CSC/ECE 517 Fall 2024 - E2465. UI for Institutions and Notification
About Expertiza
Expertiza, an open-source project built on Ruby on Rails, serves as a versatile learning management platform, enabling instructors to design and customize assignments. It facilitates collaborative learning by allowing students to select topics, form teams, and participate in peer reviews. Recently, the platform has expanded to incorporate modern frontend technologies, with components being refactored to use React.js and Typescript. This upgrade enhances the user experience, making the interface more interactive and responsive while supporting submissions in multiple formats, including URLs and wiki pages, for seamless review and evaluation.
Issues with previous Functionality
- The prior implementation effectively created user interfaces for managing roles and institutions but missed certain critical specifications.
- The project was not merged due to unnecessary dynamic role creation; only administrators required the ability to add roles, so this feature was removed to simplify the design.
- The institution management interface lacked proper authorization, allowing all users access to manage institutions, which conflicted with intended role-based permissions.
Design Goals
- Modernize the Frontend: Re-implement the existing Institutions UI from Ruby on Rails to TypeScript and ReactJS for improved modularity, performance, and maintainability.
- Authorization-Optimized UIs: Ensure role-based access by allowing only designated roles (e.g., TAs, Instructors) to manage specific functionalities in Institutions and Notifications.
- Enhanced Notifications Feature: Introduce a robust notification management system that enables course-specific notifications creation for higher roles and displays relevant notifications to enrolled students.
- Toggle and Filter Options: Implement a toggle for notifications to control active/inactive status and filter notifications for students to display only active, course-relevant alerts.
Implementation
Enhanced the Manage Institutions page UI
Improved the layout and styling of the Manage Institutions page to provide a more user-friendly interface. Adjusted table centering, optimized spacing, and updated visual elements for better alignment and readability.
Added authorization for Institutions commit
Implemented role-based access control to ensure only users with Instructor or higher roles can manage institutions, aligning permissions with user roles and preventing unauthorized access.
Added Manage Notifications Page commit
Developed a dedicated page where authorized users, such as TAs and Instructors, can create, edit, and delete course-specific notifications to streamline communication and course updates.
Following pop-up window appears on clicking create button. the Close/ Create Notification button will redirect to the Manage Notifications page.
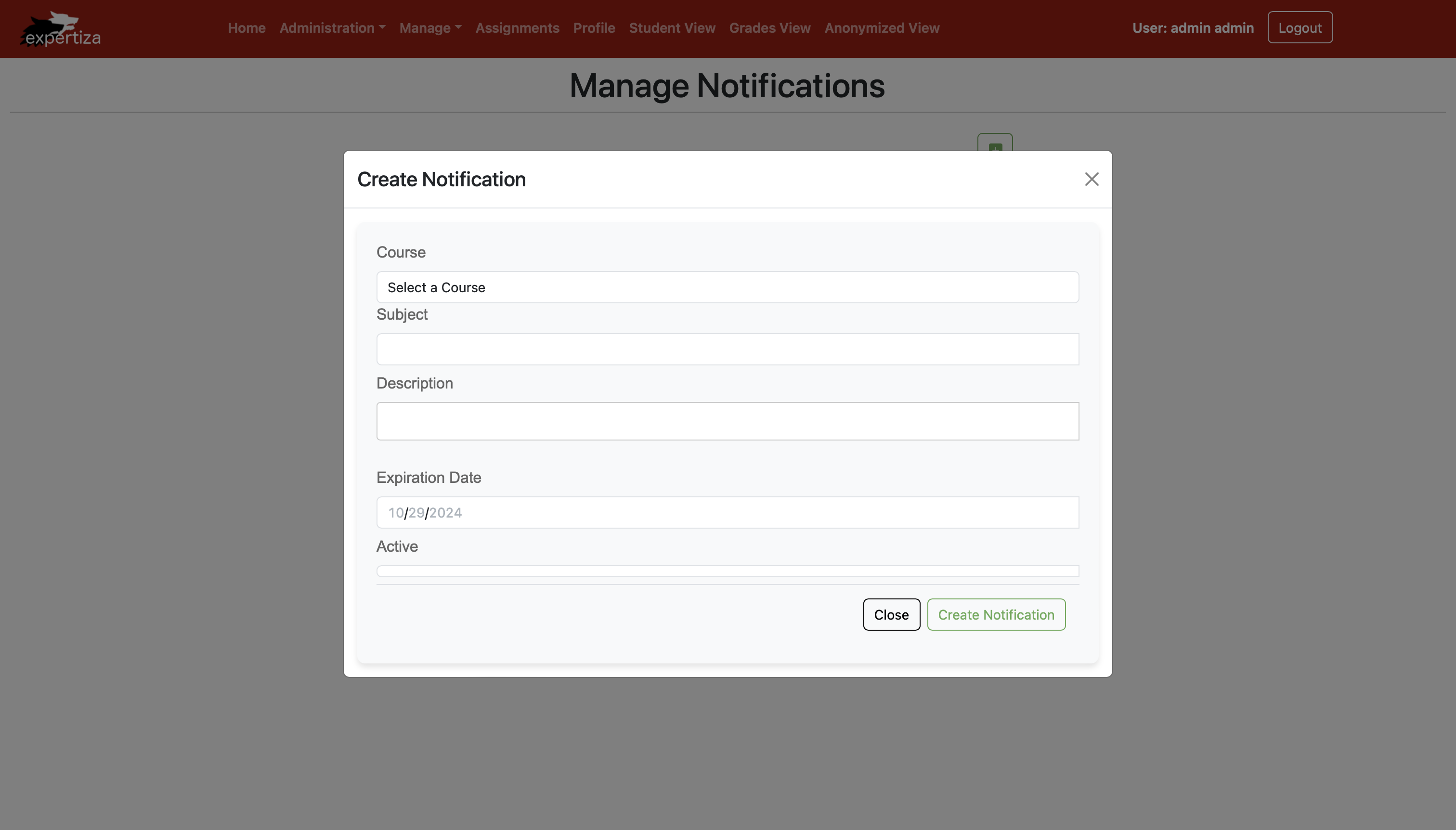
Following pop-up window appears on clicking edit button for an existing notification. the Close/ Update Notification button will redirect to the Manage Notifications page.
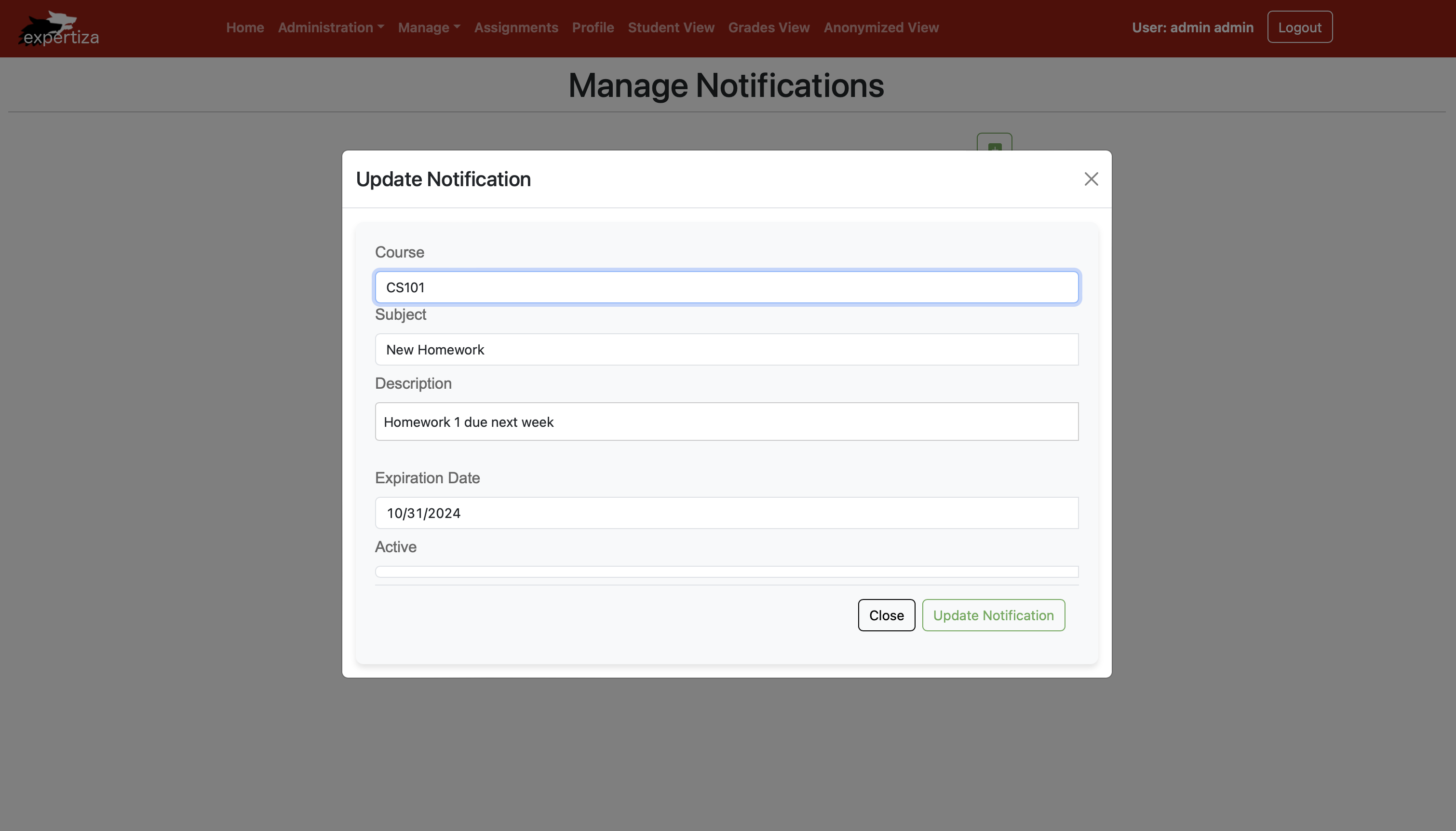
