CSC/ECE 517 Spring 2025 - E2504. Mentor-meeting management
Background
Previously, mentors had to be manually assigned by reviewing team formations, a process that required frequent monitoring and manual intervention. The implementation of automatic mentor assignment significantly reduced this workload.
Project Overview
The mentor assignment system was introduced in 2023 to streamline the process of assigning mentors to teams within Expertiza, an open-source course management platform designed for collaborative learning in large Object-Oriented Programming assignments. The primary focus of this project is to enhance the mentor management system. Within Expertiza, mentors can be assigned to teams either manually or automatically. Automatic assignment is triggered when a team reaches more than 50% of its capacity.
Challenges
- While the system successfully assigns mentors automatically, there is no functionality to notify teams and mentors via email when an assignment is made
- This feature needs to be implemented to ensure clear communication.
- The only available team listing is on the "Create Teams" page, where team members are displayed on separate lines, and mentors appear in an arbitrary position within the team structure.
- Improvements are needed to enhance clarity and usability in mentor assignment tracking.
Tasks
Email Notifications
Create a way that notifies participants and mentors, by email, of when they have been assigned to a team.
Mentor Meeting Management
Dr. Gehringer expressed to us that the current view of mentor meetings took up too much space and proposed to us a mockup of how he would like the view to look, which can be seen below.

Backend and Controller Updates
Implement a new controller to handle Create, Read, Update, Delete (CRUD) operations for mentor meetings. Update models to manage mentor meetings and trigger notifications.
Refactor Existing Codebase
Streamline logic for adding users/mentors and sending notifications. Refactor view code to improve readability and reusability.
Testing and Validation
Ensure all features, including notifications and meeting management, are thoroughly tested.
Implementation
Email Notifications
The Email Notification methods experienced a change to support SOLID Principles. Before, the code was not supporting the Single-Responsibility Principle, but now we have separated the parts of the 'add_member' method apart of the Team model. The current switch allows for an easier readability and understanding of code, while also following the SOLID Principles.
Mentor Meeting Management
The teams table for mentor meeting management was significantly enhanced with dynamic column manipulation, allowing users to add and remove meeting columns while preventing conflicts with scheduled meetings. Individual meeting deletion and improved reliability were implemented, along with a refactored meeting date display and input for better user experience. The table structure was optimized, incorporating editable meeting fields with a save feature, dynamic updates based on filter selections, and an actions column. To streamline column management, dropdowns were replaced with header buttons, a maximum of five meeting columns was enforced, and dynamic rendering was implemented based on participant data. The mentor logic and date column naming were corrected for clarity, and a destroy route for team meetings was added, ensuring a more efficient and user-friendly team management experience.
