CSC/ECE 517 Spring 2024 - E2431. Reimplement grades/view team
Introduction
Expertiza, a learning management system available as open-source software, utilizes the Ruby on Rails framework as its foundation. Its features encompass the creation of assignments, tests, assignment teams, and courses, among others. Particularly noteworthy is its comprehensive system designed to facilitate peer reviews and feedback within teams and groups. The primary focus of this project lies in developing frontend React Components, specifically targeting User, Institution, and Roles functionalities. The objective is to create a fully operational user interface for these components using React.
To Do
The main objective of this project is to redesign the front end for the grades/view_team page within Expertiza. The current layout suffers from performance issues and outdated design, resulting in decreased usability. Our aim is to improve user experience and interface efficiency by developing a refreshed front end using React JS and TypeScript. The project will concentrate on several key features including displaying team information such as names and assignment details, listing reviews and their comments/feedback for each question while ensuring consistency, indicating review scores with color-coding(Heat map) from green to red.
Design Patterns
In the re-implementation of Expertiza's front end, we have utilized several design patterns to enhance maintainability and promote code reusability:
1. Composite Design Pattern: We employ the Composite Design Pattern by nesting and composing components like Table, Modal, and others to construct our Course component effectively.
2. DRY Principle: Adhering to the DRY Principle, we leverage React's capabilities to reuse existing components such as Table and Modal, thereby minimizing redundancy in our codebase.
3. Provider Pattern: Using React's Context API, we implement the Provider Pattern to seamlessly pass props down the component tree without explicit prop drilling.
4. Observer Pattern: We harness the Observer Pattern using React's Context API alongside hooks like useEffect and useState to efficiently manage component state and side effects.
5. HOC Pattern: The HOC Pattern, or Higher-Order Component pattern, is adopted to facilitate routing for new pages by creating functions that take in a component and return a modified component with routing capabilities.
6. Mediator Pattern: Given the disparity between backend data structures and frontend requirements, we employ the Mediator Pattern to transform data as needed, ensuring compatibility and coherence within our application.
Changes Intended
- Assignment:
This component will be used to display all the assignments available. We will make sure that the route /assignment will list all the assignments in a tabular format as shown in the previous designs in the next section. Along with this list, there will be options to perform CRUD operations. For assignments, we will have the options to edit and delete a particular assignment. Also, there will be an option to create a new assignment.
Files Added
We created an assignment folder inside the pages folder which includes the essentials for the implementation of all the changes mentioned in the previous sections.
# | Change | Rationale |
---|---|---|
1 | App.tsx | Added code that sets up a React component (Assignments) to manage and display assignments. It handles data fetching from APIs, transforms and merges data, configures a dynamic table, and provides user interactions for editing and deleting assignments. Additionally, it includes error handling and logging for debugging purposes. |
2 | ReviewTable.tsx | This TypeScript file defines interfaces and transformation functions related to handling assignments. The IAssignmentRequest and IAssignmentResponse interfaces outline the expected structure for sending and receiving assignment data from the server. The transformAssignmentRequest function converts an IAssignmentRequest object into a JSON string, for sending data to the server. Conversely, the transformAssignmentResponse function parses a string representing an assignment response from the server into an IAssignmentResponse object. These utilities facilitate the communication and data transformation processes when working with assignments. |
3 | ReviewTableRow.tsx | This component is a central interface for creating and updating assignments. It employs Formik for efficient form handling, featuring fields for assignment details. The component adapts to different modes, enabling smooth creation or modification of assignments. Integrated with Redux for state management, it ensures responsive user feedback and streamlined error handling. This component is pivotal in managing the user interaction, form validation, and API communication for assignment-related tasks. |
4 | RoundSelector.tsx | Created the DeleteAssignment component that encapsulates the logic and UI elements required for a confirmation modal when deleting assignments. It communicates with the API, handles errors, and provides a clear and user-friendly interface for the deletion process. |
5 | ShowSubmission.tsx | In app.tsx, we also added a protected route for displaying assignments, where only users with a role of TA or higher can access. Additionally, it defines nested routes for creating and editing assignments using the AssignmentEditor component, with a loader function to handle data loading before rendering. |
6 | dummyData.ts | In app.tsx, we also added a protected route for displaying assignments, where only users with a role of TA or higher can access. Additionally, it defines nested routes for creating and editing assignments using the AssignmentEditor component, with a loader function to handle data loading before rendering. |
7 | grades.css | In app.tsx, we also added a protected route for displaying assignments, where only users with a role of TA or higher can access. Additionally, it defines nested routes for creating and editing assignments using the AssignmentEditor component, with a loader function to handle data loading before rendering. |
8 | utils.ts | In app.tsx, we also added a protected route for displaying assignments, where only users with a role of TA or higher can access. Additionally, it defines nested routes for creating and editing assignments using the AssignmentEditor component, with a loader function to handle data loading before rendering. |
Designs
Following designs are that of the users component which has already been done. This project aims to replicate these designs for the components that we will be creating as mentioned in the previous section.
