CSC/ECE 517 Spring 2017 E1734: Difference between revisions
Line 234: | Line 234: | ||
[[File:FILE01.jpg]] | [[File:FILE01.jpg]] | ||
== Tasks | == Tasks performed == | ||
The following list provides a summary of the tasks we have performed to implement the survey functionality. | |||
1) Change the hierarchical structure of classes. Make survey questionnaire the child of questionnaire. Global survey questionnaire, Assignment survey questionnaire and course survey questionnaire are subclasses of survey questionnaire. | |||
1) Change the hierarchical structure of classes. Make survey the child of questionnaire. Global survey, | |||
2) Creation, editing and deletion of questions, is a functionality that would be inherited into class Survey(which is a kind of questionnaire) and so into all its children surveys, from the Questionnaire class. | 2) Creation, editing and deletion of questions, is a functionality that would be inherited into class Survey(which is a kind of questionnaire) and so into all its children surveys, from the Questionnaire class. | ||
3) Add new class Survey and include functionality to assign(which is in the survey_controller currently) to this model. | 3) Add a new class called Survey Questionnaire and include functionality to assign(which is in the survey_controller currently) to this model. | ||
4) In the | 4) In the survey deployment class, add functionality to generate statistics. | ||
5) The | 5) The three kinds of surveys can be assigned to students an assignment, a course, and a global survey which is appended to one of the other two surveys. | ||
6) | 6) Use the Pending Surveys (newly added) tab on the Expertiza homepage to display any pending surveys instead of just the course evaluation surveys. The course evaluation tab’s functionality was removed because it was broken, it was replaced with the pending surveys functionality. | ||
7 | 7) "Survey deployment" functionality was broken. We made survey deployment of all forms of surveys functional. It included the following tasks: | ||
a) Ability to add new survey deployments. | a) Ability to add new survey deployments. | ||
b) View responses to the deployments. | b) View responses to the deployments. | ||
c) View statistics (in the form of pie charts) | |||
c) Delete deployments. | c) Delete deployments. | ||
== Test Plan == | == Test Plan == |
Revision as of 20:27, 29 April 2017
Improve survey functionality
Problem Description
Expertiza used to have functionality for conducting surveys. However, the existing code is inefficient and needs to be improved. This functionality has rarely been used and so isn't tested very well either. Therefore, there are parts of survey functionality that are broken or are totally unfinished.
So, there are three major problems this project will tackle.
1) Restructure and move around functionality within classes to further streamline the code.
2) Test the existing functionality and catalog what parts work and what do not. As an instance of a broken feature, take survey deployments for example. During initial testing, it is not possible to deploy any kind of survey. There are other features as well that need little fixes to make them work.
3) Fixing the broken features. This involves little bug fixes here and there, as well as finishing the features that are completely unfinished.
Requirements
1) A page to show the distribution of results for all types of questionnaires.
2) Use of inheritance so that survey questionnaire is a subclass of the questionnaire class. Also, course evaluation questionnaire and global survey questionnaire should be subclasses of survey questionnaire.
3) Use response_controller to implement the survey functionality. This should be similar to how other questionnaires like the peer review questionnaire, are implemented.
4) Use the same code to display existing survey responses as to display the responses in peer reviews.
5) For each course or assignment, the admin/instructor should be able to create a survey and have some (or all) of the participants receive the survey. The instructor should have the option to not include some questions from a global survey. The quiz takers can take the global survey and course survey consecutively.
6) An entry in the participants table called a survey_participant record must be added to indicate when a user is assigned to take part in a survey.
7) Tests need to be written for the feature.
Note: Although the requirements document mentions that the existing code needs to be scrapped and rewritten, after discussion with professor it was decided that this is not a strict requirement.
Types of Surveys
There are three kinds of surveys available in the Expertiza application. They are differentiated based on the group of people that can have access to these surveys.
The surveys are as follows:
1) Course Survey:
All the participants in a course can take the course survey.
File: course_survey_questionnaire.rb
2) Global Survey:
Any Expertiza user can take the global survey. Appended to another survey.
