CSC/ECE 517 Fall 2024 - E2458. User management and users table
About Expertiza
Expertiza is an open-source, web-based educational platform built with Ruby on Rails, collaboratively developed by students and faculty at North Carolina State University. Designed to support flexible, interactive assignment management, Expertiza enables instructors to create, customize, and assign topics for students to select based on their interests. It promotes active, team-based learning by allowing students to collaborate on projects and assignments while providing a robust peer review system. Through peer evaluations, students give and receive constructive feedback, enhancing critical thinking and self-improvement skills. Expertiza accommodates diverse submission formats, from file uploads to URLs and wiki pages, making it adaptable for a variety of assignment types and educational approaches.
Introduction
This project focused on enhancing user management in the Expertiza platform by addressing terminology inconsistencies and improving the user interface for viewing user data. Guided by mentor Ed Gehringer, the team aimed to streamline user identification terminology across the codebase and introduce flexible user display options.
Objectives
- Refactor: Replacing outdated terms from the Goldberg gem, which defined users' user-ID's as “names” and users' names as “fullnames,” with clearer labels.
- Enhancement: Expanding user display capabilities to allow viewing of all users on a single page or with options to display 25, 50, or 100 users per page.
Refactor
The codebase included over 892 instances of user
and 1000 instances of name
, thus requiring careful updates to maintain clarity and consistency across all sections of the code and interface without affecting unrelated uses of these terms. Following the first checkpoint of the project, changes and updates to terminology were spread across 243 files. The following is an example of a method directly affected by the refactoring of both name
and fullname
(link to the respective commit here):
# Before
def user_new(requested_user)
new_user = User.new
new_user.name = requested_user.name # antiquated "name"
...
new_user.fullname = requested_user.fullname # antiquated "fullname"
...
end
# After
def user_new(requested_user)
new_user = User.new
new_user.username = requested_user.username # replaced "name" with "username"
...
new_user.name = requested_user.name # replaced "fullname" with "name"
...
end
Enhancement
Prior to the enhancement, users can only choose to display 25, 50, or 100 users on a single page. The enhancement thus aimed to provide an option to display all users on a single page, providing efficient text searches for names, user IDs, roles, and other details listed, and improving overall user management experience.
Following the first checkpoint of the project, the following were included as part of the enhancement:
- Added a fourth option to display all users on a single page on top of the existing three paginate options.
- Modified the
@per_page
parameter in thepaginate_list
method to dynamically set the number of users per page based on user input or default values. - Added tests for all pagination options to verify the functionality and behavior of the
paginate_list
method.
The following files were modified for the enhancement:
- app/controllers/users_controller.rb
- spec/controllers/users_controller_spec.rb
- spec/factories/factories.rb
Changes in users_controller.rb
In users_controller.rb, the changes made were located in the paginate_list
method. Pagination options for displaying user data were enhanced by adding flexibility to the pagination choices. The original pagination options allowed only for three fixed choices: 25, 50, and 100 users per page. The new code includes an additional option to display all users on a single page by dynamically setting the per_page
parameter to the total user count (User.count
). Additionally, the @per_page
variable now checks for a per_page
parameter from user input, defaulting to the new “show all” option (4
), and the pagination logic adapts accordingly.
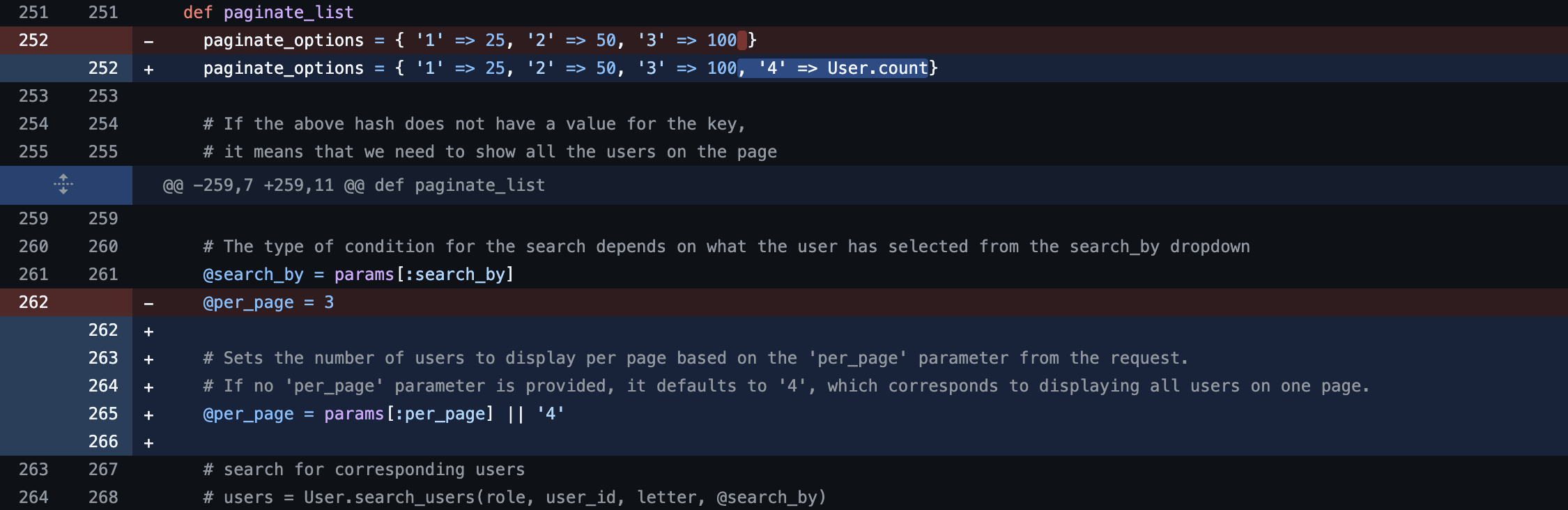
Link to video of user pagination here
Link to the changes here.
