CSC/ECE 517 Fall 2018- Project E1858. Github metrics integration
Introduction
Problem Statement
Expertiza provides Teammate Reviews under View Scores functionality for each assignment. Purpose of this project is to augment existing assignment submissions with data that can give a more realistic view of the work contribution of every team member using external tools like GitHub. This external data may include: number of commits, number of lines of code modified, number of lines added, number of lines deleted from each group’s submitted repository link from GitHub.
- 1. Teammate Reviews functionality in the View Scores page gauges teammate views on how much other team members contributed to the project. We need to augment this data with data from external tools like GitHub in order to validate that feedback. New metrics will be appended under each student data under the same functionality.
- 2. Github Metrics under View Submissions page should include a bar chart that shows number of commits by the team throughout the assignment timeline. This will help instructors to get a team overview, and aid grading process.
While this data will not have marks associated directly, it will prove useful to the instructor in differentiating the performance of team members and hence awarding marks as per contribution. Overall data for the team, like the number of committers and number of commits may also help instructors to predict which projects are likely to be merged.
Current Scenario
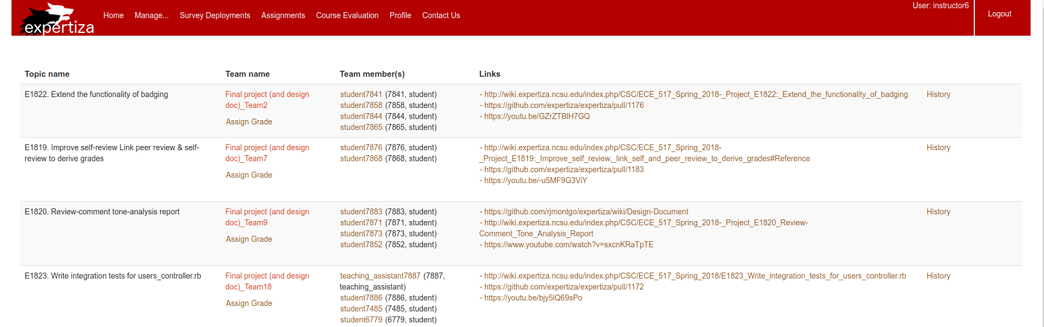
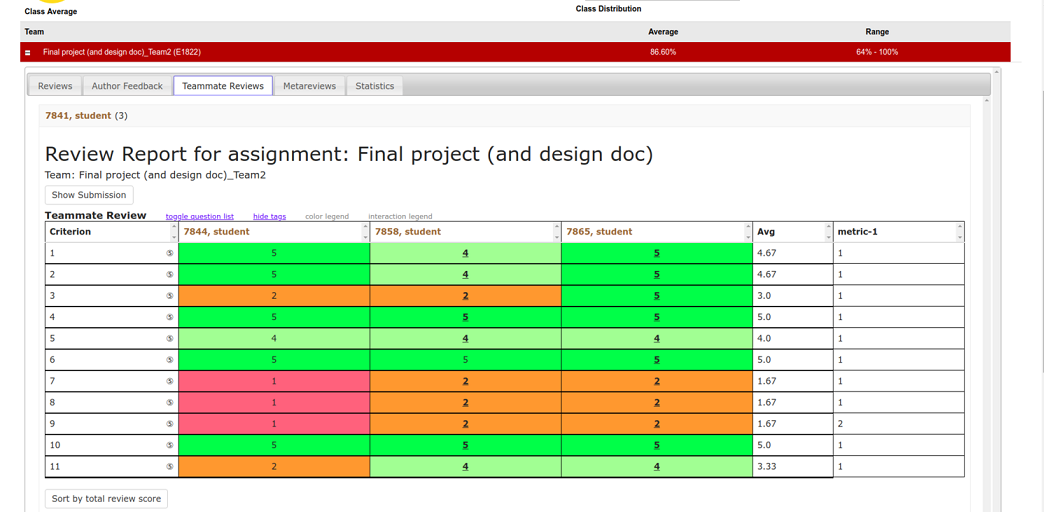
Checking commits performed by each team member on GitHub is a solution, but that is inefficient from instructor's/reviewer's perspective as there are many assignments, submissions, and tight deadlines.