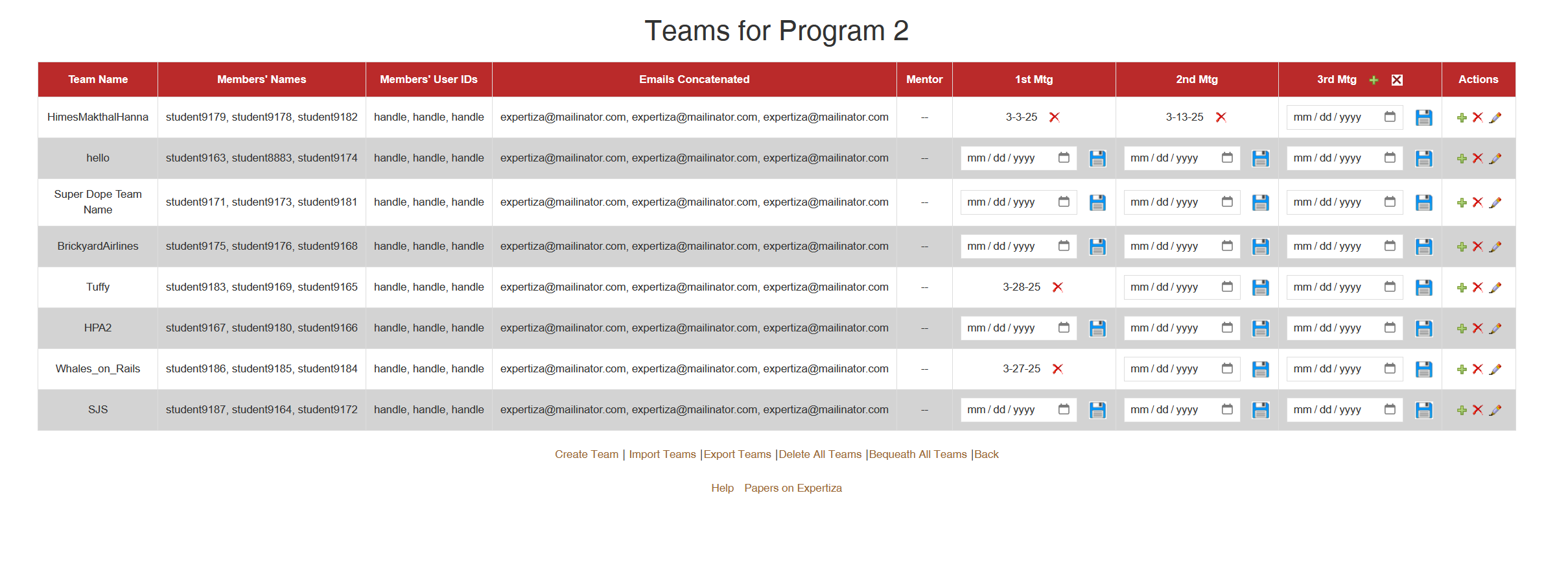
Backend and Controller Updates
The backend for mentor meeting management underwent significant restructuring to improve efficiency and maintainability. A dedicated destroy route for team meetings was implemented, along with a revised deletion process. The logic for determining mentored teams and displayed teams for mentors was refined, and the meetings table was refactored to utilize the Participants class, establishing a database relationship between mentored teams and meetings. The meetings-related logic was moved from the teams controller to a newly implemented meetings controller, which includes a filter function. An instructor table was added in paginated form, and meeting routes were standardized. The meetings controller was further enhanced with CSS styling and instance variable reorganization, culminating in the integration of @meetings into the Teams Controller model.
```
#POST /meetings def create @team = Team.find(params[:team_id]) @meeting = @team.meetings.new(meeting_params) if @meeting.save # TODO: Re-implement email notification for meeting creation render json: { status: 'success', message: 'Meeting date added' }, status: :created else render json: { status: 'error', message: 'Unable to add meeting date', errors: @meeting.errors.full_messages }, status: :unprocessable_entity end end
#DELETE /meetings/:id def destroy puts "Team ID: #{params[:team_id]}" puts "Meeting ID: #{params[:id]}" puts "Meeting: #{@meeting.inspect}" if @meeting.destroy render json: { message: 'Meeting deleted successfully' }, status: :ok else render json: { error: 'Failed to delete meeting' }, status: :unprocessable_entity end end
resources :teams, only: %i[new create edit update] do collection do get :list post :create_teams post :inherit get :delete get :delete_all get :bequeath_all end resources :meetings, only: %i[create destroy] end
Refactor Existing Codebase
This set of backend and frontend changes focuses on code cleanup, standardization, and compatibility improvements. Unnecessary whitespace was removed, and the database column "date" was renamed to "meeting_date" for clarity. The "get_dates" function was refactored to ensure compatibility with older Ruby versions. Table column names were converted to snake_case to adhere to Ruby naming conventions, and rendering partials were removed to resolve Turbo issues. Finally, an initial refactoring session was conducted with a Large Language Model (LLM) to further refine the code.
Testing and Validation
We have added tests for the teams controller, mailer model, and meetings controller that can be seen below.
With this, our implementations are covered and tested.
Relevant Links
- Github Repository: https://github.com/jmwinte3/expertiza
Team
Mentor
Ed Gehringer
Members
Jacob Winters Alex Wakefield John Buchanan