Changes in users_controller_spec.rb
Tests were added for paginate_list
in users_controller_spec.rb. Each test is designed to verify that each of the pagination options display the correct number of users per page. The tests are grouped within a paginate_list
context to focus specifically on pagination behavior. To set up the tests, 110 users are created in the database using FactoryBot.create_list(:test_user, 110)
, ensuring a sufficient number of users to test different pagination configurations.
context '#paginate_list' do
before do
# Seed the database with a number of users for pagination tests
FactoryBot.create_list(:test_user, 110)
end
it 'displays 25 users per page when per_page is 25' do
get :list, params: { per_page: '1' }
expect(assigns(:paginated_users).length).to eq(25)
end
it 'displays 50 users per page when per_page is 50' do
get :list, params: { per_page: '2' }
expect(assigns(:paginated_users).length).to eq(50)
end
it 'displays 100 users per page when per_page is 100' do
get :list, params: { per_page: '3' }
expect(assigns(:paginated_users).length).to eq(100)
end
it 'displays all users on a single page when per_page is "all"' do
get :list, params: { per_page: '4' }
expect(assigns(:paginated_users).length).to eq(110)
end
end
The first test checks that, when the per_page parameter is set to '1', only 25 users are displayed per page. The second test adjusts per_page to '2' and expects to see 50 users on the page. Similarly, the third test verifies that setting per_page to '3' results in a display of 100 users per page. The final test explores the option to display all users at once by setting per_page to '4', with the expectation that the page will show all 110 users. Link to the lines added here.
Changes in factories.rb
This change in the :test_user
factory in factories.rb modifies the username
and email
fields to dynamically generate unique values for each test user, enhancing the reliability of tests, specifically for the purpose of testing paginate_list
. Previously, every user created with this factory had the fixed username "username"
and email "abc@mailinator.com"
, which could lead to conflicts when multiple users were created. Now, a sequence is used to append an incrementing number to both the username
and email
, resulting in unique values like "username1"
, "username2"
, and "user1@mailinator.com"
, "user2@mailinator.com"
, and so on. This ensures each user has a distinct username and email, avoiding duplication issues when creating many test users, such as in the case for creating 110 dummy users to test paginate_list.
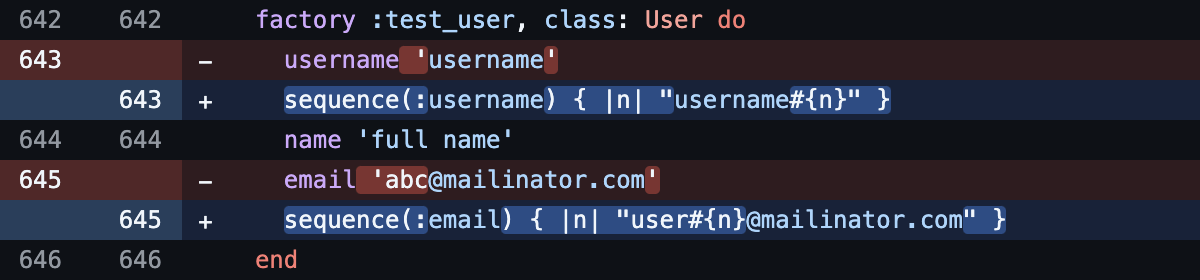
Link to the changes here.
Testing
To ensure the functionality and reliability of the updates, automated testing was implemented using GitHub Actions. All tests were executed with the command bundle exec rspec spec/
, which allowed the full suite of tests to be ran, giving confirmation that the changes met the expected standards without introducing regressions.
Team
Members
- Brandon Walia
- Kevin Dai
- Manav Patel
Mentor
- Dr. Ed Gehringer