CSC/ECE 517 Fall 2009/wiki2 5 ReflectionApis
Reflection APIs
Introduction
Reflection is a programming technique used to build code that is flexible by giving the programmer the power to modify and examine the behaviour of a program at runtime. Reflection gives the code access to internal structures of classes - constructors, fields and methods. It falls under the broad area of Metaprogramming - programs that write other programs.Reflection can be very powerful for late binding, creating new types duing run-time, examining types etc.
This wiki will walk you through the facilities provided by specific languages for using reflection. Is reflection "built in" to some o-o languages, while others require external libraries to perform reflection? What built-in and library functions are provided? Which APIs are easiest to use and why?.
Reflective Languages
The concept of reflective programming was started late back in the 80's. Since then, there have been many languages that provide reflection capabilities. Reflection requires dynamic modification of the program. For this reason, compilers would have to have some metadata format to lok back at it's own code. Java and C# do this through the Java virtual machine and the .NET VM respectively. On the other hand, since dynamic languages like Python and Ruby only try to interpret the code at run-time, more powerful forms of reflection can be achieved. Other languages like C, C++ lack reflection capabilities largely because of the metadata that they fail to maintain. The following are a few languages that support reflection:
• Java
• C#
• Ruby
• Smalltalk
• Python
• Eiffel
Is reflection "Built-in"?
Reflection naturally comes to interpreted languages. Ruby, Python, Smalltalk would not have to go out of their usual way to implement and exhibit reflective properties. On the other hand, Java and .NET look at the intermediate code to figure out the structure of a class and then try to add more at run-time. This, obviously takes a performance hit since the code cannot be compiled and optimized. Java and .NET both have references to objects as well as built-in types. Wrappers for built-in types exist that provide reflection on primitive types.
Languages like C, C++ lack reflective capabilities. They are based on the philosophy of "You don't pay for what you do't use". Maintaining information about types, methods and the like involve significant overhead. Moreover, performance can become a bottleneck, not to mention a few security concerns with reflection. C and C++ compilers optimize code to maximize performance and are not open to trade performance and overhead. If reflection is deemed necessary for such languages, there would have to be another library or a tool to take care of parsing the source code, building abstract syntax trees containing every detail of the program, build symbol tables, extract control and data flows by building a graphical representation and have custom analysers analyse this representation. The DMS Software Reengineering Toolkit is one such toolkit for C, C++ and COBOL.
Programming Language APIs
Java
The java virtual machine is capable of generating an instance of java.lang.Class for every object created. This Class consists of methods that can examine the properties of the object at runtime. In java, all the reference types are inherited from the Object class. The primitive types on the other hand have wrapper classes around them - for example int has the Integer class for it's wrapping. For example, to get the name of a class:
public static void main(String[] args) { Class c = java.lang.Integer.class; String info; info = c.getName(); System.out.println(info); //Prints out java.lang.Integer }
Similarly, an example to find out about all the methods in a class and method parameters is listed below
public class testMethod { int func1( Object obj) throws NullPointerException { if (obj == null) throw new NullPointerException(); return 1; } public static void main(String args[]) { try { Class c1 = Class.forName("testMethod"); Method mtd[] = cls.getDeclaredMethods(); for (int i = 0; i < mtd.length; i++) { Method m = methlist[i]; System.out.println("name = " + m.getName()); System.out.println("decl class = " + m.getDeclaringClass()); Class params[] = m.getParameterTypes(); for (int j = 0; j < params.length; j++) System.out.println(" param #" + j + " " + params[j]); } } catch (Throwable e) { System.err.println(e); } } }
Before using reflection, Java requires the user to import java.lang.reflect.*; This is the package that provides reflective capabilities. Java provides APIs for Retrieving class objects, Examining class modifiers and types and discovering class members. What Java lacks however is the finer granularity when compared to .NET. The Class objects that java uses tend to lose some information, for example, parameter names.
Java's reflection also includes useful methods that return classes themselves. The complete design of all the related classes can be captured using APIs such as:
•getSuperClass() : To return the classes' super class
•getClasses() : Return all members including inherited members
•getEnclosingClass() : used in case of nested classes. Returns the immediate enclosing
The APIs to extract and modify Fields, methods and constructors can be found here[1]. More information about Java's Reflection APIs along with examples can be found here[2]
C#
C# differs from Java in the way it handles reflection. While Java works at the class level, C# goes a level further to implement reflection at the assembly level. These assemblies are nothing but .DLL files. Thus, to make a long sentence short, Java looks at the .class file while C# looks for the corresponding DLL file.
C# also has a unique class called ParameterInfo which contains more metadata information. While java rips off some items like parameter names, ParameterInfo strives to hold all this information thus allowing for finer granularity reflection. Apart from these there are no major differences between the way reflection is handled in Java and C#.
