CSC/ECE 517 Spring 2025 - E2511. Reimplement participants controller.rb
About Expertiza
Expertiza is an open source project based on Ruby on Rails framework. Expertiza allows the instructor to create new assignments and customize new or existing assignments. It also allows the instructor to create a list of topics the students can sign up for. Students can form teams in Expertiza to work on various projects and assignments. Students can also peer review other students' submissions. Expertiza supports submission across various document types, including the URLs and wiki pages.
Introduction
This project aims to reimplement the participants_controller.rb
and participants_helper.rb
in the new Expertiza system. The participants_controller.rb
manages participants within assignments, and this reimplementation focuses on streamlining its methods, eliminating redundancy, and enhancing code readability and maintainability.
Requirements
- Implement
participants_controller.rb
Methods: Fully implement each controller method with the same functionality as the current controller. - Import from
participants_helper.rb
: Integrate relevant helper methods to streamline functionality and remove redundancy. - API Creation: Expose API endpoints for all necessary methods to enable seamless access and integration from the frontend. Ensure that each endpoint follows best practices for RESTful API design, including proper error handling and secure data access.
- Testing with rswag: Create test cases for all methods, focusing on edge cases, and generate rswag documentation.
- Swagger UI Video Documentation: Record a video walkthrough of API endpoints in Swagger UI, showcasing each method’s functionality.
Tasks to be Completed
- Implement participants_controller.rb Methods: Develop each method with the same functionality as the existing controller.
- Refactor and Integrate Helper Methods: Import relevant methods from participants_helper.rb to improve efficiency and eliminate code duplication.
- API Development: Expose RESTful API endpoints for necessary operations, ensuring proper error handling and secure data access.
- Testing with Rswag: Implement comprehensive test cases, including edge cases, and generate Rswag documentation.
- Swagger UI Documentation: Create a video walkthrough demonstrating API endpoints and their functionalities in Swagger UI.
- Make sure to write intuitive test cases for this controller using RSpec
Reimplement participants_controller.rb
Previous participants_controller.rb Methods / API Calls
# | Method | Endpoint | Description |
---|---|---|---|
1 | user_index | GET /participants/user/:user_id
|
Return a list of participants for a given user |
2 | assignment_index | GET /participants/assignment/:assignment_id
|
Return a list of participants for a given assignment |
3 | show | GET /participants/:id
|
Return a specified participant |
4 | add | POST /participants/:authorization
|
Assign the specified authorization to the participant and add them to an assignment |
5 | update_authorization | PATCH /participants/:id/:authorization
|
Update the specified participant to the specified authorization |
6 | destroy | DELETE /participants/:id
|
Delete a participant |
7 | participant_params | - | Permitted parameters for creating a Participant object |
participants_controller.rb Methods / API Calls
# | HTTP Method | Endpoint | Method Name | Description |
---|---|---|---|---|
1 | GET | /api/v1/participants | index | List all participants |
2 | POST | /api/v1/participants | create | Create a new participant |
3 | GET | /api/v1/participants/:id | show | Retrieve a participant by ID |
4 | PUT | /api/v1/participants/:id | update | Update a participant |
5 | DELETE | /api/v1/participants/:id | destroy | Delete a participant |
6 | GET | /api/v1/participants/user/:user_id | user_index | Get participants by user ID |
7 | GET | /api/v1/participants/assignment/:assignment_id | assignment_index | Get participants by assignment ID |
8 | POST | /api/v1/participants/:id/:authorization | add | Add a participant with authorization |
9 | PATCH | /api/v1/participants/:id/:authorization | update_authorization | Update participant authorization |
Removed Methods from participants_controller.rb
The following methods were removed as part of the reimplementation:
action_allowed?
– No impact on functionality.controller_locale
– No impact on functionality.inherit
– Redundant due to lack of direct relation between Course and Participant.bequeath_all
– Same reason as above.change_handle
– `User` model already has `handle`, so it's redundant.delete
– Replaced by `remove_participant` inassignments_controller.rb
.view_copyright_grants
– Old implementation was incorrect.get_user_info
– Covered by `belongs_to` relation in the `Participant` model.get_signup_topics_for_assignment
– Now handled by `sign_up_topics_controller.rb`.
Implementation Summary
Api::V1::ParticipantsController
was restructured to:
- Follow RESTful conventions (`index`, `show`, `create`, `update`, `destroy`)
- Add custom endpoints for `user_index`, `assignment_index`, and `update_authorization`
- Leverage strong parameters and `before_action` filters
- Integrate logic from `participants_helper.rb` (like `participant_permissions`)
- Improve error handling using status codes and JSON messages
participants_controller.rb Methods
The following custom methods were added to handle participant creation with role-based authorization and to update existing participant permissions based on a new authorization.
