CSC/ECE 517 Spring 2022 - E2228. Refactor JavaScript on Expertiza
Problem Statement
Refactor JavaScript on Expertiza for but not limited to the Assignment View. There is javascript code (pure and embedded with ruby) in a lot of .html.erb files in the entire Expertiza application. The main aim of this project is to separate this javascript code into different .js files in order to make the code clean and legible.
Background
Expertiza is a software project that uses peer review to produce reusable learning items. It also allows for the uploading of practically any document type, including URLs and wiki pages, as well as team projects. Expertiza's primary language for managing views is JavaScript. However, these scripts are not well structured and present a large scope of improvement in terms of code quality and optimization. There is javascript code which is scattered in different files all over the project. Also there are files which contain huge functions with several lines of code which violates the DRY principles and also leads to Code smells.
Files to be modified
The files that we modified for this project are as follows. These files can be found in views with the path as -
- assignments/edit/_due_dates.html.erb
- assignments/edit/_rubrics.html.erb
- assignments/edit/_general.html.erb
- assignments/edit.html.erb
Issues
1. Code comment and fix indentation
All files under consideration are poorly indented and commented. Unindented HTML code may contain bugs that are difficult to trace and make the code less readable. Additionally, it becomes hard to identify repeating codes and enforce DRY. Uncommented code makes it extremely difficult for new programmers to get hang of the application and its components. A snapshot of the existing code to illustrate this issue is shown below:

2. Ensure DRY is enforced
This requires that repeating code is put in functions. There are many places throughout the code where this principle is being violated. Repeated code reduces the quality of the code, increases the file size and makes the program run slower.A snapshot of the existing code to illustrate this issue is shown below:
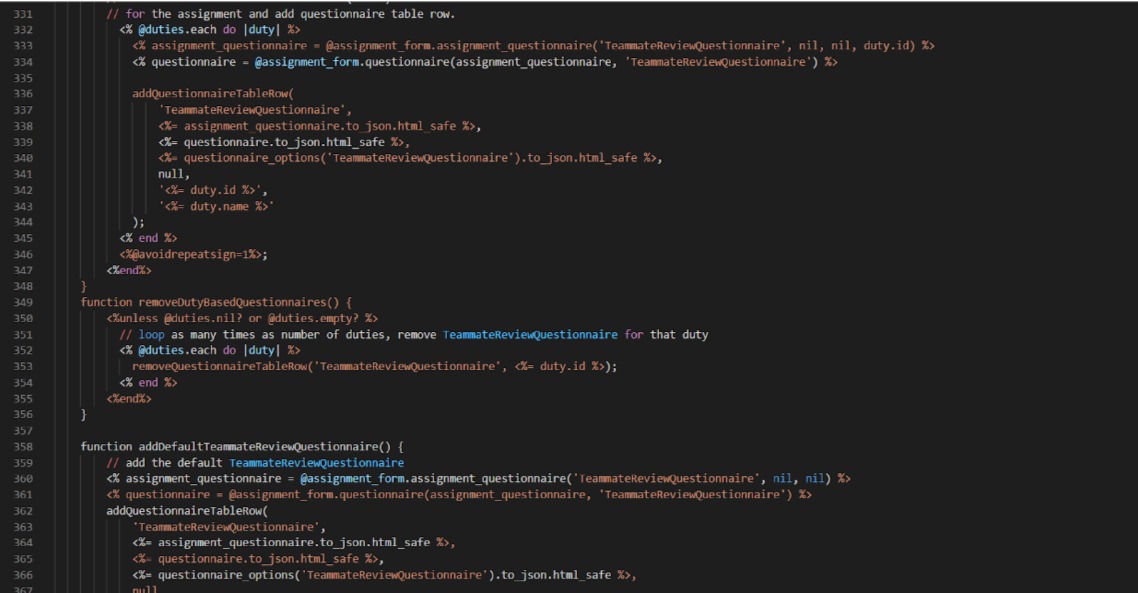
3. Isolate Javascript
For Javascript methods that do not use any erb tags, we isolate them and keep them in a separate file. This is done since we do not need embedded ruby in the Javascript function and the logic can be separated out. Performing this fix makes the code more readable and easier to handle as well as understand. A snapshot of the existing code to illustrate this issue is shown below:
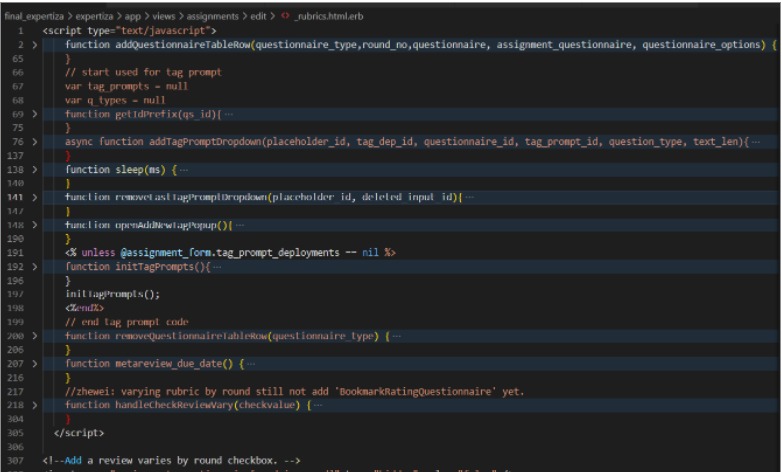
4. Violating Single Responsibility for each function.
Split big functions into smaller functions to ensure Single Responsibility for each function. There are many tasks that this function is performing which can be broken down into smaller functions that perform a single task. This will also help us write test cases for each function which will improve the code quality. A snapshot of the existing code to illustrate this issue is shown below:

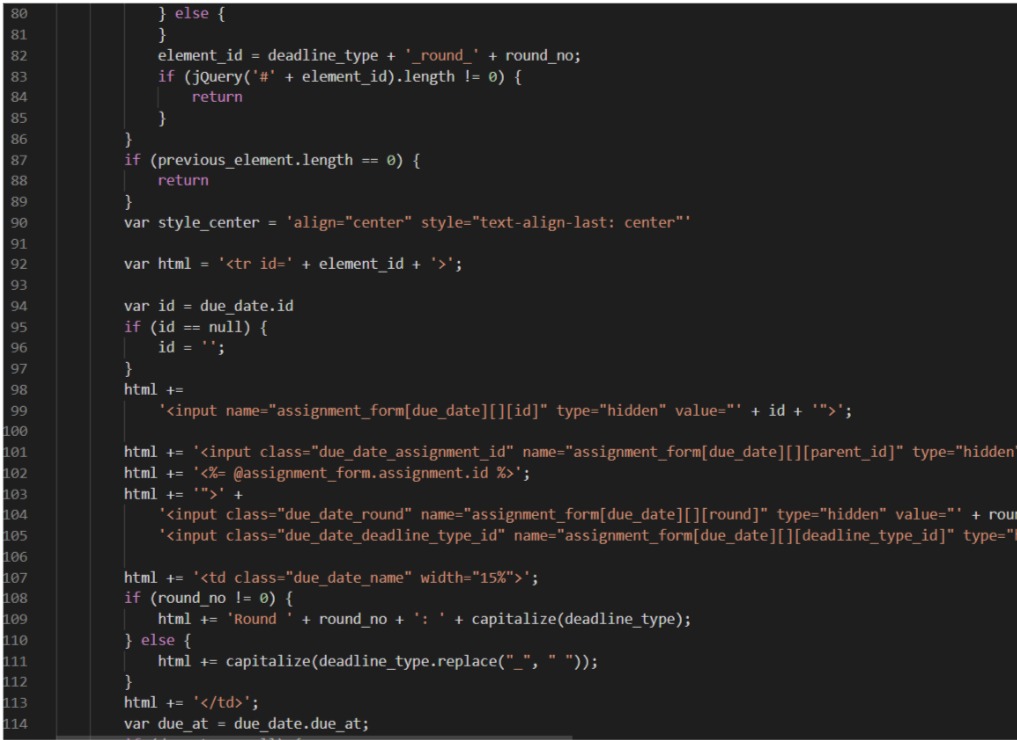