Use Case Diagram
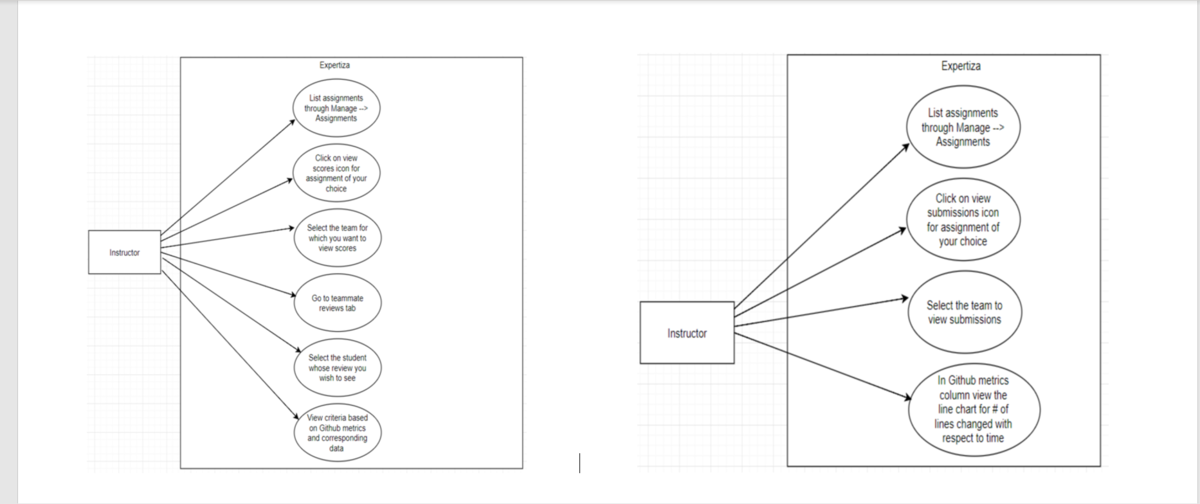
Use Case Diagram Details
Actors:
- Instructor: This actor is responsible for creating assignments and adding students to the assignment.
- Student: This actor is responsible for submitting, self-reviewing, and viewing the scores.
Database:
- The database where all the data of Expertiza is getting stored.
Pre Conditions:
- The Student should submit the assignment and self-review.
- The other students should submit the reviews of the work submitted.
Primary Sequence:
- The student should login.
- The student should browse and upload the assignment.
- The student should submit the assignment.
- The student should submit teammate-reviews.
Post Conditions:
- Instructor will be able to see the team contribution done by each team member in 'View Submissions' page using graph diagrams, as shown in the figure.
- Instructor will be able to see the work done by each student in 'Teammate Review Tab' with new metrics table appended at the end, as shown in the figure.
Design Principles
- MVC – The project is implemented in Ruby on Rails that uses MVC architecture. It separates an application’s data model, user interface, and control logic into three distinct components (model, view and controller, respectively). We intend to follow the same when implementing our end-point for pulling GitHub data.
- Dry Principle – We are trying to reuse the existing functionalities in Expertiza, thus avoiding code duplication. Whenever possible, code modification based on the existing classes, controllers, or tables will be done instead of creating the new one.
Solution Design
- The Github metrics that need to be integrated with Expertiza were finalized as below. These metrics are captured on a per-user basis:
- Total number of commits.
- Number of files changed.
- Lines of Code added
- Lines of code modified.
- Lines of code deleted.
- Pull Request Status ( includes code climate and Travis CI Build status)
- User Github metrics:
- Committer ID
- Committer Name
- Committer email ID
- A new link "Github Metrics" is provided under “View Submissions” for an assignment in the instructor view.This link opens a new tab and shows a stacked bar chart for number of commits per user vs submission timeline from assignment creation date to the deadline.
