CSC/ECE 517 Spring 2025 - E2534 UI for Assign Reviewers
Introduction
This project will improve the UI functionality to assign reviewers to assignments. This interface needs to be streamlined and only expose a few key fields, while allowing for the simple assignment and deletion of reviewers from projects. This project will focus on implementing this solution within the front end reimplementation environment using Typescript and React.
Specific Objectives
- Create a 3 column layout with "Assignment", "Contributor", and "Reviewers" columns
- Add action buttons to add reviewers to an assignment, delete outstanding reviewers
- Add buttons to unsubmit or delete each review in the review column
- Allow for the addition of reviewers by their username
Proposed Changes
Affected Repositories
- The group will be modifying and updating the expertiza/reimplementation-frontend repository for the front end project
- Changes will be made with React and Typescript
Create a 3 column layout with "Assignment", "Contributor", and "Reviewers" columns
Problem
In Expertiza, managing reviewer assignments is currently fragmented across different interfaces. The existing “Add Reviewer” UI (as shown in the screenshot) allows an instructor to assign a reviewer by entering a user login manually. While this supports basic functionality, it lacks a unified view and efficient controls for large-scale assignment management.
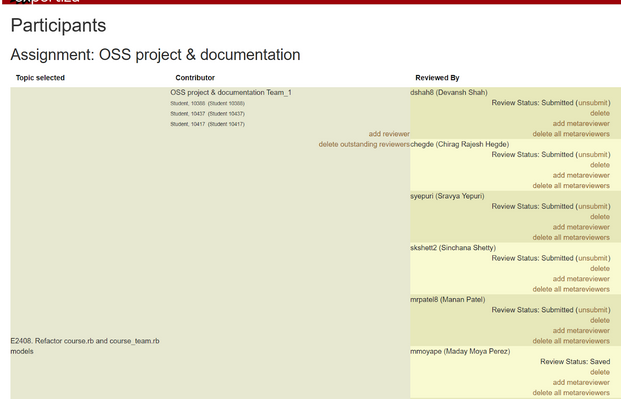
Specifically, the problems include:
Without a structured layout, instructors cannot efficiently organize or control the review process, especially in larger courses or group projects.
Solution
We propose the implementation of a 3-column table layout that clearly organizes the review mapping with integrated reviewer management controls. This layout improves visibility, reduces friction in reviewer assignment, and offers direct interaction with review statuses.
Add action buttons to add reviewers to an assignment, delete outstanding reviewers
Problem
There needs to be a reviewer management system in the form of action buttons. The two possible options include adding a reviewer to the selected topic, and deleting any outstanding reviewers from the topic that have already been assigned. Without this functionality, the users would not be able to manually add or remove reviewers from a particular topic when necessary.

Solution
The solution will focus on reimplementing the UI so that it is compatible with both the front end and back end implementations and is therefore two-pronged. One one hand, the front end/UI needs to be re-implemented, while the backend also needs to be reimplemented for API calls while maintaining the same functionality. The primary design goal will be to maintain a RESTful API structure to ensure the frontend-backend integration is seamless.
The participant.rb
file holds the backend code, while the file that stores the UI layout from the above screenshot is a page called reviews_summary
.
participant.rb
This solution will need to access multiple participants to render a view for a given topic. Key information like participant name and username will be needed. The key changes to make this file API compatible while maintaining the desired functionality includes
- Use ActiveModel::Serializers to handle JSON Responses from the front end.
- Ensure the Model Supports CRUD Operations via a RESTful API.
- This means the model will need to be correctly connected to a controller that follows RESTful conventions (index, show, create, update, destroy) and returns JSON responses.
- Handle Validations and Errors Gracefully
- Ensure that the model returns structured error responses and generates HTTP response codes that can be used to create efficient and thorough test cases.
Reviews_Summary
This page will need to render the 3 column layout for displaying topics, reviewers, and reviews. It needs to support the desired interactivity to delete and unsubmit reviews, render topics, and show information about the assignment.
Add buttons to unsubmit or delete each review in the review column
Problem
Instructors need to be able to unsubmit or delete reviews that were submitted in error or were otherwise deficient. Managing this on a per-assignment basis is helpful given the usual linear flow of assignment, submission, and review.

Solution
The button should not require supplemental interaction from the user, and should indicate the resulting status of the assignment and reviews once pressed. We may initially need to stub the relevant backend responses_controller.rb
API endpoints to destroy or unsubmit a review for testing. This functionality can be included with the reviews_summary
page mentioned previously
Allow for the addition of reviewers by their username
Problem
Instructors and TAs would like to manually assign reviewers to assignments, and would like to do this in a streamlined fashion. There needs to be functionality to add a user by entering their username, immediately associating them as a reviewer with that assignment.
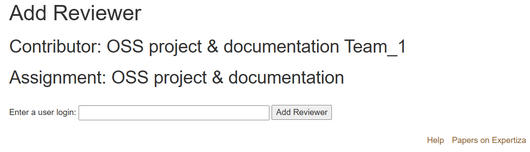
Solution
Create a separate page linked from the main 3 column interface, there should be a page that supports the input of a username and then assigns that user to the review. The backend API may need to be stubbed at this point to sufficiently test this page, as the full functionality for assigning reviewers is not present in the reimplementation project. This page will need to be included in a separate Add_Reviewer
page. Additionally, this page will need to interact with the response.rb
model to create a new review and notify the user that they have been added as a reviewer.
response.rb & responses_controller.rb
The page will need to interact with the RESTful API endpoints for Response to create a new response and assign it to a given reviewer. Logic may need to be added or stubbed to enable this functionality within the current reimplementation environment.
Add_Reviewer
This page will need to render a page with a text box to input the username of the desired reviewer. The user should then be able to assign that reviewer and formally create the desired instance of the response model once they click 'Add Reviewer'. Appropriate validations should be supported to only assign a user that already exists. Additionally, the user should be able to go back to the 3 column view previously discussed once they have completed adding a reviewer.