CSC/ECE 517 Spring 2023 -: Difference between revisions
(3 intermediate revisions by the same user not shown) | |||
Line 12: | Line 12: | ||
The main goal of this project is to reimplement essential Ruby on Rails model | The main goal of this project is to reimplement essential Ruby on Rails model classes—SignedUpTeam and ProjectTopic (renamed from SignUpTopic)—from the Expertiza repository in a new repository. Each of these model classes plays a critical role in managing topics and team sign-ups within course assignments, and the reimplementation aims to bring them into alignment with Rails best practices and the DRY (Don't Repeat Yourself) principle. | ||
The | The SignedUpTeam model represents a team that has signed up for a specific topic within an assignment. This model manages team-specific data and oversees interactions that occur during the sign-up process. The ProjectTopic model, on the other hand, represents the topics available for sign-up within an assignment, managing essential details like slot availability and assigning teams to open topics. | ||
Since this project is being implemented from scratch, a range of components have been developed to ensure the functionality is both streamlined and maintainable. To guarantee the reliability of each part of the reimplementation, unit test cases have been created to verify the integrity of the new design and implementation. | Since this project is being implemented from scratch, a range of components have been developed to ensure the functionality is both streamlined and maintainable. To guarantee the reliability of each part of the reimplementation, unit test cases have been created to verify the integrity of the new design and implementation. | ||
Line 22: | Line 22: | ||
* Comprehensive RSpec tests are essential for all reimplemented models to ensure correctness and reliability in functionality. | * Comprehensive RSpec tests are essential for all reimplemented models to ensure correctness and reliability in functionality. | ||
* Update all references from ` | * Update all references from `sign_up_topics` to `project_topics` throughout the codebase to maintain consistency with the new naming convention. | ||
* Address all code review feedback to improve code quality and alignment with best practices. | * Address all code review feedback to improve code quality and alignment with best practices. | ||
* Refactor methods with readability issues to make the code more maintainable and easier to understand. | * Refactor methods with readability issues to make the code more maintainable and easier to understand. | ||
Line 32: | Line 30: | ||
* Optimize ambiguous class and instance methods to enhance reusability and improve the overall structure of the code. | * Optimize ambiguous class and instance methods to enhance reusability and improve the overall structure of the code. | ||
* The | * The old codebase has several instances of unnecessary calls to database, such as fetching all project topics for a user when fetching first topic would be sufficient. This effects efficiency and readability. Optimize such instances of code to streamline and increase readability. | ||
== Design Strategy == | == Design Strategy == | ||
* Implemented three model classes: `sign_up_team.rb` | * Implemented three model classes: `sign_up_team.rb` and `project_topic.rb` to address project requirements. | ||
* Each model now has a focused role, improving maintainability and clarity. | * Each model now has a focused role, improving maintainability and clarity. | ||
* In signed_up_team.rb, the following functionality are being implemented:- | * In signed_up_team.rb, the following functionality are being implemented:- | ||
Line 76: | Line 69: | ||
| sign_up_team || Implemented || Manages the sign-up process for a team, checking slot availability, assigning the topic, or placing the team on the waitlist as needed | | sign_up_team || Implemented || Manages the sign-up process for a team, checking slot availability, assigning the topic, or placing the team on the waitlist as needed | ||
|} | |} | ||
== E/R Diagram == | == E/R Diagram == | ||
[[File:oodd.png |500px| | [[File:oodd.png |500px|center]] | ||
<p style="text-align: center;">Edit Button Tooltip</p> | <p style="text-align: center;">Edit Button Tooltip</p> | ||
Latest revision as of 02:30, 30 October 2024
Team Members
- Ajith Kanumuri
- Suhas Adidela
- Ravi Chandu Bollepalli
- Mentor - Dinesh Pasupuleti
- PR Request:- https://github.com/expertiza/reimplementation-back-end/pull/125
- Project Board:- https://github.com/users/ajith05/projects/1/views/1
Project Description
The main goal of this project is to reimplement essential Ruby on Rails model classes—SignedUpTeam and ProjectTopic (renamed from SignUpTopic)—from the Expertiza repository in a new repository. Each of these model classes plays a critical role in managing topics and team sign-ups within course assignments, and the reimplementation aims to bring them into alignment with Rails best practices and the DRY (Don't Repeat Yourself) principle.
