CSC/ECE 517 Fall 2010/chd 6d isb: Difference between revisions
No edit summary |
No edit summary |
||
Line 16: | Line 16: | ||
Here, The Abstract Vehicle Interface is an example of a "fat interface". One of the side-effects of fat interfaces is that any class that implements the interface ends up doing too many things and this can clearly violate the [http://en.wikipedia.org/wiki/Single_responsibility_principle Single Responsibility Principle]. | Here, The Abstract Vehicle Interface is an example of a "fat interface". One of the side-effects of fat interfaces is that any class that implements the interface ends up doing too many things and this can clearly violate the [http://en.wikipedia.org/wiki/Single_responsibility_principle Single Responsibility Principle]. | ||
==ISP for " Fat Interfaces"== | ==ISP for " Fat Interfaces "== | ||
By breaking interfaces down into smaller chunks, we potentially reduce the responsibilities of a class and thus provide higher cohesion between the methods of a particular interface. This also provides the benefit of making a clear statement to consumers of the interface of what minimum functionality is required to be implemented: everything. There uncertainty of whether a particular method or property needs to be implemented vanishes, reducing the factor of surprise. The Vehicle interface could be decomposed into ForwardMovingVehicle and BackwardMovingVehicle. If there are classes that require both functionalities, they would implement both interfaces. Alternatively, if only one interface were required, for example for Aircraft, then only the ForwardMovingVehicle interface would be implemented, avoiding giving way to empty declarations with NotImplementedException exceptions as their implementation. | By breaking interfaces down into smaller chunks, we potentially reduce the responsibilities of a class and thus provide higher cohesion between the methods of a particular interface. This also provides the benefit of making a clear statement to consumers of the interface of what minimum functionality is required to be implemented: everything. There uncertainty of whether a particular method or property needs to be implemented vanishes, reducing the factor of surprise. The Vehicle interface could be decomposed into ForwardMovingVehicle and BackwardMovingVehicle. If there are classes that require both functionalities, they would implement both interfaces. Alternatively, if only one interface were required, for example for Aircraft, then only the ForwardMovingVehicle interface would be implemented, avoiding giving way to empty declarations with NotImplementedException exceptions as their implementation. | ||
Line 24: | Line 24: | ||
= | ==Interface Pollution== | ||
Interface Pollution term is used interchangeable with Fat Interfaces. Whenever an interface is added to base class, that base class does not need, base class is polluted with that interface. In other words, base class' interface has been made "fat". | |||
=ISP in Action= | |||
Consider the following example in order to better understand The Interface Segregation Principle. A Security system includes Door objects and Door class has the following methods: | |||
[[Image:Interface1.png |center| Door Class]] | |||
=Distributed Revision Control= | =Distributed Revision Control= |
Revision as of 03:05, 11 November 2010
Introduction
The Interface Segregation Principle (ISP) focuses on the cohesiveness of interfaces with respect to the clients that use them. ISP is very similar to high cohesion principle of GRASP. ISP helps developers to change, refactor and redeploy their code easily. This principle alleviates the disadvantages of "fat" or "polluted" interfaces. [1] ISP is considered as the eye of the SOLID design principle. Two main ISP guidelines are: Classes should not be forced to depend on methods that they do not use, and the dependency of one class to another one should depend on the smallest possible interface. [3]
Brief Introduction to Interfaces in OOP
Interface separates the implementation and defines the structure, and this concept is very useful in cases where you need the implementation to be interchangeable. Apart from that an interface is very useful when the implementation changes frequently. Interface can be used to define a generic template and then one or more abstract classes to define partial implementations of the interface. Generally, interfaces are used as a group of related methods with empty bodies. Interfaces form a contract between the class and the outside world, and this contract is enforced at build time by the compiler. Interfaces are widely used in Object Oriented Languages such as Java, C# and C++.