Following pop-up window appears on clicking delete button. the Delete button will redirect to the Manage Notifications page and dispatch an alert message on top of the page that the notification was deleted successfully.
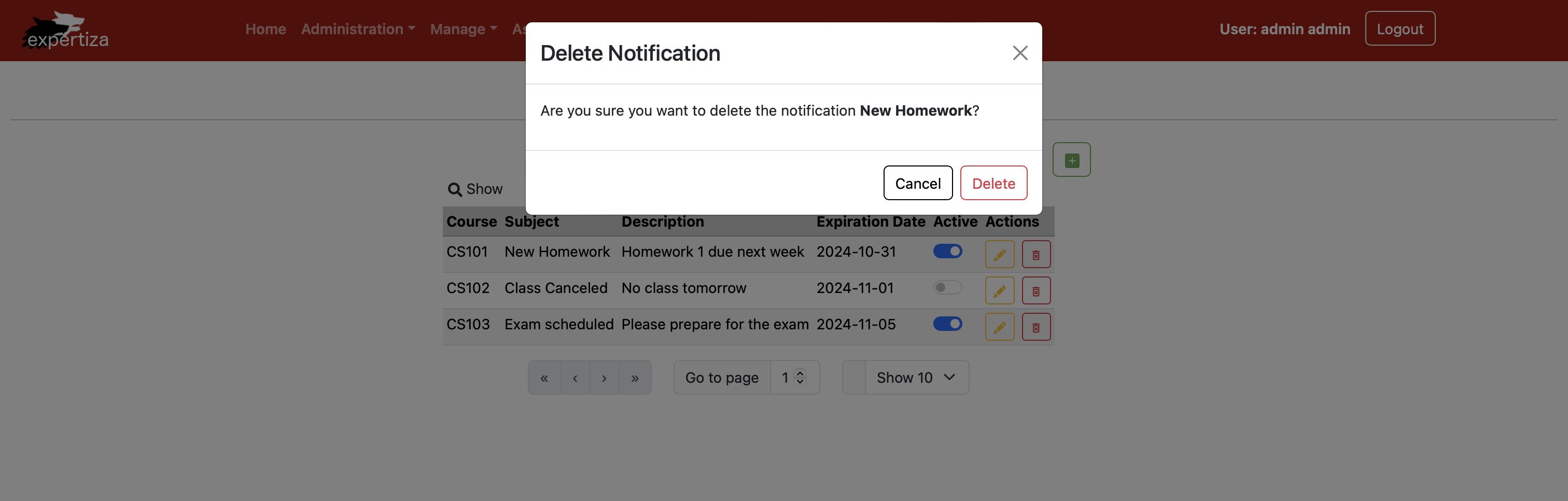
Added My Notifications Page commit
Created a page for students to view relevant, active notifications for courses they are enrolled in, ensuring they receive timely updates on assignments, exams, and other course events.
Added Toggle Notification Feature commit
Implemented a toggle option for authorized users to activate or deactivate notifications, allowing control over the visibility of notifications for students based on course requirements.
Added Notification Badge commit
Introduced a notification badge on the "My Notifications" menu item, displaying the count of new or unread notifications to keep users informed of recent updates at a glance.
Added Alert on Home Page
Implemented an alert on the home page to notify users of any unread notifications upon login, ensuring important updates are immediately visible.
Design Pattern
1. Container-Presenter Pattern
Container components are responsible for managing the logic, data fetching, and application state. Presenter components (also known as Dumb Components) focus on rendering UI elements based on the props received from the container. They don’t manage state or handle business logic directly; instead, they rely on the container for data.
- Institutions and Notifications as Container Components:
- In Institutions.tsx and Notifications.tsx, the primary components act as containers.
- Load the required data using the useLoaderData hook (e.g., fetching institution and notification data).
- Handle logic and user interactions (e.g., onEditHandle and onDeleteHandle for editing and deleting institutions/notifications).
- Handle the permissions and authorization checks, showing different options based on user roles.
- UI Table and Actions as Presenter Components:
- Components such as the Table component and column definitions in institutionColumns.tsx and notificationColumns.tsx act as presenters.
- The presenters don’t hold business logic or directly manage state; they simply render the data provided by the container components.
- For instance:
- The Table component renders the list of institutions/notifications.
- notificationColumns and institutionColumns define the columns, actions, and structure without managing the actual data or state.
2. Strategy Pattern
Used in a Limited Form: The Strategy pattern is partially used here in how we pass callback functions (handleEdit and handleDelete) to the column definitions for the table. These functions define the “strategy” for how each action should be handled, allowing flexibility without modifying the notificationColumns or institutionColumns structures.
- Implementation Detail: By passing these functions as arguments, we can customize the behavior for different actions (like editing or deleting) based on the page context. This approach allows us to define different behaviors without altering the column setup, which is a core aspect of the Strategy pattern.
Testing
Added mock data for verifying the pages are rendered as expected. There is currently no backend implemented, so the tests does not involve calling relevant API endpoints; this will be considered as future work.