Method: add
#adding a participant with authorization def add assignment = Assignment.find(params[:id]) user = User.find_or_create_by(user_params) # Now you can safely use `user` below handle = "#{user.name.parameterize}-#{SecureRandom.hex(2)}" permissions = participant_permissions(params[:authorization]) participant = assignment.participants.create!( user: user, handle: handle, can_submit: permissions[:can_submit], can_review: permissions[:can_review], can_take_quiz: permissions[:can_take_quiz], can_mentor: permissions[:can_mentor] ) render json: participant, status: :created end
Method: update_authorization
def update_authorization participant = Participant.find(params[:id]) permissions = participant_permissions(params[:authorization]) participant.update!( can_submit: permissions[:can_submit], can_review: permissions[:can_review], can_take_quiz: permissions[:can_take_quiz], can_mentor: permissions[:can_mentor] ) render json: participant, status: :ok end
participants_helper.rb Methods
The ParticipantsHelper
module contains utility methods used to assign roles and permissions to a Participant. Compared to the original implementation, this version has been heavily simplified and cleaned up.
Only the following method is included in the reimplementation:
retrieve_participant_permissions
– Returns a hash of permission flags based on the participant’s assigned role.
This method sets default permissions (submit, review, quiz, mentor) and overrides them based on the role passed in. The supported roles are: *reader
*reviewer
*submitter
*mentor
```ruby def retrieve_participant_permissions(authorization) default_permissions = { can_submit: true, can_review: true, can_take_quiz: true, can_mentor: false }
permissions_map = { 'reader' => { can_submit: false }, 'reviewer' => { can_submit: false, can_take_quiz: false }, 'submitter' => { can_review: false, can_take_quiz: false }, 'mentor' => { can_mentor: true } }
default_permissions.merge(permissions_map[authorization]) end
SOLID Principles in participants_controller.rb
The reimplementation of participants_controller.rb
follows several key SOLID design principles, promoting better structure, maintainability, and testability of the code.
Single Responsibility Principle (SRP)
- A class should have only one reason to change.*
The controller now only manages routing and API logic. Role-based permission logic is abstracted into a helper method (or strategy), and user creation is encapsulated using strong params.
Examples:
add
method handles only participant creation.participant_permissions
cleanly maps roles to permission flags.- Deprecated methods like
inherit
andbequeath_all
were removed to reduce clutter.
Open/Closed Principle (OCP)
- Software should be open for extension, but closed for modification.*
The controller supports new roles or permission logic without modifying existing methods. By using participant_permissions
or a Strategy Pattern, the controller can support additional behaviors without structural changes.
Example:
- You can add new roles (e.g., Observer, Facilitator) by extending the permission logic externally.
Role-Based Strategy Pattern
As part of this reimplementation, a Strategy Design Pattern was introduced to streamline role-based permission handling, replacing the large conditional structure previously present in participants_helper.rb
.
This pattern allows each role to encapsulate its own permission logic, enabling easier testing, extension, and adherence to SOLID principles.
Structure
The strategy pattern consists of the following components located under the Strategies/roles
directory:
mentor_strategy.rb
reviewer_strategy.rb
student_strategy.rb
teacher_assistant_strategy.rb
role_strategy.rb
– Base module defining required methodsrole_context.rb
– Context class that uses strategies dynamically
How it Works
Each role strategy implements the get_permissions
method to return a role-specific permission hash, for example:
- Example from MentorStrategy
def get_permissions
{ can_submit: false, can_review: false, can_take_quiz: false, can_mentor: true }
end
API Testing with RSpec and Swagger
As part of ensuring the stability and correctness of the newly reimplemented ParticipantsController, comprehensive API tests were added using RSpec and rswag (Swagger integration). These tests validate both the happy path and edge cases for participant-related endpoints.
Structure
The tests are defined in the following file: spec/requests/api/v1/participants_spec.rb
The structure includes the following tested endpoints:
Endpoint: GET /api/v1/participants/user/:user_id
Description: Returns all participants associated with a given user.
Test Coverage:
- 200 OK – Returns list of participants
- 404 Not Found – User ID does not exist
- 401 Unauthorized – Invalid or missing token
Assertions:
- Response is an array of participant objects.
- Each object contains correct user_id and assignment_id.
Endpoint: GET /api/v1/participants/assignment/:assignment_id
Description: Returns all participants associated with a specific assignment.