- In "View Scores" for an assignment in the instructor view, under Teammate Reviews tab, a new table for Github Metrics is added, which shows following Github metrics per user:
- Student Name/ID, Email ID, lines of code added, lines of code deleted, number of commits
- For GitHub integration, we have used GitHub GraphQL API v4. We have used OAuth gem for authentication purpose.
- We parse the link to PR to get data associated with it. We have also handled projects which do not have PR link, but just a link to the repository.
Implemented Solution
Files Modified
- app/controllers/auth_controller.rb
- app/controllers/grades_controller.rb
- app/helpers/grades_helper.rb
- app/views/assignments/list_submissions.html.erb
- app/views/grades/_tabbing.html.erb
- app/views/grades/_teammate_reviews_tab.html.erb
- app/views/grades/view.html.erb
- app/views/grades/view_team.html.erb
- config/application.rb
- config/initializers/load_config.rb
- config/initializers/omniauth.rb
- config/routes.rb
Files Added
- app/views/grades/view_github_metrics.html.erb
- config/github_auth.yml
First Change
- A new table "Github Metrics" is added under Manage-> Assignments -> View Scores -> Teammate Reviews. Below is the screenshot of the implementation.
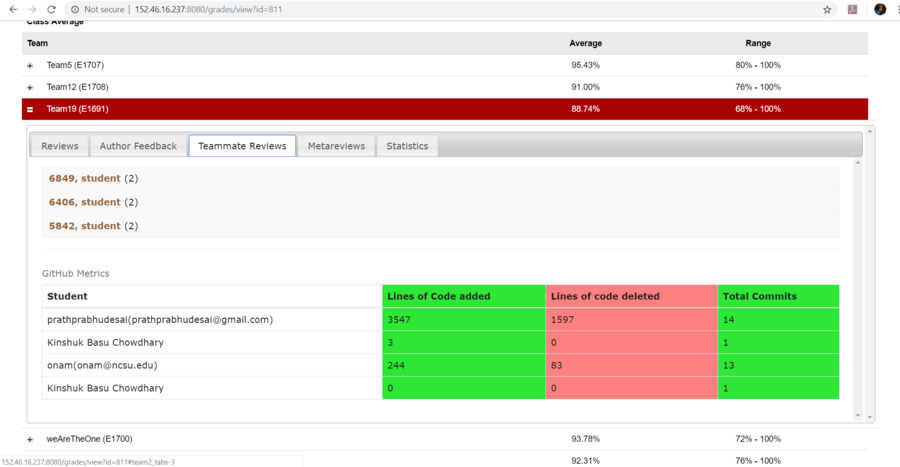
Second Change
- The second change is in the View Submissions page, where we have added a link "Github Metrics" to a new page.
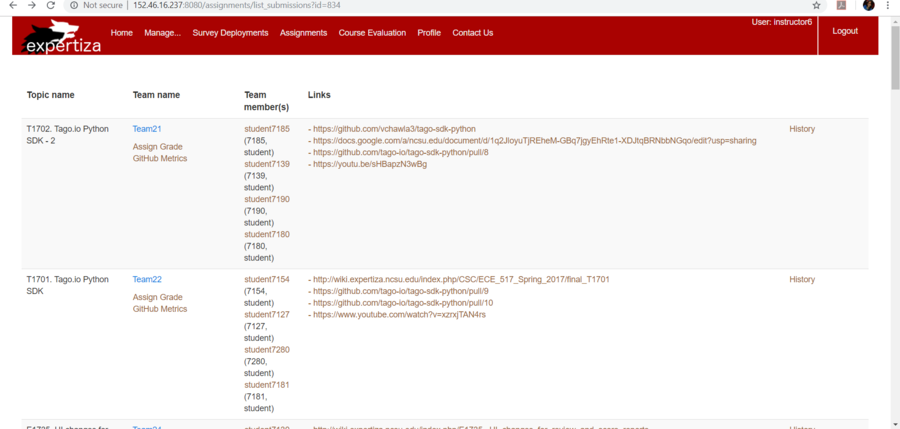
- The new page appears after clicking on the link "Github metrics", that shows bar chart for # of commits per day. We have also added other relevant information about Pull Request, such as total commits, lines of code added, lines of code modified, PR merge status, check status.
