CSC/ECE 517 Fall 2024 - E2472. Reimplement responses controller.rb
Introduction
Background
Expertiza has Response objects, which represent a response that is created by a user. An instructor, TA, or student can perform several kinds of operations on Responses, such as, ”Delete a response”, “Update a response”, and “Create a response”. These operations can be done on each of the items of a rubric, survey, or quiz.When creating the answer blanks, it needs to cycle through the Questions on the Questionnaire in the order specified by their sequence numbers.
Motivation
This project in particular intends that the students collaborate with each other and work on making enhancements to the code base by applying the concepts of Rails,RSpec, DRY code,Test driven development etc. This provides an opportunity for students to contribute to an open source project and learn further about software deployment.
Currently, the Responses controller holds methods that perform multiple tasks and others with a few lines of code. Its Edit and New methods, in particular, could be rewritten to make the code more DRY.
Tasks Identified
- Follow the convention for controller name
- Reimplement the edit and delete method
- Reimplement the assign_action_parameters and set_content methods
- Reduce the multiple calls to the sort_questions method
- Changing methods to accept parameters
Classes
- controllers/Responses_controller.rb
- models/Response.rb
Modules
- helpers/response_helper.rb
Follow the convention for controller name
The naming convention for controllers dictates that they be named in the plural, but Expertiza predates that convention and uses the singular for a few controllers including the Responses controller. The reimplementation is to follow convention. For that reason, response_controller.rb was renamed responses_controller.rb
Reimplementing the Edit and Delete method
The edit function contains many functionalities that need to be rewritten into separate functions because these functionalities do not apply to the action of editing.
BLOCK 1: The following block contains functionality for sorting reviews.
This part of the code is moved into the response model as a separate function:
BLOCK 2: The following block contains functionality for checking if a reviewer is a team:
if @map.team_reviewing_enabled ... end
This part of the code is moved into the application_controller.rb file as a separate method:
def map_team_reviewing_enabled?(map_team_reviewing_enabled) map_team_reviewing_enabled == true end
BLOCK 3: There is redundant code for locking a response in the edit and delete methods:
if @map.team_reviewing_enabled @response = Lock.get_lock(@response, current_user, Lock::DEFAULT_TIMEOUT) if @response.nil? response_lock_action return end end
This redundant code is removed from both methods and reimplemented as a separate method and placed in the respnse_helper.rb Module:
# locks a response based on an authenticated user def lock_response(map,curr_response) if map_team_reviewing_enabled?(map.team_reviewing_enabled) this_response = Lock.get_lock(curr_response, current_user, Lock::DEFAULT_TIMEOUT) if this_response.nil? response_lock_action end return this_response end return curr_response end
Reimplement the assign_action_parameters and set_content methods
In the controller, there are multiple methods to set parameters (assign_action_parameters, set_content).
Although these methods are much better than duplicating code elsewhere, the idea of using 2 different methods to set parameters seems illogical. So, these two methods were combined into one to make the program more readable. The new method is shown here:
Reduce the multiple calls to the sort_questions method
Items/Questions always need to be displayed in order. In the controller and helper, there were 4 calls to sort_questions, whose implementation is only a single line. Since items only need to be sorted right as they are being displayed, it was not clear why four calls were needed. So, 3 calls to sort_questions were deleted as they were not necessary. The method to sort questions is only called once inside the set_action_parameters method which is used by the view action.
Also, the sort_questions method was renamed to sort_items since many of these items are not questions.
Changing methods to accept parameters using the DRY principle
Many methods manipulate objects. Some methods that are meant to check the value of a parameter also update parameter values that they are not supposed to update. Also, many methods assume that the current value of a parameter is the correct one, but this should not be the case. To make the application more DRY, many methods are updated to use accept parameters and use these parameters to find a specific parameter, and then return this value to the caller. Also, this change makes the methods easier to reuse in the long run. For illustration, the before and after images of the methods are shown below:
METHOD 1: questionnaire_from_response_map
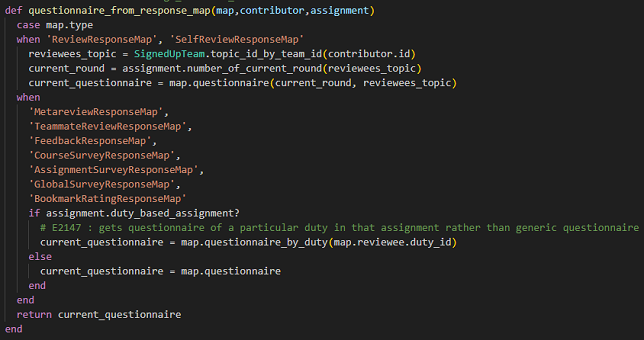
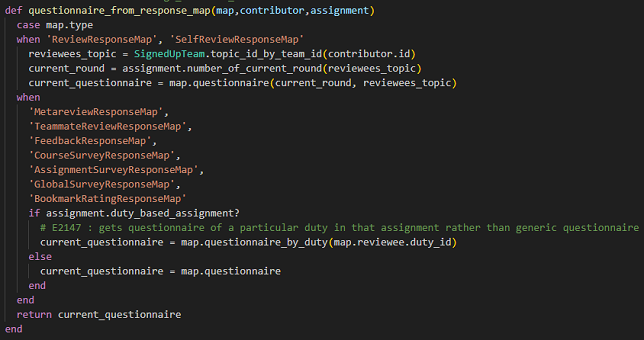
METHOD 2: questionnaire_from_response
METHOD 3: set_dropdown_or_scale
METHOD 4: store_total_cake_score
Impact Analysis
Affected Classes
Changed existent classes
- controllers/responses_controller.rb
- controllers/application_controller.rb
- models/responses.rb
Changed existent Modules
- response_helper.rb
Running the Project Locally
The project could be run locally by cloning the Github repository expertiza and then running the following commands sequentially.
bundle install rake db:create:all rake db:migrate rails s