The functionality of the APIs available in C# closely resemble the ones in Java differing in their hierarchies and names. Here is a small example that shows the C# way of reflecting on an assembly.
using System; using System.Reflection; public class ReflectMe { public static void Main( ) { Assembly assem = Assembly.Load("Mscorlib.dll"); Type[] assemTypes = assem.GetTypes(); foreach(Type t in assemTypes) { Console.WriteLine("Type =>{0}", t); } Console.WriteLine(assemTypes.Length); } }
Examples
IBM Rational ClearCase is a widely used version control software with a secure version management and easy to follow interface. It follows the guidelines of the best practices for source code management with version control. It centralizes the code, making it accessible to anyone in the software development team for a particular development. The ClearCase software supports parallel development and easy merging techniques. One of the most useful features of IBM Rational ClearCase is the views. It provides two different views, SnapShot and Dynamic. SnapShot views help to create local copy of the code from the codebase while the dynamic one is used when the codebase has to be modified (during merge). This feature helps to maintain the version of the code by allowing the developer to choose the level of access required for the code.
As discussed in the previous section, the ClearCase maintains the main branch as the baseline and when a new project has to be derived from the main branch, a sub-branch is created which uses the baseline. This sub branch is used to build the project on. Every developer who is a part of the project team and for whom the project's main branch is accessible is given a unique id. Whenever a file is checked out by a developer, his name appears on the ClearCase view. The rest of the team can always see which file is being modified / looked at by which team members. This feature sometimes helps the developer to decide whether he should access the file to modify it now or not. Also, the ClearCase maintains every version of a file. Every time a file is checked out, a copy is maintained, so that when the modified version of the file is checked in, the view still has the old version of the file as well. One of the most powerful features of this tool is that every time a project is successfully completed and needs to be released to make it accessible to the other projects (branches of the main branch), it can be merged with the main branch which makes it accessible to all the other branches.
The following diagram is taken from the IBM Boulder site. It is a snapshot of the ClearCase.
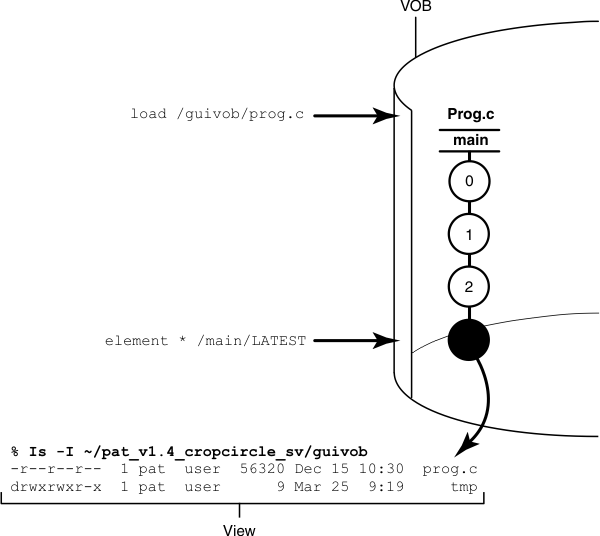
The above diagram is a snapshot view of a shared file Prog.c which is in the clearcase. The circles show the different versions of the file, i.e. the number of circles are basically the number of times the file was checked out and checked back in. The dark circle means that the file has been currently checked out by someone called "user" as shown. The file Prog.c, when checked out is copied locally in the user's machine. The changes that the user makes remains local until he checks in the file again.
The details of the tool can be found in the IBM publication cited in the References section of this page.
Another widely used tool for source code management is Subversion - an open source initiative. Subversion has superseeded CVS as the versioning control system of choice since it includes important functionality like deleting and renaming directories which were absent in CVS. The users of SVN range from those working on very small classroom projects to mammoth ones like the ones in SUN Microsystems. SVN uses a relational database, BerkleyDB as the backend and works much faster than CVS. With space for adding metadata with each file and support for "all or nothing" commits, SVN is more stable and allows for better management of the codebase.
The open source document of SVN can be found in the References section of this page.
Conclusion
Source Code Management or Revision Control is a way of helping the software development for faster and efficient delivery and reduce the amount of rework or manual code maintenance. In a typical development team setup, it becomes a critical tool which is used by every team member. Hence it becomes very important for the version control systems to be accurate and user friendly. Not only does it need to be simple to understand, but it also has to be reliable and transparent without any conflicts.
See also
• For Beginners
The high level overview of SCM best practices.
• For Advanced Readers
An in-depth view of source code management using different patterns. It helps in organizing related lines of development into appropriately diverging and converging streams of source code changes.
This is the product RedBook released by IBM on IBM Rational ClearCase. It explains the different features of their Version Control tool which follow the best practices discussed in this page.
• For Developers
The IBM Rational ClearCase Version Control tool document contains tutorials and examples that are easy to follow.
Information about SVN and its free download versions by O'Reilly media can be found here
References
1. http://www.ibm.com/developerworks/library/j-dyn0603/
2. http://oreilly.com/catalog/progcsharp/chapter/ch18.html
3. http://www.25hoursaday.com/CsharpVsJava.html#reflection