CSC/ECE 517 Fall 2010/ch4 4d PR: Difference between revisions
No edit summary |
No edit summary |
||
Line 179: | Line 179: | ||
} | } | ||
==Conclusion== | ==Conclusion== | ||
Every programming language support namespaces at different abstraction level. They are not just containers but designed to allow simple,fast and efficient usage and understanding of the code. | Every programming language support namespaces at different abstraction level. They are not just containers but designed to allow simple,fast and efficient usage and understanding of the code. Namespaces actually provide luxu | ||
1. Protect code from name clashes | 1. Protect code from name clashes | ||
2. Namespace gives better structure to program | 2. Namespace gives better structure to program |
Revision as of 01:57, 16 October 2010
Namespaces is a set of names which are bound together to form unique identifier in order to resolve conflict with same names from different context. Namespaces give you control over the visibility of the properties and methods that you create.
Overview
A namespace is a way to keep names organized.Lets use an analogy to file system in the computer which uses directories and sub directories.Namespaces in this context could be viewed as the path to a file in the directory/subdirectory which can uniquely identify each file.
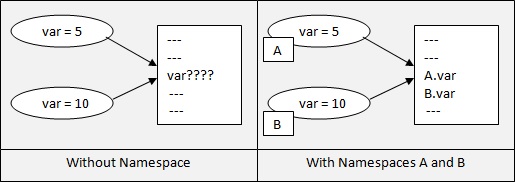
Types of Namespaces
Implicit Namespaces
Implicit Namespaces are the abstraction defined by language itself in order to resolve name conflicts.Some of the constructs of implicit namespaces in different languages are records,dictionaries,objects, environments,packages,interface,scope resolution.
Blocks
Blocks are often used as an abstraction to resolve naming conflicts. In many languages blocks determine scope of the variables. Hence a variable declared in a particular block has its scope within the block. Hence, we can think of a block as a namespace.
Below is the example that shows how a block resolves naming ambiguities.
main() { int i=5; ------ ------ { int i=10; ------ ------ //printing I here gives value equal to 10 } //printing I here gives value equal to 5 }
Objects
In JavaScript, a namespace is actually an object which is used to references methods, properties and other objects.Objects are all we need to create namespaces in Javascript.
Below is an example of a modules in Javascript:
var Val = { Hello: function() { alert("Hello...!!!"); }, Goodbye: function() { alert("Goodbye...!!"); } } // In a nearby piece of code... Val.Hello(); Val.Goodbye();
Packages
Packages can be used as a mechanism to organize classes into namespaces which are mapped to file system. A unique namespace is provided by each package which gives flexibility to define same identifier name over different packages.
Below is an example of a package in Java:
package A; class A1 { int var = 4; } package B; class B1 { int var = 12; }
Modules
In some languages, different constructs are used to identify namespaces. Languages like Ruby maintains namespace at higher level of abstraction known as modules which can be thought to be similar to packages in Java.
Below is an example of a modules in Ruby:
module Trig PI = 3.141592654 def Trig.sin(x) # .. end def Trig.cos(x) # .. end end module Moral VERY_BAD = 0 BAD = 1 def Moral.sin(badness) # ... end end require 'trig' require 'moral' y = Trig.sin(Trig::PI/4) wrongdoing = Moral.sin(Moral::VERY_BAD)
Attributes
XML namespaces provide method for qualifying element and attribute names by associating them with namespaces identified by unique URI references.For example, Id could be in both vocabularies,employee, student, hence we can provide uniqueness for each vocabulary using namespaces to avoid conflicts.
A namespace is declared using the reserved XML attribute xmlns, the value of which must be an Internationalized Resource Identifier (IRI), usually a Uniform Resource Identifier (URI) reference.
Below is an example of a attributes as a namespace in XML:
<root xmlns:fruits="http://www.w3schools.com/fruits" xmlns:furniture="http://www.w3schools.com/furniture"> <fruits:table> <fruits:tr> <fruits:td>Apples</fruits:td> <fruits:td>Bananas</fruits:td> </fruits:tr> </fruits:table> <furniture:table> <furniture:name>Sofa</f:name> </furniture:table> </root>
Explicit Namespaces
Namespaces can be explicitly manipulated and composed, making it quite a simple matter to combine, rename and compose packages or modules. Hence some languages use keyword "namespaces" for declaring namespaces. For example, C, Cpp, PHP, C#. Below is the CPP code which depicts use of namespace keyword.
namespace Square{ int length = 4; } namespace Rectangle{ int length = 12; int breath = 8; } int main () { cout << Square::length << endl; //output 4 cout << Rectangle::length << endl; //output 12 cout << length << endl; return 0; }
Similarly, PHP uses the namespace keyword to distinguish between identifiers.Below is an example of PHP using namespace keyword:
<?php Namespace A; Const NAME = ‘this is constant’ Function FUN() { ----- ----- return _MYVARIABLE_; } Class C { function print_this() { ------ ------ return _THIS_METHOD_; } } ?>
Similarly, C# also uses namespace keyword to distinguish names. Below is the code of C# showing use of namespace keyword.
namespace A { public class A1 { static void Main() { B.B1.welcome(); } } } namespace B { public class B1 { public static void welcome() { Console.WriteLine("Welcome"); } } }
Conclusion
Every programming language support namespaces at different abstraction level. They are not just containers but designed to allow simple,fast and efficient usage and understanding of the code. Namespaces actually provide luxu 1. Protect code from name clashes 2. Namespace gives better structure to program 3. It becomes easy to maintain large codes with namespaces 4. Custom Branding 5. Integration with Future Web 2.0 Sites
References
[1] Matteo Bordin, Tullio Vardanega "A Domain-specific Metamodel for Reusable Object-Oriented High-Integrity Components"
[2] Van Deursen "Domain-Specific Languages versus Object-Oriented Frameworks: A Financial Engineering Case Study"
[3] Jan Heering, Marjan Mernik "Domain-Specific Languages for Software Engineering"
[4] Martin Karlsch "A model-driven framework for domain specific languages demonstrated on a test automation language"
[5] Uwe Zdun "Concepts for ModelDriven Design and Evolution of DomainSpecific Languages"
[6] Dimitrios S. Kolovos, Richard F. Paige, Tim Kelly, and Fiona A.C. Polack "Requirements for Domain-Specific Languages"
[7] Paul Klint, Joost Visser "Domain-Specific Languages: An Annotated Bibliography1 Arie van Deursen"