The SignedUpTeam model represents a team that has signed up for a specific topic within an assignment. This model manages team-specific data and oversees interactions that occur during the sign-up process. The ProjectTopic model, on the other hand, represents the topics available for sign-up within an assignment, managing essential details like slot availability and assigning teams to open topics.
Since this project is being implemented from scratch, a range of components have been developed to ensure the functionality is both streamlined and maintainable. To guarantee the reliability of each part of the reimplementation, unit test cases have been created to verify the integrity of the new design and implementation.
Issue Description
- Comprehensive RSpec tests are essential for all reimplemented models to ensure correctness and reliability in functionality.
- Update all references from `sign_up_topics` to `project_topics` throughout the codebase to maintain consistency with the new naming convention.
- Address all code review feedback to improve code quality and alignment with best practices.
- Refactor methods with readability issues to make the code more maintainable and easier to understand.
- Optimize ambiguous class and instance methods to enhance reusability and improve the overall structure of the code.
- The old codebase has several instances of unnecessary calls to database, such as fetching all project topics for a user when fetching first topic would be sufficient. This effects efficiency and readability. Optimize such instances of code to streamline and increase readability.
Design Strategy
- Implemented three model classes: `sign_up_team.rb` and `project_topic.rb` to address project requirements.
- Each model now has a focused role, improving maintainability and clarity.
- In signed_up_team.rb, the following functionality are being implemented:-
Method Name | Status | Description |
---|---|---|
drop_off_team_waitlists | Implemented | Removes the team from all waitlists for topics within the assignment. |
find_first_existing_sign_up | Implemented | Finds the first existing sign-up entry for a given topic and team, checking for both active and waitlisted sign-ups. |
create_signed_up_team | Implemented | Creates a SignedUpTeam instance with specified topic_id and team_id if the topic is available for sign-up. |
get_team_id | Implemented | Retrieves the team ID associated with a given SignedUpTeam entry. |
delete_signed_up_team | Implemented | Deletes the signed-up team entry for a specific topic, managing any dependent records as necessary. |
- Add necessary schema migrations to rename signup topic to project topic.
- In project_topic.rb, the following functionality are being implemented:-
Method Name | Status | Description |
---|---|---|
slot_available? | Implemented | Checks if there are available slots for the topic based on the maximum allowed choosers. Returns true if available. |
assign_topic_to_team | Implemented | Assigns the topic to a team by updating the sign-up entry to set is_waitlisted as false and assign the topic_id. |
save_waitlist_entry | Implemented | Saves a sign-up entry as a waitlist entry by setting is_waitlisted to true and assigning the topic_id. |
sign_up_team | Implemented | Manages the sign-up process for a team, checking slot availability, assigning the topic, or placing the team on the waitlist as needed |
E/R Diagram
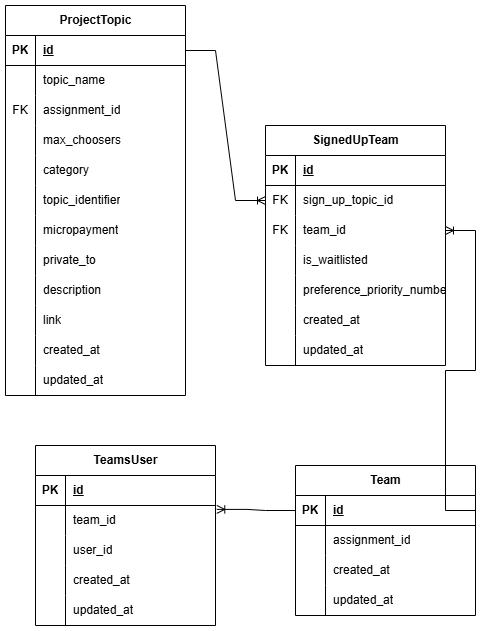
Edit Button Tooltip
Test Plan
- Upgrade RSpec test cases for `signed_up_team.rb` to validate `delete_signed_up_team` and `get_team_participants` methods.
- Enhance and expand RSpec test cases for `project_topic.rb` to validate participant retrieval, slot availability, and assignment status handling.
- Develop RSpec test cases for `waitlist.rb` to validate adding teams to the waitlist, removing teams from the waitlist, and querying the waitlist status of a team.
- Add general RSpec test cases for error handling and ensure correct JSON serialization of data..
- Test Cases for signed_up_team.rb model
- Test Cases for waitlist.rb model
- Test Cases for signup_topic.rb