Display
The following design for users shows the tabular format in which the users are displayed. For our new components, we will be replicating this tabular format with the headers depending on the component.
[File:Display.png|800px|center]]
Existing GradesView Page
The following design for users shows the modal to create a new user.
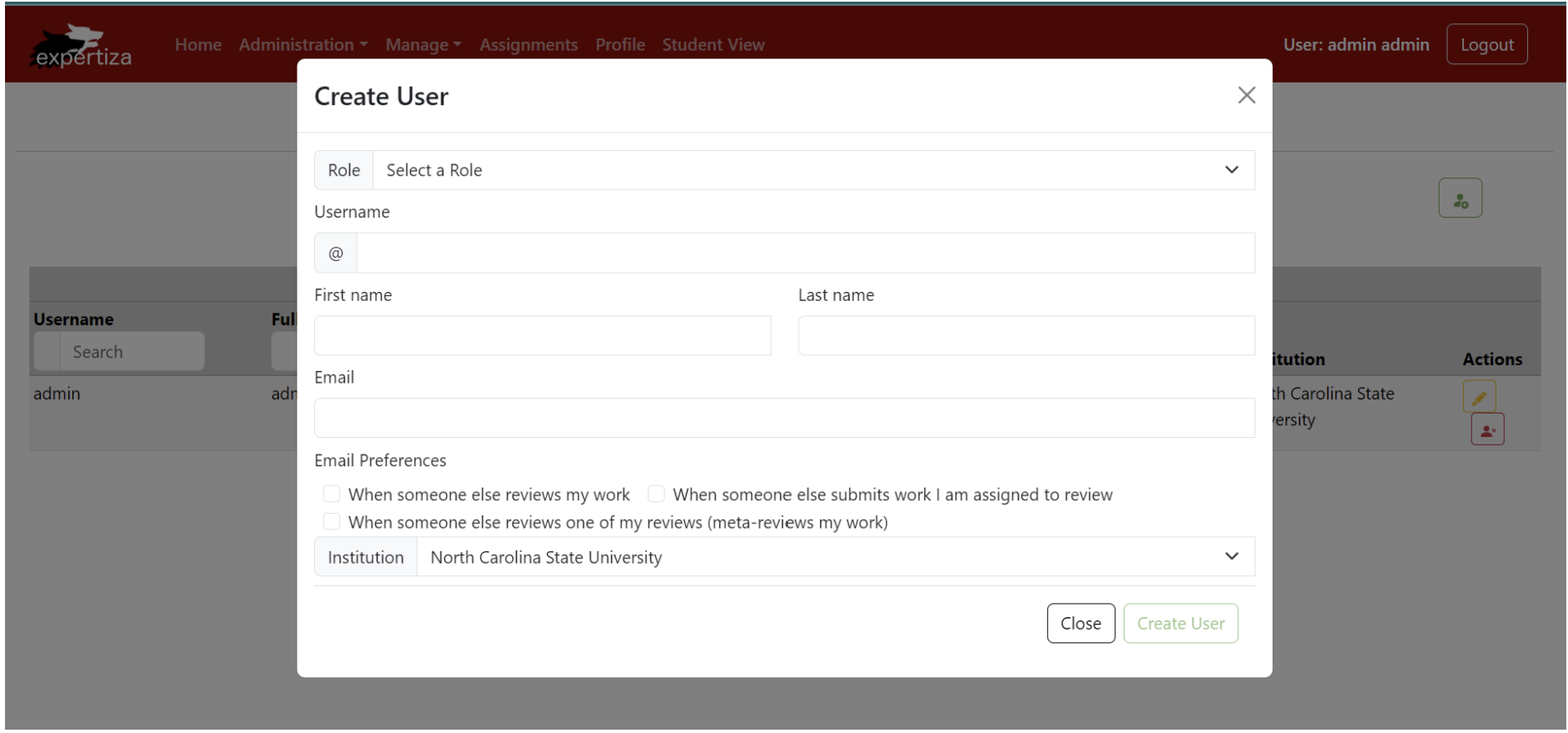
Reimplementation Done
The following are the snapshots of the functionality we tried to implement by leveraging the power of ReactJS and Typescript.