File: global_survey_questionnaire.rb
3) Assignment Survey:
All the participants in an assignment can take the assignment survey.
File: assignment_survey_questionnaire.rb
Class Design
Existing class structure:
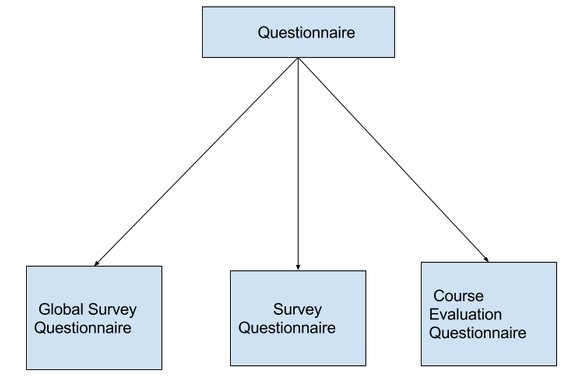
Updated class structure:
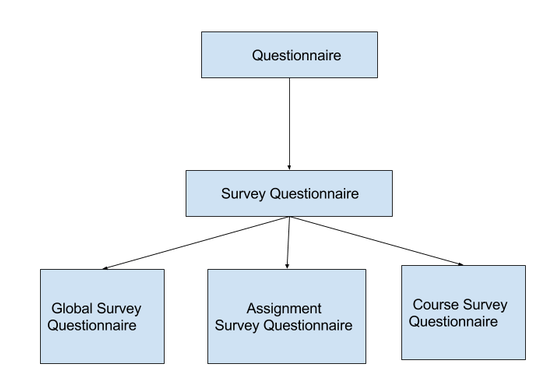
New Class Functionality:
Questionnaire:
Contains the basic information and methods required for posing questions to students or users in general. It is inherited by Classes Quiz, Survey, etc.
We can reuse this class's functionality to create, edit and delete surveys. The functionality for checking if questions have been answered can also be borrowed from this class.
Survey Questionnaire:
This project will introduce a new class called Survey Questionnaire.
This class will inherit from the Questionnaire class and it will be a superclass for the three different kinds of survey classes.
This class will include the assign_participants() method that can be used by any of the child classes to assign participants for their respective surveys.
This class will also have the statistics_generation functionality to be used to provide statistics for any kind of survey. This is the one that will take care of the requirement for getting the distribution of responses.
Global Survey Questionnaire:
This class will inherit from class Survey Questionnaire.
This class will be set the display_type for the survey to be global. So, while assigning participants all the users of Expertiza will be able to see this particular survey.
This kind of survey can only be created by admins of the Expertiza system.
Course Survey Questionnaire:
This is also another type of survey and will inherit from class Survey Questionnaire.
Surveys of this type, however, will only be available to the class to which this survey would be assigned.
That is, Course participants receive the course survey questionnaire.
Assignment Survey Questionnaire:
This class will also inherit from the class Survey Questionnaire.
It will allow for an association between an assignment and a questionnaire to be established. Additionally, it maintains the instructor id who will create the association.
A flag for a score outside an acceptable range will also be set. It could also be used to play a part in the total score calculation for the assignment.
All participants in the assignment receive the assignment survey questionnaire.
Database Schema
There are database tables that are used to track the deployment of the surveys, that are used to help keep track of the participants for each survey and that keep track of all the responses for the surveys we create. We would not be making any or would make only minimal design changes to these tables. Our main task would be to go in and fix things that are not currently operational given what we already have.
To give an idea about these database tables we have included them below.
