CSC/ECE 517 Spring 2022 - E2228. Refactor JavaScript on Expertiza
Problem Statement
Refactor JavaScript on Expertiza for but not limited to the Assignment View
Background
Expertiza is a software project that uses peer review to produce reusable learning items. It also allows for the uploading of practically any document type, including URLs and wiki pages, as well as team projects.Expertiza’s primary language for managing views is JavaScript. However, these scripts are not well structured and present a large scope of improvement in terms of code quality and optimization.
Files to be modified
- assignments/edit/_due_dates.html.erb
- assignments/edit/_rubrics.html.erb
- assignments/edit/_general.html.erb
- assignments/edit.html.erb -> 76 lines of JS
What needs to be done
- Most JavaScript functions are present as part of erb files. The erb files that use JavaScript but do not use arguments that can be accessed by Ruby code have to be extracted and included as a JavaScript partial.
- For functions that utilize JavaScript and Ruby code, we have two choices
- Refactor the existing methods to ensure that it follows DRY, ensure functions are modular and follow Single Responsibility. - Use an npm package called erb to inject erb code into JavaScript code.
- Optimize all the code so that they follow DRY principles.
- Documentation for each method and proper indentation is absent. Our aim would be to provide descriptive comments for each method as well as make code readable by properly indentation.
- Introduce the Single Responsibility Principle, which stipulates that a method or function should only be used for one reason and thereby increases code reuse.
- Identify and remove functions do not serve any purpose as well as remove unnecessary documentation/methods that have been commented out.
- Change deprecated JavaScript syntax to ES6 syntax.
Issues and potential fixes
1. Code comment and fix indentation
ISSUE: All files under consideration are poorly indented and commented. Unindented HTML code may contain bugs that are difficult to trace and make the code less readable. Additionally, it becomes hard to identify repeating codes and enforce DRY. Uncommented code makes it extremely difficult for new programmers to get hang of the application and its components.

Indent codes and add comments might fix this problem.
2. Move all ajax requests into a separate function.
In the files under consideration, AJAX have been embedded inside Javascript functions, which in-turn have been defined in erb files. Although the code is functional, it adds needless complexity and clutters erb files with Javascript, JQuery, AJAX and HTML code snippets. A snapshot of the existing code to illustrate this issue is shown below:
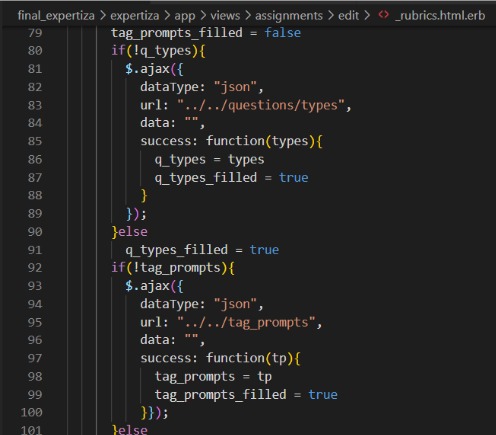
The AJAX script can be defined in functions that can take the request params such as dataType, url, data, and returns. Since most AJAX queries have similar structure, these functions can be reused for different conditionals by passing relevant parameters. This fix makes code simpler, manageable, replicable and most importantly, DRY.
3. Ensure DRY is enforced
ISSUE: This requires that repeating code is put in functions. There are many places throughout the code where this principle is being violated. Repeated code reduces the quality of the code, increases the file size and makes the program run slower.
4. Isolate Javascript
ISSUE: For Javascript methods that do not use any erb tags, we isolate them and keep them in a separate file. This is done since we do not need embedded ruby in the Javascript function and the logic can be separated out. Performing this fix makes the code more readable and easier to handle as well as understand.
Fix: As can be seen in the code snippet above, all the javascript functions have been included in the .html.erb file. These can be moved to a separate directory, for instance app/assets/javascript/
5. Violating Single Responsibility for each function.
ISSUE: Split big functions into smaller functions to ensure Single Responsibility for each function. There are many tasks that this function is performing which can be broken down into smaller functions that perform a single task. This will also help us write test cases for each function which will improve the code quality.
6. Have css in separate files
ISSUE: The CSS code is cluttering erb files. The CSS code should be moved into a different file so that in future if there are any changes to be made in the UI which will need the CSS code to be modified, it will not affect the main erb files.
7. Remove unused and useless code
Instances of unused code found in some files. Removing code blocks which have no code in them.
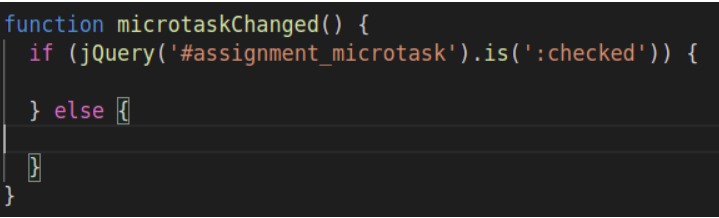
8. Refactor variable, function and class names
ISSUE: Variables, classes and functions have been defined using names that do not explain their functionalities in full capacity.

9. Code comment and fix indentation
ISSUE: All files under consideration are poorly indented and commented. Unindented HTML code may contain bugs that are difficult to trace and make the code less readable. Additionally, it becomes hard to identify repeating codes and enforce DRY. Uncommented code makes it extremely difficult for new programmers to get hang of the application and its components.