Test Coverage:
- 200 OK – Returns matching participants
- 404 Not Found – Assignment ID does not exist
- 401 Unauthorized – Token is invalid or missing
Assertions:
- Response contains participants with matching assignment_id.
Endpoint: GET /api/v1/participants/:id
Description: Retrieves a single participant by ID.
Test Coverage:
- 200 OK – Returns the correct participant
- 404 Not Found – Invalid participant ID
- 401 Unauthorized – No or invalid token
Assertions:
- JSON contains user_id and assignment_id matching test data.
Endpoint: DELETE /api/v1/participants/:id
Description: Deletes a participant by ID.
Test Coverage:
- 204 No Content – Participant deleted successfully
- 404 Not Found – Deleting a non-existent participant
- 401 Unauthorized – Missing or invalid auth token
Assertions:
- Response body is empty on successful deletion.
Authentication
Before each test, an admin login is performed, and a valid JWT token is captured and passed in the Authorization header for secure access. before(:all) do
post '/login', params: { user_name: 'admin2@example.com', password: 'password123' } @token = JSON.parse(response.body)['token']
The token is used like so:
let(:valid_headers) { { 'Authorization' => "Bearer #{@token}" } }
Error Handling Covered
Each endpoint is tested for:
- 200 OK – Valid request returns expected data.
- 404 Not Found – Invalid user_id, assignment_id, or participant_id.
- 401 Unauthorized – Missing or invalid JWT token.
Benefits
- Confidence in refactoring: Ensures controller changes don’t break expected behavior.