Displaying Assignments
Our aim was to display relevant information while listing out the assignments on this page.
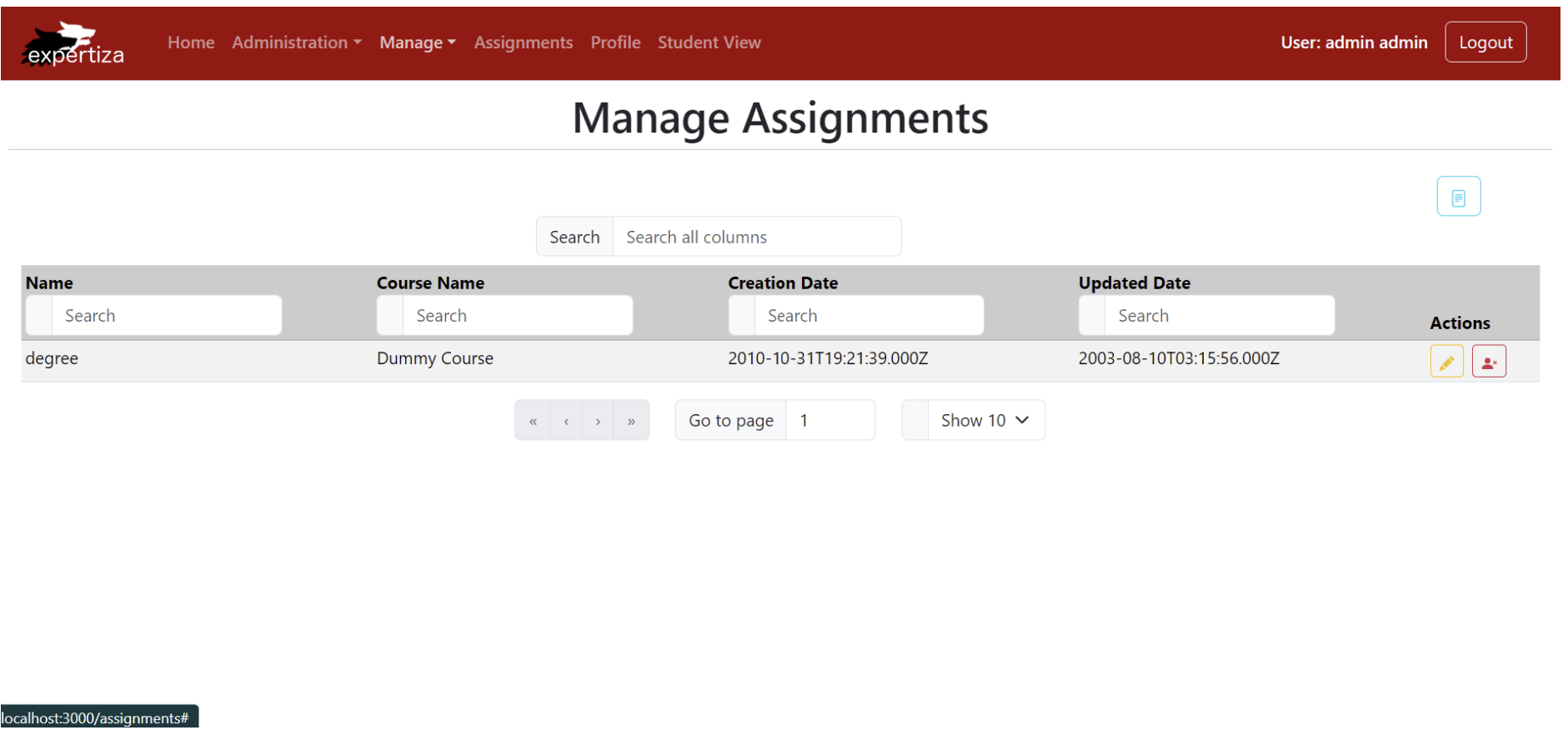
Creating Assignments

