'absolute', right: '20px', backgroundColor: 'transparent', color: '#986633', border: 'none', cursor: 'pointer', textDecoration: 'underline' }} > Send Email To Reviewers </button> </div> <ul className="actions"> <li><a href="#">Your team</a> (View and manage your team)</li> <li><a href="#">Your work</a> (View your work)</li> <li><a href="#">Others' work</a> (Give feedback to others on their work)</li> <li> <a href="#">Your scores</a> (View feedback on your work) <span> | </span> <a href="#">Alternate View</a> </li> <li><a href="#">Change your handle</a> (Provide a different handle for this assignment)</li> </ul> <div className="timeline"> {items.map((item, index) => ( <div key={index} className={`timeline-item ${getStatusClass(item.date)}`}> <li> <div className="timeline-circle"></div> <span className="timeline-date">{item.date}</span> <span className="timeline-label">{item.label}</span> </li> </div> ))} </div> <div className="footer"> <a href="javascript:window.history.back()">Back</a> | <a href="http://wiki.expertiza.ncsu.edu/index.php/Expertiza_documentation" target="_blank">Help</a> | <a href="http://research.csc.ncsu.edu/efg/expertiza/papers" target="_blank">Papers on Expertiza</a> </div> </div> ); }; export default StudentTask;
- App.tsx :
In App.tsx, we added routing code to integrate the StudentTask component, enabling users to navigate directly to the student task interface through a specific route. By importing StudentTask from ./pages/StudentTasks/StudentTask, the component becomes accessible within the main application.
The routing setup defines an object that specifies the path and its associated component:
import StudentTask from "./pages/StudentTasks/StudentTask"; { path: "/student_tasks", element: <StudentTask /> }
This code snippet creates a new route at /student_tasks, which renders the StudentTask component whenever that path is accessed. This setup provides smooth navigation to the student tasks page, where students can easily view and manage their assignments, actions, and timelines directly from this route.
Usage of SOLID Principles
- Single Responsibility Principle :
The StudentTask.tsx component follows the Single Responsibility Principle by concentrating on one main task: managing the interface for viewing and interacting with student assignment tasks. Each function within the component, such as getStatusClass or handleSendEmail, serves a specific purpose, all working together to present assignment-related information and actions seamlessly.
Here’s a code snippet illustrating SRP:
const getStatusClass = (date: string) => { const deadline = new Date(date); if (currentDate > deadline) return 'past-deadline'; else return 'approaching-deadline'; }; const handleSendEmail = () => { window.location.href = "mailto:reviewers@example.com?subject=Review Reminder&body=Please review the submission for Program 2."; };
Each function here has one specific task, whether it’s determining the status class of a deadline or handling an email action, ensuring the component remains modular and focused.
- Interface Segregation Principle (ISP):
The Interface Segregation Principle suggests using smaller, more focused interfaces rather than large, general ones. In StudentTask.tsx, this principle is partially implemented by defining distinct interfaces like StudentTaskProps and TimelineItem, each with minimal, specific responsibilities. This approach makes each type easier to manage and use where needed without unnecessary complexity.
Here’s a code snippet illustrating isp:
interface StudentTaskProps { assignmentName: string; } interface TimelineItem { date: string; label: string; }
Keeping interfaces small and focused allows us to change or extend each one without impacting unrelated parts of the application.
Improvements
In our reimplementation, we've significantly improved the user interface, prioritizing clarity, usability, and an enhanced user experience. By tackling the main challenges found in the original Expertiza website, we set out to develop a design that is both more intuitive and visually appealing.
Timeline Alignment
In our redesigned interface, we’ve significantly improved the alignment of timeline deadlines to boost the user experience. By arranging deadlines in a clear, sequential format, users can effortlessly follow the flow of submission and review stages, lowering the chance of missing any crucial dates.
This structured layout allows users to distinguish between different phases at a glance, making it clear when specific actions need to be taken. Unlike the original Expertiza site, where deadlines might feel cluttered and challenging to track, our design displays each milestone within a cohesive, easy-to-navigate timeline.
