CSC/ECE 517 Fall 2010/ch3 S30 RJ
Decomposition, Message Forwarding, and Delegation versus Inheritance in OOP Design
Most OOP overviews explain what the concept of Inheritance is in Object Oriented Programming, and how Inheritance works, but not many OOP overviews discuss alternatives when Inheritance may not be the best design choice for an OO application. This topic briefly introduces the OOP concepts of Inheritance, Decomposition, Message Forwarding, and Delegation, and then discusses when Decomposition, Message Forwarding, and Delegation could be considered as potential alternatives to the use of Inheritance for some OOP applications.
It needs to be noted that different OOP authors interchange and blur the meaning of what is meant by Decomposition, Message Forwarding, and Delegation in OOP. In general discussions about Decomposition, Message Forwarding, and Delegation in the context of OOP are suggesting alternative ways to accomplish OOP designs other than utilizing a traditional OOP design that uses pure Inheritance principles.
Inheritance in OOP Design
Inheritance is one of the key concepts in Object Oriented Programming. In OOP, parent classes describe and define attributes and capabilities of objects in a parent class. Subsequently subclasses can be defined to create objects that inherit or acquire the attributes and capabilities of objects that belong to the parent class, or Superclass of the child, or subclass. Programming code that is created by programmers to describe the attributes and methods of objects in a parent class can easily be reused by objects that are created in subclasses of the parent class. Using inheritance in a OO application is appropriate when the relationship between the Superclass and subclass is a 'Is-A' relationship. Consider the concept of single inheritance through class extension[1]
class Fruit { //... } class Apple extends Fruit { //... }
In the code above, class Apple is related to class Fruit by inheritance, because Apple extends Fruit. In this example, Fruit is the superclass and Apple is the subclass.
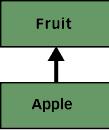
Decomposition in OOP Design
A useful starting definition for Decomposition in OOP is "In OO decomp, we think in terms of identifying active, autonomous agents which are responsible for doing the work, and which communicate with each other to get the overall problem solved." [1]
The 'autonomous agents' mentioned above would correspond to leveraging known classes and their various objects to get a task or process done, rather than taking the time to design a grand Superclass and subclass inheritance hierarchy which contains all the needed objects and classes that are required to address a given task or process. Mixins in Ruby on Rails would be an example of decomposition in OOP, since Mixins allow useful methods from classes to be easily mixed-in, or used as utility methods by classes that may not be suitably related in a traditional inheritance hierarchy.
Composition in OOP Design ??
An alternative to a inheritance relationship is a composition relationship[2], whereby instance variables of an object refer to other objects.
class Fruit { //... } class Apple { private Fruit fruit = new Fruit(); //... }
In the example above, class Apple is related to class Fruit by composition, because Apple has an instance variable that holds a reference to a Fruit object. The UML diagram for this composition relationship is shown below in Figure 2.
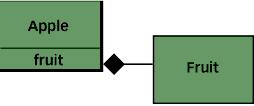
Message Forwarding in OOP Design
Delegation in OOP Design
Delegation in OOP is a technique or strategy whereby an object relies upon or receives services from another object in order to accomplish a task or process, rather than having an object have all required services or methods defined within itself. Delegation in OOP strives to allow one object to reuse functionality that another object can readily provide. Delegation in OOP can therefore be said to realize the benefits of code reuse, but not in an official OOP code reuse manner that is usually implied as a reason for implementing traditional OOP inheritance. Instead of creating new code in a parent or Superclass that can then be reused by a child or subclass object, delegation invokes services from other objects, thereby taking advantage of code that already exists to support the other existing object. The functionality available in the use of mixins in Ruby on Rails would be an example of delegation in OOP.
References:
References: <references/>