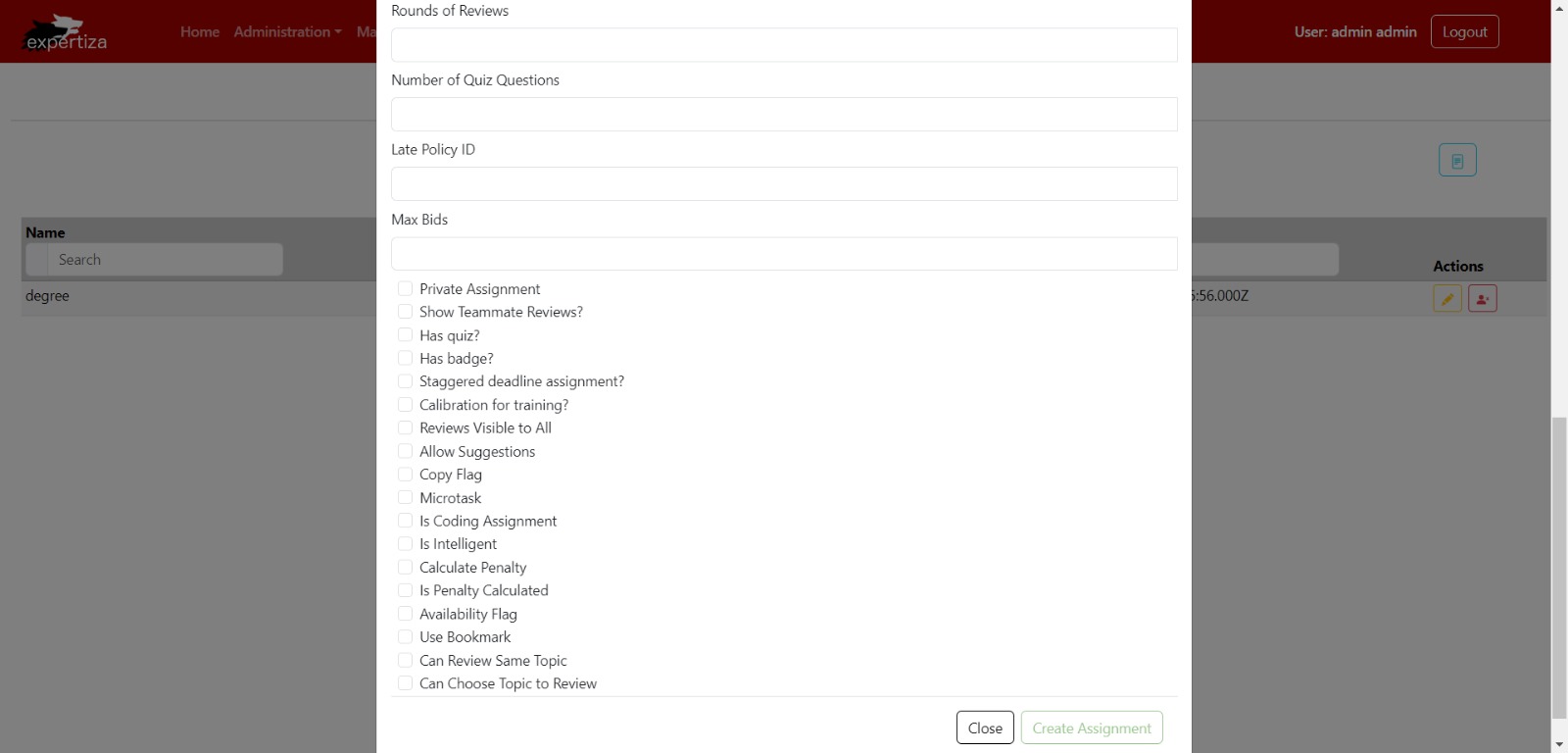
Updating Assignments


These design patterns play a pivotal role in ensuring the scalability, maintainability, and extensibility of our front-end implementation for Expertiza.
