CSC/ECE 517 Fall 2012/ch1 1w17 ir: Difference between revisions
(→Ruby) |
No edit summary |
||
Line 25: | Line 25: | ||
Here is an example that shows how Object.extend(module) adds the methods defined in a module at the object's level. | Here is an example that shows how Object.extend(module) adds the methods defined in a module at the object's level. | ||
<pre> | <pre> | ||
module | module RubyExample | ||
def helloWorld | def helloWorld | ||
"Hello user! Welcome to Ruby!" | "Hello user! Welcome to Ruby!" | ||
Line 36: | Line 36: | ||
<pre> | <pre> | ||
obj = Object.new | obj = Object.new | ||
obj.extend( | obj.extend(RubyExample) | ||
obj.helloWorld #=> "Hello user! Welcome to Ruby!" | obj.helloWorld #=> "Hello user! Welcome to Ruby!" | ||
</pre> | |||
As mentioned earlier, everything in Ruby is an object. The following confirms this fact: | |||
<pre> | |||
>> RubyExample.object_id | |||
=> 45097 | |||
>> RubyExample.class | |||
=> Module | |||
>> RubyExample.respond_to?(:extend) | |||
=> true | |||
</pre> | |||
Now this is not the only way to extend object functionality in Ruby. We can also do the same thing by | |||
<pre> | |||
RubyExample = Module.new do | |||
def helloWorld | |||
"Hello user! Welcome to Ruby!" | |||
end | |||
end | |||
RubyExample.extend(RubyExample) | |||
RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!" | |||
</pre> | |||
So, the above alternative method clarifies the fact that RubyWorld is just an instance of the class Module. We can also do the same thing by including the extend() call within the module definition. | |||
<pre> | |||
module RubyExample | |||
extend RubyExample | |||
def helloWorld | |||
"Hello user! Welcome to Ruby!" | |||
end | |||
end | |||
RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!" | |||
</pre> | |||
In Ruby, the keyword 'self' refers to the current or default object itself. So another way of implementing the above is as follows | |||
<pre> | |||
module RubyExample | |||
extend self | |||
def helloWorld | |||
"Hello user! Welcome to Ruby!" | |||
end | |||
end | |||
RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!" | |||
</pre> | </pre> | ||
Revision as of 20:34, 8 September 2012
Extending Objects
The ‘extend’ feature in computer programming languages allow the programmer to dynamically extend the functionality of an object at runtime, as opposed to extending functionality at compile time. This feature can be found in Object Oriented Programming (OOP) Languages.
Introduction
The programming paradigm OOP uses 'objects' for designing applications. These objects consist of an encapsulated set of data fields and methods, and are usually an instance of a class. These objects can communicate with each other through messages (or 'methods') and process data in object oriented programming. But in order to increase an object's functionality, it is necessary to add the features of one object to another. This can be done dynamically at run time, without repeating the code, by extending the features of one class to another. This can be done by the use of the 'extend' keyword. Many OOP oriented languages support the 'extend' feature.
An object is an entity that serves as a container for data and also controls access to the data. Associated with an object is a set of attributes, which are essentially no more than variables belonging to that object. Also associated with an object is a set of functions that provide an interface to the functionality of the object, called methods. - Hal Fulton[2]
Languages Using 'extend' Functionality
C++
Example Code Snippet
Java
Example Code Snippet
Ruby
Ruby is a OOP language. It differs from the previous two OOP languages mentioned above in how it interprets what 'object-oriented' means and the different terminologies for the concepts it employs.
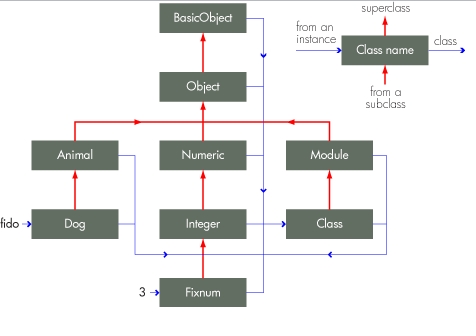
In Ruby, everything is an object and all objects are instances of a class. Even classes are instances of class objects. So when there is a class, .new is called to get an instance of the class and when there is an instance, .class is called to obtain its class [3]. So each class has a superclass and if it doesn't specify any class from which it inherits, then it inherits directly from the Object class.
Example Code Snippet
Here is an example that shows how Object.extend(module) adds the methods defined in a module at the object's level.
module RubyExample def helloWorld "Hello user! Welcome to Ruby!" end end
Now, we can extend this module's functionality (i.e, the Greet method) to any object we instantiate. Below we can see that the module's methods have been mixed in to the object.
obj = Object.new obj.extend(RubyExample) obj.helloWorld #=> "Hello user! Welcome to Ruby!"
As mentioned earlier, everything in Ruby is an object. The following confirms this fact:
>> RubyExample.object_id => 45097 >> RubyExample.class => Module >> RubyExample.respond_to?(:extend) => true
Now this is not the only way to extend object functionality in Ruby. We can also do the same thing by
RubyExample = Module.new do def helloWorld "Hello user! Welcome to Ruby!" end end RubyExample.extend(RubyExample) RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!"
So, the above alternative method clarifies the fact that RubyWorld is just an instance of the class Module. We can also do the same thing by including the extend() call within the module definition.
module RubyExample extend RubyExample def helloWorld "Hello user! Welcome to Ruby!" end end RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!"
In Ruby, the keyword 'self' refers to the current or default object itself. So another way of implementing the above is as follows
module RubyExample extend self def helloWorld "Hello user! Welcome to Ruby!" end end RubyExample.helloWorld #=> "Hello user! Welcome to Ruby!"
Python
Example Code Snippet
Advantages
Disadvantages
Conclusion
See also
References
<references/>