Table: Survey Deployments
Element | Type | Attributes |
---|---|---|
course_evaluation_id | Integer | Limit: 4 |
start_date | Datetime | |
end_date | Datetime | |
num_of_students | Integer | Limit: 4 |
last_reminder | Datetime | |
course_id | Integer | Limit: 4, Default: 0, Null: false |
Table: Response_Maps
To incorporate the survey functionality, we have made the following mappings:
REVIEWED_OBJECT_ID corresponds to COURSE_ID / ASSIGNMENT_ID
REVIEWER_ID corresponds to PARTICIPANT_ID ( id in Participants table )
REVIEWEE_ID corresponds to SURVEY_DEPLOYMENT_ID ( id in Survey_Deployments table )
Element | Type | Attributes |
---|---|---|
reviewed_object_id | Integer | Limit: 4 |
reviewer_id | Integer | Limit: 4 |
reviewee_id | Integer | Limit: 4 |
type | String | Limit: 255 |
created_at | Datetime | |
updated_at | Datetime | |
calibrate_to | tinyint | Default: 0 |
Table: Participants
Participants in the survey are present in this table. Parent_id denotes course/assignment id.
( Note - FIELDS THAT ARE RELEVANT TO SURVEY FUNCTIONALITY are mentioned below )
Element | Type | Attributes |
---|---|---|
user_id | Integer | Limit: 4 |
parent_id | Integer | Limit: 4 |
Table: Responses
Responses table contains a map_id which is the id in Response_Maps table
Element | Type | Attributes |
---|---|---|
map_id | Integer | Limit: 4 |
UML Diagram
Tasks performed
The following list provides a summary of the tasks we have performed to implement the survey functionality.
1) Change the hierarchical structure of classes. Make survey questionnaire the child of questionnaire. Global survey questionnaire, Assignment survey questionnaire and course survey questionnaire are subclasses of survey questionnaire.
2) Creation, editing and deletion of questions, is a functionality that would be inherited into class Survey(which is a kind of questionnaire) and so into all its children surveys, from the Questionnaire class.
3) Add a new class called Survey Questionnaire and include functionality to assign(which is in the survey_controller currently) to this model.
4) In the survey deployment class, add functionality to generate statistics.
5) The three kinds of surveys can be assigned to students an assignment, a course, and a global survey which is appended to one of the other two surveys.
6) Use the Pending Surveys (newly added) tab on the Expertiza homepage to display any pending surveys instead of just the course evaluation surveys. The course evaluation tab’s functionality was removed because it was broken, it was replaced with the pending surveys functionality.
7) "Survey deployment" functionality was broken. We made survey deployment of all forms of surveys functional. It included the following tasks:
a) Ability to add new survey deployments. b) View responses to the deployments. c) View statistics (in the form of pie charts) c) Delete deployments.
Test Plan
Testing tool:
RSpec (For the automated tests)
Test # | Type | Description | Steps |
---|---|---|---|
Test case 1: | Automated | Test to check whether an instructor can create a survey |
1. Login to Expertiza as an instructor. 2. Click Manage… and go to Questionnaire. 3. Select new public/private item of the type of survey you want to create. 4. In the Questionnaire view, create the questionnaire by Clicking Create. |
Test case 2: | Manual | Test to check whether a survey is deployed to the appropriate users |
1. After creation of a survey that a particular student can participate in, login as that student. 2. The student can then participate in the survey by clicking on Pending Surveys. 3. If the survey appears there, that indicates that the survey has been deployed successfully. |
Test case 3: | Automated | Test to check whether the statistics of survey responses is displayed |
1. Login to Expertiza as an instructor. 2. Assuming that a survey is created, and students have participated in it, we can check the distribution of results. 3. Click on Survey Deployments in the page header. 4. Click Statistics of a particular survey that was created to view the statistics of responses. |
Test case 4: | Manual | Test to check that only allowed users can participate in the survey |
1. Login to Expertiza as a student that is not included as a participant in the survey. 2. By clicking on Surveys, the list of surveys that the student can currently take is displayed. 3. If the survey that was created is not visible, then the user is correctly excluded. |
Test case 5: | Automated | Test to check whether an instructor can view responses of a survey deployment |
1. Login to Expertiza as an instructor. 2. Assuming that a survey is created, and students have participated in it, the instructor can check the responses to each question. 3. Click on Survey Deployments in the page header. 4. Click View Responses of a particular survey that was created to view the responses. |