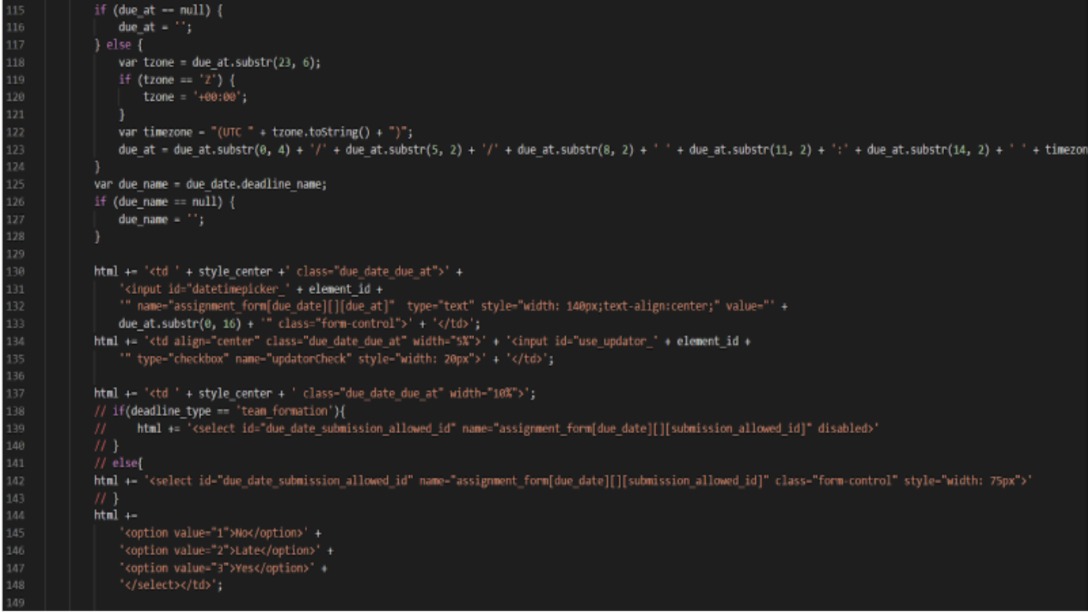
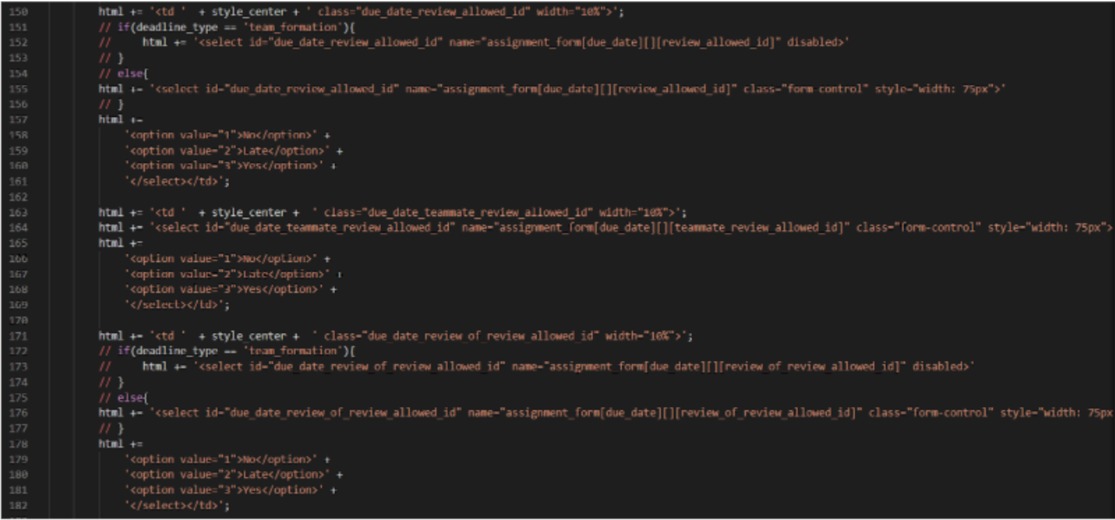
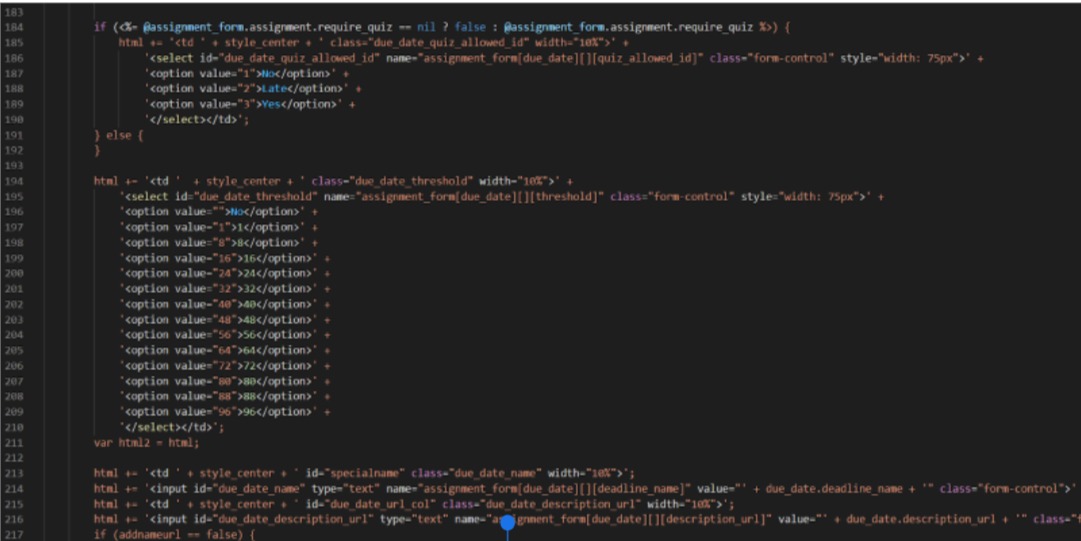
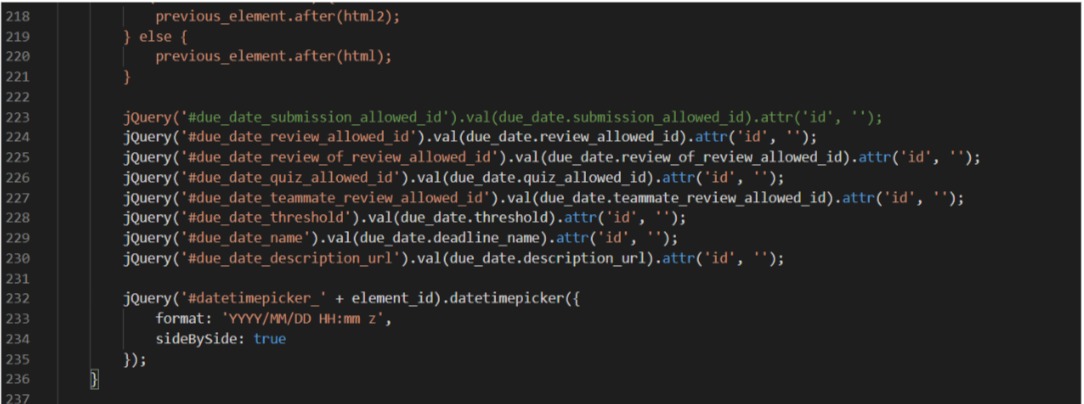
5. Have css in separate files
The CSS code is cluttering erb files. The CSS code should be moved into a different file so that in future if there are any changes to be made in the UI which will need the CSS code to be modified, it will not affect the main erb files. A snapshot of the existing code to illustrate this issue is shown below:
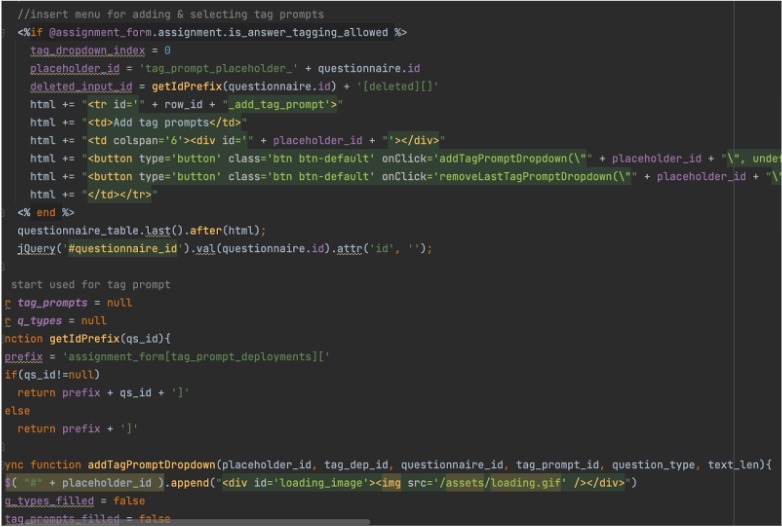
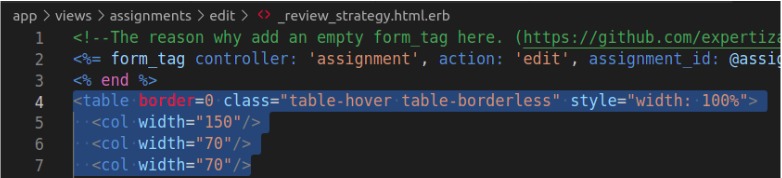
6. Remove unused and useless code
Instances of unused code found in some files. Removing code blocks which have no code in them. A snapshot of the existing code to illustrate this issue is shown below:
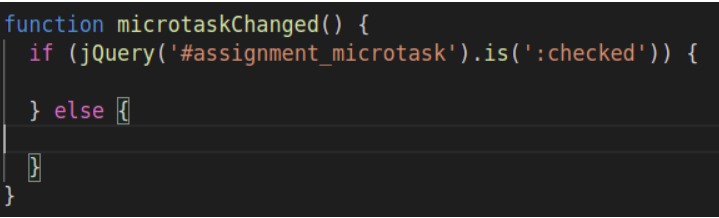
7. Refactor variable, function and class names
Variables, classes and functions have been defined using names that do not explain their functionalities in full capacity.A snapshot of the existing code to illustrate this issue is shown below:

Plan of Action
After analyzing the problem statement along with the background of the project we came up with the following plan of action:
- Move javascript code from the html.erb files into separate js files. This is to ensure that the code is clean and legible to analyse. Putting javascript in separate js files helps in avoiding code smells.
- Remove any unnecessary/unused functions from the code, ensuring that it doesn't affect the functionality of the code. This is to ensure that the code doesn't slow down because of any unused functionality getting executed at runtime.
- Refactor the existing methods to ensure that it follows DRY, ensure functions are modular and follow Single Responsibility which stipulates that a method or function should only be used for one reason and thereby increases code reuse.
- Provide descriptive comments for each method as well as make code readable by adding proper indentation. This is done in order to make the code legible
- Fix any indentation errors in the code. This is to ensure that the code is clean.
Goals Acheived
- Pure js functions have been moved to assests/javascripts folder.
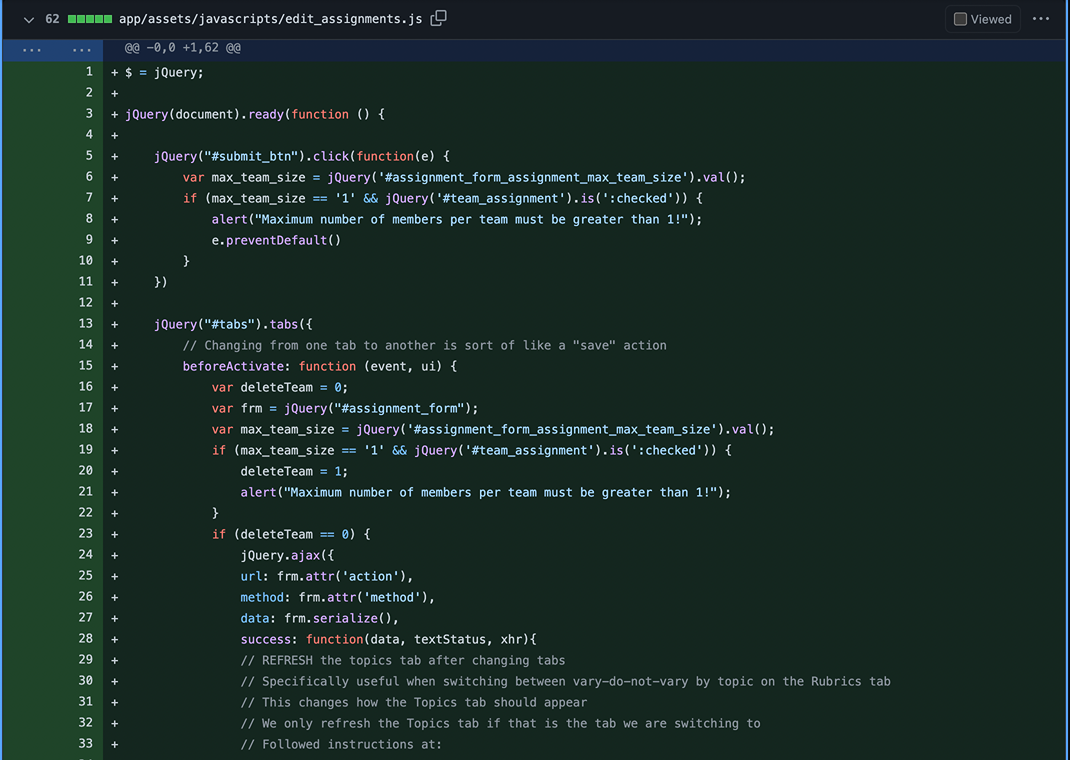
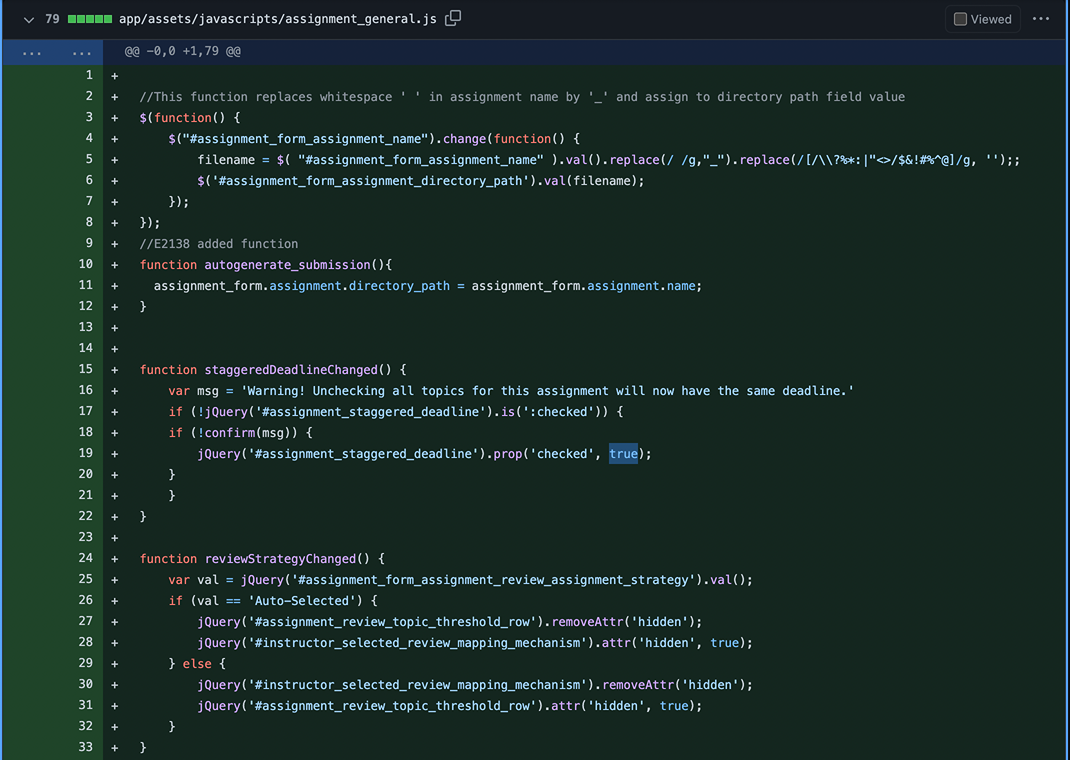
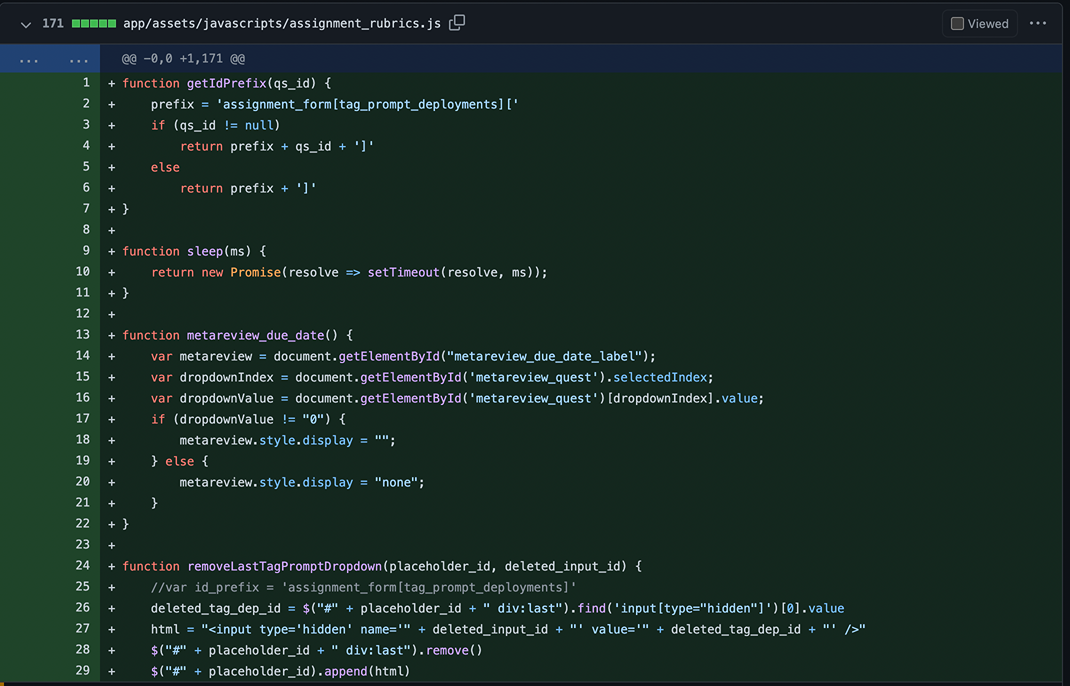
- Unused functions that have been declared but not used anywhere have been removed.
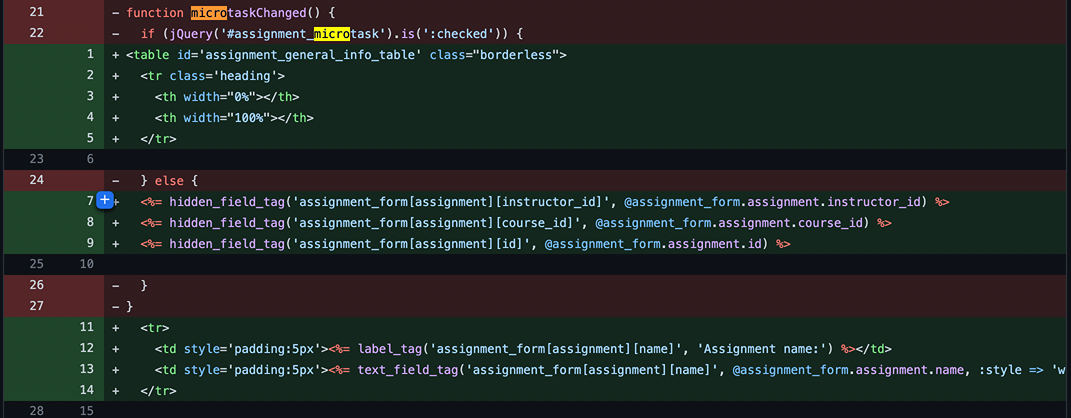
- The indentations have been fixed and comments have been added.
