CSC/ECE 517 Fall 2012/ch2b 2w37 ms
Adapter pattern and the related patterns (Bridge, Decorator, Facade)
The adapter design pattern allows the user to make changes to the existing class with other class libraries without changing the code for the existing class. The Bridge, Decorator and the Facade pattern look somewhat similar to the adapter pattern but their intent is different and that intent is what separates the above patterns from each other.
Adapter Pattern
Adapter pattern plays an important role when you want to incompatible interfaces to work together.The real world example for an adapter pattern is the travel power adapter.Different countries have different socket and plug configuration. So you can use an adapter to fit a plug into a socket that initially was not possible due to different interface designs.
Implementattion
Adapter implementation using inheritance
This method can be used when you have incompatible method that needs to be used in other class. Using inheritance a "is-a" relationship is established between the base class and super class. The new compatible methods will be contained in the inherited adapter class.
public class CylindricalSocket { public String supply(String cylinStem1, String cylinStem1) { System.out.println("Power power power..."); } } public class RectangularAdapter extends CylindricalSocket { public String adapt(String rectaStem1, Sting rectaStem2) { //some conversion logic String cylinStem1 = rectaStem1; String cylinStem2 = rectaStem2; return supply(cylinStem1, cylinStem2); } } public class RectangularPlug { private String rectaStem1; private String rectaStem2; public getPower() { RectangulrAdapter adapter = new RectangulrAdapter(); String power = adapter.adapt(rectaStem1, rectaStem2); System.out.println(power); } }
Adapter implementation using composition
The other approach is to have a base class as an attribute in the adapter class. This creates an "has-a"relationship between the base class and the sub class.
public class CylindricalSocket { public String supply(String cylinStem1, String cylinStem1) { System.out.println("Power power power..."); } } public class RectangularAdapter { private CylindricalSocket socket; public String adapt(String rectaStem1, Sting rectaStem2) { //some conversion logic socket = new CylindricalSocket(); String cylinStem1 = rectaStem1; String cylinStem2 = rectaStem2; return socket.supply(cylinStem1, cylinStem2); } } public class RectangularPlug { private String rectaStem1; private String rectaStem2; public getPower() { RectangulrAdapter adapter = new RectangulrAdapter(); String power = adapter.adapt(rectaStem1, rectaStem2); System.out.println(power); } }
Composition or inheritance to implement Adapter Pattern?
- Composition is preferred over inheritance since using composition it is easy to change the behavior of a class.
Adapter pattern in Java Library
- java.io.InputStreamReader(InputStream)
- java.io.OutputStreamWriter(OutputStream)
Bridge Pattern
As stated by GoF a bridge design patterns intent is to “Decouple an abstraction from its implementation so that the two can vary independently”.
Elements of Bridge Design Pattern
- Abstraction: This is where the abstraction interface is defined.
For example: A Vehicle class which has a manufacture method.
- Refined Abstraction: Refined Abstraction extends the interface defined by Abstraction.
For example, a Car class or a Bike class which has a manufacture method which is more refined than the Vehicle class.
- Implementor: It defines the interface for the implementation classes. It defines the basic operation.
For example, the Workshop class acts as an implementor with the work() method.
- Concrete Implementation: This implements the Implementor interface and gives it a more concrete implementation.
For example the Produce and the Assemble class with the work() method provides the concrete implementation.
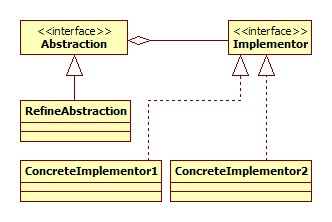
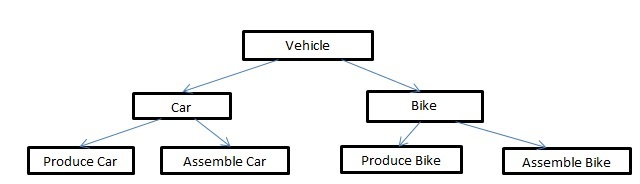
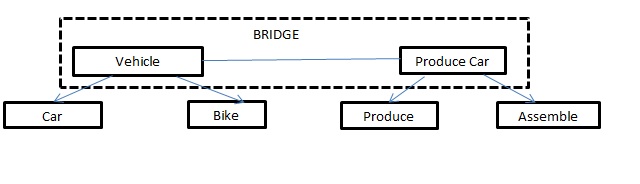
Example code for Bridge pattern
Vehicle Interface
// abstraction in bridge pattern abstract class Vehicle { protected Workshop workShop1; protected Workshop workShop2; protected Vehicle(Workshop workShop1, Workshop workShop2) { this.workShop1 = workShop1; this.workShop2 = workShop2; } abstract public void manufacture(); }
Car class
//Refine abstraction 1 in bridge pattern public class Car extends Vehicle { public Car(Workshop workShop1, Workshop workShop2) { super(workShop1, workShop2); } @Override public void manufacture() { System.out.print("Car "); workShop1.work(); workShop2.work(); } }
Bike Class
// Refine abstraction 2 in bridge pattern public class Bike extends Vehicle { public Bike(Workshop workShop1, Workshop workShop2) { super(workShop1, workShop2); } @Override public void manufacture() { System.out.print("Bike "); workShop1.work(); workShop2.work(); } }
Workshop Interface
// Implementor for bridge pattern public interface Workshop { abstract public void work(); }
Produce Class
// Concrete implementation 1 for bridge pattern public class Produce implements Workshop { @Override public void work() { System.out.print("Produced"); } }
Assemble Class
//Concrete implementation 2 for bridge pattern
public class Assemble implements Workshop { @Override public void work() { System.out.println(" Assembled."); } }
Demonstration of bridge design pattern
public class BridgePattern { public static void main(String[] args) { Vehicle vehicle1 = new Car(new Produce(), new Assemble()); vehicle1.manufacture(); Vehicle vehicle2 = new Bike(new Produce(), new Assemble()); vehicle2.manufacture(); } }
Output:
Car Produced Assembled. Bike Produced Assembled.
