CSC/ECE 517 Fall 2009/wiki2 2 pz
Introduction to AJAX
AJAX was first coined by Jesse James Garrett of Adaptive Path in February 2005 in his essay "Ajax: A New Approach to Web Applications”. Ajax (asynchronous JavaScript and XML) is more of a technique than it is a specific technology. Its greatest advantage in web browser is to enable the client to request data from server asychronously without reloading the page. When you submit data using an Ajax-powered form, the server returns an HTML fragment that contains the server's response and displays only the data that is new or changed as opposed to refreshing the entire page. This technology makes Internet applications smaller, faster and more user friendly.
Another real strength of AJAX is that developers don’t need to learn some new language or scrap their existing investment in server-side technology. Ajax is a client-side approach and can interact with J2EE, .NET, PHP, Ruby, and CGI scripts—it really is server agnostic. Short of a few minor security restrictions, you can start using Ajax right now, leveraging what you already know.
AJAX has many applications. One of the most popular one is the google map. Using the conventional web method, when user want to magnify the map or look around, every time when he moves the map, there will be a reloading “white screen”, it’s unacceptable for the users. With AJAX, while looking around in google map, the request for data could be processed asynchronously, this feature could make the page uninterrupted and continuous.
Introduction to MVC
Model–view–controller(MVC) obviously is consist of three parts: model, view and controller. Each of them is somehow independent from each other; meanwhile they have some kind of connections with each other.
- Model is the main logic domain of an application, storing data and defining means of how to change and process the data. The state of model can be access under the request by view, and can be change by the controller. One model could have multiple views and controllers.
- View presents the state of model and data to the outer world, and could be changed by the controller. View could access the data of model freely; however, it could not change the model. The view should change when the corresponding model changes.
- Controller is the method for the user to control the application. The controller acts as the input to the application, it changes the model and as the user requests. The controller is also responsible for creating and selecting views which sometimes is delegated to a specific object; this is known as the Application Controller pattern for web MVC and View Handler for GUI MVC.
AJAX Applications in MVC
Java

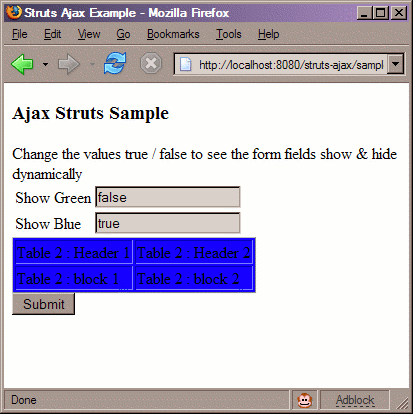
If the MVC architecture is implemented by using Java, the following Java technologies can be applied to various components of the MVC pattern.
- Model: The Model part can be implemented using the entity bean and session bean. It also can be implemented by a Java Servlet using a business object framework such as Spring.
- View: The View part can be created by a JavaServer Page (JSP) using JavaServer Faces (JSF) Technology.
- Controller: The Controller part can be simply implemented by a Java Servlet.
For example, Struts is an open-source web application framework for developing Java EE web applications using MVC architecture [1]. Building AJAX in Struts will be a good help for the developers to implement dynamic web pages, but with most of the application running in Java on the web server.
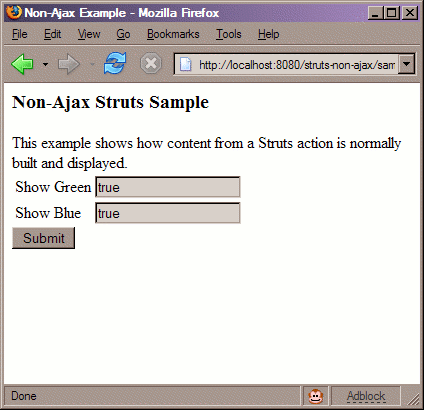
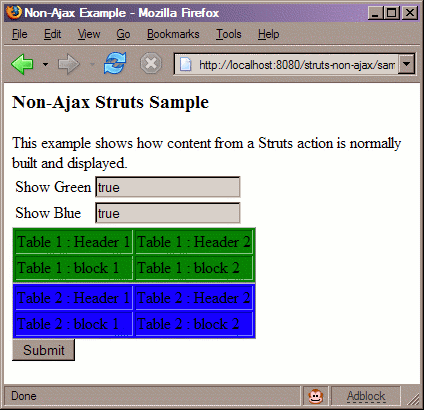
Here is an example which shows that how AJAX will change and help the application in Struts.
This application is based on the sample applications provided with Struts. The user can enter values (true or false) to choose either hiding or display blue and green tables. Figure 1 shows the screen on initial page load. Figure 2 shows the screen after the user has entered values and pressed “Submit” button.
Now, if the user wants to hide the green table, the user has to enter “false” in the “Show green” field, and then press “Submit” button.
Now take a look at the Figures 3 and 4 below. The user doesn’t need to press the “Submit” button to submit the decision. The user only needs to change the value in the textbox, because the screen automatically updates without the screen going blank, giving the result. (Figure 4)
For more information, please see [2].
ASP.NET
In general, AJAX makes an asynchronous request to the web server through XMLHttpRequest. A piece of JavaScript code prepares the call, runs it asynchronously, and then processes the response in a callback function which is responsible for updating the user interface with downloaded data using the DOM services. To make convenience for developers, Microsoft provided such facilities in ASP.NET AJAX as the partial rendering API and JavaScript proxy for Web services.