Fat Interfaces
As stated above, ISP is used to overcome the problems introduced by "fat interfaces". In order to clarify this term, consider the example given below.
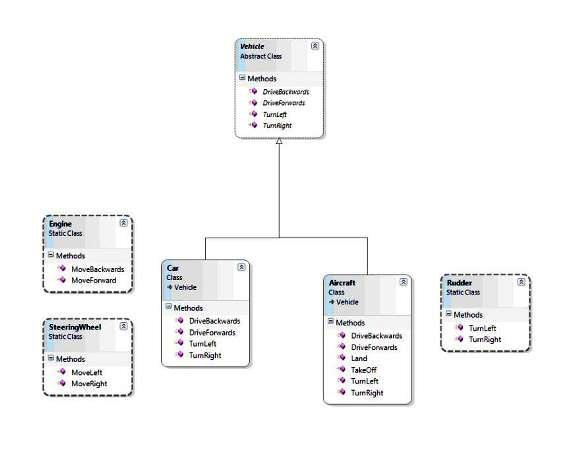
Cars, Motorcycles and/or Aircrafts classes implements the Abstract Vehicle interface that defines methods like: TurnLeft, TurnRight, DriveForwards and DriveBackwards. All these methods are going to be used by the classes which implements this interface. There will not be any problems if Cars class implements this interface, since every car turns left, turns right, drives forwards and drive backwards. Assume that Aircraft class also implements Vehicle Interface. Any aircraft can turn left, turn right and drive forwards. On the other hand, no aircraft can drive backwards by itself. Another example: if Motorcycle class implements Vehicle abstract interface, it has to use DriveBackwards method, although it cannot drive backwards by itself. Here, The Abstract Vehicle Interface is an example of a "fat interface". One of the side-effects of fat interfaces is that any class that implements the interface ends up doing too many things and this can clearly violate the Single Responsibility Principle.
ISP for " Fat Interfaces "
By breaking interfaces down into smaller chunks, we potentially reduce the responsibilities of a class and thus provide higher cohesion between the methods of a particular interface. This also provides the benefit of making a clear statement to consumers of the interface of what minimum functionality is required to be implemented: everything. There uncertainty of whether a particular method or property needs to be implemented vanishes, reducing the factor of surprise. The Vehicle interface could be decomposed into ForwardMovingVehicle and BackwardMovingVehicle. If there are classes that require both functionalities, they would implement both interfaces. Alternatively, if only one interface were required, for example for Aircraft, then only the ForwardMovingVehicle interface would be implemented, avoiding giving way to empty declarations with NotImplementedException exceptions as their implementation. If we apply ISP to the above example, new "thin" interfaces would be like below.
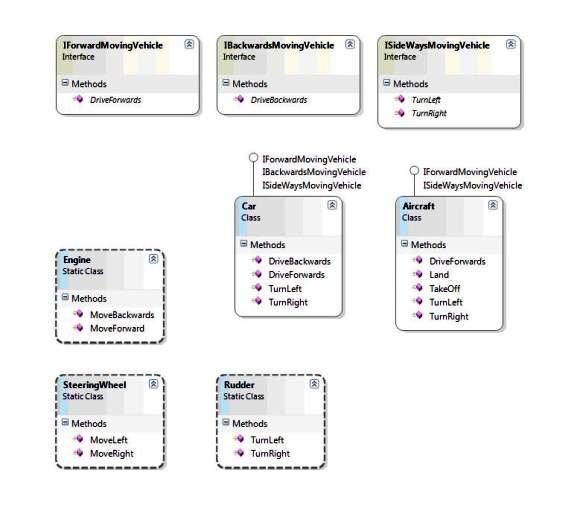
Interface Pollution
Interface Pollution term is used interchangeable with Fat Interfaces. Whenever an interface is added to base class, that base class does not need, base class is polluted with that interface. In other words, base class' interface has been made "fat".
