MainPage: Difference between revisions
No edit summary |
|||
Line 210: | Line 210: | ||
RSpec has been successfully applied to test the entire controllers directory, ensuring that all controller actions across the application are thoroughly tested. All test examples have passed without breaking any functionality, confirming the reliability and robustness of the controllers, with automated testing through GitHub Actions providing immediate feedback on code changes. | RSpec has been successfully applied to test the entire controllers directory, ensuring that all controller actions across the application are thoroughly tested. All test examples have passed without breaking any functionality, confirming the reliability and robustness of the controllers, with automated testing through GitHub Actions providing immediate feedback on code changes. | ||
[[File: | [[File:Test01.png|thumb|Figure 2: RSpec test results for all controllers and GitHub Actions showing successful test execution]] | ||
==Team Information== | ==Team Information== |
Revision as of 18:00, 24 March 2025
Expertiza Background
The open-source project Expertiza is built using Ruby on Rails, maintained by both the staff and students at NC State. This platform grants instructors complete authority over managing class assignments. Expertiza is packed with versatile tools to support various types of assignments, including options for peer evaluations, forming groups, and adding topics. For a comprehensive overview of all that Expertiza provides, visit the Expertiza wiki.
About Controller
The review_mapping_controller
function is responsible for assigning reviewers to specific assignments. This controller plays a key role in allocating reviews across different student groups or individuals, handling both peer and self-assessment scenarios. Additionally, it manages student requests for reviews and facilitates extra bonus reviews that align with the assignment's requirements.
Functionality of review_mapping_controller
The review_mapping_controller
is a vital part of a system dedicated to managing the assignment and allocation of reviewers for different types of evaluations, such as peer reviews and self-assessments. Its main role is to coordinate the assignment of these reviews across individual students or various groups, using an intricate process that factors in elements like fairness, diverse perspectives, and alignment with assignment criteria. Through this coordination, the controller ensures that every participant receives a thorough and balanced assessment, supporting the overall quality and reliability of the evaluation process.
In addition, the review_mapping_controller
is essential for handling student requests for additional assessments, often driven by needs for extra feedback, additional credit opportunities, or reevaluation. When processing these requests, the controller must adhere to assignment guidelines and carefully evaluate the feasibility of granting extra assessments without affecting the integrity or fairness of the review process. This may involve accounting for practical constraints, such as reviewer availability and workload distribution, to ensure an equitable experience for all participants.
Problem Statement
The review_mapping_controller
poses challenges due to its extensive size, complexity, and lack of comments, which make it hard for developers to fully understand its functions. To improve this, a thorough refactoring is recommended to divide lengthy methods into smaller, more understandable sections. This approach would break down complex logic into modular parts, each handling a particular task within the controller's scope. Additionally, refactoring should focus on eliminating redundant code, consolidating repeated segments into reusable functions or utilities to improve code quality and minimize errors. By reorganizing the controller code and enhancing its documentation, developers can gain a clearer insight into its functionality, simplifying maintenance, troubleshooting, and potential updates.
Tasks
- Refine Extensive Methods: Methods like
assign_reviewer_dynamically
,add_reviewer
,automatic_review_mapping
, andpeer_review_strategy
will be further broken down for greater clarity and efficiency. - Clarify Variable Names: Variables will be renamed to make their roles more transparent, enhancing readability and comprehension.
- Use Subclass Methods Instead of Switch Statements: Existing switch statements will be replaced with subclass methods, contributing to a more modular and scalable code structure.
- Introduce Subclass Models: New models for subclasses will be implemented to streamline organization and simplify management.
- Eliminate Hardcoded Parameters: Any hardcoded parameters will be removed to increase adaptability across different use cases.
- Enhance Comments: Additional comments will be included where helpful, and any vague or redundant comments will be clarified or removed.
- Expand Test Coverage: The test suite will be extended to ensure thorough testing, particularly following the refactoring process.
Implementation
automatic_review_mapping_strategy
Original Code
The automatic_review_mapping_strategy function was complex and handled multiple responsibilities, making it difficult to maintain and test. It lacked proper separation of concerns and had unclear variable names.
Updated Code
The function was refactored to focus on specific tasks, such as:
- Initializing reviewer counts and filtering eligible teams
- Creating a review strategy based on assignment parameters
- Assigning reviews in phases (initial and remaining)
automatic_review_mapping_staggered
Original Code
The automatic_review_mapping_staggered function had limited error handling and lacked proper validation of input parameters. It also had unclear success/failure messages.
Updated Code
The function was improved to:
- Validate input parameters before processing
- Provide clear error messages for invalid inputs
- Handle exceptions gracefully with descriptive messages
save_grade_and_comment_for_reviewer
Original Code
The save_grade_and_comment_for_reviewer function lacked proper transaction handling and had insufficient validation of review grades. Error handling was basic and not comprehensive.