The package that the ASP.NET MVC install includes the jQuery library which makes up AJAX calls that hide the nitty-gritty details of the XMLHttpRequest object with their own API, so the user can use this library to prepare your direct AJAX calls directed at URLs of choice. This is the most natural way of having AJAX in ASP.NET MVC applications. However, Microsoft provides facilities to make AJAX happen in a way that is similar to partial rendering in WebForms.
There’s only one way of placing AJAX calls, and it’s all about using the XMLHttpRequest object. On top of this, some frameworks and JavaScript libraries have built their own infrastructure, with the sole purpose of making programming easier and faster.
Here is an example[3].
PHP
Usually, the AJAX application flow using PHP should be:
- There is an HTML page which is styled with CSS.
- The user will click on some buttons to submit something.
- JavaScript sends request to the server (to a PHP script).
- JavaScript updates the original HTML page without refresh the page.
Mapping these processes to MVC architecture should be:
- Model: This is the PHP script which is the business logic, writing something to a database and so on.
- View: The HTML page which is styled with CSS and the JavaScript that updates the page.
- Controller: There should be two controllers. One is on the server side and the other is on the client side. The first one is a PHP script that receives requests and asks the Model for the response. The second one is the JavaScript that decides what happens on an action of a button or something and sends an appropriate AJAX request to the PHP controller.
For example, the features which Akelos PHP Framework ports from Rails are listing below:
Active Record (Model):
- Associations (belongs_to, has_one, has_many, has_and_belongs_to_many)
- Finders (not so cool as Ruby on Rails but you can still do $Project->findFirstBy('language AND start_year:greater', 'PHP', '2004'); )
- Acts as (tree, nested_set, list)
- Callbacks
- Transactions
- Validators
- Lockings
- Observers
- Versioning
- Scaffolds
- Support for MySQL, PostgreSQL and SQLite (might work with other databases supported by ADOdb)
Action Controller:
- Filters
- Pagination
- Helpers
- Mime Type
- Mime Response
- Code Generation
- Flash messages
- URL Routing
- Response handler
- Url rewriter
Action View:
- Templates (using Sintags, plain PHP or your favorite engine)
- Web 2.0 javascript using prototype and script.aculo.us
- Helpers
- Partials
- Template Compilers
For more information, please see [4].
Ruby on Rails
In Ruby on Rails, the application’s work is divided into three parts which are separate but cooperative by the Model-View-Controller pattern.
- ActiveRecord (Model): The Model part is built in ActiveRecord library which provides an interface and binds together the tables in a relational database and the Ruby program code which manipulates database records. The field names of database tables can automatically generate Ruby method names, and so on.
- ActionView (View): The View is built in ActionView library. This library is an Embedded Ruby based system for data presentation by providing corresponding templates. If there is a Web connection to the Rails application, it will result in the displaying of a view.
- ActionController (Controller): The Controller part is built in ActionController. It is a data agent to translate interactions with ActionView which is the presentation engine into actions to be performed by ActiveRecord which is the database interface.
In summary, ActiveRecord provides a range of programming methods and paths for manipulating data from an SQL database. ActionController and ActionView provide the platform for controlling and displaying that data. Rails is responsible for tying them all together. The cookbook of Ruby on Rails can be a good example for this.[5]
In rails, once the browser has rendered and displayed the initial web page, different user actions cause it to trigger an Ajax operation or display a new web page like refresh.
Here is a simple sequence of activities:
- User action triggers This user action could be a click on a button, typing user id, address, or pointing to a movie post to find out the detail information about the movie.
- Server called by web client The web client sends data associated with the trigger to an action handler on the server. The data might be either the ID of a checkbox, the text in an entry field, or a whole form.
- The server does processing The rails server does something with the data and returns an HTML fragment to the web client.
- The client updates the response from server The client-side JavaScript, which Rails creates automatically, receives the HTML fragment and uses it to update a specified part of the current page's HTML.
To get Ajax support in the Rails application, you need to include the necessary JavaScript files in the layout. Rails is bundled with several libraries that make using Ajax very easy. Two libraries prototype and script.aculo.us are very popular.
To add Prototype and script.aculo.us support to the application, open up the standard.html.erb layout file in app/views/layouts, add the following line just before the </head> tag, and save your changes:
<%= javascript_include_tag :defaults %>
This includes both the Prototype and script.aculo.us libraries in the template so their effects will be accessible from any of the views.
Now add the following code at the bottom of app/views/subject/list.html.erb
<p id="add_link"><%= link_to_function("Add a Subject", "Element.remove('add_link'); Element.show('add_subject')")%></p> <div id="add_subject" style="display:none;"> <% form_remote_tag(:url => {:action => 'create'}, :update => "subject_list", :position => :bottom, :html => {:id => 'subject_form'}) do %> Name: <%= text_field "subject", "name" %> <%= submit_tag 'Add' %> <% end %> </div>
For more information, please see[6].
References
Fundamentals of Ajax by Ryan Asleson and Nathaniel T. Schutta.
Ajax (programming) Wikipedia.
Model–view–controller Wikipedia.
Ajax programming with Struts 2 by Oleg Mikheev.
AjaxStruts Struts Wiki.
ASP.NET MVC Framework Wikipedia.
Learn ASP.NET MVC Microsoft ASP.NET website.
AJAX MVC by Stoyan Stefanov.
Building With Ajax and Rails Webmonkey.