ISP in Action
Consider the following example in order to better understand The Interface Segregation Principle. A Security system includes Door objects and Door class has the following methods:
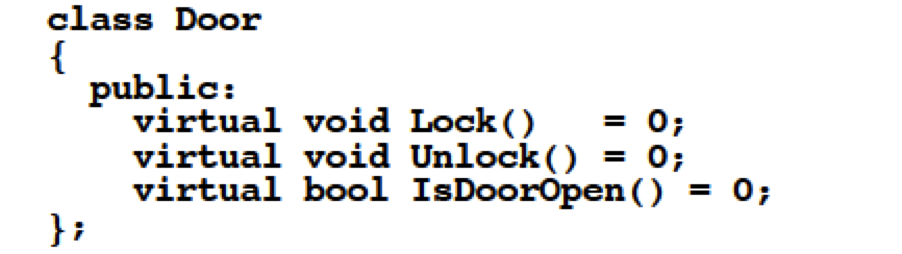
Distributed Revision Control
The first truly distributed version control system was the BitKeeper, which was developed by Linus Torvalds in December 1999. Distributed revision control (DVCS) is a fairly new concept in revision control. With it's peer-to-peer approach, each peer's copy of the code base is a bona-fide repository. It synchronizes by exchanging patches between peers. It's advantages over centralized revision control include [16][17]:
- By default, only the working copy of the code base exist.
- Since, communication with a central server is not required, common operations are much more faster. Peers need to communicate only when pushing or pulling changes with other peers.
- Each working copy exists as a remote backup of the code base.
Open systems are the most recent phenomenon in distributed revision control. They are characterized by their support for independent branches, and heavy reliance on merge operations. It is generally characterized by the following features -
- Peers are free to join as and when they wish without going through any elaborate approval process
- Each working copy works like a branch
- Selective changes can be "cherry-picked", pulling them from specific peers.
One of the first closed source DVCS was the Sun WorkShop TeamWare which was widely used in enterprise settings.[18]
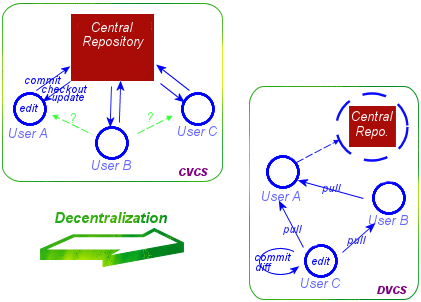
Bazaar is one of the most famous open distributed style tree versioning tool. In addition to Bazaar (Mar,2005), many other distributed version control software are available. They include: Darcs (Nov, 2004), Monotone(Apr, 2003), Mercurial(Apr, 2005), Git(Apr, 2005).
Comparison of version control systems can be found here.
Summary
The Interface Segregation Principle is very simple at heart and although it states something that would seem somewhat obvious, it is many times ignored. By abiding to ISP, we can improve the quality of our code by making sure that we not only comply with the exact requirements of an interface by implementing everything defined in the contract, but we also aim for reducing the responsibilities of a class by minimizing an interface, and thus complying with the Single Responsibility Principle.
References
[1] The Interface Segregation Principle, Engineering Notebook for C++, 1996.
[2] Interface Segregation Principle, Retrieved April, 2010.
[3] Interface Segregation Principle, Ravij Narula, 2010.
[4] What is an Interface? The Java Tutorials by Oracle, 2010. [5] SVN vs CVS, 2005. Retrieved September, 2010.
[6] Neary, David, Subversion Building a better CVS.Linux Magazine, (30), 59-63.
[7] Amitash, Difference between CVS and Subversion. Retrieved September, 2010.
[8] Apache Subversion, 2010. Retrieved September, 2010.
[9] Revision control, 2010. Retrieved September, 2010.
[10] Distributed revision control, 2010. Retrieved September, 2010.
[11] Auvray S., Distributed Version Control Systems: A Not-So-Quick Guide Through, May 07, 2010