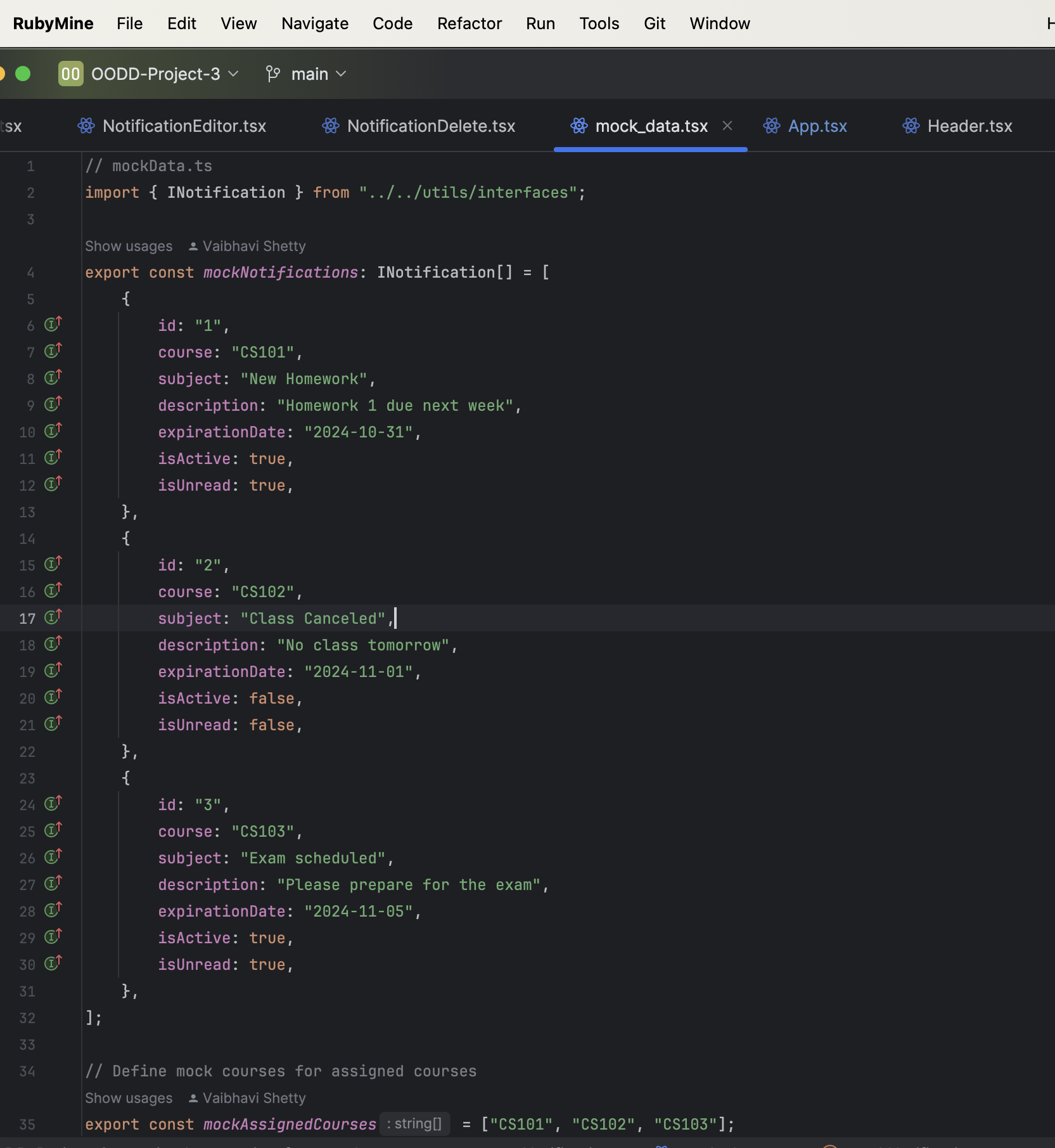
Following is the video demonstrating the UI workflows. Since this is a UI project, the functionality is tested manually.
- Test Video Link: https://youtu.be/h7CiRxTgctA
Team
Mentor Jay Patel : jhpatel9@ncsu.edu
- Vaibhavi Shetty: vshetty2@ncsu.edu
- Soubarnica Suresh ssomang@ncsu.edu
Relevant Links
- Github Repository: https://github.com/SoubarnicaSuresh/reimplementation-front-end
- Pull Request: https://github.com/expertiza/reimplementation-front-end/pull/67