Updated Code
The function was enhanced to:
- Implement proper transaction handling for data consistency
- Add comprehensive validation for review grades
- Provide detailed error messages for validation failures
start_self_review
Original Code
The start_self_review function had limited team validation and unclear error handling. It lacked proper checks for existing self-reviews and team membership.
Updated Code
The function was improved to:
- Validate team existence and membership
- Check for existing self-reviews to prevent duplicates
- Provide clear error messages for various failure scenarios
assign_reviewers_for_team
Original Code
The assign_reviewers_for_team function had complex logic for handling review assignments and lacked proper separation of concerns. Variable names were unclear and error handling was insufficient.
Updated Code
The function was enhanced to:
- Use clear and descriptive variable names
- Separate review assignment logic into helper methods
- Implement comprehensive error handling
peer_review_strategy
Original Code
The peer_review_strategy function had complex participant selection logic and lacked proper validation of review assignments. Error handling was basic and not comprehensive.
Updated Code
The function was improved to:
- Implement clear participant selection logic
- Add validation for review assignments
- Provide detailed error messages for assignment failures
Design Document
Current Status
The project has been refactored to improve code readability, modularity, and maintainability. This refactoring has focused on breaking down complex methods into smaller, single-purpose functions, enhancing error handling, and reducing redundant code through reusable helper methods. These changes align with Ruby best practices and the SOLID principles, making the code easier to understand, extend, and maintain.
Highlights of the Refactored Controller
- Improved modularity with clearly defined helper methods.
- Consistent error handling and validation checks for invalid user assignments and outstanding reviews.
- Reduction in redundant code by centralizing frequently used logic, enhancing the controller's readability and scalability.
Key Changes
automatic_review_mapping_strategy
- Modularization: Split the complex review mapping logic into smaller, focused methods
- Helper Methods: Introduced ReviewMappingHelper for initialization and filtering
- Phased Assignment: Separated review assignment into initial and remaining phases
- Improved Readability: Enhanced method and variable naming for clarity
automatic_review_mapping_staggered
- Input Validation: Added comprehensive parameter validation
- Error Handling: Improved error messages and exception handling
- Flow Control: Added early returns for invalid conditions
- User Feedback: Enhanced flash messages for better user experience
save_grade_and_comment_for_reviewer
- Data Consistency: Added transaction support for grade saving
- Validation: Enhanced review grade validation
- Error Handling: Improved error messages and exception handling
- Response Format: Added support for both HTML and JS responses
start_self_review
- Team Validation: Added comprehensive team existence checks
- Error Handling: Improved error messages and redirection
- Duplicate Prevention: Added checks for existing self-reviews
- User Experience: Enhanced feedback messages for various scenarios
assign_reviewers_for_team
- Code Organization: Separated review assignment logic into helper methods
- State Management: Improved handling of review counts and timestamps
- Variable Naming: Enhanced clarity of variable names
- Error Prevention: Added checks for review needs and team eligibility
peer_review_strategy
- Participant Selection: Improved participant selection logic
- Error Handling: Added validation for review assignments
- Code Structure: Enhanced method organization and readability
- Feedback: Added error messages for failed assignments
Principles Applied
- Single Responsibility Principle (SRP): Each method now has a single responsibility, with helper methods extracted for distinct actions.
- Open/Closed Principle: The refactored code can be extended with additional helper functions or strategies without modifying existing methods.
- DRY (Don't Repeat Yourself): Repeated code segments, especially for participant/reviewer/team assignment, were extracted into reusable methods, reducing redundancy.
- Error Handling: Improved resilience by checking for conditions like invalid users, duplicate reviewer assignments, and outstanding reviews.