Aligning the timeline properly results in a cleaner interface with reduced mental effort for the user. They can quickly scan through and understand the chronological order without struggling through scattered elements. Each deadline is visibly linked to its relevant tasks, which strengthens overall comprehension.
Additionally, this organized view aids users in planning their activities more efficiently. Seeing how deadlines are interconnected at a glance enables them to prioritize and manage their time more effectively. This streamlined setup simplifies deadline management, fostering proactive interaction with the platform and offering a more intuitive experience compared to the original Expertiza layout.
In our redesign, the navigation links have been strategically improved to enhance usability. By grouping the "Back," "Help," and "Papers on Expertiza" links at the bottom of the interface, we’ve created a more intuitive navigation experience. This setup streamlines access to essential resources, which simplifies the overall workflow.
With these links consolidated in one area, users can easily find what they need without being distracted by scattered options. This design enables users to move back in their tasks, seek help, or access relevant papers quickly and easily—all in one click. Ultimately, this organized layout fosters a smoother user experience, minimizing frustration and encouraging more productive engagement with the platform.
Technologies Used
In this project, we chose React.js and TypeScript to create a strong, scalable user interface.
- React.js a widely used JavaScript library, is ideal for building interactive, single-page applications. Its component-based structure lets us develop reusable UI elements, which streamlines both the creation and upkeep of the platform. React's state management features also ensure a smooth user experience as people move through different areas of the application.
- TypeScript, an extension of JavaScript, introduces static typing to the code, which boosts development efficiency and reduces potential errors. By catching issues during compile time instead of runtime, TypeScript helps us build a more reliable and robust application. Its type-checking system enhances code clarity and readability, simplifying teamwork and future maintenance.
Using React.js and TypeScript together has allowed us to create an application that is user-friendly, efficient, and well-suited to our users' needs.
Scope for future improvement
Database Integration
This change will allow dynamic data retrieval, ensuring the application always shows the latest information. Also, some tables might require adjustments—such as adding or removing columns—to keep the structure in sync with any updates or changes.
Notification System Integration
Implementing real-time notifications would enable students to receive instant alerts on upcoming deadlines, new peer review requests, and task updates, keeping them proactively informed. Notifications could appear within the app, as well as via optional email or SMS alerts, ensuring critical information is accessible even outside the platform. This feature would reduce the need for students to constantly check for updates, streamlining task management and supporting timely completion of assignments.
Search and Filter Functionality
Adding search and filter features allows users to quickly find specific tables or records in the database. User-friendly tools like keyword search, criteria-based filters, and instant suggestions enhance the experience, while persistent filters and robust error handling support smooth navigation and efficient access to relevant information.
Testing
Testing was initially outside the scope of our project, as the main focus was frontend development. However, validating the functionality and user experience of the interface remains essential. Therefore, manual testing procedures will be implemented to confirm the correct operation of UI components. These procedures include:
- Ensuring that timeline items display with the correct status indicators based on deadlines.
- Ensuring that the status colors (e.g., past-deadline vs. approaching-deadline) update accurately based on the current date, clearly indicating the urgency of each deadline.
- Verifying all added buttons and links to ensure they function as expected.
Peer Review Information
For those who wish to access the Expertiza application linked to this assignment, the login details are provided below:
Admin credentials: Username -> admin, Password -> password123
The expertiza application can be accessed by running the front end and back end simultaneously on your local laptop. The instructions on how to run the application is on the respective repositories.
Github repository
Here are the links to the front-end and back-end reimplementations:
Front end:
github [1]
These changes can be viewed in this pull request [2].
Team
Mentor:
Kashika Malick (kmalick@ncsu.edu)
Students:
Pankhuri Priya (ppriya2@ncsu.edu)
Sreeja Nukarapu (snukara@ncsu.edu)
Navyatej Tummala (ntummal3@ncsu.edu)