CSC/ECE 517 Spring 2023 - E2339. Reimplement signed up team.rb, sign up topic.rb, sign up sheet.rb (Project 4)
Team Members
- Ajith Kanumuri
- Suhas Adidela
- Ravi Chandu Bollepalli
- Mentor - Dinesh Pasupuleti
- PR Request:- https://github.com/expertiza/reimplementation-back-end/pull/125
- Project Board:- https://github.com/users/ajith05/projects/1/views/1
Project Description
The main goal of this project is to reimplement essential Ruby on Rails model classes—SignUpTeam, SignUpTopic, and SignUpSheet—from the Expertiza repository in a new repository. Each of these model classes plays a critical role in managing topics and team sign-ups within course assignments, and the reimplementation aims to bring them into alignment with Rails best practices and the DRY (Don't Repeat Yourself) principle.
The SignUpTeam model represents a team that has signed up for a specific topic within an assignment. This model manages team-specific data and oversees interactions that occur during the sign-up process. The SignUpTopic model, on the other hand, represents the topics available for sign-up within an assignment, managing essential details like slot availability and assigning teams to open topics. Lastly, the SignUpSheet class serves as a module, storing the methods needed to create and manage instances of SignUpTeam for assignment topics.
Since this project is being implemented from scratch, a range of components have been developed to ensure the functionality is both streamlined and maintainable. To guarantee the reliability of each part of the reimplementation, unit test cases have been created to verify the integrity of the new design and implementation.
Issue Description
- Comprehensive RSpec tests are essential for all reimplemented models to ensure correctness and reliability in functionality.
- Update all references from `sign_up_teams` to `signed_up_teams` throughout the codebase to maintain consistency with the new naming convention.
- Address all code review feedback to improve code quality and alignment with best practices.
- Currently, there is no specific waitlist model to manage waitlisting, upgrading, or handling multiple registrations. This functionality needs to be considered and integrated effectively.
- Refactor methods with readability issues to make the code more maintainable and easier to understand.
- Optimize ambiguous class and instance methods to enhance reusability and improve the overall structure of the code.
- The `SignedUpTeam` class currently contains methods with multiple responsibilities, such as finding participants and teams, releasing topics, and removing signed-up teams. This violates the Single Responsibility Principle, making the class more challenging to understand and maintain. Separate these responsibilities for improved clarity.
- Some methods use complex SQL queries with multiple joins, which can be challenging to read and understand. Simplify these SQL queries by leveraging ActiveRecord's query interface to enhance readability and maintainability.
- The codebase has several instances of repeated code, such as finding signed-up teams for a given topic. This repetition violates the DRY principle, making the code harder to maintain and increasing the potential for errors. Consolidate these instances to streamline the code.
Design Strategy
- Implemented three model classes: `sign_up_team.rb`, `sign_up_topic.rb`, and `waitlist.rb` to address project requirements.
- Removed `sign_up_sheet.rb`; refactored its methods into `sign_up_topic.rb` and `waitlist.rb` for modularity.
- Each model now has a focused role, improving maintainability and clarity.
- In signed_up_team.rb, the following functionality are being implemented:-
Method Name | Status | Description |
---|---|---|
drop_off_team_waitlists | Implemented | Removes the team from all waitlists for topics within the assignment. |
find_first_existing_sign_up | Implemented | Finds the first existing sign-up entry for a given topic and team, checking for both active and waitlisted sign-ups. |
create_signed_up_team | Implemented | Creates a SignedUpTeam instance with specified topic_id and team_id if the topic is available for sign-up. |
get_team_id | Implemented | Retrieves the team ID associated with a given SignedUpTeam entry. |
delete_signed_up_team | Implemented | Deletes the signed-up team entry for a specific topic, managing any dependent records as necessary. |
- Add necessary schema migrations to rename signup topic to project topic.
- In project_topic.rb, the following functionality are being implemented:-
Method Name | Status | Description |
---|---|---|
slot_available? | Implemented | Checks if there are available slots for the topic based on the maximum allowed choosers. Returns true if available. |
assign_topic_to_team | Implemented | Assigns the topic to a team by updating the sign-up entry to set is_waitlisted as false and assign the topic_id. |
save_waitlist_entry | Implemented | Saves a sign-up entry as a waitlist entry by setting is_waitlisted to true and assigning the topic_id. |
sign_up_team | Implemented | Manages the sign-up process for a team, checking slot availability, assigning the topic, or placing the team on the waitlist as needed |
Refactoring and Enhancements
- Refactor instance methods to make them more concise (e.g., rename `slot_available?` to `available?`, `create_signed_up_team` to `sign_up_team`, `drop_off_team_waitlists` to `drop_team_waitlists`).
- Rename the model from “Sign Up Team” to “Signed Up Team” for improved clarity.
- Remove the `is_waitlisted` attribute, utilizing the `Waitlist` model for more efficient waitlist querying.
- Implement tests for error conditions and edge cases to ensure robust error handling.
- Add tests to validate the use of `@counter_cache`, ensuring accurate relation counts.
- Create any required empty models and define necessary model-association relations.
- Integrate waitlist management within `project_topic.rb`, delegating related functionalities to the `Waitlist` model.
- Add cascading delete functionality for related records across models to maintain data integrity.
- Include JSON serializers to format data for REST API responses.
- Use `shoulda-matchers` for comprehensive testing of model validations and associations.
- Integrate `FactoryBot` to automate model record generation in tests, improving test efficiency.
- Introduce `preference_priority_number` to maintain backward compatibility during migrations.
E/R Diagram
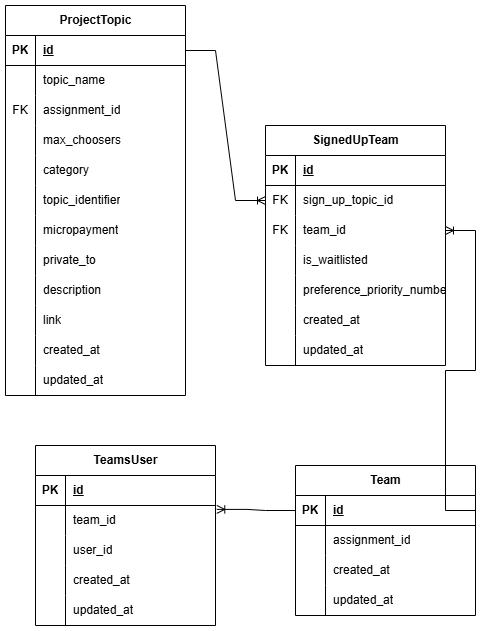
Edit Button Tooltip
Test Plan
- Upgrade RSpec test cases for `signed_up_team.rb` to validate `delete_signed_up_team` and `get_team_participants` methods.
- Enhance and expand RSpec test cases for `project_topic.rb` to validate participant retrieval, slot availability, and assignment status handling.
- Develop RSpec test cases for `waitlist.rb` to validate adding teams to the waitlist, removing teams from the waitlist, and querying the waitlist status of a team.
- Add general RSpec test cases for error handling and ensure correct JSON serialization of data..
- Test Cases for signed_up_team.rb model
- Test Cases for waitlist.rb model
- Test Cases for signup_topic.rb