Test Coverage Improvements
Spec File Changes
The test suite has been significantly enhanced to provide comprehensive coverage of the refactored controller methods. The following improvements were made to the spec file:
automatic_review_mapping_staggered
- Test Structure: Organized tests into clear contexts for different scenarios
- Mocking: Properly mocked dependencies using RSpec's allow and expect methods
save_grade_and_comment_for_reviewer
- Factory Usage: Utilized FactoryBot for creating test objects
- Format Testing: Added tests for different response formats
- Error Handling: Comprehensive error scenario testing
start_self_review
- Edge Cases: Added tests for various edge cases
- Success Scenarios: Comprehensive testing of successful operations
automatic_review_mapping_strategy
- Strategy Testing: Added tests for different review mapping strategies
- Parameter Testing: Testing with different parameter combinations
Key Testing Improvements
- Better Organization: Tests are now organized into logical contexts and scenarios
- Comprehensive Coverage: Added tests for success, failure, and edge cases
- Proper Mocking: Improved use of RSpec's mocking capabilities
- Factory Usage: Consistent use of FactoryBot for test data creation
- Error Handling: Thorough testing of error scenarios and messages
Final Changes and Testing
Overview
The refactoring process has significantly improved the codebase by modularizing functions, enhancing documentation, and expanding test coverage. The following sections detail the key changes and testing improvements:
Modularization and Helper Integration
- ReviewMappingHelper Module: Centralized common functionality
- Controller Refactoring: Simplified controller methods
Enhanced Documentation
- YARD-Style Comments: Added comprehensive method documentation
- Method Descriptions: Clear documentation of method purposes and parameters
Testing Improvements
- Comprehensive Test Coverage: Added thorough testing of all functionality
- Error Handling Tests: Improved testing of error scenarios
- Edge Case Testing: Added testing of boundary conditions
Key Testing Improvements
- Better Organization: Tests are now organized into logical contexts and scenarios
- Mocking and Stubbing: Improved use of RSpec's mocking capabilities for better isolation
- Factory Usage: Consistent use of FactoryBot for test data creation
- Edge Cases: Comprehensive testing of boundary conditions and error scenarios
- Response Format Testing: Added tests for different response formats (HTML/JS)
Benefits of the Changes
- Improved Maintainability: Modular code structure makes future updates easier
- Better Testability: Isolated components are easier to test
- Enhanced Documentation: Clear documentation helps new developers understand the code
- Reduced Duplication: Centralized helper methods eliminate code redundancy
- Robust Error Handling: Comprehensive error scenarios are now properly handled
RSpec Test Results
Test Coverage Overview
RSpec has been successfully used to test the ReviewMappingController and ReviewMappingHelper, ensuring comprehensive coverage and reliability. All tests have passed, demonstrating that the controller's functionality and helper methods are working as expected, with proper validation of key behaviors, error handling, and edge cases.
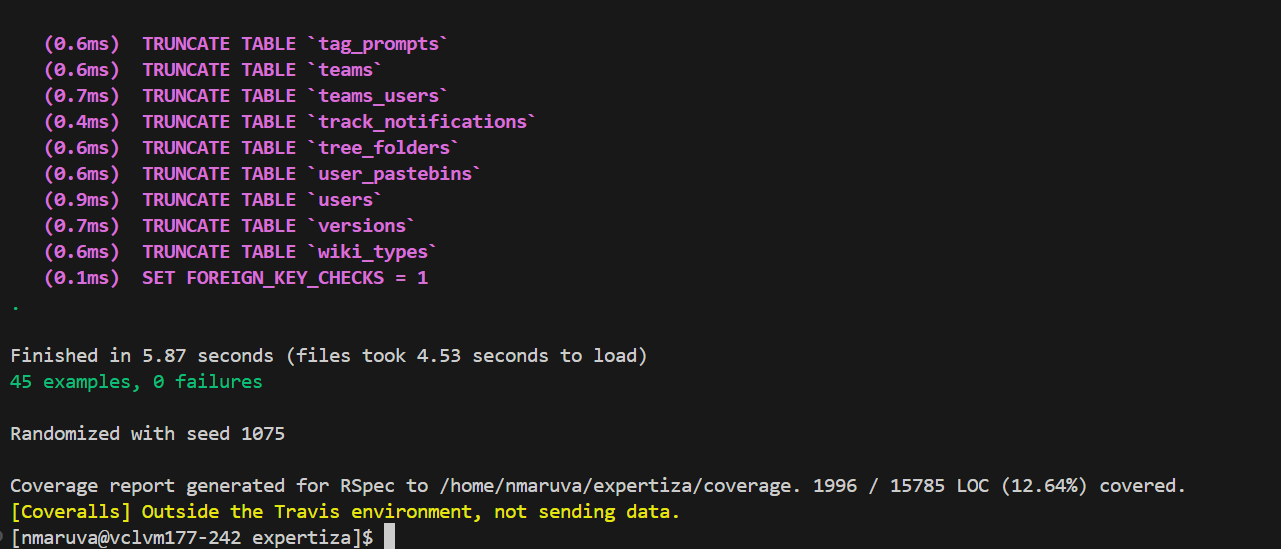
RSpec has been successfully applied to test the entire controllers directory, ensuring that all controller actions across the application are thoroughly tested. All test examples have passed without breaking any functionality, confirming the reliability and robustness of the controllers, with automated testing through GitHub Actions providing immediate feedback on code changes.

Team Information
Project Team
Mentor
- Anish Toorpu
Team Members
- Niharika Maruvanahalli Suresh
- Cristian Salitre
- Aditya Anand Kulkarni