- Live API Docs: Swagger UI automatically reflects documented test cases.
- Security: Token-based auth is enforced and tested for all protected endpoints.
Sample of the Swagger UI API working
APIs of all the participants_controller.rb
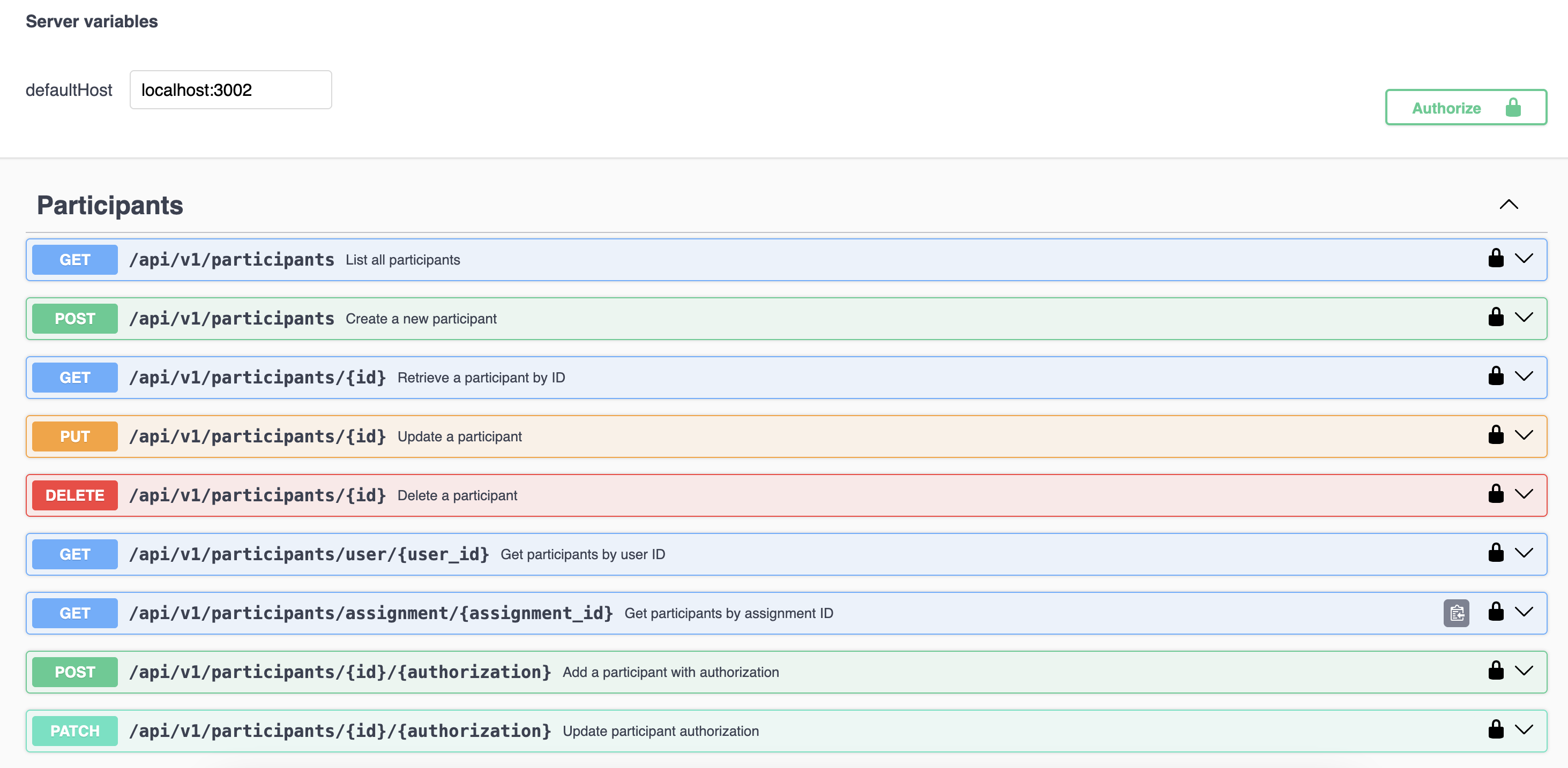
Updating a user by specific id
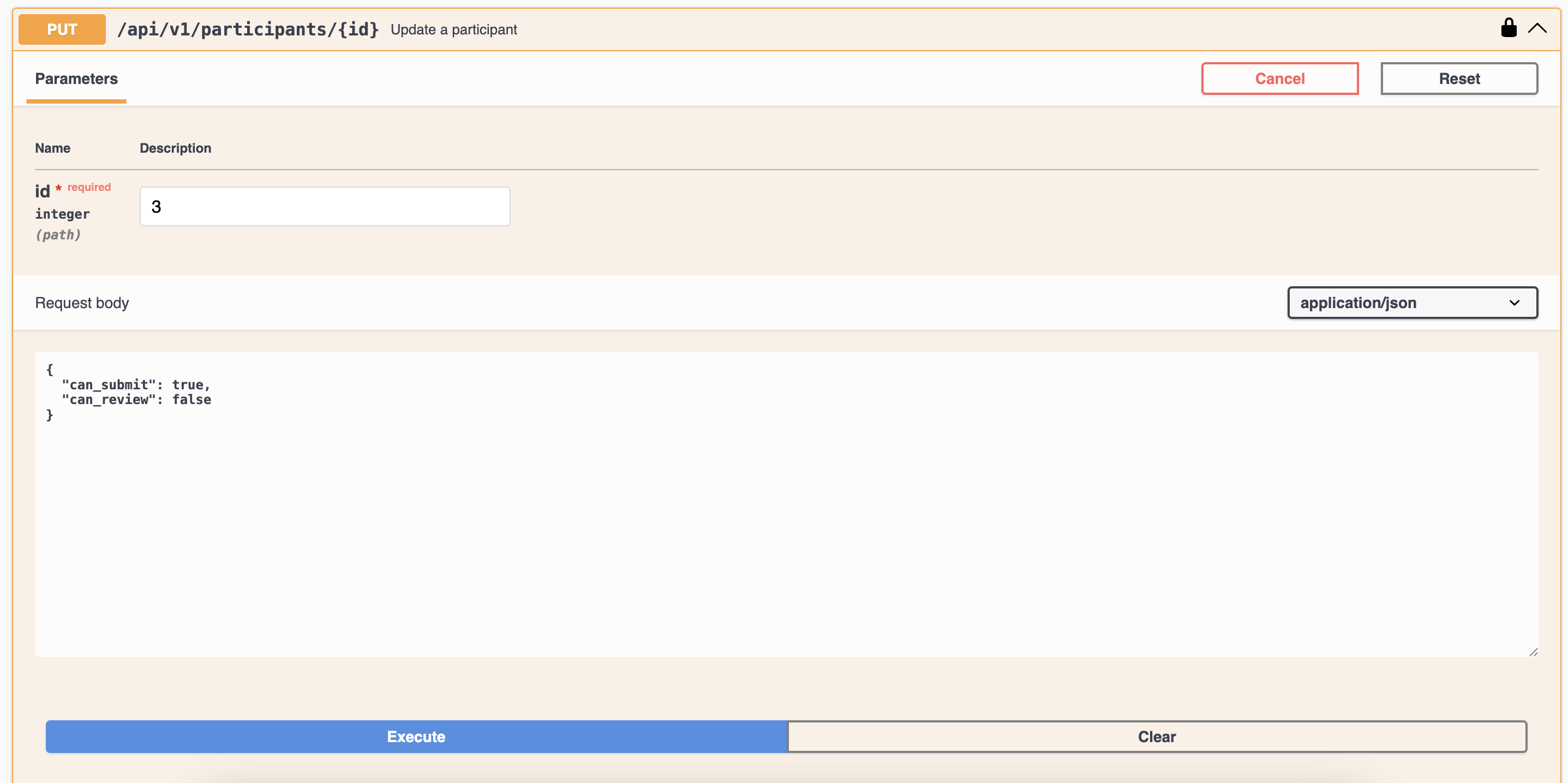
Request successful with User updated
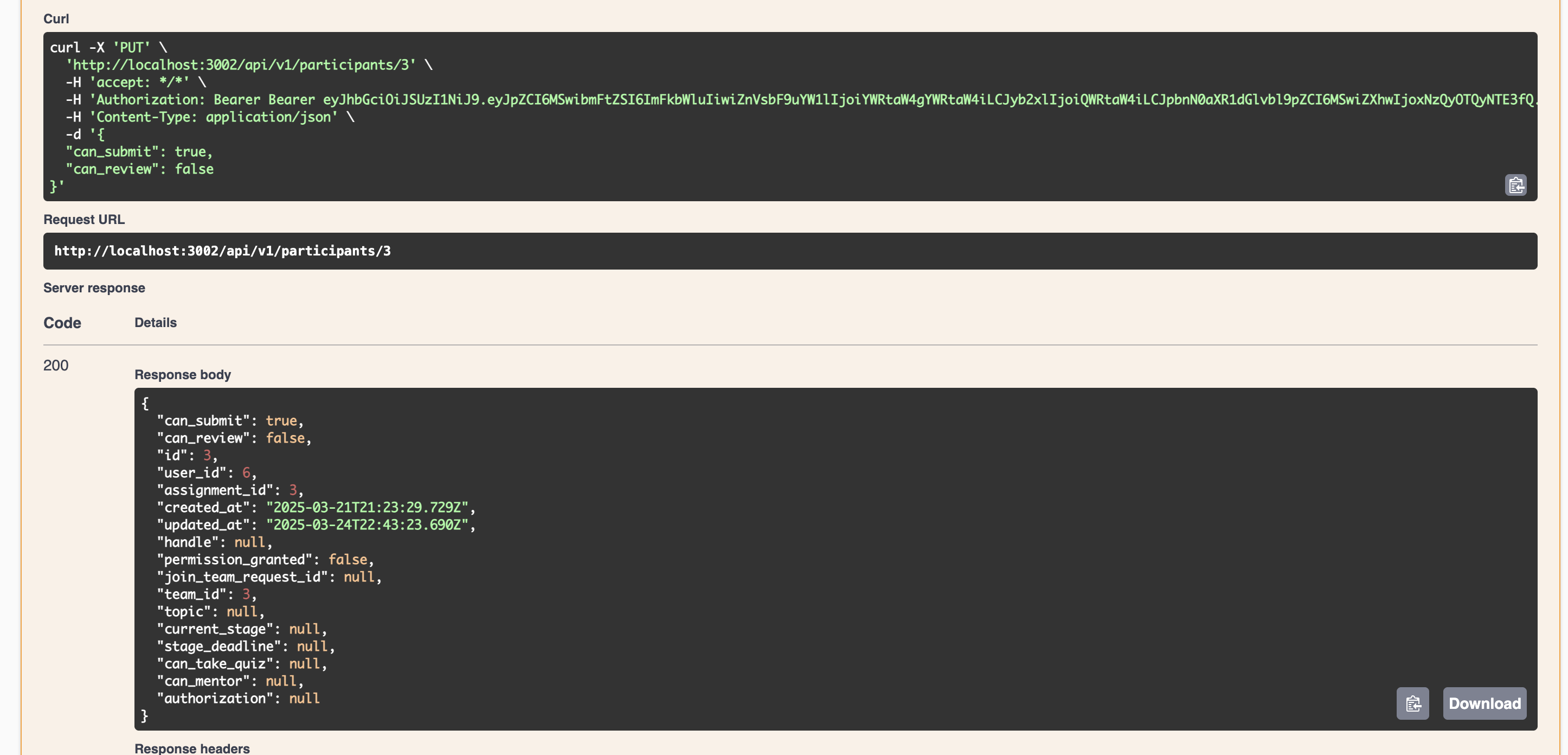
Resources
- Expertiza GitHub Repository
- Rails Routing Guide
- Github Repository
- Github Project Board
- Video Link
- Github Pull Request
- VCL Hosting Swagger
Team Members
- Vansh Dodiya (vkdodiya@ncsu.edu)
- Brian Huynh (bhhuynh@ncsu.edu)
- Akhil Adusumilli (aadusum@ncsu.edu)
Mentor
- Aniruddha Rajnekar