Dummy Data
The purpose of the dummy data is test the functionality of our frontend. For this purpose we have chosen JSON data as a template for test data, the future team working on the backend can delete this file if the data can be successfully retrieved from the database.
JSON Files
JSON files can be found here [Link]
{ "team": "Straw Hat Pirates", "members": ["Chaitanya Srusti", "Nisarg Nilesh Doshi", "Aniruddha Rajnekar", "Malick, Kashika"], "grade": "Grade for submission", "comment": "Comment for submission", "late_penalty": 0 }
[ [ { "questionNumber": "1", "questionText": "What is the main purpose of this feature?", "reviews": [ { "score": 4, "comment": "Great work on this aspect!" }, { "score": 3, "comment": "Could use some improvement here." }, { "score": 4, "comment": "The presentation was well-organized and clear. However, some points could have been elaborated further to provide a deeper understanding of the topic." }, { "score": 5, "comment": "The speaker demonstrated a profound understanding of the subject matter, making the session engaging and informative." }, { "score": 4, "comment": "The visuals were compelling and helped in understanding complex concepts easily. However, there were a few slides with too much text, which made it hard to follow at times." }, { "score": 5, "comment": "The use of real-world examples made the concepts more relatable and easier to grasp. Additionally, the speaker was engaging and kept the audience hooked throughout." }, { "score": 4, "comment": "The interactive exercises were beneficial in reinforcing the learning. However, there were a few technical glitches that disrupted the flow." }, { "score": 5, "comment": "The hands-on activities were the highlight of the session, providing practical experience that complemented the theoretical learning." }, { "score": 4, "comment": "The guest speaker brought a fresh perspective to the topic, offering valuable insights that sparked further discussions among the participants." }, { "score": 5, "comment": "The case studies presented were enlightening, providing practical examples that showcased the application of theoretical concepts." } ], "RowAvg": 0, "maxScore": 5 }, // Add more question as needed ..... ], [ { "questionNumber": "1", "questionText": "What is the main purpose of this feature?", "reviews": [ { "score": 4, "comment": "The design is polished and professional." }, { "score": 5, "comment": "Certain design elements make the feature a joy to use." }, { "score": 3, "comment": "Some design aspects could benefit from refinement." }, { "score": 4, "comment": "The design is user-friendly, with intuitive navigation." }, { "score": 5, "comment": "Certain design elements enhance user interaction effectively." }, { "score": 4, "comment": "Some design aspects may require further attention." }, { "score": 4, "comment": "The design encourages exploration and discovery." }, { "score": 5, "comment": "The feature's design sets a new standard for user interfaces." }, { "score": 4, "comment": "Certain design elements are confusing and could be clarified." }, { "score": 4, "comment": "The design offers an inviting and engaging experience." } ], "RowAvg": 0, "maxScore": 5 }, // Add more rounds as needed... ], // Add more rounds as needed... ]
Project Mentor
Kashika Mallick (kmallick@ncsu.edu)
Team Members
Aniruddha Rajnekar (aarajnek@ncsu.edu)
Chaitanya Srusti (crsrusti@ncsu.edu)
Nisarg Doshi (ndoshi2@ncsu.edu)