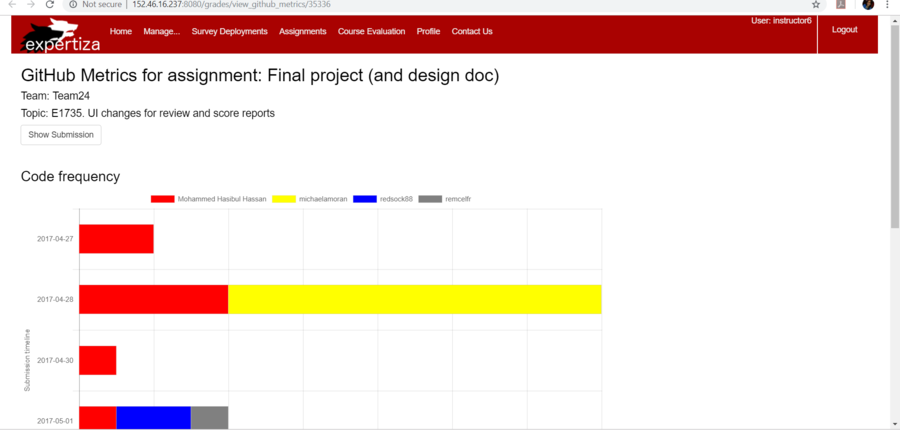
Test Plan
Change 1: GitHub metrics in teammate reviews
1) Log in as an instructor (instructor6/password)
2) Navigate to assignments through Manage --> Assignments
3) Select "View scores" icon for the assignment of your choice
4) Select the team for which you wish to view scores
5) Go to "Teammate Reviews" tab
6) View data per team member based on different GitHub metrics (e.g. lines of code added/changed/removed etc.)
Change 2: Bar chart for # of commits changed by the overall team
1) Log in as an instructor (instructor6/password)
2) Navigate to assignments through Manage --> Assignments
3) Select "View submissions" icon for the assignment of your choice
4) Click on the "Github metrics" link for the team whose metrics you wish to view
5) A new page opens and shows # of commits changed per team member since the start of the assignment
RSpec Tests
- Following feature tests were added to the Grades_Controller_Spec.rb
describe "#get_statuses_for_pull_request" do before(:each) do allow(Net::HTTP).to receive(:get) {"{\"team\":\"rails\",\"players\":\"36\"}"} end it 'makes a call to the GitHub API to get status of the head commit passed' do expect(controller.get_statuses_for_pull_request('qwerty123')).to eq({"team" => "rails", "players" => "36"}) end end describe '#view_github_metrics' do context 'when user hasn\'t logged in to GitHub' do before(:each) do @params = {id: 900} session["github_access_token"] = nil end it 'stores the current participant id and the view action' do get :view_github_metrics, @params expect(session["participant_id"]).to eq("900") expect(session["github_view_type"]).to eq("view_submissions") end it 'redirects user to GitHub authorization page' do get :view_github_metrics, @params expect(response).to redirect_to(authorize_github_grades_path) end end end describe '#view' do context 'when user hasn\'t logged in to GitHub' do before(:each) do @params = {id: 900} session["github_access_token"] = nil end it 'stores the current assignment id and the view action' do get :view, @params expect(session["assignment_id"]).to eq("900") expect(session["github_view_type"]).to eq("view_scores") end it 'redirects user to GitHub authorization page' do get :view, @params expect(response).to redirect_to(authorize_github_grades_path) end end end
Change-log for Reviewers
This section will be removed in the final draft. It is just here for convenience of reviewers to know which sections were majorly updated from last review.
- Added Solution Design for the final implemented design
- Added Implemented Solution to show feature additions to Expertiza
- Added Feature test cases for Grades controller