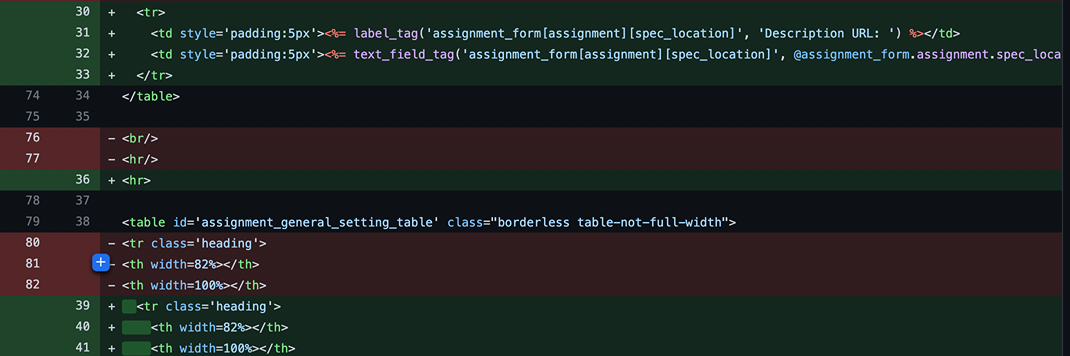
- The code containing CSS script has been moved.
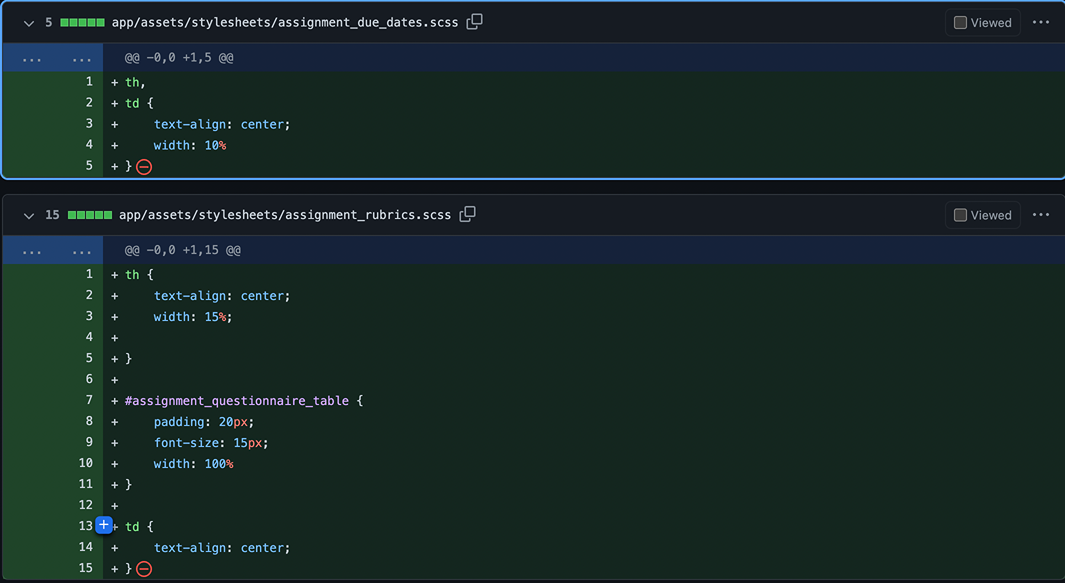
Roadblocks
- The JavaScript functions take in embedded ruby content as variables. This ruby content is actually ruby objects which are accessing database content. Copying these into JavaScript is not possible, because the changes made on these JavaScript variables will not reflect on the database, as we desire (since we are working on "edit" action).
- Secondly, Javascript is client side and Ruby is server side. Converting variables from language to another will add to network load, as this requires a client-server communication, and impact the performance.
- Repeating codes could not be converted into callable functions because these repeating code lines have javascript and ruby variables intermingling in the javascript function. Hence it has been left as is.
Testing Plan
This project was a refactoring project where we had to separate JavaScript code from html.erb files. All the JS code was used to render the frontend which is why there is no test cases possible.
Conclusion
Following is the summary that we could conclude:
- We analyzed the entire codebase and identified potential problems with the JavaScript code.
- Pure javascript code can be moved into a completely new file and can be called from the .html.erb files
- We need to find a way to separate the javascript code embedded with ruby into different files.
- Refactoring this code might not improve the performance as converting variables from one language to another will add to network load.
Useful Links
- Wiki page link: https://expertiza.csc.ncsu.edu/index.php/CSC/ECE_517_Spring_2022_-_E2228._Refactor_JavaScript_on_Expertiza
- Github pull request: https://github.com/expertiza/expertiza/pull/2404
- Forked repository link: https://github.com/KrishnaSatya10/expertiza/tree/beta
- https://go.ncsu.edu/e2228-refactor-javascript-on-expertiza