Bridge vs Adapter pattern
- Two incompatable classes can be made to work together using adapter pattern.
- Bridge pattern creates two separate hierarchies by separating the abstraction from the implementation.
Decorator Pattern
The decorator pattern adds responsibility to an object dynamically. The decorator design pattern is used to extend the behavior of an object dynamically. In order to extend the behavior we have to construct an wrapper around the object. Inheritance is not feasible since it is applied to an entire class. With the decorator pattern it is possible to select any particular instance and modify its behavior leaving the other instances unmodified.
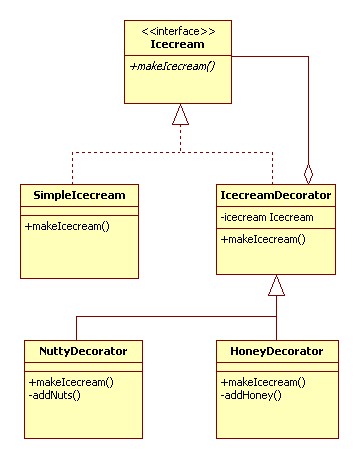
Implementation of Decorator Pattern
First start with the interface which will be used by the class having the decoration design. The example below explains how you first create a base ice cream and then decorate that ice cream by adding new toppings. The added topping changes the behavior of that ice cream.
The interface below contains an makeIcecream() method which has not been implemented yet.
public interface Icecream { public String makeIcecream(); }
The SimpleIceCream Class provides an implementation to the makeIcecream() method. This is the class which acts as a base class on which decorations will be added.
public class SimpleIcecream implements Icecream { @Override public String makeIcecream() { return "Base Icecream"; } }
The class below is the crux of the design pattern. Here is where all the Decoration is actually provided to an instance of the object.It contains an attribute of type Icecream. Once the instance is assigned using the constructor the instance method will be invoked.
abstract class IcecreamDecorator implements Icecream { protected Icecream specialIcecream; public IcecreamDecorator(Icecream specialIcecream) { this.specialIcecream = specialIcecream; } public String makeIcecream() { return specialIcecream.makeIcecream(); } }
The NuttyDecorator and HoneyDecorator are the two concrete classes that implement the abstract decorator class IcecreamDecorator.
public class NuttyDecorator extends IcecreamDecorator { public NuttyDecorator(Icecream specialIcecream) { super(specialIcecream); } public String makeIcecream() { return specialIcecream.makeIcecream() + addNuts(); } private String addNuts() { return " + cruncy nuts"; } }
public class HoneyDecorator extends IcecreamDecorator { public HoneyDecorator(Icecream specialIcecream) { super(specialIcecream); } public String makeIcecream() { return specialIcecream.makeIcecream() + addHoney(); } private String addHoney() { return " + sweet honey"; } }
Decorator pattern in Java Library
- java.io.BufferedReader;
- java.io.FileReader;
- java.io.Reader;
Adaptor Pattern vs Decorator Pattern
- Adapter provides a different interface to its subject.
- Decorator provides an enhanced interface.
http://sourcemaking.com/design_patterns/decorator
Facade Pattern
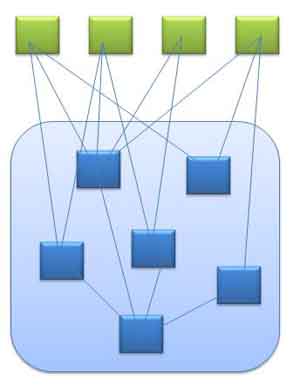
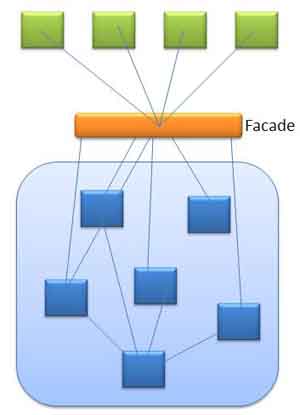